First understand the concept of the Observer pattern: an object makes itself observable by adding a method that allows another object, an observer, to register itself. When an observable object changes, it sends messages to registered observers. These observers use this information to perform operations independent of the observable object. The result is that objects can talk to each other without having to understand why. The observer pattern is an event system, which means that this pattern allows a class to observe the state of another class. When the state of the observed class changes, the observing class can receive notifications and take corresponding actions; observation The operator pattern provides you with the ability to avoid tight coupling between components.
UML structure diagram:
Problems solved by the observer pattern
In our development process, we should have more or less encountered the problem that changing part of the code will cause a series of other changes. Obviously we want to It is unlikely to completely avoid this situation, but we should also try to reduce dependencies on other components as much as possible, and the observer pattern is designed to solve this problem.
For example, we have a post object with the following code:
class Post { protected $_userid = null; protected $_ip = null; protected $_content = null; function __construct() { // ... } // 发帖方法 public function addPost() { // ... 发帖逻辑 } }
The above is an ordinary post object. As the number of posts and visits increases, the operators start to quit. The company also often receives complaint calls saying that our website contains a lot of sensitive content and spam advertisements, so we need to conduct content review: first, review of users, some blacklisted users should be prohibited from posting; second, review of IP ; The third is the review of content-sensitive words. So our code looks like the following:
class Post { protected $_userid = null; protected $_ip = null; protected $_content = null; function __construct() { } public function addPost() { if (!Postscan::checkUserid($tihs->_userid)) { return false; } if (!Postscan::ipUserid($tihs->_ip)) { return false; } if (!Postscan::checkContent($tihs->_content)) { return false; } // ... } }
As more and more fields need to be reviewed, the addPost method becomes longer and longer, and the publishing object can only be tightly embedded in the system. middle.
Implementation of Observer Pattern
The core of the observer pattern is to separate the observer from the subject. When the subject knows that an event occurs, the observer needs to be notified. At the same time, we do not want to create a conflict between the subject and the observer. The relationship is hard-coded, so let’s modify our code above:
//主体必须实现的接口 interface Observable { public function attach(Observer $observer); public function detach(Observer $observer); public function notify(); } //观察者必须实现的接口 interface Observer { public function do(Observable $subject); } class Post implements Observable { protected $_userid = null; protected $_ip = null; protected $_content = null; protected $_observerlist = array(); function __construct() { } public function attach(Observer $observer) { $this->_observerlist[] = $observer; } public function detach(Observer $observer) { foreach ($this->_observerlist as $key => $value) { if ($observer === $value) { unset($this->_observerlist[$key]) } } } public function notify() { foreach ($this->_observerlist as $value) { if (!$value->do($this)) { return false; } } return true; } public function addPost() { if (!$this->notify()) { return false; } // ... } }
With the above code, we can easily add audit rules.
SPL code
The observer pattern is a very common and commonly used design pattern, so much so that the SPL extension has encapsulated the corresponding classes and methods for us. The following code is based on the three elements provided by SPL: SplObserver, SplSubject , the code implemented by SplObjectStorage
class Post implements SplSubject { protected $_userid = null; protected $_ip = null; protected $_content = null; protected $_storage = new SplObjectStorage(); function __construct() { } public function attach(SplObject $observer) { $this->_storage->attach($observer); } public function detach(SplObject $observer) { $this->_storage->detach($observer); } public function notify() { foreach ($this->_storage as $value) { if (!$value->update($this)) { return false; } } return true; } public function addPost() { if (!$this->notify()) { return false; } // ... } }
is very simple. The most important thing is to understand that in this example, we have separated some auditing methods from the post class, and the post object can also be used as Other publishing types.
The implementation of the above content is the observer mode of PHP design pattern introduced by the editor to you. I hope it will be helpful to everyone!
The above introduces the observer pattern example of PHP design pattern, including the relevant content. I hope it will be helpful to friends who are interested in PHP tutorials.
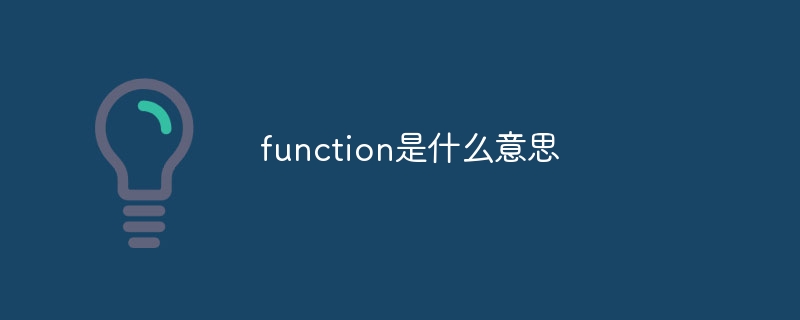
function是函数的意思,是一段具有特定功能的可重复使用的代码块,是程序的基本组成单元之一,可以接受输入参数,执行特定的操作,并返回结果,其目的是封装一段可重复使用的代码,提高代码的可重用性和可维护性。
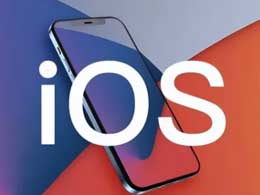
每年Apple发布新的iOS和macOS大版本之前,用户都可以提前几个月下载测试版抢先体验一番。由于公众和开发人员都使用该软件,所以苹果公司为两者推出了developer和public版即开发者测试版的公共测试版。iOS的developer版和public版有什么区别呢?从字面上的意思来说,developer版是开发者测试版,public版是公共测试版。developer版和public版面向的对象不同。developer版是苹果公司给开发者测试使用的,需要苹果开发者帐号才可以收到下载并升级,是
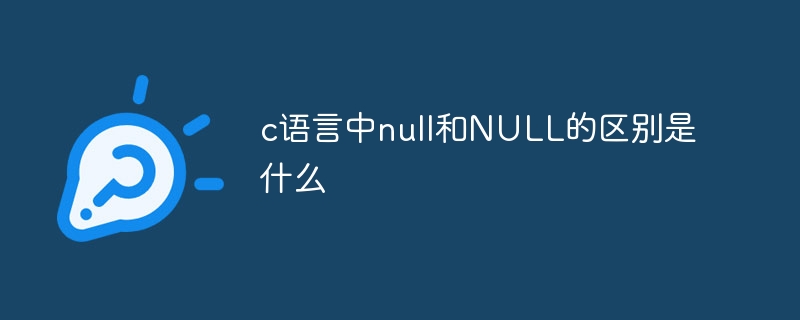
c语言中null和NULL的区别是:null是C语言中的一个宏定义,通常用来表示一个空指针,可以用于初始化指针变量,或者在条件语句中判断指针是否为空;NULL是C语言中的一个预定义常量,通常用来表示一个空值,用于表示一个空的指针、空的指针数组或者空的结构体指针。
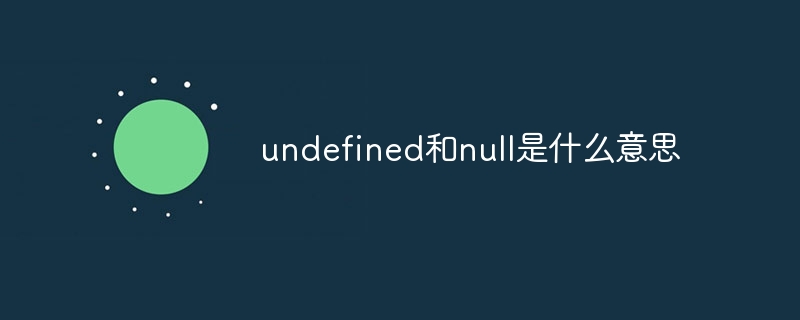
在JavaScript 中,undefined和null都代表着“无”的概念:1、undefined 表示一个未初始化的变量或一个不存在的属性,当声明了一个变量但没有对其赋值时,这个变量的值就是undefined,访问对象中不存在的属性时,返回的值也是undefined;2、null表示一个空的对象引用,在某些情况下,可以将对象的引用设置为null,以便释放其占用的内存。
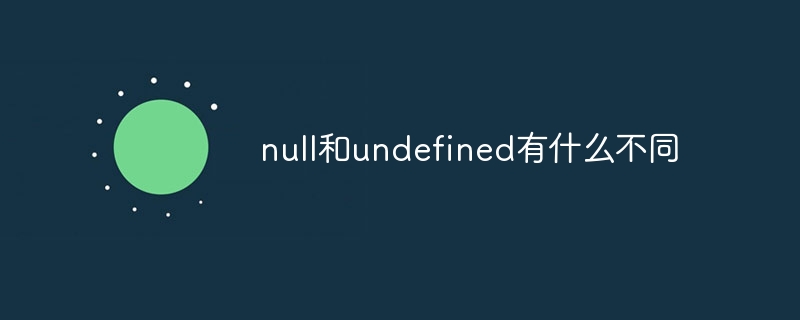
null和undefined的区别在:1、语义含义;2、使用场景;3、与其它值的比较;4、与全局变量的关系;5、与函数参数的关系;6、可空性检查;7、性能考虑;8、在JSON序列化中的表现;9、与类型的关系。详细介绍:1、语义含义,null通常表示知道这个变量不会拥有任何有效的对象值,而undefined则通常表示变量未被赋值,或者对象没有此属性;2、使用场景等等。
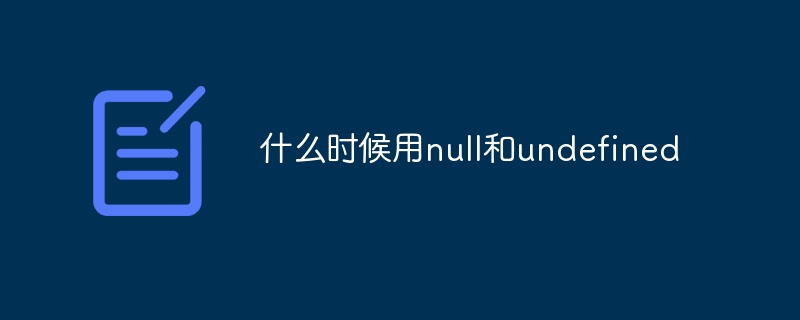
null和undefined都表示缺少值或未定义的状态,根据使用场景的不同,选择使用null还是undefined有以下一些指导原则:1、当需要明确指示一个变量为空或无效时,可以使用null;2、当一个变量已经声明但尚未赋值时,会被默认设置为undefined;3、当需要检查一个变量是否为空或未定义时,使用严格相等运算符“===”来判断变量是否为null或undefined。
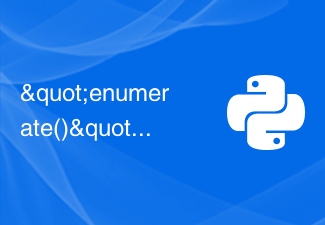
在本文中,我们将了解enumerate()函数以及Python中“enumerate()”函数的用途。什么是enumerate()函数?Python的enumerate()函数接受数据集合作为参数并返回一个枚举对象。枚举对象以键值对的形式返回。key是每个item对应的索引,value是items。语法enumerate(iterable,start)参数iterable-传入的数据集合可以作为枚举对象返回,称为iterablestart-顾名思义,枚举对象的起始索引由start定义。如果我们忽
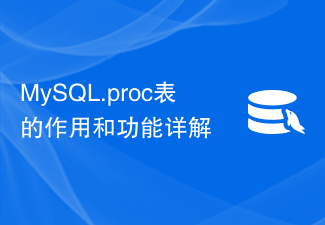
MySQL.proc表的作用和功能详解MySQL是一种流行的关系型数据库管理系统,开发者在使用MySQL时常常会涉及到存储过程(StoredProcedure)的创建和管理。而MySQL.proc表则是一个非常重要的系统表,它存储了数据库中所有的存储过程的相关信息,包括存储过程的名称、定义、参数等。在本文中,我们将详细解释MySQL.proc表的作用和功能


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
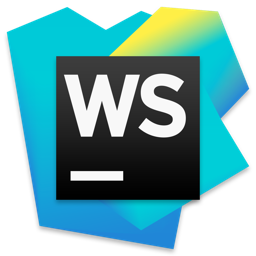
WebStorm Mac version
Useful JavaScript development tools
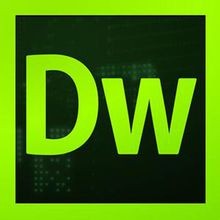
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Chinese version
Chinese version, very easy to use
