


The definition of a binary tree is as follows: a non-empty binary tree consists of three basic parts: the root node and the left and right subtrees. There are three traversal methods depending on the access position of the node:
① NLR: Preorder Traversal (also known as Preorder Traversal)
——The operation of accessing a node occurs before traversing its left and right subtrees.
② LNR: Inorder Traversal (InorderTraversal)
——The operation of accessing a node occurs by traversing its left and right subtrees (between).
③ LRN: Postorder Traversal
——The operation of accessing a node occurs after traversing its left and right subtrees.
As shown below:
Binary trees were previously written in C, and recursive traversal can also be simply simulated with PHP. The following example is considered the simplest kind of traversal (refer to the Internet).
<code><span>/** * 二叉树遍历 * @blog<http:> */</http:></span> class Node { <span>public</span><span>$value</span>; <span>public</span><span>$left</span>; <span>public</span><span>$right</span>; } <span>//前序遍历,访问根节点->遍历子左树->遍历右左树</span> function preorder(<span>$root</span>){ <span>$stack</span><span>=</span><span>array</span>(); array_push(<span>$stack</span>, <span>$root</span>); <span>while</span>(<span>!</span>empty(<span>$stack</span>)){ <span>$center_node</span><span>=</span> array_pop(<span>$stack</span>); echo <span>$center_node</span><span>-></span>value<span>.</span><span>' '</span>; <span>if</span>(<span>$center_node</span><span>-></span>right <span>!=</span><span>null</span>) array_push(<span>$stack</span>, <span>$center_node</span><span>-></span>right); <span>if</span>(<span>$center_node</span><span>-></span>left <span>!=</span><span>null</span>) array_push(<span>$stack</span>, <span>$center_node</span><span>-></span>left); } } <span>//中序遍历,遍历子左树->访问根节点->遍历右右树</span> function inorder(<span>$root</span>){ <span>$stack</span><span>=</span><span>array</span>(); <span>$center_node</span><span>=</span><span>$root</span>; <span>while</span> (<span>!</span>empty(<span>$stack</span>) <span>||</span><span>$center_node</span><span>!=</span><span>null</span>) { <span>while</span> (<span>$center_node</span><span>!=</span><span>null</span>) { array_push(<span>$stack</span>, <span>$center_node</span>); <span>$center_node</span><span>=</span><span>$center_node</span><span>-></span>left; } <span>$center_node</span><span>=</span> array_pop(<span>$stack</span>); echo <span>$center_node</span><span>-></span>value <span>.</span><span>" "</span>; <span>$center_node</span><span>=</span><span>$center_node</span><span>-></span>right; } } <span>//后序遍历,遍历子左树->访问子右树->遍历根节点</span> function postorder(<span>$root</span>){ <span>$pushstack</span><span>=</span><span>array</span>(); <span>$visitstack</span><span>=</span><span>array</span>(); array_push(<span>$pushstack</span>, <span>$root</span>); <span>while</span> (<span>!</span>empty(<span>$pushstack</span>)) { <span>$center_node</span><span>=</span> array_pop(<span>$pushstack</span>); array_push(<span>$visitstack</span>, <span>$center_node</span>); <span>if</span> (<span>$center_node</span><span>-></span>left <span>!=</span><span>null</span>) array_push(<span>$pushstack</span>, <span>$center_node</span><span>-></span>left); <span>if</span> (<span>$center_node</span><span>-></span>right <span>!=</span><span>null</span>) array_push(<span>$pushstack</span>, <span>$center_node</span><span>-></span>right); } <span>while</span> (<span>!</span>empty(<span>$visitstack</span>)) { <span>$center_node</span><span>=</span> array_pop(<span>$visitstack</span>); echo <span>$center_node</span><span>-></span>value<span>.</span><span>" "</span>; } } <span>//创建如上图所示的二叉树</span><span>$a</span><span>=</span><span>new</span> Node(); <span>$b</span><span>=</span><span>new</span> Node(); <span>$c</span><span>=</span><span>new</span> Node(); <span>$d</span><span>=</span><span>new</span> Node(); <span>$e</span><span>=</span><span>new</span> Node(); <span>$f</span><span>=</span><span>new</span> Node(); <span>$a</span><span>-></span>value <span>=</span><span>'A'</span>; <span>$b</span><span>-></span>value <span>=</span><span>'B'</span>; <span>$c</span><span>-></span>value <span>=</span><span>'C'</span>; <span>$d</span><span>-></span>value <span>=</span><span>'D'</span>; <span>$e</span><span>-></span>value <span>=</span><span>'E'</span>; <span>$f</span><span>-></span>value <span>=</span><span>'F'</span>; <span>$a</span><span>-></span>left <span>=</span><span>$b</span>; <span>$a</span><span>-></span>right <span>=</span><span>$c</span>; <span>$b</span><span>-></span>left <span>=</span><span>$d</span>; <span>$c</span><span>-></span>left <span>=</span><span>$e</span>; <span>$c</span><span>-></span>right <span>=</span><span>$f</span>; <span>//前序遍历</span> preorder(<span>$a</span>); <span>//结果是:A B D C E F</span> inorder(<span>$a</span>); <span>//结果是: D B A E C F</span> postorder(<span>$a</span>); <span>//结果是: D B E F C A</span></code>
').addClass('pre-numbering').hide(); $(this).addClass('has-numbering').parent().append($numbering); for (i = 1; i ').text(i)); }; $numbering.fadeIn(1700); }); });http://www.phpddt.com/php/binary-tree-traverse.html
The above introduces the non-recursive method of binary tree traversal and stack simulation implementation in PHP, including aspects of it. I hope it will be helpful to friends who are interested in PHP tutorials.
![宏碁关怀中心服务仍在初始化[修复]](https://img.php.cn/upload/article/000/465/014/171055772117927.jpg)
本文将指导您解决在WindowsPC上出现的宏碁护理中心服务初始化错误消息的问题。当AcerCareCenter应用程序无法正常启动时,通常是由于应用程序损坏、过时或与其他软件发生冲突。修复宏碁关怀中心服务仍在初始化错误如果您在Windows11/10PC上看到AcerCareCenterService仍在初始化错误消息,请使用以下建议来解决此问题:重新启动ACCStd.exe进程以管理员身份运行AcerCareCenter暂时禁用您的防病毒软件检查干净启动状态重新安装宏碁关怀中心联系支持部门开
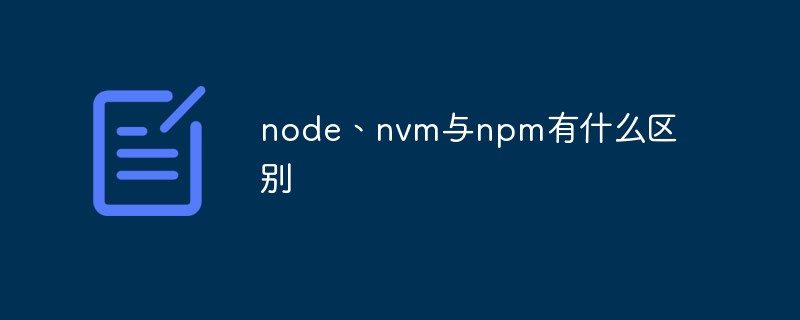
node、nvm与npm的区别:1、nodejs是项目开发时所需要的代码库,nvm是nodejs版本管理工具,npm是nodejs包管理工具;2、nodejs能够使得javascript能够脱离浏览器运行,nvm能够管理nodejs和npm的版本,npm能够管理nodejs的第三方插件。
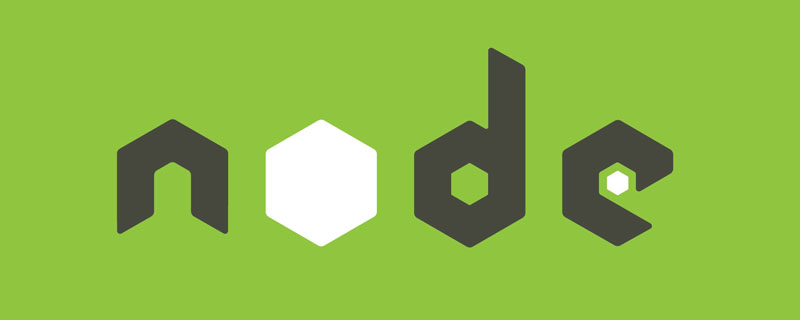
Vercel是什么?本篇文章带大家了解一下Vercel,并介绍一下在Vercel中部署 Node 服务的方法,希望对大家有所帮助!
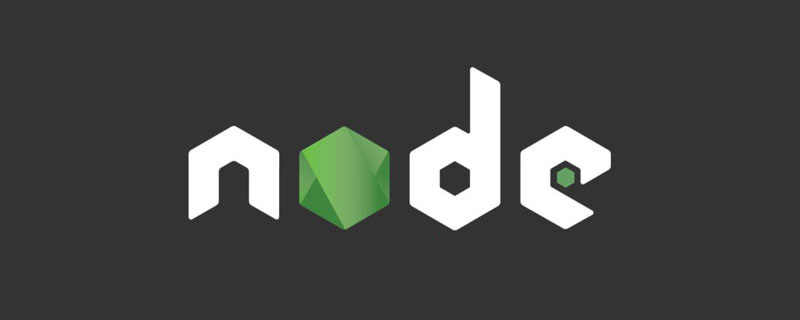
node怎么爬取数据?下面本篇文章给大家分享一个node爬虫实例,聊聊利用node抓取小说章节的方法,希望对大家有所帮助!
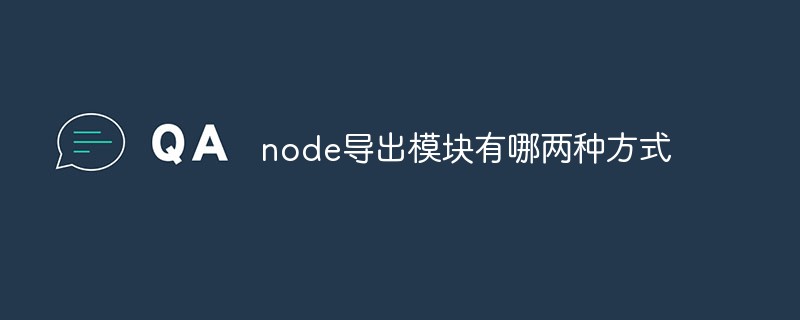
node导出模块的两种方式:1、利用exports,该方法可以通过添加属性的方式导出,并且可以导出多个成员;2、利用“module.exports”,该方法可以直接通过为“module.exports”赋值的方式导出模块,只能导出单个成员。
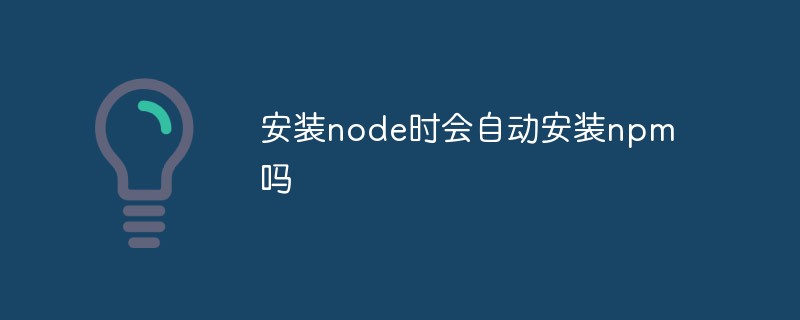
安装node时会自动安装npm;npm是nodejs平台默认的包管理工具,新版本的nodejs已经集成了npm,所以npm会随同nodejs一起安装,安装完成后可以利用“npm -v”命令查看是否安装成功。
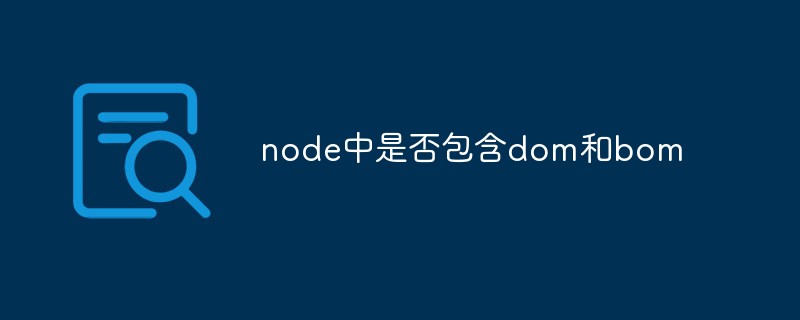
node中没有包含dom和bom;bom是指浏览器对象模型,bom是指文档对象模型,而node中采用ecmascript进行编码,并且没有浏览器也没有文档,是JavaScript运行在后端的环境平台,因此node中没有包含dom和bom。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
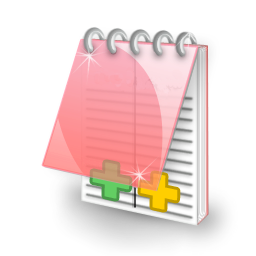
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
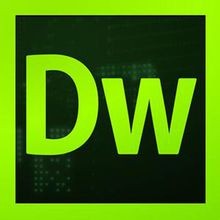
Dreamweaver CS6
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
