The bubbling event is to click on the child node, which will trigger the click event of the parent node and ancestor node upwards.
In our usual development process, we will definitely encounter the situation of wrapping a div in a div (this div can be an element). However, events are added to both divs. If we click on the div inside, we We want to handle the events of this div, but we don't want the events of the outer div to be executed as well. At this time, we need to prevent bubbling.
To put it in layman’s terms, you are watching TV at home and hiding in your own small room, but you don’t want the sound to reach the ears of your parents next door. At this time, you may be hiding under the quilt, or the sound insulation effect of the wall is very poor. Well, blocking sound can be understood as blocking bubbling.
<style> #content{ width: 140px; border: 1px solid blue; } #msg{ width: 100px; height: 100px; margin: 20px; border: 1px solid red; } </style> <body> <div id="content"> 外层div <div id="msg"> 内层div </div> </div> </body>
Display results
The corresponding jQuery code is as follows:
<script type="text/javascript" src="js/jquery-1.8.3.js"></script> <script type="text/javascript"> $(function(){ // 为内层div绑定click事件 $("#msg").click(function(){ alert("我是小div"); }); // 为外层div元素绑定click事件 $("#content").click(function(){ alert("我是大div"); }); // 为body元素绑定click事件 $("body").click(function(){ alert("我是body"); }); }); </script>
When the small div is clicked, the click event of the large div and body will be triggered. When the large div is clicked, the click event of the body will be triggered.
How to prevent this kind of bubbling event from happening?
Modify as follows:
<script type="text/javascript" src="js/jquery-1.8.3.js"></script> <script type="text/javascript"> $(function(){ // 为内层div绑定click事件 $("#msg").click(function(event){ alert("我是小div"); event.stopPropagation(); // 阻止事件冒泡 }); // 为外层div元素绑定click事件 $("#content").click(function(event){ alert("我是大div"); event.stopPropagation(); // 阻止事件冒泡 }); // 为body元素绑定click事件 $("body").click(function(event){ alert("我是body"); event.stopPropagation(); // 阻止事件冒泡 }); });
event.stopPropagation(); // Prevent events from bubbling
Sometimes clicking the submit button will have some default events. For example, jump to another interface. But if it does not pass verification, it should not jump. At this time, you can set event.preventDefault(); //Prevent the default behavior (form submission).
html part
<body> <form action="test.html"> 用户名:<input type="text" id="username" /> <br/> <input type="submit" value="提交" id="sub"/> </form> </body>
<script type="text/javascript" src="js/jquery-1.8.3.js"></script> <script type="text/javascript"> $(function(){ $("#sub").click(function(event){ //获取元素的值,val() 方法返回或设置被选元素的值。 var username = $("#username").val(); //判断值是否为空 if(username==""){ //提示信息 //alert("文本框的值不能为空"); $("#msg").html("<p>文本框的值不能为空.</p>"); //阻止默认行为 ( 表单提交 ) event.preventDefault(); } }); }); </script>
//Prevent default behavior (form submission) event.preventDefault();
Another way to prevent default behavior is to return false. The effect is the same.
The code is as follows:
<script type="text/javascript" src="js/jquery-1.8.3.js"></script> <script type="text/javascript"> $(function(){ $("#sub").click(function(event){ //获取元素的值,val() 方法返回或设置被选元素的值。 var username = $("#username").val(); //判断值是否为空 if(username==""){ //提示信息 //alert("文本框的值不能为空"); $("#msg").html("<p>文本框的值不能为空.</p>"); //阻止默认行为 ( 表单提交 ) //event.preventDefault(); return false; } }); }); </script>
Similarly, the above bubbling event can also be handled by return false.
<script type="text/javascript" src="js/jquery-1.8.3.js"></script> <script type="text/javascript"> $(function(){ // 为内层div绑定click事件 $("#msg").click(function(event){ alert("我是小div"); //event.stopPropagation(); // 阻止事件冒泡 return false; }); // 为外层div元素绑定click事件 $("#content").click(function(event){ alert("我是大div"); //event.stopPropagation(); // 阻止事件冒泡 return false; }); // 为body元素绑定click事件 $("body").click(function(event){ alert("我是body"); //event.stopPropagation(); // 阻止事件冒泡 return false; }); });
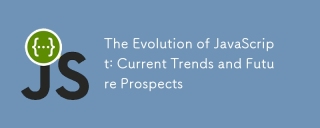
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
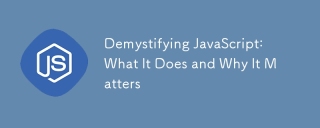
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
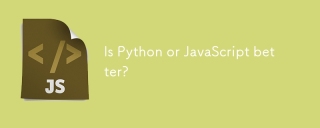
Python is more suitable for data science and machine learning, while JavaScript is more suitable for front-end and full-stack development. 1. Python is known for its concise syntax and rich library ecosystem, and is suitable for data analysis and web development. 2. JavaScript is the core of front-end development. Node.js supports server-side programming and is suitable for full-stack development.
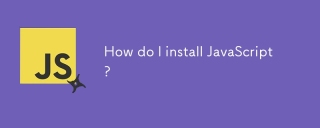
JavaScript does not require installation because it is already built into modern browsers. You just need a text editor and a browser to get started. 1) In the browser environment, run it by embedding the HTML file through tags. 2) In the Node.js environment, after downloading and installing Node.js, run the JavaScript file through the command line.
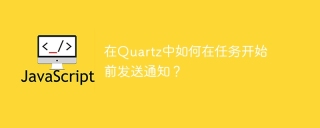
How to send task notifications in Quartz In advance When using the Quartz timer to schedule a task, the execution time of the task is set by the cron expression. Now...
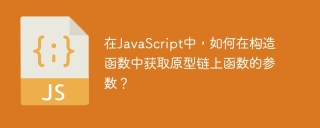
How to obtain the parameters of functions on prototype chains in JavaScript In JavaScript programming, understanding and manipulating function parameters on prototype chains is a common and important task...
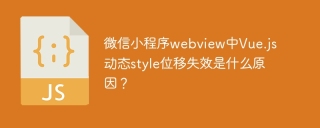
Analysis of the reason why the dynamic style displacement failure of using Vue.js in the WeChat applet web-view is using Vue.js...
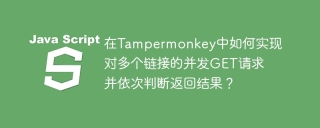
How to make concurrent GET requests for multiple links and judge in sequence to return results? In Tampermonkey scripts, we often need to use multiple chains...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.