


Use single quotes instead of double quotes to enclose strings, it will be faster. Because PHP will search for variables in strings surrounded by double quotes, single quotes will not. Note: only echo can do this, it is a "function" that can take multiple strings as parameters (Annotation: PHP Manual It is said that echo is a language structure, not a real function, so the function is enclosed in double quotes).
28. Try to cache as much as possible, you can use memcached. memcached is a high-performance in-memory object caching system that can be used to accelerate dynamic web applications and reduce database load. Caching of OP codes is useful so that scripts do not have to be recompiled for each request.
29. When operating a string and need to check whether its length meets certain requirements, you will naturally use the strlen() function. This function executes quite quickly because it does not do any calculations and just returns the known length of the string stored in the zval structure (C's built-in data structure used to store PHP variables). However, since strlen() is a function, it will be somewhat slow, because the function call will go through many steps, such as lowercase letters (Annotation: refers to the lowercase function name, PHP does not distinguish between uppercase and lowercase function names), hash search, Will be executed together with the called function. In some cases, you can use the isset() trick to speed up the execution of your code.
(Example below)
if (strlen($foo)
(Compare with the following technique)
if ( ! isset($foo{5) })) { echo "Foo is too short"; }
Calling isset() happens to be faster than strlen(), because unlike the latter, isset(), as a language construct, means that its execution does not require Function search and lowercase letters. That is, you don't actually spend much overhead in the top-level code checking the string length.
34. When executing the increment or decrement of variable $i, $i++ will be slower than ++$i. This difference is specific to PHP and does not apply to other languages, so please don't modify your C or Java code and expect it to be instantly faster, it won't work. ++$i is faster because it only requires 3 instructions (opcodes), while $i++ requires 4 instructions. Post-increment actually creates a temporary variable that is subsequently incremented. Prefix increment increases directly on the original value. This is a form of optimization, as done by Zend's PHP optimizer. It's a good idea to keep this optimization in mind because not all command optimizers do the same optimizations, and there are a large number of Internet Service Providers (ISPs) and servers that don't have command optimizers installed.
35. It doesn’t have to be object-oriented (OOP). Object-oriented is often very expensive, and each method and object call consumes a lot of memory.
36. It is not necessary to use classes to implement all data structures, arrays are also very useful.
37. Don’t subdivide the methods too much. Think carefully about which codes you really plan to reuse?
38. When you need, you can always break the code into methods.
39. Try to use as many PHP built-in functions as possible.
40. If there are a large number of time-consuming functions in the code, you can consider using C extensions to implement them.
41. Profile your code. The checker will tell you which parts of the code take how much time. The Xdebug debugger includes inspection routines that evaluate the overall integrity of your code and reveal bottlenecks in your code.
42. mod_zip can be used as an Apache module to instantly compress your data and reduce data transmission volume by 80%.
43. When file_get_contents can be used instead of file, fopen, feof, fgets and other series of methods, try to use file_get_contents because it is much more efficient! But pay attention to the PHP version problem of file_get_contents when opening a URL file;
44. Perform as few file operations as possible, although PHP’s file operation efficiency is not low; 45. Optimize the SELECT SQL statement, where possible Perform as few INSERT and UPDATE operations as possible (I was criticized on the update page);
46. Use PHP internal functions as much as possible (but in order to find a function that does not exist in PHP, I wasted what I could have done) The time to write a custom function is a matter of experience! );
47. Do not declare variables inside the loop, especially large variables: objects (this seems to be not just a problem in PHP?); 48. Try not to loop and nest assignments in multi-dimensional arrays;
49. Do not use regular expressions when you can use PHP’s internal string manipulation functions;
50. foreach is more efficient, try to use foreach instead of while and for loops;
51. Use single quotes instead of double quotes Quote string;
52. "Use i+=1 instead of i=i+1. It conforms to the habits of c/c++ and is more efficient";
53. For global variables, you should unset() them after use.
Receive LAMP Brothers’ original PHP tutorial CD/"Essential PHP in Details" for free. For details, please contact the official website customer service: http://www.lampbrother.net
PHPCMSSecondary development http: //yun.itxdl.cn/online/phpcms/index.php?u=5
WeChat development
Mobile Internet Server Side Development http://yun.itxdl.cn/online/server/index.php?u=5
JavascriptCourse http:// yun.itxdl.cn/online/js/index.php?u=5
CTOTraining Camp 5
|
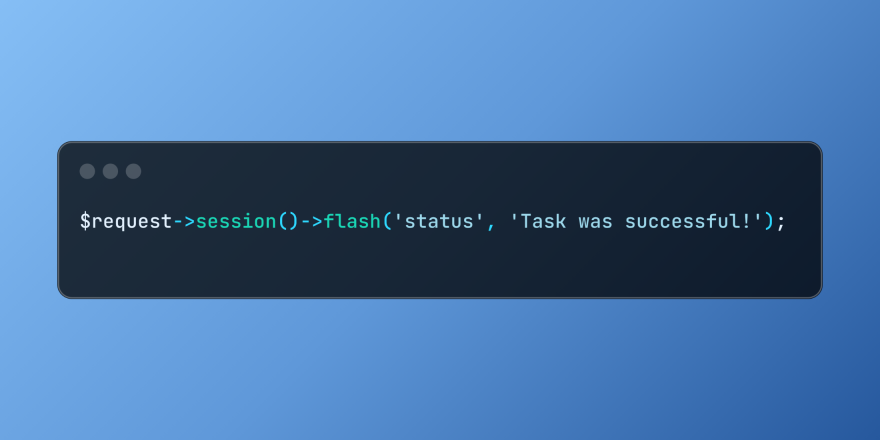
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
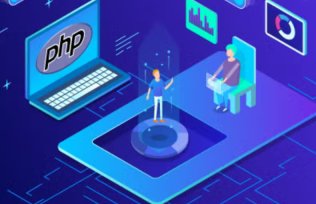
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
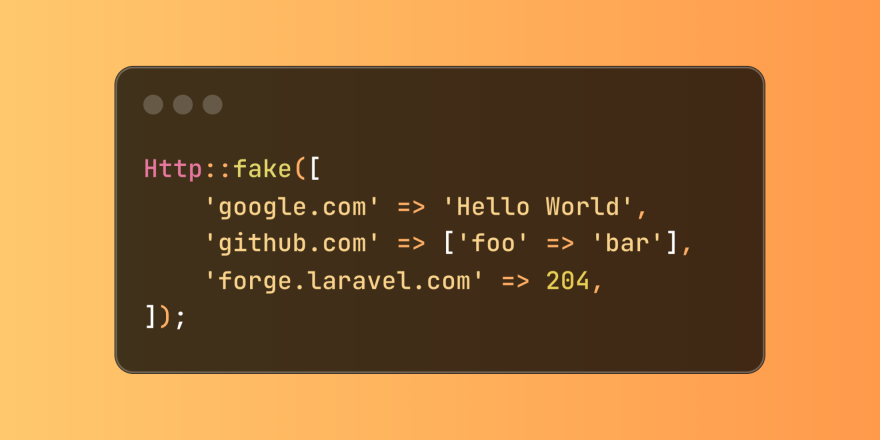
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
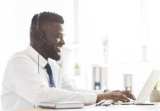
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
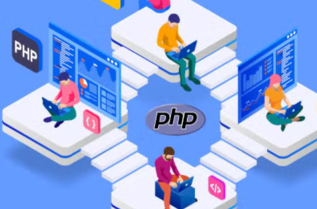
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot
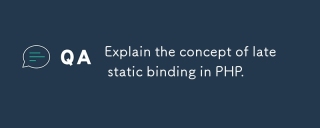
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
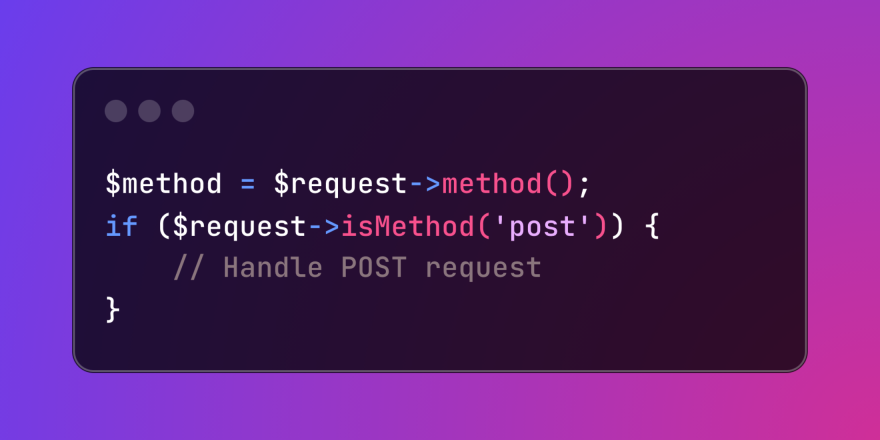
Laravel simplifies HTTP verb handling in incoming requests, streamlining diverse operation management within your applications. The method() and isMethod() methods efficiently identify and validate request types. This feature is crucial for building
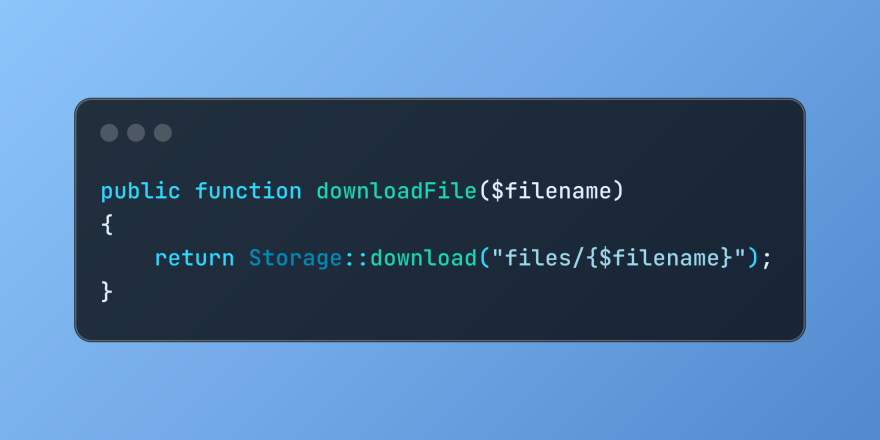
The Storage::download method of the Laravel framework provides a concise API for safely handling file downloads while managing abstractions of file storage. Here is an example of using Storage::download() in the example controller:


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
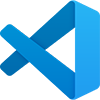
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
