Usage of String type
Review the well-known string usage, for example
let str = 'orange'; str.indexOf('o'); //0 str.indexOf('n'); //3 str.indexOf('c'); //-1
Here 0 and 3 are the positions where o and n appear in the string respectively. The starting index is 0. And -1 means no match.
Someone once asked me why -1 is not null or undefined. Ask the person who made the rules! He looked helpless.
Everyone sees nothing interesting here, don’t worry, here is another example
let numStr = '2016'; numStr.indexOf('2'); //0 numStr.indexOf(2); //0
There is a small point here that indexOf will do a simple type conversion, convert the number into the string '2' and then execute it.
Use of Number type
You may wonder whether the number type has an indexOf method because it will do implicit conversion! Let me tell you clearly that there is no, the above example
let num = 2016; num.indexOf(2); //Uncaught TypeError: num.indexOf is not a function
Do you have to use the indexOf method for the number type? Then convert it to String, follow the above example to write
//二逼青年的写法 num = '2016'; num.indexOf(2); //0 //普通青年的写法 num.toString().indexOf(2); //0 //文艺青年的写法 ('' + num).indexOf(2); //0
The first way of writing is simple and direct, and it is not impossible for known shorter numbers. But what should we do when the num variable changes for different data?
The second way of writing is the most commonly used, but it is a bit longer than the third way of writing. Haha, in fact, both are fine. People who are obsessed with code prefer the use of the third
Array type
Everyone, cheer up, the big boss is coming.
Everyone is very familiar with array methods, but they ignore that arrays have the indexOf method (my personal feeling).
It’s just talk without practice. What problems did you encounter and what should you pay attention to?
let arr = ['orange', '2016', '2016']; arr.indexOf('orange'); //0 arr.indexOf('o'); //-1 arr.indexOf('2016'); //1 arr.indexOf(2016); //-1
The examples are not broken down in detail here. Four use cases are enough to illustrate the problem.
arr.indexOf('orange') outputs 0 because 'orange' is the 0th element of the array, and the index is matched and returned.
arr.indexOf('o') outputs -1 because this method does not perform the indexOf match again on a per-element basis.
arr.indexOf('2016') outputs 1 because this method returns the following list of the first array element from the beginning of the match until the match is reached, rather than returning the index of all matches.
arr.indexOf(2016) output -1 Note: No implicit type conversion will be done here.
Now that the trap has been discovered, we might as well get to the bottom of it. Go to the MDN official website to find out. Friends who are interested in this topic can jump directly to Array.prototype.indexOf()
For those who just want to know more, here is the official Description.
indexOf() compares searchElement to elements of the Array using strict equality (the same method used by the === or triple-equals operator).
It is clear at a glance that strict equality (===) is used here. Please pay more attention when making similar judgments. Don't make the mistake of thinking that numbers will be converted to strings, and strings will not be converted to numbers.
Summary
The accumulation of small knowledge points is not a topic for in-depth discussion, so the second parameter of indexOf() is not explained here. I believe everyone knows the role of the second parameter. If you don’t know, you can see here String.prototype .indexOf(), and then take a look at the second parameter combined with the link to the array above.
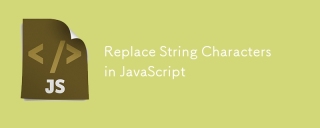
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
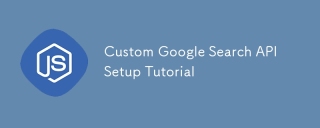
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
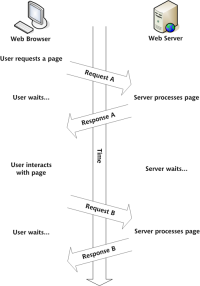
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
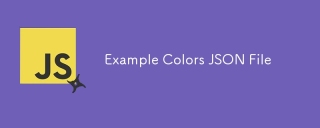
This article series was rewritten in mid 2017 with up-to-date information and fresh examples. In this JSON example, we will look at how we can store simple values in a file using JSON format. Using the key-value pair notation, we can store any kind
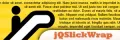
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
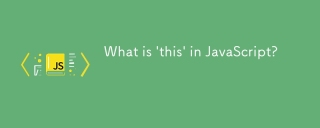
Core points This in JavaScript usually refers to an object that "owns" the method, but it depends on how the function is called. When there is no current object, this refers to the global object. In a web browser, it is represented by window. When calling a function, this maintains the global object; but when calling an object constructor or any of its methods, this refers to an instance of the object. You can change the context of this using methods such as call(), apply(), and bind(). These methods call the function using the given this value and parameters. JavaScript is an excellent programming language. A few years ago, this sentence was

jQuery is a great JavaScript framework. However, as with any library, sometimes it’s necessary to get under the hood to discover what’s going on. Perhaps it’s because you’re tracing a bug or are just curious about how jQuery achieves a particular UI
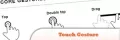
This post compiles helpful cheat sheets, reference guides, quick recipes, and code snippets for Android, Blackberry, and iPhone app development. No developer should be without them! Touch Gesture Reference Guide (PDF) A valuable resource for desig


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
