


1. Database operation
1. Connect MYSQL data
mysql_connect()
e.g.
$db = mysql_connect(MYSQL_HOST, MYSQL_USER, MYSQL_PASSWORD) or die('Unable to connect, please check connection paremeters');
2. Select the database
mysql_select_db()
After connecting to the database, the database selected by PHP by default may not be the database we need in subsequent operations. To ensure that the database is selected correctly, a database selection statement is usually added after the database connection statement. .
e.g.
mysql_select_db(MYSQL_DB, $db) or die(mysql_error($db ));
3. Execute SQL statement
mysql_query()
This function sends the SQL statement to the currently active database and executes the statement, returning the result.
e.g.
$query = “SELECT * FROM $table”
$ result = mysql_query($query, $db) or die(mysql_error($db));
4. Close the database
mysql_close()
This function is used to close the database and does not need to continue Active database, but this method is not required. Generally, PHP will automatically close databases that are no longer active.
e.g.
mysql_close($db);
5. Release SQL results
mysql_free_result()
This function is used to release the memory occupied by the execution result of mysql_query(). This function is rarely called , unless the result is very large and takes up too much memory; usually the occupied memory is automatically released after the PHP script is executed.
2. SQL execution result operations
1. Return a row in the execution result
mysql_fetch_row()
Return the numerical array of the current row of the execution result. After executing this function, The result points to the next line.
e.g.
$row = mysql_fetch_row($result);
Processing execution results is generally placed in a while loop, traversing each row
e.g.
while($row = mysql_fetch_row($result))
{……}
2. Alternatives to mysql_fetch_row()
mysql_fetch_array()
mysql_fetch_assoc()
mysql_fetch_array() returns an array of key-value pairs, where the key is the column name of the queried table ;
Mysql_fetch_assoc() can sort the results first (if assigned to optional parameters), which is equivalent to mysql_fetch_array()+MYSQL_ASSOC
3. Field (column) attributes of the execution result
mysql_fetch_field()
4. Query the table name in the database
mysql_list_tables()
e.g.
$db_name = MYSQL_DB;
$result = mysql_list_tables($db_name);
echo "The database contains the following tables:";
while ($row = mysql_fetch_row($result))
{
echo $row[0];
}
5. Query the column name (field name) of the database
mysql_list_fields()
e.g.
$fields = mysql_list_fields($db_name,$table);
$columns = mysql_num_fields($fields);
for ($i = 0; $i echo mysql_field_name($fields, $i);
3. Other functions
1. mysql_num_rows()
Returns the number of rows in the execution result.
e.g.
$num = mysql_num_rows($result);
2. mysql_num_fields()
Returns the number of columns (number of fields) of the execution result.
e.g. $num = mysql_num_fields($result);
3.mysql_set_charset()
Set the encoding of the execution result to prevent garbled characters when displaying Chinese on the web page.
e.g.
$query = “select * from $table_name”;
mysql_query('set names utf8′);
$result = mysql_query($query, $db) or die(mysql_error($db));
Note:
1. The uppercase codes in the article are predefined content, such as define(MYSQL_HOST, 'localhost');
2. This article only summarizes the main functions of PHP operating database, please refer to the complete content Related content of the PHP manual.
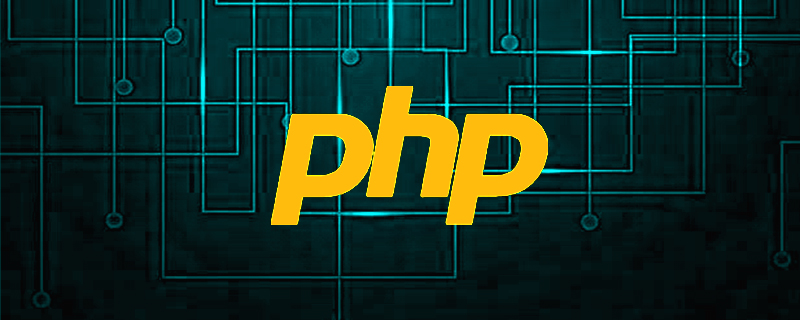
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
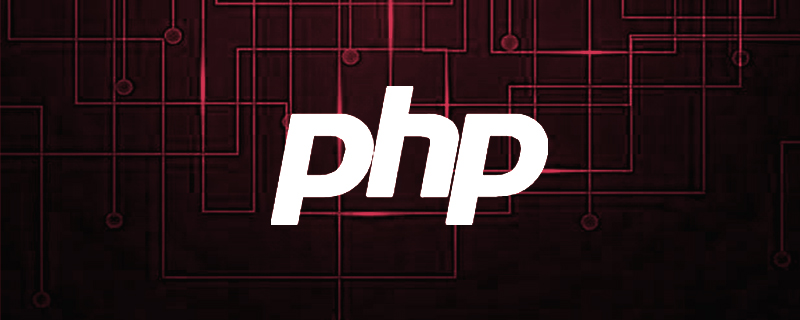
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
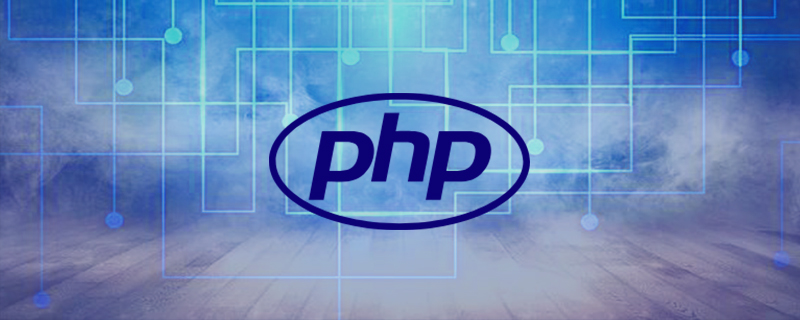
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
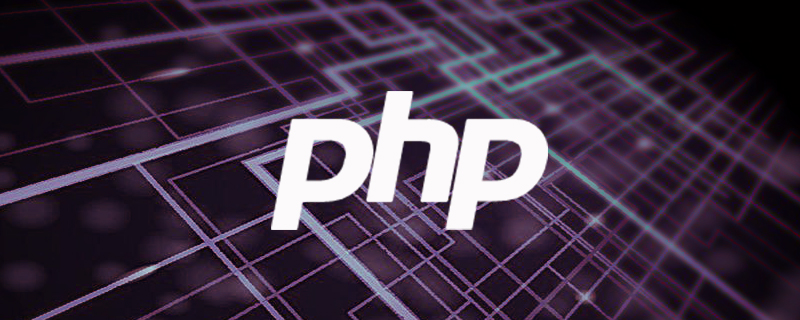
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
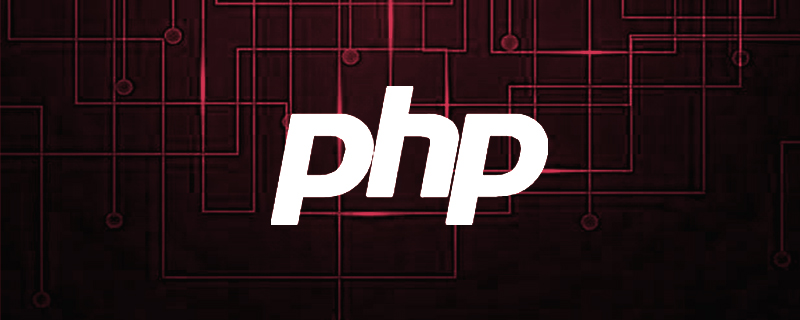
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
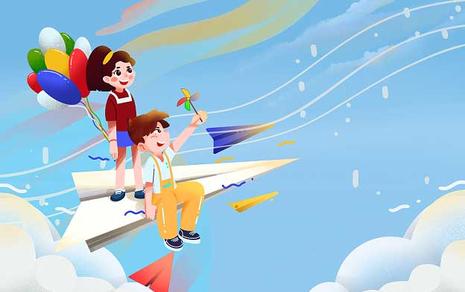
推荐适合地理信息科学专业学生用的电脑1.推荐2.地理信息科学专业学生需要处理大量的地理数据和进行复杂的地理信息分析,因此需要一台性能较强的电脑。一台配置高的电脑可以提供更快的处理速度和更大的存储空间,能够更好地满足专业需求。3.推荐选择一台配备高性能处理器和大容量内存的电脑,这样可以提高数据处理和分析的效率。此外,选择一台具备较大存储空间和高分辨率显示屏的电脑也能更好地展示地理数据和结果。另外,考虑到地理信息科学专业学生可能需要进行地理信息系统(GIS)软件的开发和编程,选择一台支持较好的图形处
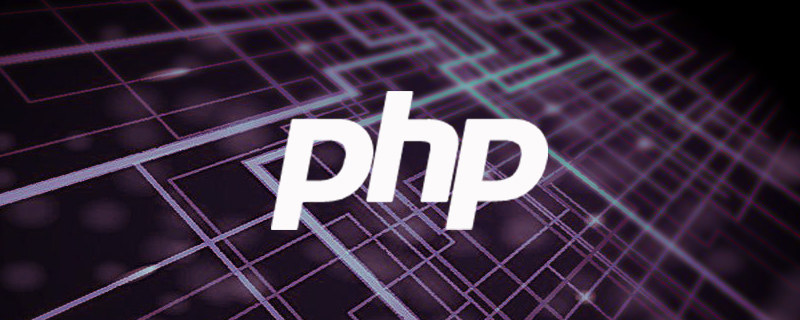
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
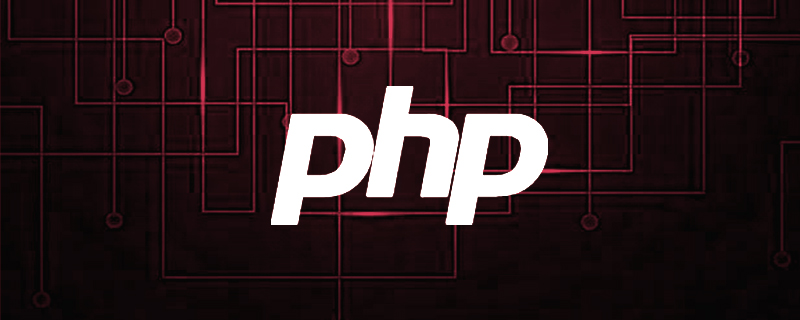
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
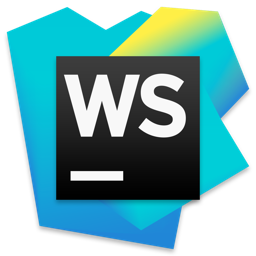
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
