Python’s namespace is something that Python programmers must understand. Learning about Python’s namespace will enable us to essentially master some trivial rules in Python.
Next, I will reveal the essence of Python namespaces in four parts: 1. The definition of namespaces; 2. The search order of namespaces; 3. The life cycle of namespaces; 4. BIF through locals() and globals() Accessing namespaces
The focus is on the fourth part, where we will observe the contents of namespaces.
1. Namespace
Python uses something called a namespace to record the trajectory of variables. A namespace is a dictionary whose keys are variable names and whose values are the values of those variables.
A namespace is a mapping from names to objects. Most namespaces are currently implemented as Python dictionaries.
Anywhere in a Python program, there are several available namespaces.
1. Each function has its own namespace, called the local namespace, which records the variables of the function, including function parameters and locally defined variables.
2. Each module has its own namespace, called the global namespace, which records the variables of the module, including functions, classes, other imported modules, module-level variables and constants.
3. There is also a built-in namespace, which can be accessed by any module and stores built-in functions and exceptions.
2. Namespace search order
When a line of code wants to use the value of variable x, Python will search for the variable in all available namespaces, in the following order:
1. Local namespace: specifically The current function or class method. If the function defines a local variable x, or a parameter x, Python will use it and then stop searching.
2. Global namespace: specifically refers to the current module. If the module defines a variable, function, or class named x, Python will use it and then stop searching.
3. Built-in namespace: global for each module. As a last resort, Python will assume that x is a built-in function or variable.
4. If Python cannot find x in these namespaces, it will give up the search and raise a NameError exception, such as NameError: name 'aa' is not defined.
The situation of nested functions:
1. First search
in the namespace of the current (nested or lambda) function 2. Then search in the namespace of the parent function
3. Then Search in the module namespace
4. Finally, search in the built-in namespace
Example:
info = "Adress : " def func_father(country): def func_son(area): city= "Shanghai " #此处的city变量,覆盖了父函数的city变量 print(info + country + city + area) city = " Beijing " #调用内部函数 func_son("ChaoYang "); func_father("China ")
Output: Adress: China Shanghai ChaoYang
In the above example, info is in the global In the namespace, country is in the namespace of the parent function, and city and area are in the namespace of its own function. There are different lifetimes.
1. The built-in namespace is created when the Python interpreter starts, and will always be retained and will not be deleted.
3. A local namespace is created when the function is called, and is deleted when the function returns a result or throws an exception. Each recursively called function has its own namespace.
i=i+1
y=123
del y
print(y)
func3()
#Error: UnboundLocalError: local variable 'y' referenced before assignment
#Remove "del y" After the statement, it runs normally
4. Namespace access 1. The local namespace can be accessed using locals() BIF. locals returns a dictionary of name/value pairs. The keys of this dictionary are variable names in the form of strings, and the values of the dictionary are the actual values of the variables. Example:
def func1(i, str):
x = 12345
print(locals())
func1(1, "first")输出:{'str': 'first', 'x': 12345, 'i': 1}
2、全局 (模块级别)命名空间可以通过 globals() BIF来访问。
示例:
'''Created on 2013-5-26''' import copy from copy import deepcopy gstr = "global string" def func1(i, info): x = 12345 print(locals()) func1(1 , "first") if __name__ == "__main__": print("the current scope's global variables:") dictionary=globals() print(dictionary)
输出:(我自己给人为的换行、更换了顺序,加颜色的语句下面重点说明)
{
'__name__': '__main__',
'__doc__': 'Created on 2013-5-26',
'__package__': None,
'__cached__': None,
'__file__': 'E:\\WorkspaceP\\Test1\\src\\base\\test1.py',
'__loader__': <_frozen_importlib.sourcefileloader object at>,
'copy':
'__builtins__':
'gstr': 'global string',
'dictionary': {...},
'func1':
'deepcopy':
}
总结
1、模块的名字空间不仅仅包含模块级的变量和常量,还包括所有在模块中定义的函数和类。除此以外,它还包括了任何被导入到模块中的东西。
2、我们看到,内置命名也同样被包含在一个模块中,它被称作 __builtin__。
3、回想一下 from module import 和 import module 之间的不同。
使用 import module,模块自身被导入,但是它保持着自已的名字空间,这就是为什么您需要使用模块名来访问它的函数或属性:module.function 的原因。
但是使用 from module import function,实际上是从另一个模块中将指定的函数和属性导入到您自己的名字空间,这就是为什么您可以直接访问它们却不需要引用它们所来源的模块。使用 globals 函数,您会真切地看到这一切的发生,见上面的红色输出语句。
3、 locals 与 globals 之间的一个重要的区别
locals 是只读的,globals 不是
示例:
def func1(i, info): x = 12345 print(locals()) locals()["x"]= 6789 print("x=",x) y=54321 func1(1 , "first") globals()["y"]= 9876 print( "y=",y)
输出:
{'i': 1, 'x': 12345, 'info': 'first'}
x= 12345
y= 9876
解释:
locals 实际上没有返回局部名字空间,它返回的是一个拷贝。所以对它进行改变对局部名字空间中的变量值并无影响。
globals 返回实际的全局名字空间,而不是一个拷贝。所以对 globals 所返回的 dictionary 的任何的改动都会直接影响到全局变量。
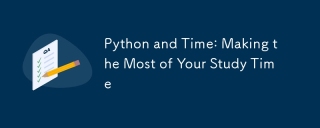
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
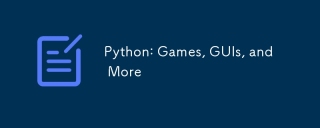
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
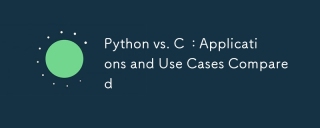
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
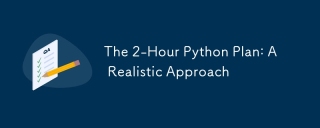
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
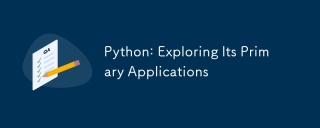
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
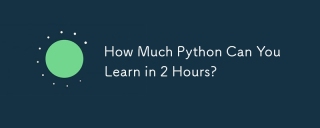
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
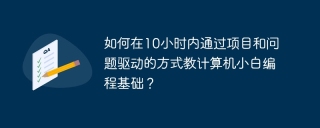
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
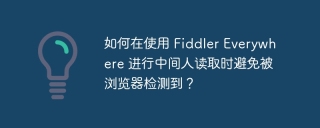
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
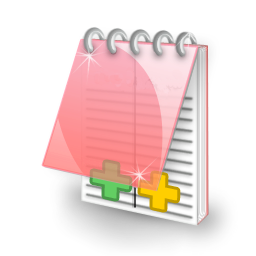
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
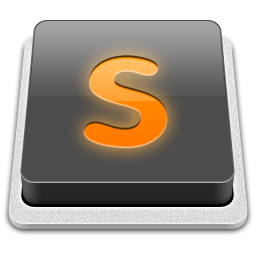
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Notepad++7.3.1
Easy-to-use and free code editor