


Object-Oriented Programming in PHP: Your Approach to Large PHP Projects_PHP Tutorial
This article introduces object-oriented programming (OOP) in PHP. I'll demonstrate how to use object-oriented concepts to write better programs with less code. Good luck to everyone.
The concept of object-oriented programming has different views for each author. Let me remind you what an object-oriented language should have:
- Data abstraction and information hiding
- Inheritance
- Polymorphism
How to use classes for encapsulation in PHP:
class Something {
// In OOP classes are usually named starting with a cap letter.
var $x;
function setX($v) {
// Methods start in lowercase then use lowercase to seprate
// words in the method name example getValueOfArea()
$this->x=$v;
} }
function getX() {
return $this->x;
?>
Of course you can use your own method, but it is always good to have a standard.
Data members of classes in PHP are defined using "var". Data members have no type until they are assigned a value. A data member may be an integer, an array, an associative array (associative array) or even an object. Methods are defined as functions in the class. To access data members in the method, you must use $this->name like this Method, otherwise it is a local variable of a function for the method.
Use new to create an object
$obj = new Something;
Then use the member function
$obj->setX(5);
$see = $obj->getX();
The setX member function assigns 5 to the member variable in the object (not the class) obj, and then getX returns the value 5.
You can also use object references to access member variables, for example: $obj->x=6; However, this is not a good object-oriented programming method. I insist that you use member functions to set the value of member variables and member functions to read member variables. If you believe that member variables are not accessible except by using member functions, you will become a good object-oriented programmer. But unfortunately PHP itself has no way to declare a variable as private, so bad code is allowed to exist.
Inheritance in PHP is declared using extend .
class Another extends Something {
var $y;
function setY($v) {
// Methods start in lowercase then use lowercase to seperate
// words in the method name example getValueOfArea()
$this->y=$v;
}
function getY() {
return $this ->y;
}
}
?>
In this way, the object of class "Another" has all the member variables and method functions of the parent class, plus its own
member variables and member functions. For example:
$obj2=new Another;
$obj2->setX(6);
$obj2->setY(7);
Multiple inheritance is not allowed Supported, so you can't have a class inherit from multiple classes.
In inherited classes you can redefine to redefine methods. If we redefine getX in "Another", then we can no longer access the member function getX in "Something". Similarly, if we redefine getX in "Something" If a member variable with the same name as the parent class is declared in the inherited class, then the variable of the inherited class will hide the variable with the same name of the parent class.
You can define a constructor of a class. The constructor is a member function with the same name as the class and is called when you create an object of the class.
class Something {
var $x;
function Something($y) {
$this->x=$ y;
}
function setX($v) {
$this->x=$v; return $this->x;
} }
}
?>
So you can create an object using the following method: ;
The constructor automatically assigns the value 5 to the member variable x. The constructor and member functions are ordinary PHP functions, so you can use default parameters.
function Something($x="3",$y="5")
Then:
$obj=new Something(); // x=3 and y=5
$obj=new Something(8); // x=8 and y=5
$obj=new Something(8,9); // x=8 and y=9
Default parameters are defined in the same way as C++, so you cannot pass a value to Y but let X take the default value. The actual parameters are passed from left to right. When there are no more actual parameters, the function will Use default parameters.
Only when the constructor of the inherited class is called, the object of the inherited class is created, and the constructor of the parent class is not called. This is a feature of PHP that is different from other object-oriented languages, because the constructor call chain It is a characteristic of object-oriented programming. If you want to call the base class's constructor, you have to call it explicitly in the inheriting class's constructor. This works because all methods of the parent class are available in the inherited class.
function Another() {
$this->y=5;
$this->Something(); //explicit call to base class constructor .
}
?>
A good mechanism in object-oriented programming is to use abstract classes. Abstract classes are ones that cannot be instantiated but are used to define interfaces for inherited classes. kind. Designers often use abstract classes to force programmers to only inherit from a specific base class, so they can be sure that the new class has the required functionality, but there is no standard way to do this in PHP, however:
If you need this feature when defining a base class, you can ensure that it cannot be instantiated by calling "die" in the constructor. Now define the functions of the abstract class and call "die" in each function. If In an inherited class, if the programmer directly calls the function of the base class without redefining it, an error will occur. Additionally, you need to make sure that since PHP has no types, some objects are created from inherited classes that inherit from the base class, so add a method in the base class to identify the class (return "some identifier") and verify this when you receive Comes in handy to an object as a parameter. But there is no solution for a rogue program, because he can redefine this function in the inherited class, usually this method only works for lazy programmers. Of course, the best way is to prevent the program from touching the base class code and only provide the interface.
Overloading is not supported in PHP. In object-oriented programming, you can overload a member function with the same name by defining different parameter types and numbers. PHP is a loosely typed language, so parameter type overloading is useless. Similarly, overloading with different number of parameters will not work.
Sometimes it is useful in object-oriented programming to overload constructors so you can create different objects in different ways (by passing a different number of parameters).A little trick can do this:
class Myclass {
function Myclass() {
$name="Myclass".func_num_args();
$this->$name();
//Note that $this->$name() is usually wrong but here
//$name is a string with the name of the method to call.
}
Function MyClass1 ($ x) {
Code;
}
Function MyClass2 ($ x, $ y) {
code;
}
}
?>
The purpose of overloading can be partially achieved through this method.
$obj1=new Myclass(1); //Will call Myclass1
$obj2=new Myclass(1,2); //Will call Myclass2
It feels pretty good!
Polymorphism
Polymorphism is defined as the ability of an object to decide which method to call when an object is passed as a parameter at run time. For example, use a class to define the method "draw", and inherit the class to redefine the behavior of "draw" to draw a circle or a square, so that you have a function with a parameter of x , in which you can call $x->draw( ). If polymorphism is supported, then the call to the "draw" method depends on the type of object x . Polymorphism is naturally supported in PHP (think about this situation if compiled in a C++ compiler, which method is called? However, you don't know what the type of the object is, of course this is not the case now). Fortunately, PHP supports polymorphism.
function niceDrawing($x) {
//Supose this is a method of the class Board.
$x->draw();
}
$obj=new Circle(3,187);
$obj2=new Rectangle(4,5);
$board->niceDrawing($obj); //will call the draw method of Circle.
$board->niceDrawing($obj2); //will call the draw method of Rectangle.
?>
Object-oriented programming in PHP
Pure object theorists believe that PHP is not a true object-oriented language, and this is right. PHP is a hybrid language, you can use it with object-oriented or traditional structural programming methods. For large projects, however, you may or need to use a pure object-oriented approach to defining classes and use only objects and classes in your project. Larger and larger projects will benefit from using an object-oriented approach. Object-oriented projects are easier to maintain, easier to understand, and easier to reuse. This is the basis of software engineering. Using these concepts in website design is the key to future success.
Advanced Object-Oriented Technologies in PHP
After reviewing the basic concepts of object-oriented, I will introduce some more advanced technologies.
Serialization
PHP does not support persistent objects. In object-oriented languages, persistent objects are objects that retain their state and functionality after being called multiple times by the application. This means There is a way to save objects to a file or database and then reload the object. This mechanism is called serialization. PHP has a serialization function that can be called on an object. The serialization function returns a string representing the object. Then the serialization function saves the member data instead of the member function.
In PHP4, if you serialize an object to the string $s, then delete the object, and then deserialize the object to $obj, you can still call the object's method function. But I don't recommend this approach because (a) this feature may not be supported in the future and (b) it leads to a phantom if you save the serialized object to disk and exit the program. You cannot deserialize this object and expect the object's methods to still be valid when rerunning this script in the future, because the serialized string does not represent any member functions. Finally, serializing member variables of saved objects is very useful in PHP, and that's all. (You can serialize associative arrays and arrays to disk).
Example:
$obj=new Classfoo();
$str=serialize($obj);
// Save $str to disk
//...some months later
//Load str from disk
$obj2=unserialize($str)
?>
In the above example, you can restore member variables without member functions (according to the documentation). This results in $obj2->x being
the only way to access member variables (since there are no member functions).
There are some other ways to solve this problem, but I leave it to you because it will mess up this clean document.
I hope PHP will fully support serialization in the future.
Use classes to manipulate saved data
One of the nice things about PHP and object-oriented programming is that you can easily define classes to manipulate something and call the appropriate class when needed . Suppose there is an HTML file and you need to select a product by selecting the product's ID number. Your data is saved in the database and you want to display the product's information, such as price, etc. You have different kinds of products, and the same action has different meanings for different products. For example, displaying a sound means playing it, while for other products it might be displaying an image stored in a database. You can do it using object-oriented programming and PHP, with less code but better.
Define a class, define the methods that the class should have, and then define each product class (SoundItem class, ViewableItem class, etc.) through inheritance, and redefine the methods of each product class so that they As you need. Define a class for each product type based on the product type field of the table you store in the database. A typical product table would have fields (id, type, price, description, etc.). In the script you get the type information from the database table, and then instantiate the object of the corresponding class:
$obj=new $type();
$obj-> ;action();
?>
This is a feature of PHP comparison. You can call the display method or other methods of $obj without caring about the type of the object. With this technique, you don't need to modify the script when you add a new type of object. This method is a bit powerful, it is to define the methods that all objects should have regardless of their type, and then implement them in different ways in different classes, so that they can be used for different types of objects in scripts, without if, No two programmers are ever happy in the same file. Do you believe that programming is such a joy? Low maintenance and reusable?
If you lead a group of programmers, the best way is to divide tasks so that each person can be responsible for certain classes and objects. Internationalization can be solved using the same techniques, making the appropriate classes correspond to the different languages chosen by the user, etc.
Copy and Clone
When you create an object $obj, you can use $obj2 = $obj to copy an object. The new object is a copy (not a reference) of $obj. So after the assignment, the new object has $obj with the same new state. Sometimes you don't want this and just want to create a new object identical to obj and call the new object's constructor as if you had used the new command. This can be achieved through PHP's serialization and using a base class from which other classes must inherit.
Entering the Dangerous Zone
When you serialize an object, you get a string with a specific format. If you are curious, you may be able to explore the secrets. , one thing in the string is the name of the class, you can unpack it:
$herring = serialize($obj);
$vec = explode(": ",$herring);
$nam = str_replace(""", "", $vec[2]);
?>
Suppose you create a class "Universe" and use All classes inherit from "Universe". You can define a clone method in "Universe":
class Universe {
function __clone() {
$herring=serialize($this);
$vec=explode(":",$herring);
$nam=str_replace(""", "",$vec[2]);
$ret= new $nam;
return $ret;
}
}
//Then:
$obj=new Something();
/ /Something extends Universe !!
$other=$obj->__clone();
?>
What you get is a new object of class Something as if using new , and the constructor is called and so on.I don't know if this will work for you, but it's a good practice that the Universe class knows the name of the class it inherits from. The only limit for you is your imagination! ! !
Note: I am using PHP4, and some things in the article may not be suitable for PHP3.
-End-
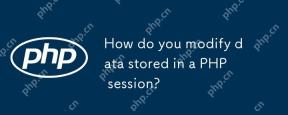
TomodifydatainaPHPsession,startthesessionwithsession_start(),thenuse$_SESSIONtoset,modify,orremovevariables.1)Startthesession.2)Setormodifysessionvariablesusing$_SESSION.3)Removevariableswithunset().4)Clearallvariableswithsession_unset().5)Destroythe
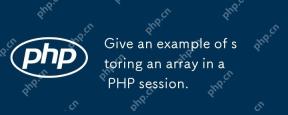
Arrays can be stored in PHP sessions. 1. Start the session and use session_start(). 2. Create an array and store it in $_SESSION. 3. Retrieve the array through $_SESSION. 4. Optimize session data to improve performance.
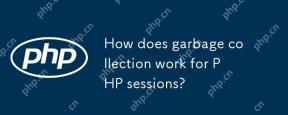
PHP session garbage collection is triggered through a probability mechanism to clean up expired session data. 1) Set the trigger probability and session life cycle in the configuration file; 2) You can use cron tasks to optimize high-load applications; 3) You need to balance the garbage collection frequency and performance to avoid data loss.
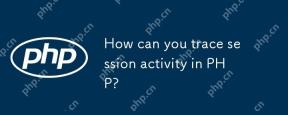
Tracking user session activities in PHP is implemented through session management. 1) Use session_start() to start the session. 2) Store and access data through the $_SESSION array. 3) Call session_destroy() to end the session. Session tracking is used for user behavior analysis, security monitoring, and performance optimization.
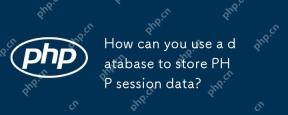
Using databases to store PHP session data can improve performance and scalability. 1) Configure MySQL to store session data: Set up the session processor in php.ini or PHP code. 2) Implement custom session processor: define open, close, read, write and other functions to interact with the database. 3) Optimization and best practices: Use indexing, caching, data compression and distributed storage to improve performance.
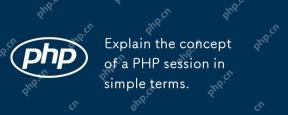
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
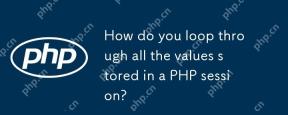
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
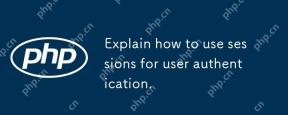
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
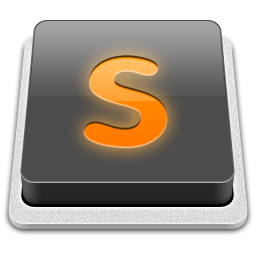
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
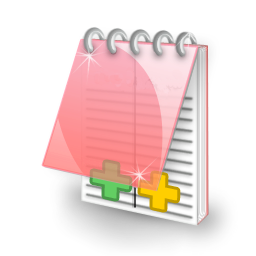
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
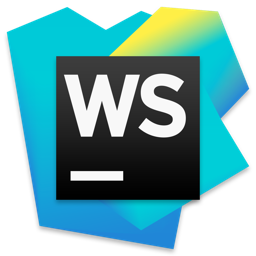
WebStorm Mac version
Useful JavaScript development tools
