Saving Checkbox data in the database in PHP (1)_PHP tutorial
Introduction
Checkbox is a very useful page form item. When allowing users to make multiple selections, it can even allow users to select all items or none. However, while this is a great form element, in our work there is always some confusion about how to properly save selections. This article will describe how to correctly save checkbox selections in the database while following good database design principles.
Requirements
This article will explain how to correctly save the selections in the user database. Although useful PHP code is included here, I will express it from a database design perspective, so you can easily implement it using any database and server-side scripting language. I just want to provide a how-to that you can apply to your own site. If you want to run the source code here, you need to install php, mysql and a web server.
Example 1: Recruitment site
Suppose you are asked to make a recruitment website that allows job-seeking software developers to fill in their skills so that employers can access the site and base their job search results on job seekers. Skills to find the right employees. You also know that a developer can have more than one skill set, so you decide to design your site that way.
Every job seeker will be allowed to visit this site, register as a user, and enter his skills. Checkbox will come in handy. You may want to make a page like this:
__ PHP __ MySQL __ Zope
__ Perl __ Javascript __ JSP
[Submit]
Every job applicant can choose the skills he possesses. Obviously the options are different for different people. One might be PHP and MySQL, others might just be JSP. How will you save these selections? A natural idea is to create a field for each option so that it works fine initially. But then you may find that trouble comes when you want to expand or adjust, and you may have to modify your table structure.
A good method should be like this:
You should have a user table containing the user's registration information, such as username, password and other content you need. If you directly use the source code given later in this article, you need to build a simple table as follows:
id username
1 User1
2 User2
3 User3
us First create a table "const_skills" using the following SQL statement:
SQL> CREATE TABLE const_skills (
id int not null primary key,
value varchar(20) );
Now we add skills:
SQL> INSERT INTO const_skills(id, value) VALUES (1, "PHP");
SQL> INSERT INTO const_skills(id, value) VALUES (2, "MySQL ");
SQL> INSERT INTO const_skills(id, value) VALUES (3, "Zope");
SQL> INSERT INTO const_skills(id, value) VALUES (4, "Perl");
SQL> INSERT INTO const_skills(id, value) VALUES (5, "Javascript");
SQL> INSERT INTO const_skills(id, value) VALUES (6, "JSP");
Your const_skills It should now look like this:
id value
1 PHP
2 MySQL
3 Zope
4 Perl
5 Javascript
6 JSP
This table only allows users to select the corresponding skills. Now, create another table lookup_skills using the following SQL:
SQL> CREATE TABLE lookup_skills (
id int not null auto_increment primary key,
uid int ,
skill_id int );
The purpose of this table lookup_skills is to provide a mapping relationship from the user table to the development skills table. In other words, it lets us save developers and the skills they have, so when a candidate completes their selection and clicks submit, we will fill in the form with those values selected in the checkbox. For each selected skill, we add a record to this table and record the user ID and the ID of the selected item. (I believe everyone knows this. I translated this, hehe...)
Before we look at the code for inserting records, let’s design this page first. There should be a form for the content that we can query database and take the checkbox label from the const_skills table to create this checkbox form item.
代码如下:
/* insert code to connect to your database here */
/* get the checkbox labels */
$skills = get_checkbox_labels("const_skills");
/* create the html code for a formatted set of
checkboxes */
$html_skills = make_checkbox_html($skills, 3, 400, "skills[]");
? >
Check off your web development skills:
function get_checkbox_labels($table_name) {
/* make an array */
$arr = array();
/* construct the query */
$query = "SELECT * FROM $table_name";
/* execute the query */
$qid = mysql_query($query);
/* each row in the result set will be packaged as
an object and put in an array */
while($row= mysql_fetch_object($qid)) {
array_push($arr, $row);
}
return $arr;
}
/* Prints a nicely formatted table of checkbox choices.
$arr is an array of objects that contain the choices
$num is the number of elements wide we display in the table
$width is the value of the width parameter to the table tag
$name is the name of the checkbox array
$checked is an array of element names that should be checked
*/
function make_checkbox_html($arr, $num, $width, $name, $checked) {
/* create string to hold out html */
$str = "";
/* make it */
$str .= "n";
$str .= "n";
/* determine if we will have to close add
a closing tr tag at the end of our table */
if (count($arr) % $num != 0) {
$closingTR = true;
}
$i = 1;
if (isset($checked)) {
/* if we passed in an array of the checkboxes we want
to be displayed as checked */
foreach ($arr as $ele) {
$str .= "id"";
foreach ($checked as $entry) {
if ($entry == $ele- >value) {
$str .= "checked";
continue;
}
}
$str .= " >";
$str .= "$ele- >value";
if ($i % $num == 0) {
$str .= "n";
} else {
$str .= "n";
}
$i++;
}
} else {
/* we just want to print the checkboxes. none will have checks */
foreach ($arr as $ele) {
$str .= "id" >";
$str .= "$ele- >value";
if ($i % $num == 0) {
$str .= "n";
} else {
$str .= "n";
}
$i++;
}
}
/* tack on a closing tr tag if necessary */
if ($closingTR == true) {
$str .= "n";
} else {
$str .= "n";
}
return $str;
}
? >
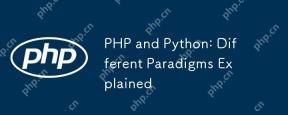
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
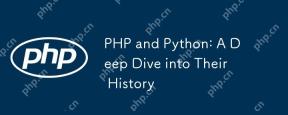
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
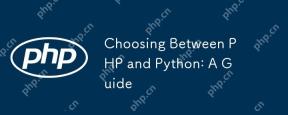
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
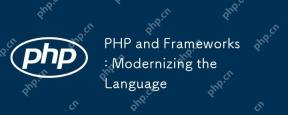
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
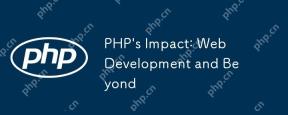
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
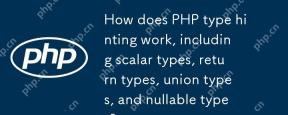
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
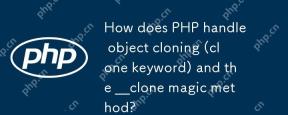
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
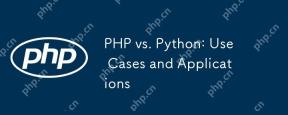
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool