With the growth in the number of rich web applications and users' high expectations for fast interactive responses, developers have begun to use JavaScript libraries to complete some repetitive tasks quickly and efficiently. One of the most popular JavaScript libraries is jQuery. But the large number of applications of jQuery has brought another question: What are the best practices and what are the bad practices when using JavaScript libraries?
Background
In this article, I will introduce you to some good practices (at least in my opinion) when writing, debugging and reviewing JavaScript code. In fact, I selected 7 of the most common scenarios.
1. Use CDN and its fallback address (fallback)
CDN stands for Content Delivery Network and is a server that caches JavaScript files. After using a CDN, your application can use the CDN cache instead of reloading the library files from your server every time a new user initiates a request. Google, Microsoft and JQuery all provide CDN services.
Given that the network is not always 100% reliable and the server may go down for some reason, you must ensure that your application can still run normally even if these things happen. At this time we need to use the fallback address: when the application cannot find the cache library, it will fall back and use the server file.
Google CDN is like this:
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"> </script>
Microsoft CDN is like this:
<script src="//ajax.aspnetcdn.com/ajax/jquery/jquery-1.9.0.min.js"> </script>
It should be noted that we did not specify the URL protocol as http but used //. This is because the CDN server supports http and https. If your website has SSL certification, you can load files normally without modification.
Also, like I mentioned before, we also need a fallback address in case there is a problem with the CDN server.
<script>Window.JQuery || document.write(‘<script src=”script/localsourceforjquery”></script>’)
Of course, you can also use Require to configure the jQuery you need, but I think this is good.
2. Limit DOM interaction
Manipulating the DOM tree with JavaScript has a performance cost. The same goes for jQuery. So, try to minimize interaction with the DOM. When I was helping a colleague of mine improve the speed of displaying data, I saw him using a selector inside a loop. This is a performance killer! This is what he wrote:
containerDiv = $("#contentDiv"); for(var d =0; d < TotalActions; d++) { containerDiv.append("<div><span class='brilliantRunner'>" + d + "</span></div>"); }
What’s the problem? At first glance, there’s nothing wrong with it. And my colleagues also said that this code is very fun to run! I'm really pissed off! When TotalActions is less than 50, no problem is noticed; but when it reaches 25,000, the speed slows down a lot. The reason (I also found it through Google) is that DOM interaction is placed in the loop.
For this feature, (after many failed attempts) I replaced the direct DOM interaction in the loop with a push operation on an array, and then joined the arrays with an empty string as the delimiter. In the end, the program certainly became smoother and more efficient.
var myContent=[]; for(var d = 0; d < TotalActions; d++) { myContent.push("<div><span class='brilliantRunner'>" + d + "</span></div>"); } containerDiv.html(myContent.join(""));
3. Cache
The most important and distinctive thing about jQuery is its selector and the way to find HTML elements in the DOM tree. However, I have seen many times that some developers call the same selector multiple times in the same function, such as $("#divid"). Although jQuery selects elements very quickly, don't look for the same element every time. So, you can cache your elements like this:
var $divId = $("#divId")
Then in the following code, you can use $divId.
For the code below:
var thefunction = function() { $("#mydiv").ToggleClass("zclass"); $("#mydiv").fadeOut(800); } var thefunction2 = function() { $("#mydiv").addAttr("name"); $("#mydiv").fadeIn(400); }
We can modify it like this and use chain syntax to make it look more beautiful:
var mydiv =$("#mydiv"); var thefunction = function() { mydiv.ToggleClass("zclass").fadeOut(800); } var thefunction2 = function() { mydiv.addAttr("name").fadeIn(400); }
But then again, you don’t have to cache everything every time. Look at the example below:
$("#link").click(function() { $(this).addClass("gored"); }
在这里,我既没有用 $(“#link”),或者将其缓存起来,而是使用的$(this)。因为在这个例子中,我操作的对象就是这个链接本身。
4、find 和 filter
最近,在使用find()来获取jQuery对象结合的时候,我产生了一些困惑。然后我发现,这个操作可以替换为用filter()方法来实现。理解这两者的区别非常重要:
find: 将会从选定的元素开始,一直向下查找DOM树
filter: 是在jQuery集合当中查找
5、end()
当在jQuery集合中进行链式操作的时候,我有时候需要回到父对象去进行一些操作。比如你正在一个表格的第二行应用CSS,然后希望回到表格对象,对其添加一些样式。在你对行应用完样式之后,只要使用end()方法,你就会自动回到表格对象,然后随意的对其添加样式吧!
(译者注:find()、filter()和end()原文是大写,其实应该是小写)
6、对象字面量
当你通过链式语法来操作元素的CSS属性的时候,你可以使用对象字面量方式来提升性能。比如这段代码:
$("#myimg").attr("src", "thepath").attr("alt", "the alt text");
变成下面这样之后,不仅避免了操作DOM元素,而且还不用多次调用相关的设置方法:
$("#myimg").attr({"src": "thepath", "alt": "the alt text"});
7、善用CSS类
尽可能使用CSS类而不要写内联CSS代码。我想这一点就不需要举例说明了吧。
希望这篇文章能够帮助你编写更好的jQuery应用程序,真正的帮助到大家。
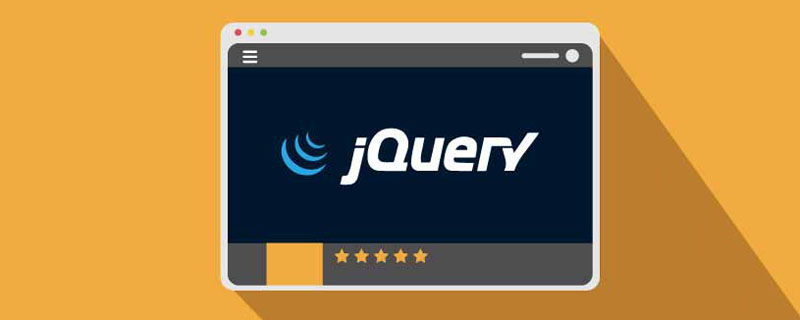
实现方法:1、用“$("img").delay(毫秒数).fadeOut()”语句,delay()设置延迟秒数;2、用“setTimeout(function(){ $("img").hide(); },毫秒值);”语句,通过定时器来延迟。
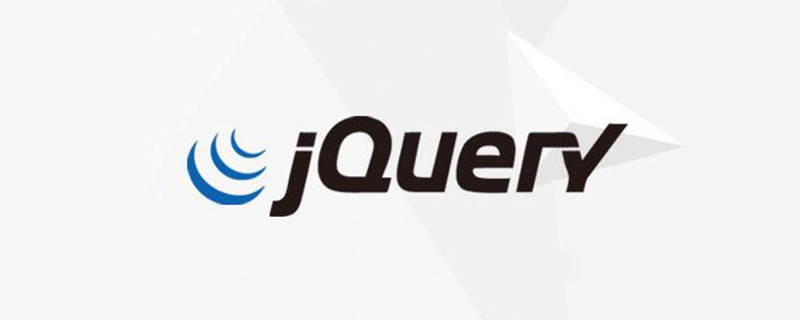
修改方法:1、用css()设置新样式,语法“$(元素).css("min-height","新值")”;2、用attr(),通过设置style属性来添加新样式,语法“$(元素).attr("style","min-height:新值")”。
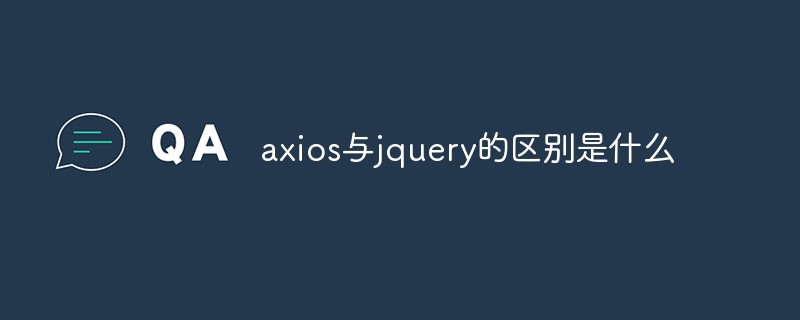
区别:1、axios是一个异步请求框架,用于封装底层的XMLHttpRequest,而jquery是一个JavaScript库,只是顺便封装了dom操作;2、axios是基于承诺对象的,可以用承诺对象中的方法,而jquery不基于承诺对象。
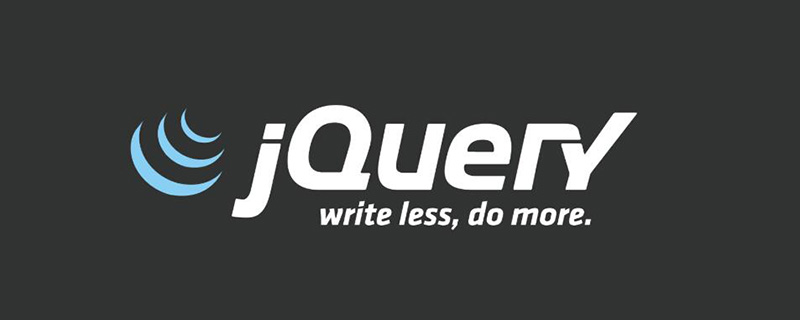
增加元素的方法:1、用append(),语法“$("body").append(新元素)”,可向body内部的末尾处增加元素;2、用prepend(),语法“$("body").prepend(新元素)”,可向body内部的开始处增加元素。
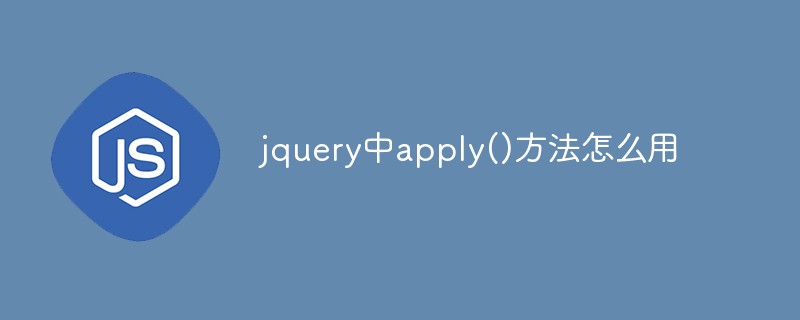
在jquery中,apply()方法用于改变this指向,使用另一个对象替换当前对象,是应用某一对象的一个方法,语法为“apply(thisobj,[argarray])”;参数argarray表示的是以数组的形式进行传递。
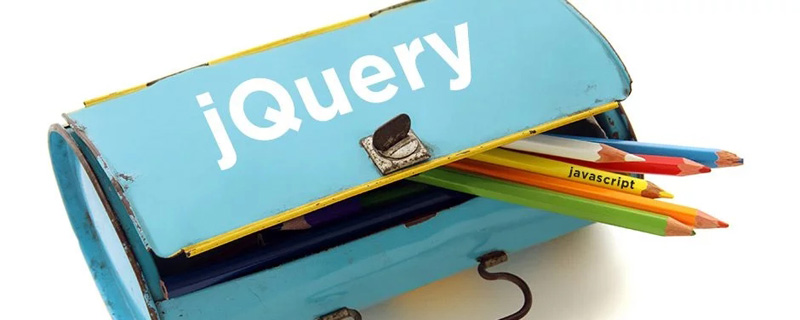
删除方法:1、用empty(),语法“$("div").empty();”,可删除所有子节点和内容;2、用children()和remove(),语法“$("div").children().remove();”,只删除子元素,不删除内容。
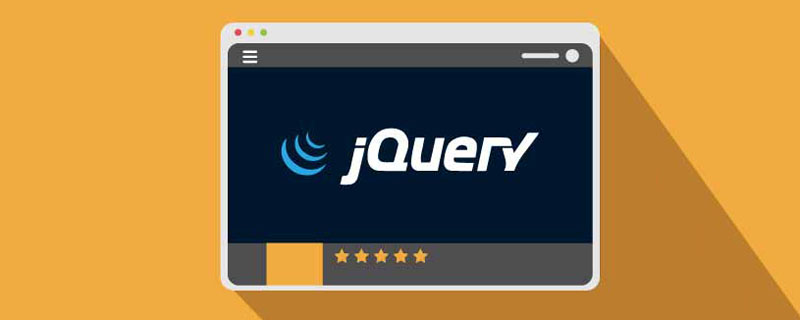
on()方法有4个参数:1、第一个参数不可省略,规定要从被选元素添加的一个或多个事件或命名空间;2、第二个参数可省略,规定元素的事件处理程序;3、第三个参数可省略,规定传递到函数的额外数据;4、第四个参数可省略,规定当事件发生时运行的函数。
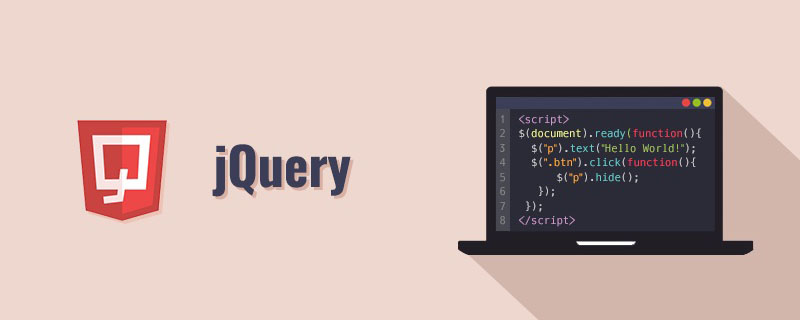
去掉方法:1、用“$(selector).removeAttr("readonly")”语句删除readonly属性;2、用“$(selector).attr("readonly",false)”将readonly属性的值设置为false。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
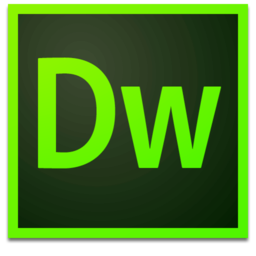
Dreamweaver Mac version
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
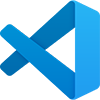
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Chinese version
Chinese version, very easy to use
