This article mainly introduces the relevant information of Java Web simple paging display example code. This article achieves the paging effect by calculating the total number of pages and querying the specified page data. It is very good and has reference value. Friends in need You can refer to
This article uses two methods: (1) Calculate the total number of pages. (2) Query the specified page data to achieve a simple paging effect.
Idea: First of all, you must provide a paging query method in the DAO object. Call this method in the control layer to find the data of the specified page, and display the data of the page through EL expressions and JSTL in the presentation layer.
Let me show you the renderings first:
Digression: The paging display is implemented using the design idea of "presentation layer-control layer-DAO layer-database". Is there anything that needs to be improved? Let’s bring it up and learn and make progress together. Without further ado, let’s get into the topic. The detailed steps are as follows:
1. DAO layer - database
The JDBCUtils class is used to open and close the database. The core code is as follows:
import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; public class JDBCUtils { private Connection conn=null; private PreparedStatement pstmt=null; /** * connect 连接数据库 * @return */ public Connection connect(){ String user="root"; String password="1234"; String driverClass = "com.mysql.jdbc.Driver"; String jdbcUrl = "jdbc:mysql://localhost:3306/book"; try { Class.forName(driverClass); conn = DriverManager.getConnection(jdbcUrl, user, password); } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); } return conn; } /** * close 关闭数据库 * @param conn * @param pstmt * @param resu */ public void close(Connection conn,PreparedStatement pstmt,ResultSet result){ if(conn != null){ try { conn.close(); } catch (SQLException e) { // TODO Auto-generated catch block } } if(pstmt != null){ try { pstmt.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } if(result != null){ try { result.close(); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } }
The method getPage() and the method listUser() in the UserDao class are used to calculate the total number of pages and query the data of the specified page respectively. The core code is as follows:
import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.ArrayList; import java.util.List; import com.db.JDBCUtils; public class UserDao { /** * 计算总的页数 * @return */ public int getPage(){ int recordCount=0,t1=0,t2=0; PreparedStatement pstmt=null; ResultSet result=null; JDBCUtils jdbc=new JDBCUtils(); Connection conn=jdbc.connect(); String sql="select count(*) from books"; try { pstmt=conn.prepareStatement(sql); result=pstmt.executeQuery(); result.next(); recordCount=result.getInt(1); t1=recordCount%5; t2=recordCount/5; } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); }finally{ jdbc.close(conn, pstmt, result); } if(t1 != 0){ t2=t2+1; } return t2; } /** * 查询指定页的数据 * @param pageNo * @return */ public List<User> listUser(int pageNo){ PreparedStatement pstmt=null; ResultSet result=null; List<User> list=new ArrayList<User>(); int pageSize=5; int page=(pageNo-1)*5; JDBCUtils jdbc=new JDBCUtils(); Connection conn=jdbc.connect(); String sql="select * from books order by id limit ?,?"; try { pstmt=conn.prepareStatement(sql); pstmt.setInt(1, page); pstmt.setInt(2, pageSize); result=pstmt.executeQuery(); while(result.next()){ User user=new User(); user.setId(result.getInt(1)); user.setName(result.getString(2)); user.setNumber(result.getString(3)); list.add(user); } } catch (Exception e) { // TODO Auto-generated catch block e.printStackTrace(); }finally{ jdbc.close(conn, pstmt, result); } return list; } }
The User class is used to store the queried data. The core The code is as follows:
public class User { private int id; private String name; private String number; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getNumber() { return number; } public void setNumber(String number) { this.number = number; } }
2. Control layer
ListUser class internally calls the UserDao object to query data and assign the page to display the data. The core code is as follows:
import java.io.IOException; import java.io.PrintWriter; import java.util.ArrayList; import java.util.List; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.dao.User; import com.dao.UserDao; public class ListUser extends HttpServlet { public ListUser() { super(); } public void destroy() { super.destroy(); // Just puts "destroy" string in log // Put your code here } public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setCharacterEncoding("utf-8"); int pageNo = 1; UserDao userdao=new UserDao(); List<User> lists=new ArrayList<User>(); String pageno=request.getParameter("pageNos"); if(pageno != null){ pageNo=Integer.parseInt(pageno); } lists=userdao.listUser(pageNo); int recordCount=userdao.getPage(); request.setAttribute("recordCount", userdao.getPage()); request.setAttribute("listss", lists); request.setAttribute("pageNos", pageNo); request.getRequestDispatcher("userlist.jsp").forward(request, response); } public void init() throws ServletException { // Put your code here } }
3. The presentation layer
outputs the page userlist.jsp, using EL and JSTL to output query results. The core code is as follows:
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %> <%@ taglib uri="http://java.sun.com/jsp/jstl/fmt" prefix="fmt"%> <%@ taglib uri="http://java.sun.com/jsp/jstl/functions" prefix="fn"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>My JSP 'userlist.jsp' starting page</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> <style type="text/css"> th,td{width: 150px;border: 2px solid gray;text-align: center;} body{text-align: center;} a{text-decoration: none;} table {border-collapse: collapse;} </style> </head> <body> <h2 id="图书信息">图书信息</h2> <table align="center"> <tr><td>书号</td><td>书名</td><td>库存量</td></tr> </table> <table align="center"> <c:forEach items="${listss}" var="person"> <tr> <td class="hidden-480">${person.id}</td> <td class="hidden-480">${person.name }</td> <td class="hidden-480">${person.number }</td> </tr> </c:forEach> </table> <br> <c:if test="${pageNos>1 }"> <a href="ListUser?pageNos=1" >首页</a> <a href="ListUser?pageNos=${pageNos-1 }">上一页</a> </c:if> <c:if test="${pageNos <recordCount }"> <a href="ListUser?pageNos=${pageNos+1 }">下一页</a> <a href="ListUser?pageNos=${recordCount }">末页</a> </c:if> <form action="ListUser"> <h4 align="center">共${recordCount}页   <input type="text" value="${pageNos}" name="pageNos" size="1">页 <input type="submit" value="到达"> </h4> </form> </body> </html>
The above is the simple paging display example code of Java Web introduced by the editor. , I hope it will be helpful to you. If you have any questions, please leave me a message and the editor will reply to you in time. I would also like to thank you all for your support of the PHP Chinese website!
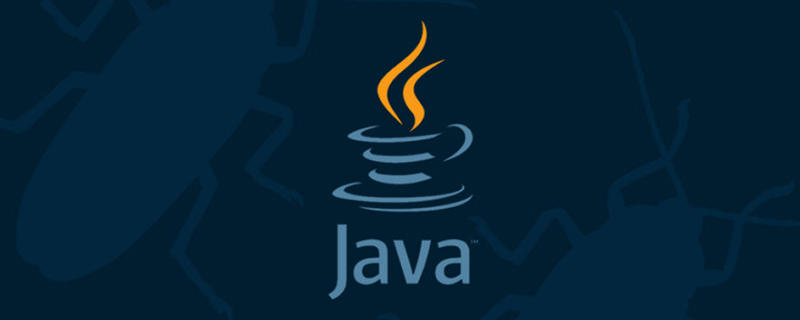
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
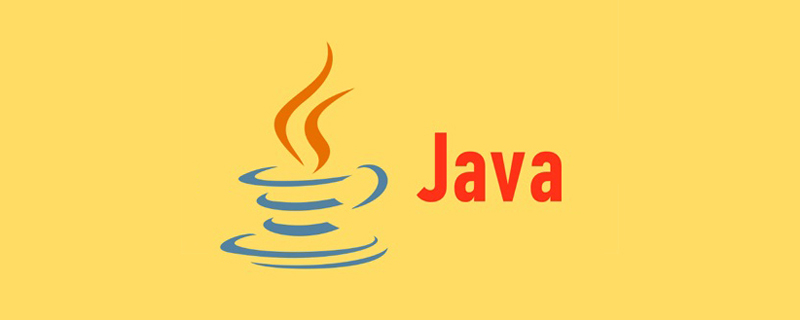
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
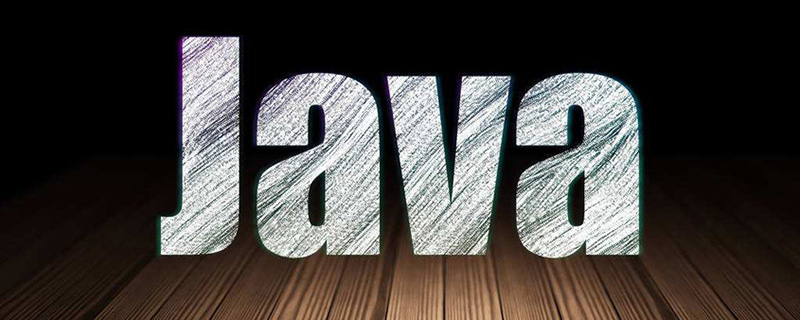
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
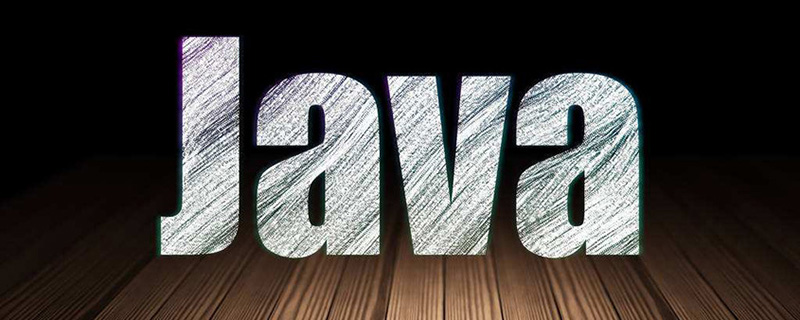
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
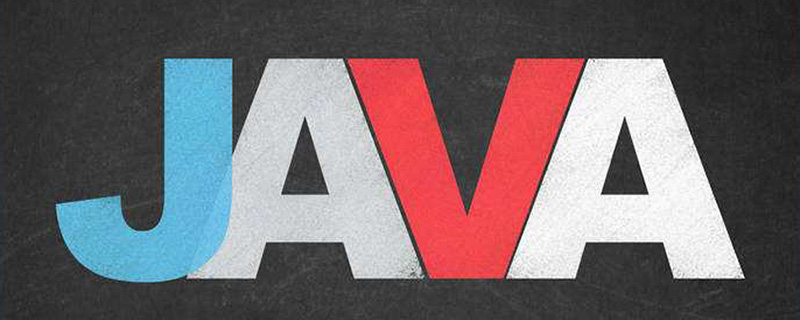
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
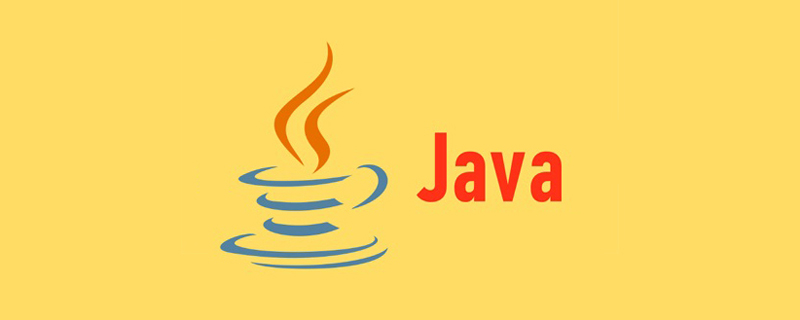
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
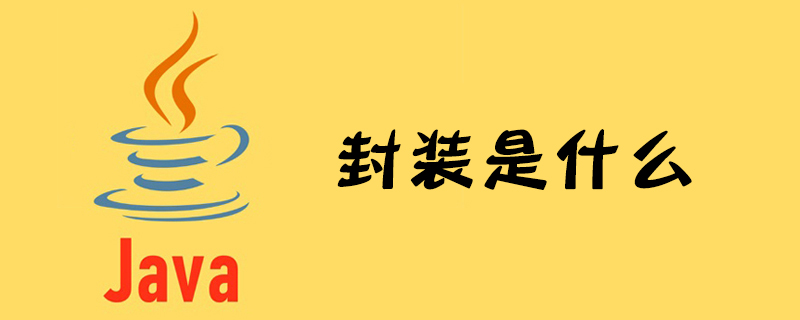
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
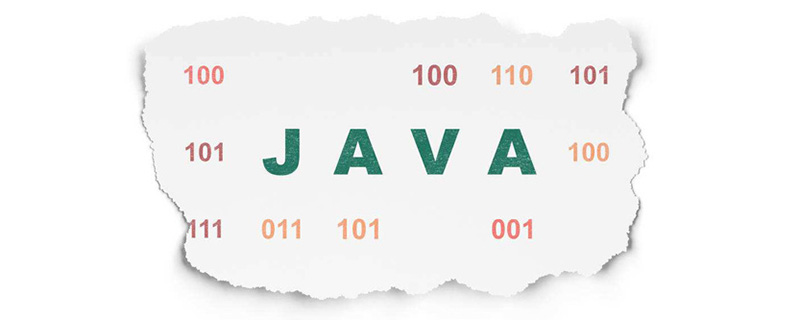
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
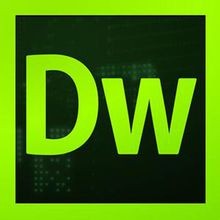
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
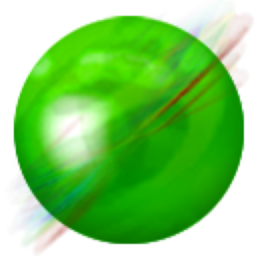
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
