Building a simple interactive website (2)
5.5 Counter
Let us add a counter to the homepage. This example has been told many times, but it is still useful to demonstrate how to read and write files and create your own functions. counter.inc contains the following code:
/*
|| A simple counter
*/
function get_hitcount($counter_file)
{
/* Return the counter to zero
This way, if the counter has not been used, the initial The value will be 1
Of course you can also set the initial value to 20000 to fool people
*/
$count=0;
// If the file to store the counter already exists, read its contents
if ( file_exists($counter_file ) )
{
$fp=fopen($counter_file,"r");
// We only took the top 20, I hope your site will not be too popular
$count=0+fgets($fp,20 );
// Since the function fgets() returns a string, we can automatically convert it to an integer by adding 0
fclose($fp);
// The file operation is completed
}
// Increase the count value once
$count++;
//Write the new count value to the file
$fp=fopen($counter_file,"w");
fputs($fp,$count);
fclose($fp);
# Return count value
return ($count);
}
?>
Then we change the front.php3 file to display this counter:
include("include/counter.inc");
// I put the count value Place it in the file counter.txt, read and output
printf ("
n",
get_hitcount("counter.txt" ));
include("include/footer.inc");
?>
Check out our new front.php3
5.6 Feedback Form
Let us add another feedback form for your visitors to fill out and e-mail for you. For example, we use a very simple method to implement it. We only need two pages: one to provide the viewer with an input form; the other to obtain the form data, process it, and mail it to you.
Getting form data in PHP is very simple. When a form is sent, each element contained in the form is assigned a corresponding value, and can be used like a reference to a general variable.
> ;
In process_form.php3, the variable $mytext is assigned the entered value - very simple! Similarly, you can get variable values from form elements such as list boxes, check boxes, radio boxes, buttons, etc. The only thing you have to do is give each element in the form a name so that you can reference it later.
According to this method, we can generate a simple form containing three elements: name, e-mail address and message. When the visitor sends the form, the PHP page (sendfdbk.php3) that processes the form reads the data, checks whether the name is empty, and finally emails the data to you.
Form: form.php3
include("include/common.inc");
$title = "Feedback";
include("include/header.inc");
?>
if ( ! empty($searchstr) )
{
// empty() is used to check whether the query string is empty
// If it is not empty, call grep query
echo "
n";
// call grep queries all files in case-insensitive mode
$cmdstr = "grep -i $searchstr *";
$fp = popen( $cmdstr, "r" ); // Execute the command and output the pipe
$myresult = array(); // Store query results
while( $buffer = fgetss ($fp, 4096))
{
{
// grep returns this format: File name: the number of lines in the matching string
// So we use the function split () Separate and process data
list($fname, $fline) = split(":",$buffer, 2);
// We only output the result of the first match
if ( !defined($myresult[$fname ]))
$myresult[$fname] = $fline;
}
// Now we store the results in the array, we can process and output them
if ( count($myresult) )
{
echo "< ;OL>n";
while(list($fname,$fline) = each($myresult))
echo "
$fname : $fline
echo "n";
}
else
{
// If there are no query results
echo "Sorry. Search on $searchstr> ;
returned no results.
n";
}
pclose($fp);
}
?>
include("include/footer.inc");
?>
Comments :
PHP_SELF is a built-in variable in PHP. Contains the current file name.
fgets() reads the file line by line, with a maximum length of 4096 (specified) characters.
fgetss() is similar to fgets(), except that it parses the output HTML tags.
split() has a parameter of 2, because we only need to split the output into two parts. Also need to omit ":".
each() is an array operation function, used to traverse the entire array more conveniently.
popen(), pclose() have very similar functions to fopen(), fclose(), except that pipeline processing is added.
Please note that the above code is not a good way to implement a search engine. This is just an example to help us learn PHP better. Ideally you should build a database of keywords and then search them.
The above has introduced the PHP novice on the road (6), including the content of the novice driving on the road. I hope it will be helpful to friends who are interested in PHP tutorials.
🎜
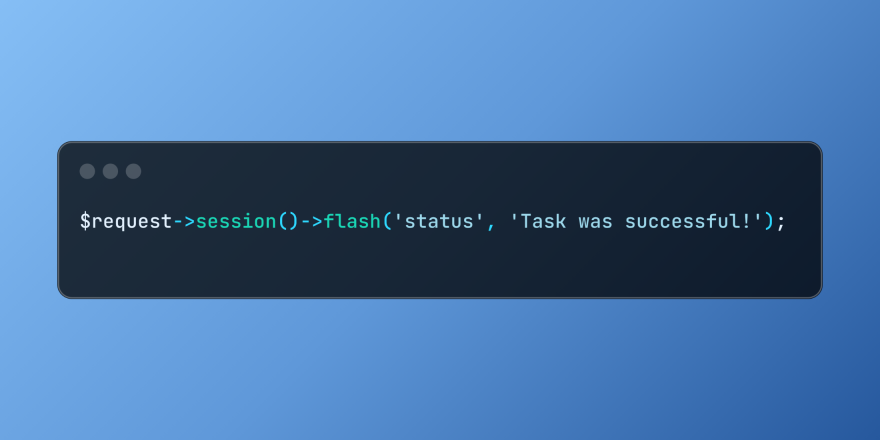
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
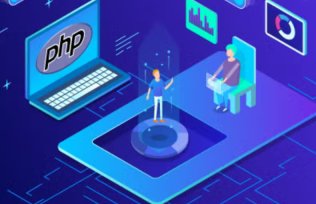
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
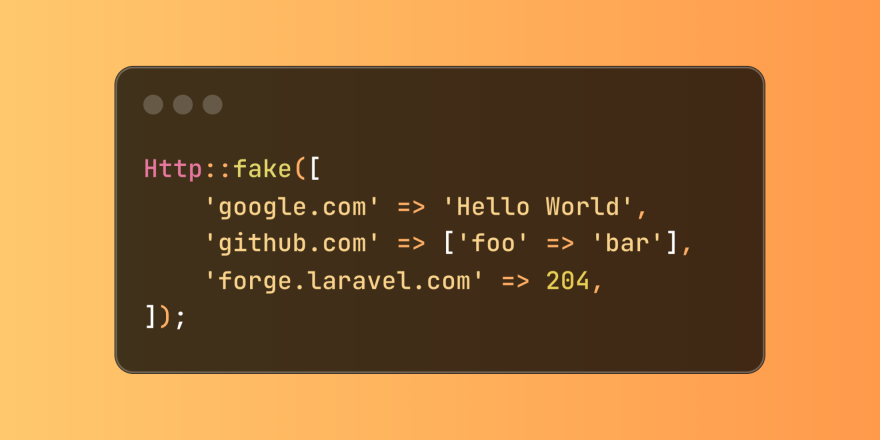
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
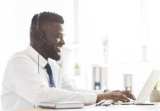
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
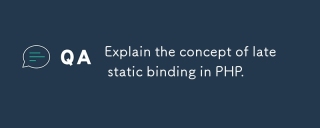
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
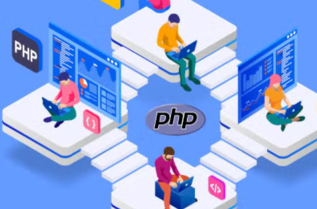
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
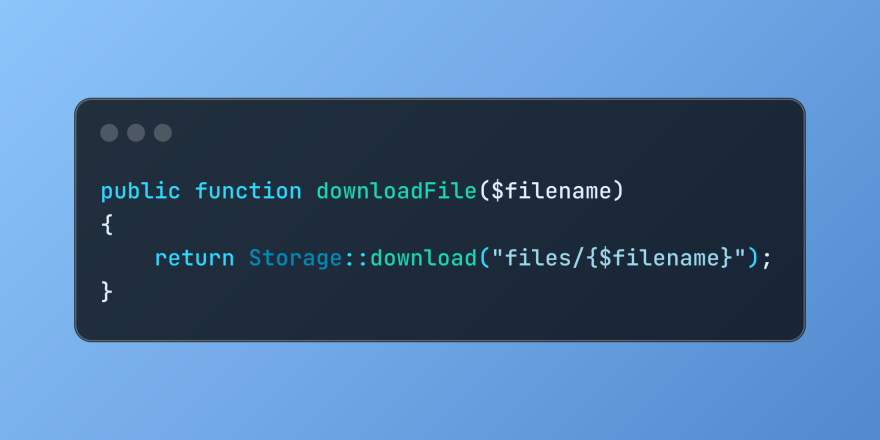
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:
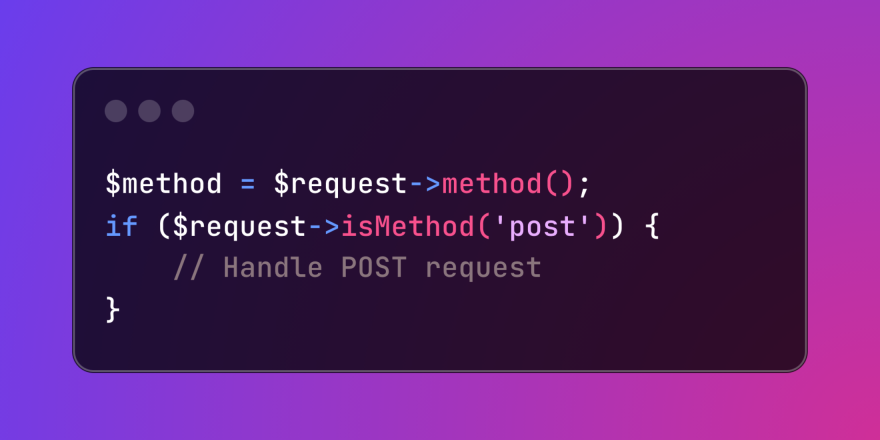
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
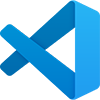
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
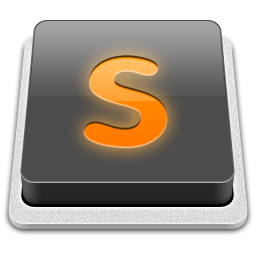
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
