[Abstract] Object-oriented programming (OOP) is a basic skill for our programming, and PHP4 provides good support for OOP. How to use OOP ideas to perform advanced programming of PHP is very meaningful for improving PHP programming capabilities and planning a good Web development architecture.
Object-oriented programming (OOP) is a basic skill for our programming, and PHP4 provides good support for OOP. How to use OOP ideas to perform advanced programming of PHP is very meaningful for improving PHP programming capabilities and planning a good Web development architecture. Below we will use examples to illustrate the practical significance and application methods of using PHP's OOP for programming.
When we usually build a website with a database backend, we will consider that the program needs to be suitable for different application environments. What is different from other programming languages is that in PHP, a series of specific functions are used to operate the database (if you do not use the ODBC interface). Although this is very efficient, the encapsulation is not enough. If there is a unified database interface, then we can apply it to a variety of databases without making any modifications to the program, thereby greatly improving the portability and cross-platform capabilities of the program.
To complete OOP in PHP, you need to implement object encapsulation, that is, write a class. We can achieve a simple encapsulation of the database by generating a new SQL class. For example:
PHP:
Copy the code The code is as follows:
class SQL
{
var $Driver; //Actual database driver subclass
var $connection; //Shared database Connection variables
function DriverRegister($d)
{
if($d!="")
{
$include_path = ini_get("include_path");
$DriverFile = $include_path."/".$d.". php";
//The driver storage path must be under the INCLUDE_PATH set in the PHP.ini file
if(file_exists($DriverFile)) //Check whether the driver exists
{
include($DriverFile);
$this- >Driver = new $d();
//Generate the corresponding database driver class based on the driver name
return true;
}
}
return false; //Failed to register the driver
}
function Connect($host,$user ,$passwd,$database)//Function to connect to the database
{
$this->Driver->host=$host;
$this->Driver->user=$user;
$this-> ;Driver->passwd=$passwd;
$this->Driver->database=$database;
$this->connection = $this->Driver->Connect();
}
function Close()//Close database function
{
$this->Driver->close($this->connection);
}
function Query($queryStr)//Database string query function
{
return $this->Driver->query($queryStr,$this->connection);
}
function getRows($res)//Find rows
{
return $this->Driver->getRows( $res);
}
function getRowsNum($res)//Get the row number
{
return $this->Driver-> getRowsNum ($res);
}
}
?>
We operate by MySQL database is an example. We write a database driver class MySQL. In this class, we further encapsulate the functions related to MySQL database operations. Put the file containing this class and the file name is MySQL.php under the PHP system include_path, and it can be used normally. Note that when writing database driver files, the file name should be consistent with the class name.
PHP:
Copy code The code is as follows:
Class MySQL
{
var $host;
var $user;
var $passwd;
var $database;
function MySQL() //利用构造函数实现变量初始化
{
$host = "";
$user = "";
$passwd = "";
$database = "";
}
function Connect()
{
$conn = MySQL_connect($this->host, $this->user,$this->passwd) or
die("Could not con nect to $ this->host");
MySQL_select_db($this->database,$conn) or
die("Could not swi tch to database $ this->database;");
return $conn;
}
function Close($conn)
{
MySQL_close($conn);
}
function Query($queryStr, $conn)
{
$res =MySQL_query($queryStr, $conn) or
die("Could not que ry database");
return $res;
}
function getRows($res)
{
$rowno = 0;
$rowno = MySQL_num_rows($res);
if($rowno>0)
{
for( $row=0;$row{
$rows[$row]=MySQL_fetch_row($res);
}
return $rows;
}
}
function getRowsNum($res)
{
$rowno = 0;
$rowno = mysql_num_rows($res);
return $rowno;
}
}
?>
同样我们要封装其他的“数据库驱动”到我们 的SQL类中,只需要建立相应的类,并以同名命名驱动文件,放到PHP的include 目录就可以了。
完成封装以后,就可以 在PHP中按照OOP的思想来实现对数据库的编程了。
PHP:
复制代码 代码如下:
Include(“SQL.php”);
$sql = new SQL; //生成新的Sql对象
if($sql-> DriverRegister(“MySQL”& lt;font color="#007700">)) //注册数据库驱动
{
$sql->Connect(“localhost”,”root”&l t;font color="#007700">,””,”test”&l t;font color="#007700">);
$res=$sql->query(“select & lt;font color="#007700">* from test”); //返回查询记录集
$rowsnum = $sql->getRowsNum($res);
if($rowsnum > 0)
{
$rows = $sql->getRows($res);
foreach($rows as $row) //循环取出记录集内容
{
foreach($row as $field){
print $field;}
}
}
$sql->Close();
}
?>
在实际应用中,我们还可以根据实际需求对各种对象类做进一步扩 展。在PHP中,还提供了一系列复杂的OOP方法,例如继承,重载,引用,串行化 等等。充分调动各种方法并灵活运用,就能够使你的网站更合理和结构化,开发 和维护也更容易。
以上就介绍了 PHP面向对象编程快速入门,包括了方面的内容,希望对PHP教程有兴趣的朋友有所帮助。
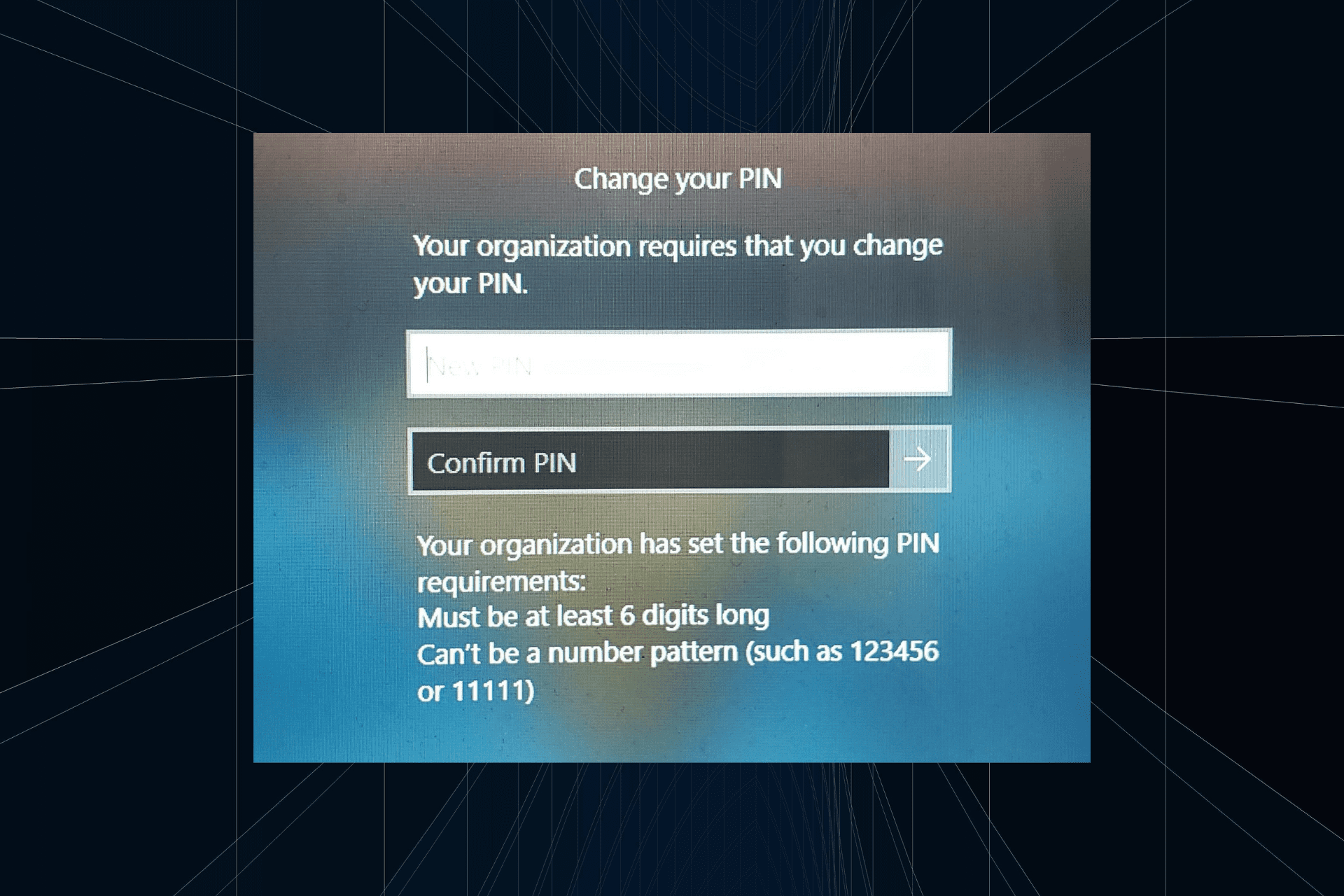
“你的组织要求你更改PIN消息”将显示在登录屏幕上。当在使用基于组织的帐户设置的电脑上达到PIN过期限制时,就会发生这种情况,在该电脑上,他们可以控制个人设备。但是,如果您使用个人帐户设置了Windows,则理想情况下不应显示错误消息。虽然情况并非总是如此。大多数遇到错误的用户使用个人帐户报告。为什么我的组织要求我在Windows11上更改我的PIN?可能是您的帐户与组织相关联,您的主要方法应该是验证这一点。联系域管理员会有所帮助!此外,配置错误的本地策略设置或不正确的注册表项也可能导致错误。即
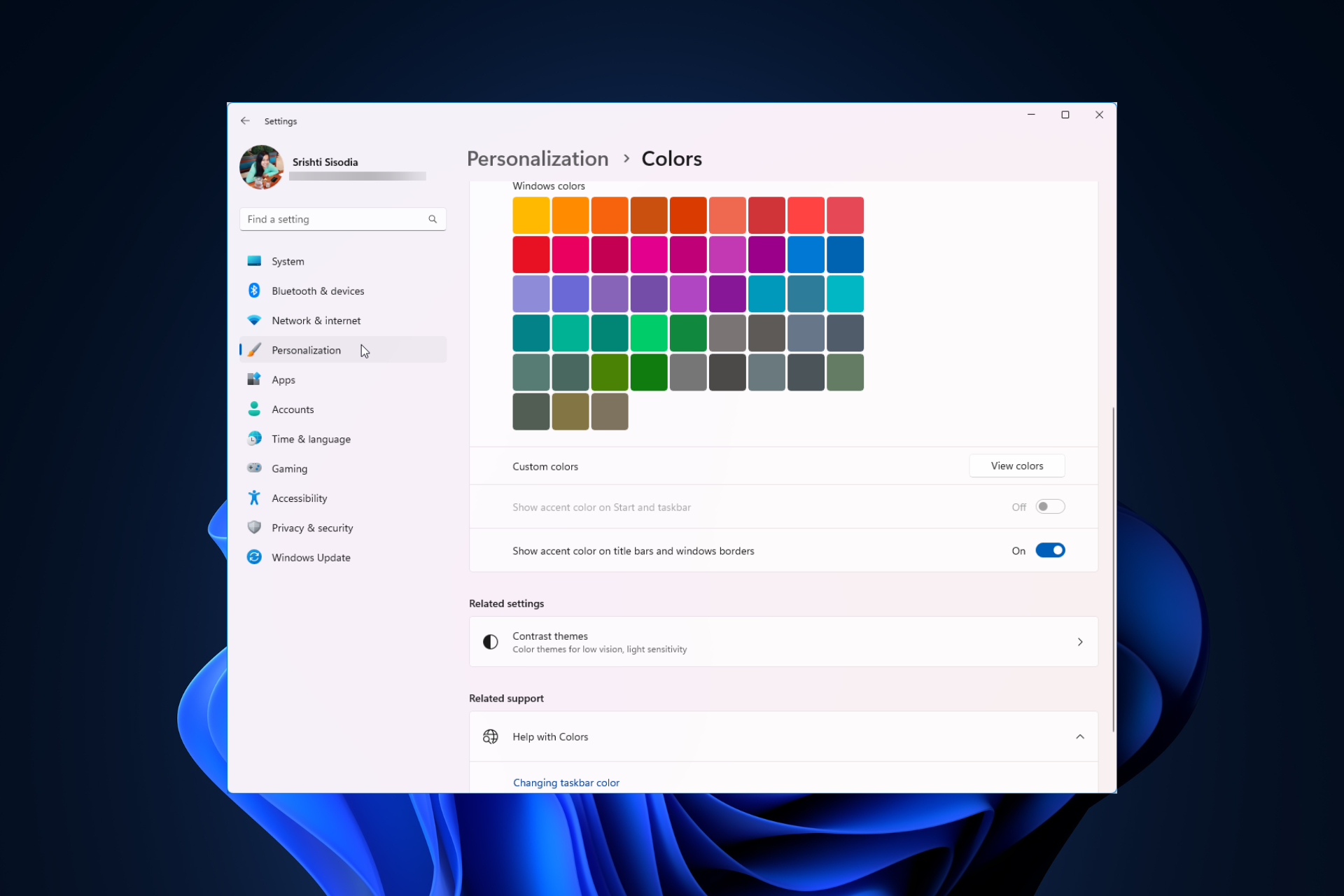
Windows11将清新优雅的设计带到了最前沿;现代界面允许您个性化和更改最精细的细节,例如窗口边框。在本指南中,我们将讨论分步说明,以帮助您在Windows操作系统中创建反映您的风格的环境。如何更改窗口边框设置?按+打开“设置”应用。WindowsI转到个性化,然后单击颜色设置。颜色更改窗口边框设置窗口11“宽度=”643“高度=”500“>找到在标题栏和窗口边框上显示强调色选项,然后切换它旁边的开关。若要在“开始”菜单和任务栏上显示主题色,请打开“在开始”菜单和任务栏上显示主题
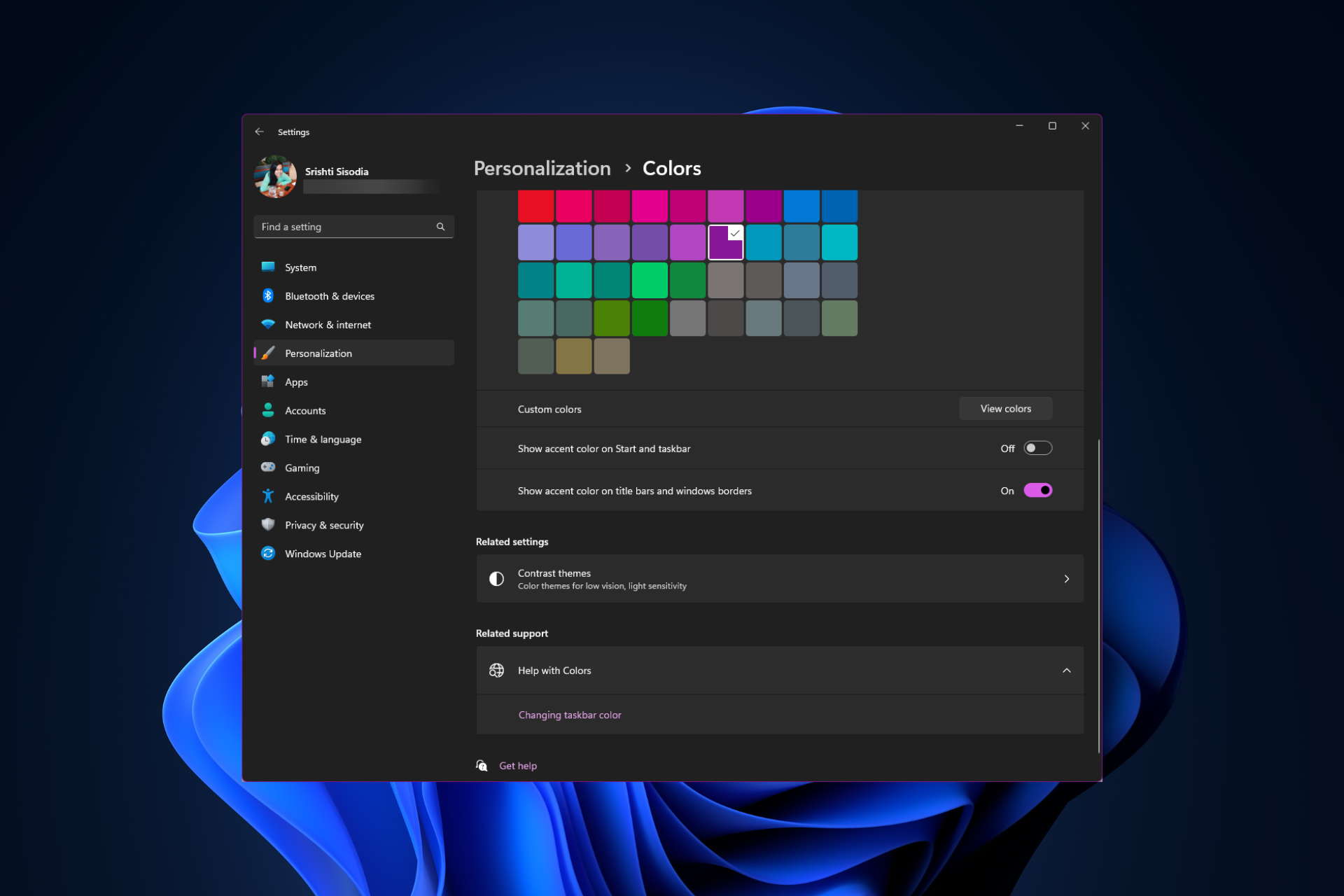
默认情况下,Windows11上的标题栏颜色取决于您选择的深色/浅色主题。但是,您可以将其更改为所需的任何颜色。在本指南中,我们将讨论三种方法的分步说明,以更改它并个性化您的桌面体验,使其具有视觉吸引力。是否可以更改活动和非活动窗口的标题栏颜色?是的,您可以使用“设置”应用更改活动窗口的标题栏颜色,也可以使用注册表编辑器更改非活动窗口的标题栏颜色。若要了解这些步骤,请转到下一部分。如何在Windows11中更改标题栏的颜色?1.使用“设置”应用按+打开设置窗口。WindowsI前往“个性化”,然
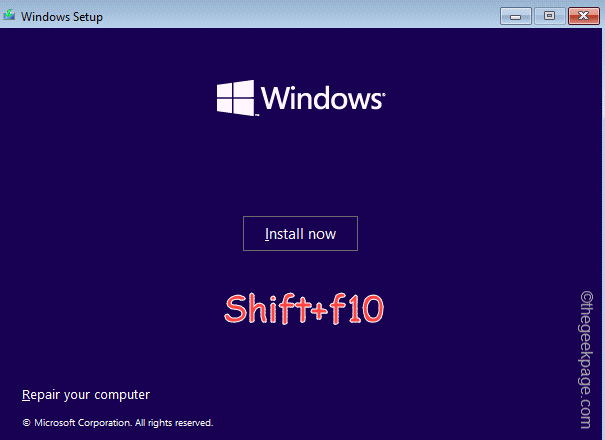
您是否在Windows安装程序页面上看到“出现问题”以及“OOBELANGUAGE”语句?Windows的安装有时会因此类错误而停止。OOBE表示开箱即用的体验。正如错误提示所表示的那样,这是与OOBE语言选择相关的问题。没有什么可担心的,你可以通过OOBE屏幕本身的漂亮注册表编辑来解决这个问题。快速修复–1.单击OOBE应用底部的“重试”按钮。这将继续进行该过程,而不会再打嗝。2.使用电源按钮强制关闭系统。系统重新启动后,OOBE应继续。3.断开系统与互联网的连接。在脱机模式下完成OOBE的所
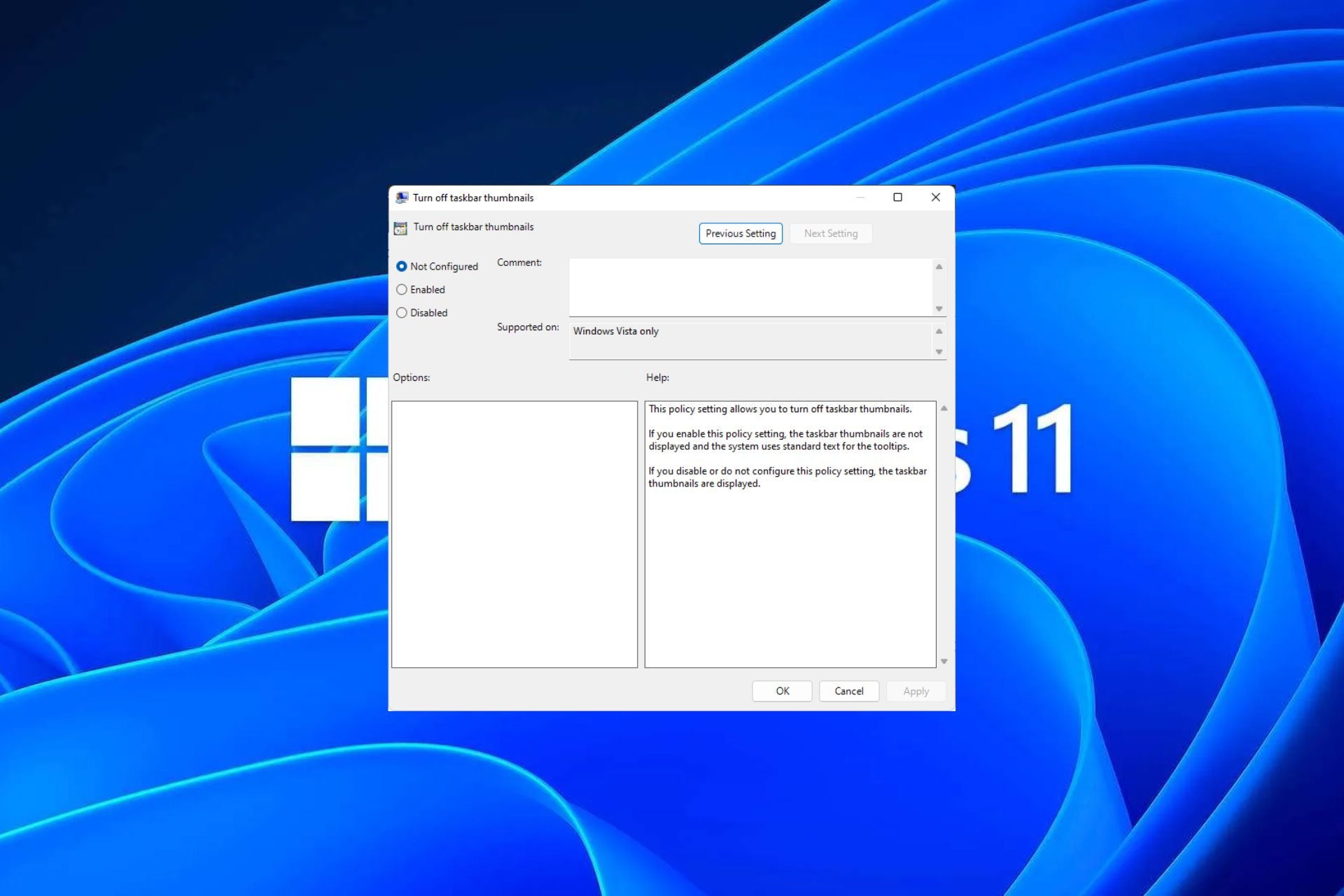
任务栏缩略图可能很有趣,但它们也可能分散注意力或烦人。考虑到您将鼠标悬停在该区域的频率,您可能无意中关闭了重要窗口几次。另一个缺点是它使用更多的系统资源,因此,如果您一直在寻找一种提高资源效率的方法,我们将向您展示如何禁用它。不过,如果您的硬件规格可以处理它并且您喜欢预览版,则可以启用它。如何在Windows11中启用任务栏缩略图预览?1.使用“设置”应用点击键并单击设置。Windows单击系统,然后选择关于。点击高级系统设置。导航到“高级”选项卡,然后选择“性能”下的“设置”。在“视觉效果”选
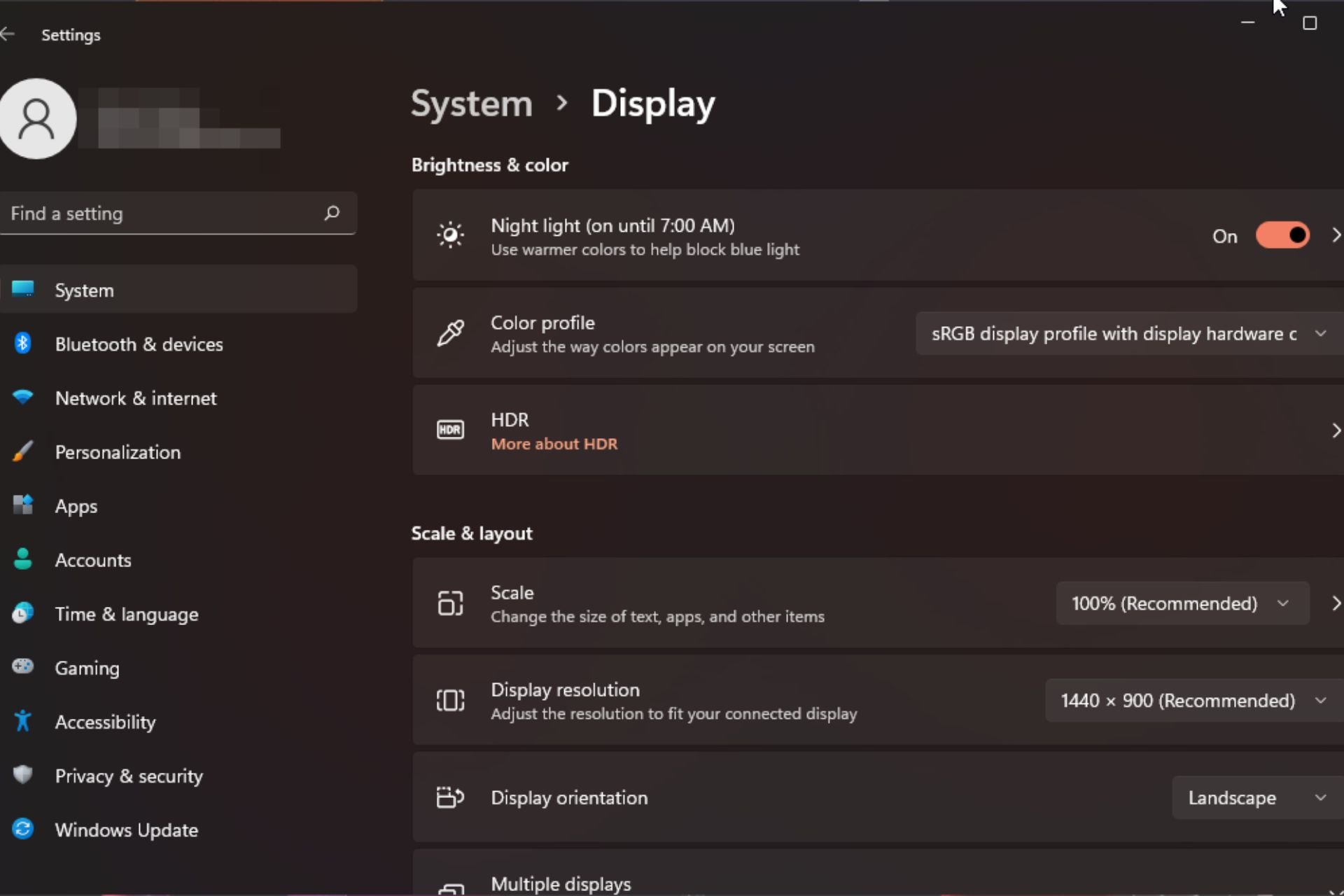
在Windows11上的显示缩放方面,我们都有不同的偏好。有些人喜欢大图标,有些人喜欢小图标。但是,我们都同意拥有正确的缩放比例很重要。字体缩放不良或图像过度缩放可能是工作时真正的生产力杀手,因此您需要知道如何对其进行自定义以充分利用系统功能。自定义缩放的优点:对于难以阅读屏幕上的文本的人来说,这是一个有用的功能。它可以帮助您一次在屏幕上查看更多内容。您可以创建仅适用于某些监视器和应用程序的自定义扩展配置文件。可以帮助提高低端硬件的性能。它使您可以更好地控制屏幕上的内容。如何在Windows11
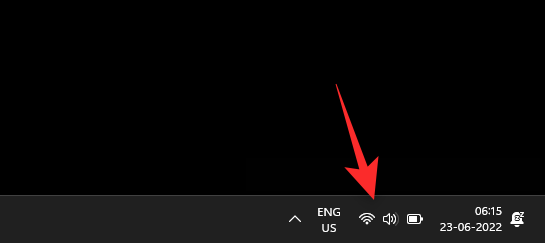
屏幕亮度是使用现代计算设备不可或缺的一部分,尤其是当您长时间注视屏幕时。它可以帮助您减轻眼睛疲劳,提高易读性,并轻松有效地查看内容。但是,根据您的设置,有时很难管理亮度,尤其是在具有新UI更改的Windows11上。如果您在调整亮度时遇到问题,以下是在Windows11上管理亮度的所有方法。如何在Windows11上更改亮度[10种方式解释]单显示器用户可以使用以下方法在Windows11上调整亮度。这包括使用单个显示器的台式机系统以及笔记本电脑。让我们开始吧。方法1:使用操作中心操作中心是访问
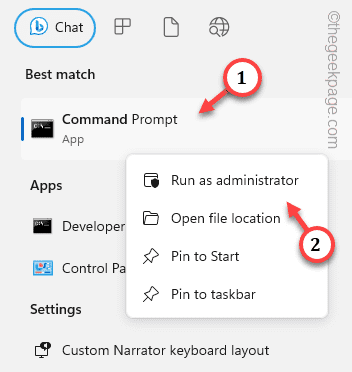
Windows上的激活过程有时会突然转向显示包含此错误代码0xc004f069的错误消息。虽然激活过程已经联机,但一些运行WindowsServer的旧系统可能会遇到此问题。通过这些初步检查,如果这些检查不能帮助您激活系统,请跳转到主要解决方案以解决问题。解决方法–关闭错误消息和激活窗口。然后,重新启动计算机。再次从头开始重试Windows激活过程。修复1–从终端激活从cmd终端激活WindowsServerEdition系统。阶段–1检查Windows服务器版本您必须检查您使用的是哪种类型的W


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
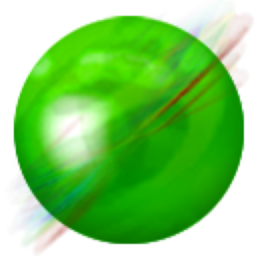
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment