xpath is a language for finding information in xml documents. xpath is used to navigate through elements and attributes in XML documents. Its return value may be nodes, node collections, text, and a mixture of nodes and text, etc.
Before studying this document, you should have a certain understanding of XML nodes, elements, attributes, text, processing instructions, comments, root nodes, namespaces and node relationships, as well as xpath.
XML learning address: http://www.runoob.com/xml/xml-tutorial.html
xpath basic syntax learning address: http://www.runoob.com/xpath/xpath-tutorial.html
xpath official documentation : https://yunpan.cn/cvc4tEIGy5EvS Access password 9d16
This article mainly introduces: using xpath operation to operate xml in Java.
1) First, how to use xpath technology in dom4j
Import the jar package supported by xPath. jaxen-1.1-beta-6.jar (first import the dom4j package, dom4j download address: http://www.dom4j.org/dom4j-1.6.1/).
As shown in the figure after importing the package:
If you don’t know how to import the package, please refer to my previous blog: Summary of Java Obtaining XML Nodes and Reading XML Documents Node
2) There are two main points for using the xpath method in Java:
List
Node selectSingleNode("xpath expression"); Query a node object
Below Just use examples to illustrate how to use it.
1. How to use selectNodes:
package com.vastsum.demo; import java.io.File; import java.io.FileOutputStream; import java.util.List; import org.dom4j.Document; import org.dom4j.Element; import org.dom4j.Node; import org.dom4j.io.OutputFormat; import org.dom4j.io.SAXReader; import org.dom4j.io.XMLWriter; /** * * @author shutu008 *selectNode的使用方法 */ public class xpathDemo { public static void main(String[] args) throws Exception { Document doc = new SAXReader().read(new File("./src/contact.xml")); /** * @param xpath 表示xpath语法变量 */ String xpath=""; /** * 1. / 绝对路径 表示从xml的根位置开始或子元素(一个层次结构) */ xpath = "/contactList"; xpath = "/contactList/contact"; /** * 2. // 相对路径 表示不分任何层次结构的选择元素。 */ xpath = "//contact/name"; xpath = "//name"; /** * 3. * 通配符 表示匹配所有元素 */ xpath = "/contactList/*"; //根标签contactList下的所有子标签 xpath = "/contactList//*";//根标签contactList下的所有标签(不分层次结构) /** * 4. [] 条件 表示选择什么条件下的元素 */ //带有id属性的contact标签 xpath = "//contact[@id]"; //第二个的contact标签 xpath = "//contact[2]"; //选择最后一个contact标签 xpath = "//contact[last()]"; /** * 5. @ 属性 表示选择属性节点 */ xpath = "//@id"; //选择id属性节点对象,返回的是Attribute对象 xpath = "//contact[not(@id)]";//选择不包含id属性的contact标签节点 xpath = "//contact[@id='002']";//选择id属性值为002的contact标签 xpath = "//contact[@id='001' and @name='eric']";//选择id属性值为001,且name属性为eric的contact标签 /** *6. text() 表示选择文本内容 */ //选择name标签下的文本内容,返回Text对象 xpath = "//name/text()"; xpath = "//contact/name[text()='张三']";//选择姓名为张三的name标签 List<Node> list = doc.selectNodes(xpath); for (Node node : list) { System.out.println(node); } //写出xml文件 //输出位置 FileOutputStream out = new FileOutputStream("d:/contact.xml"); //指定格式 OutputFormat format = OutputFormat.createPrettyPrint(); format.setEncoding("utf-8"); XMLWriter writer = new XMLWriter(out,format); //写出内容 writer.write(doc); //关闭资源 writer.close(); } }
2. How to use selectSingleNode
package com.vastsum.demo; import java.io.File; import java.util.Iterator; import org.dom4j.Attribute; import org.dom4j.Document; import org.dom4j.Element; import org.dom4j.io.SAXReader; /** * * @author shutu008 *selectSingleNode的使用 */ public class xpathDemo1{ public static void main(String[] args) throws Exception{ //读取XML文件,获得document对象 SAXReader saxReader = new SAXReader(); Document doc = saxReader.read(new File("./src/contact.xml")); //使用xpath获取某个节点 String xpath = ""; //对contact元素 id="001"的节点,操作 xpath = "//contact[@id = '001']"; Element contactElem = (Element)doc.selectSingleNode(xpath); //设置这个节点的属性值 contactElem.addAttribute("name", "001"); //输出这个节点的所有属性值 for(Iterator it = contactElem.attributeIterator();it.hasNext();){ Attribute conAttr = (Attribute)it.next(); String conTxt = conAttr.getValue(); String conAttrName = conAttr.getName(); System.out.println(conAttrName+" = "+conTxt); } } }

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
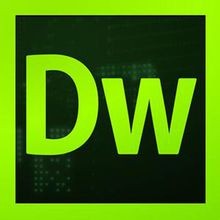
Dreamweaver CS6
Visual web development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
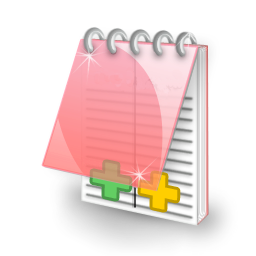
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.