Traits
Many of my PHP developer friends don’t know much about traits, which is a new concept introduced in PHP 5.4.0. Traits look like interfaces but work like classes, so what exactly are they? It's neither.
A trait has partial implementation (such as constants, properties and methods) that can be embedded into one or more actual PHP classes. Traits have two responsibilities: indicating what a class can do (similar to an interface); and providing a modular implementation (similar to a class).
You may already have some understanding of traits in other languages. For example, the functions of Ruby's modules and mixins are very similar to PHP's traits.
Why should we use traits
PHP language uses the classic inheritance model. This means that you start with a common root class that provides the basic implementation. From the root class, more specific classes are created that directly inherit the various implementations of the parent class. This is called an inheritance hierarchy, and many programming languages use this common pattern.
To make it easier to understand, imagine that you travel back in time to high school to study biology. Do you still remember the phylum, order, family, genus and species of the organisms you studied? There are six major realms in total. The realm is derived from the door, the phylum is derived from the class, the class is derived from the order, the order is derived from the family, the family is derived from the genus, and the genus is followed by the species. Each descent down the species hierarchy represents a specific characteristic.
The classic inheritance model works well in most cases. But what if there are two unrelated classes that need to implement similar behavior? For example, one PHP class is called RetailStore, and another PHP class is called Car. They can be said to be two completely unrelated classes, and they cannot share a common parent class in terms of inheritance relationship. However, both classes require the longitude and latitude from the geographic location to display map coordinates.
We created traits to solve this problem. They can inject partial implementation into unrelated classes. Traits also facilitate code reuse.
When I encounter this problem, my first solution (and the worst) is to create a public parent class Geocodable for the RetailStore and Car classes to inherit. This solution is really bad, because forcing two unrelated classes to share a common ancestor looks very awkward in their respective inheritance hierarchies.
My second solution (slightly better) is to create a Geocodable interface that defines what methods are needed to implement geolocation. Both RetialStore and Car classes can implement this Geocodable interface. It is indeed a good solution to allow each class to retain its natural inheritance relationship. But we still need to repeat the definition in the interface in each class, which is not a DRY solution.
DRY is the abbreviation of Do not repeat yourself. As a good programming practice, we should never repeat the same code in multiple places. There cannot be a situation where you have to passively modify the same code in other places because you have changed one piece of code.
My third solution (the best solution) is to construct a Geocodable trait and define and implement related methods in it. I can add Geocodable traits to the RetailStore class and Car class without disrupting the class inheritance hierarchy.
How to construct a trait
The following shows how to define a PHP trait:
<?php trait MyTrait { // 此处是trait的具体实现 }
As a good habit, we should do one trait per file, just like the definition of classes and interfaces .
Let’s go back to our Geocodable example to better demonstrate the use of traits. We all know that the RetailStore class and the Car class need to support the geographical positioning function, and we can all agree that inheritance and interfaces are not the best solution. Instead, we construct a Geocodable trait that returns a longitude and latitude coordinate that can be marked on the map. Example 2-12 shows our complete Geocodable trait.
Example 2-12 Definition of Geocodable trait
<span style="font-size:14px;"><?php trait Geocodable { /** @var string */ protected $address; /** @var \Geocoder\Geocoder */ protected $geocoder; /** @var \Geocoder\Result\Geocoded */ protected $geocoderResult; public function setGeocoder(\Geocoder\GeocoderInterface $geocoder) { $this->geocoder = $geocoder; } public function setAddress($address) { $this->address = $address; } public function getLatitude() { if (isset($this->geocoderResult) === false) { $this->geocodeAddress(); } return $this->geocoderResult->getLatitude(); } public function getLongitude() { if (isset($this->geocoderResult) === false) { $this->geocodeAddress(); } return $this->geocoderResult->getLongitude(); } protected function geocodeAddress() { $this->geocoderResult = $this->geocoder->geocode($this->address); return true; } }</span>
Geocodable trait only defines the properties and methods required to implement the geographical location function without any additional functions.
Our Geocodable trait defines properties of three classes:
To be continued...
The above introduces [Modern PHP] Chapter 2 New Features Three Traits, including aspects of the content. I hope it will be helpful to friends who are interested in PHP tutorials.
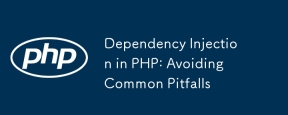
DependencyInjection(DI)inPHPenhancescodeflexibilityandtestabilitybydecouplingdependencycreationfromusage.ToimplementDIeffectively:1)UseDIcontainersjudiciouslytoavoidover-engineering.2)Avoidconstructoroverloadbylimitingdependenciestothreeorfour.3)Adhe
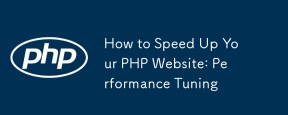
ToimproveyourPHPwebsite'sperformance,usethesestrategies:1)ImplementopcodecachingwithOPcachetospeedupscriptinterpretation.2)Optimizedatabasequeriesbyselectingonlynecessaryfields.3)UsecachingsystemslikeRedisorMemcachedtoreducedatabaseload.4)Applyasynch
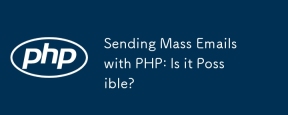
Yes,itispossibletosendmassemailswithPHP.1)UselibrarieslikePHPMailerorSwiftMailerforefficientemailsending.2)Implementdelaysbetweenemailstoavoidspamflags.3)Personalizeemailsusingdynamiccontenttoimproveengagement.4)UsequeuesystemslikeRabbitMQorRedisforb
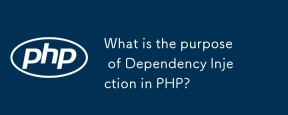
DependencyInjection(DI)inPHPisadesignpatternthatachievesInversionofControl(IoC)byallowingdependenciestobeinjectedintoclasses,enhancingmodularity,testability,andflexibility.DIdecouplesclassesfromspecificimplementations,makingcodemoremanageableandadapt
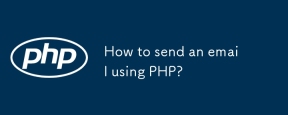
The best ways to send emails using PHP include: 1. Use PHP's mail() function to basic sending; 2. Use PHPMailer library to send more complex HTML mail; 3. Use transactional mail services such as SendGrid to improve reliability and analysis capabilities. With these methods, you can ensure that emails not only reach the inbox, but also attract recipients.
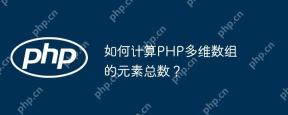
Calculating the total number of elements in a PHP multidimensional array can be done using recursive or iterative methods. 1. The recursive method counts by traversing the array and recursively processing nested arrays. 2. The iterative method uses the stack to simulate recursion to avoid depth problems. 3. The array_walk_recursive function can also be implemented, but it requires manual counting.
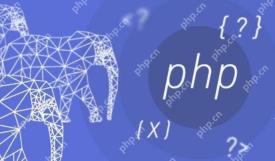
In PHP, the characteristic of a do-while loop is to ensure that the loop body is executed at least once, and then decide whether to continue the loop based on the conditions. 1) It executes the loop body before conditional checking, suitable for scenarios where operations need to be performed at least once, such as user input verification and menu systems. 2) However, the syntax of the do-while loop can cause confusion among newbies and may add unnecessary performance overhead.
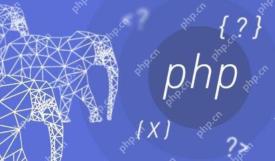
Efficient hashing strings in PHP can use the following methods: 1. Use the md5 function for fast hashing, but is not suitable for password storage. 2. Use the sha256 function to improve security. 3. Use the password_hash function to process passwords to provide the highest security and convenience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
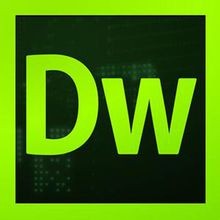
Dreamweaver CS6
Visual web development tools
