Function bool array_multisort (array &$arr [, mixed $arg = SORT_ASC [, mixed $arg = SORT_REGULAR [, mixed $...]]] )
Parameter description: The function sorts multiple arrays or multidimensional arrays
The first parameter is an array, each subsequent parameter may be an array, or the following sort order flag
SORT_ASC - Default, sort in ascending order
SORT_DESC - Sort in descending order
Can be specified later Sorting type
SORT_REGULAR - Default. Arrange each item in regular order.
SORT_NUMERIC - Sort each item in numerical order.
SORT_STRING - Sort each item in alphabetical order.
Example code
$arr1 = array('10', 11, 100, 100, 'a');
$arr2 = array(1, 2, 3, '2', 5);
array_multisort($arr1, $arr2);
result is:
$arr1
Array ( [0] => 10 [1] => a [2] => 11 [3] => 100 [4] => 100 )
# '10' is converted to the integer 10 when compared with 11, 100, 100, which is smaller than the other three numbers
# '10' is used as a string when compared with 'a', and its first character is '1' ascii The code value is 49 which is less than 'a' (the ascii value is 97), so '10' is the smallest element
# 'a' is converted to an integer 0 when comparing the other three numbers, which is less than the other three numbers
$arr2
Array ( [0] => 1 [1] => 5 [2] => 2 [3] => 2 [4] => 3 )
# $arr2 element 1 corresponds to the position of $arr1 element '10', so it is ranked at [0] position
# $arr1[2] => 100, $arr1[3] => 100 corresponds to $arr2 element 3, '2 respectively '. 3 is greater than '2', so the sorted subscript of $arr1[2] => 100 corresponding to 2 is
3, and the sorted subscript of $arr1[3] => 100 corresponding to 3 is 4
Summary
1. The number of array elements participating in the sorting remains the same
2. The positions of the sorted array elements correspond to, for example, '10' => 1 , 11 => 2
3. The following arrays Sort based on the order of the previous array
4. If the previous array encounters equal elements, compare the following array
array_multisort — Sort multiple arrays or multi-dimensional arrays
Description
bool array_multisort ( array $ar1 [, mixed $arg [, mixed $... [, array $... ]]] )
Returns TRUE on success, or if Returns FALSE on failure.
array_multisort() can be used to sort multiple arrays at once, or to sort multi-dimensional arrays according to one or more dimensions.
Associative (string) key names remain unchanged, but numeric key names will be re-indexed.
The input array is treated as a table column and sorted by row - this is similar to the functionality of SQL's ORDER BY clause. The first array is the main array to be sorted. If the rows (values) in the array are compared to be the same, they are sorted according to the size of the corresponding value in the next input array, and so on.
The parameter structure of this function is somewhat unusual, but very flexible. The first parameter must be an array. Each of the following arguments can be an array or a sort flag listed below.
Sort order flags:
SORT_ASC - Sort in ascending order
SORT_DESC - Sort in descending order
Sort type flags:
SORT_REGULAR - Sort in descending order Items are compared according to the usual method
SORT_NUMERIC - items are compared according to numeric values
SORT_STRING - items are compared according to strings
Two similar sorting flags cannot be specified after each array. The sort flags specified after each array are valid only for that array - before that, the default values SORT_ASC and SORT_REGULAR.
#1 Sort multiple arrays
$ar1 = array("10", 100, 100, "a");
$ar2 = array(1, 3, "2", 1);
array_multisort($ar1, $ar2) ;
var_dump($ar1);
var_dump($ar2);
?>
After sorting in this example, the first array will contain "10" ,"a",100,100. The second array will contain 1,1,"2",3. The order of the items in the second array is exactly the same as the order of the corresponding items (100 and 100) in the first array.
array(4) {
[0]=> string(2) "10"
[1]=> string(1) "a"
[2]=> int(100)
[3]=> int(100)
}
array(4) {
[0]=> int(1)
[1]=> int(1)
[2]=> string(1) "2"
[3]=> int(3)
}
#2 Sort multi-dimensional array
$ar = array (array ("10", 100, 100, "a"), array (1, 3, "2", 1));
array_multisort ($ar[0], SORT_ASC, SORT_STRING,
$ar[1], SORT_NUMERIC, SORT_DESC);
?>
After sorting in this example, the first array will contain 10, 100, 100, "a" (as ascending order of strings), and the second array will contain 1, 3, "2", 1 (as numerically descending order).
#3 Sorting multi-dimensional array
$ar = array(
array("10", 11, 100, 100, "a"),
array( 1, 2, "2", 3, 1)
);
array_multisort($ar[0], SORT_ASC, SORT_STRING,
$ar[1], SORT_NUMERIC, SORT_DESC);
var_dump($ar);
?>
After sorting in this example, the first array will become "10", 100, 100, 11, "a" (treated as strings in ascending order). The second array will contain 1, 3, "2", 2, 1 (treated as numbers in descending order).
array(2) {
[0]=> array(5) {
[0]=> string(2) "10"
[1]=> int(100)
[2]=> int(100)
[3]= > int(11)
[4]=> string(1) "a"
}
[1]=> array(5) {
[0]=> int (1)
[1]=> int(3)
[2]=> string(1) "2"
[3]=> int(2)
[4 ]=> int(1)
}
}
#4 Sort the database results
In this example, each cell in the data array represents a table One line. This is a typical collection of data recorded in a database.
The data in the example is as follows:
volume | edition
-------+--------
67 | 2
86 | 1
85 | 6
98 | 2
86 | 6
67 | 7
The data are all stored in the array named data. This is usually obtained from the database through a loop, such as mysql_fetch_assoc().
$data[] = array('volume' => 67, 'edition' => 2);
$data[] = array('volume' => 86, 'edition' => 1);
$data[] = array('volume' => 85, 'edition' => 6);
$data[] = array ('volume' => 98, 'edition' => 2);
$data[] = array('volume' => 86, 'edition' => 6);
$data [] = array('volume' => 67, 'edition' => 7);
?>
In this example, the volume will be sorted in descending order and the edition will be sorted in ascending order.
Now you have an array with rows, but array_multisort() requires an array with columns, so use the following code to get the columns and then sort them.
// Get the list of columns
foreach ($data as $key => $row) {
$volume[$key] = $row[' volume'];
$edition[$key] = $row['edition'];
}
// Sort the data in descending order according to volume and in ascending order according to edition
// Use $data as the last parameter, sort by common key
array_multisort($volume, SORT_DESC, $edition, SORT_ASC, $data);
?>
The data collection is now sorted, the results are as follows :
volume | edition
-------+--------
98 | 2
86 | 1
86 | 6
85 | 6
67 | 2
67 | 7
Example #5 Case-insensitive sorting
SORT_STRING and SORT_REGULAR are both case-sensitive. Uppercase letters will appear before lowercase letters.
To perform case-insensitive sorting, sort by lowercase letters of the original array.
$array = array('Alpha', 'atomic ', 'Beta', 'bank');
$array_lowercase = array_map('strtolower', $array);
array_multisort($array_lowercase, SORT_ASC, SORT_STRING, $array);
print_r($array);
?>
The above routine will output:
Array
(
[0] => Alpha
[1] => atomic
[2] => bank
[3] => Beta
)
【Translator's Note】This function is quite useful. To help you understand, please look at the following example:
Example #6 Ranking
$grade = array("score" => array(70, 95, 70.0, 60, "70"),
"name" => ; array("Zhang San", "Li Si", "Wang Wu",
"Zhao Liu", "Liu Qi"));
array_multisort($grade["score"], SORT_NUMERIC, SORT_DESC,
// Use scores as numerical values, sort from high to low
$grade["name"], SORT_STRING, SORT_ASC);
// Use names as strings, sort from small to large
var_dump($grade);
?>
The above routine will output:
array(2) {
["score"]=>
array(5) {
[0]=>
int(95)
[1]=>
string(2) "70"
[2]=>
float(70)
[3]=>
int(70)
[4]=>
int(60)
}
["name"]=>
array(5) {
[0 ]=>
string(5) "Li Si"
[1]=>
string(6) "Liu Qi"
[2]=>
string(7 ) "Wang Wu"
[3]=>
string(9) "Zhang San"
[4]=>
string(8) "Zhao Liu"
}
}
In this example, the array $grade containing grades is sorted from high to low by score, and people with the same score are sorted by name from small to large. After sorting, Li Si ranked first with 95 points, and Zhao Liu ranked fifth with 60 points. There is no objection. Zhang San, Wang Wu and Liu Qi all scored 70 points, and their rankings were arranged alphabetically by their names, with Liu first, Wang second and Zhang last. For the sake of distinction, the three 70 points are represented by integers, floating point numbers and strings respectively, and their sorted results can be clearly seen in the program output.
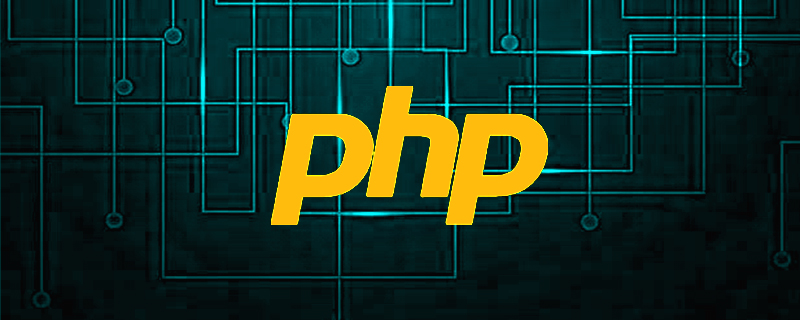
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
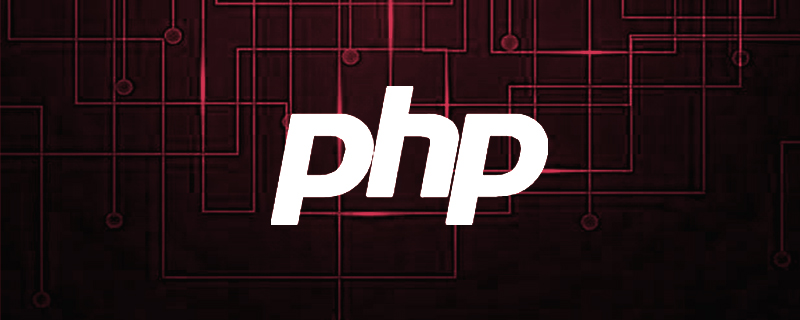
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
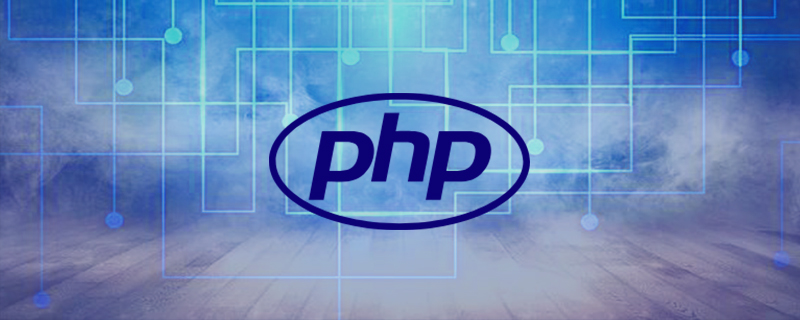
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
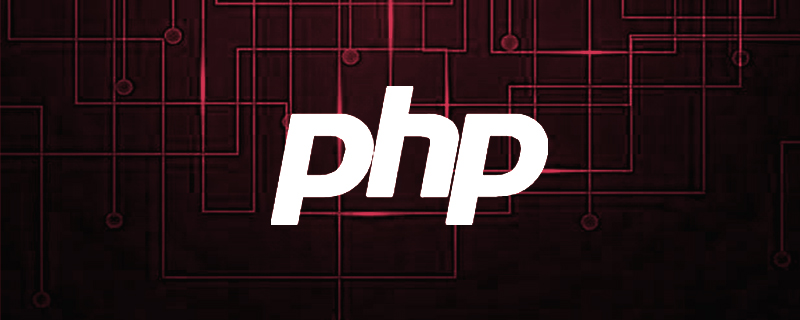
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
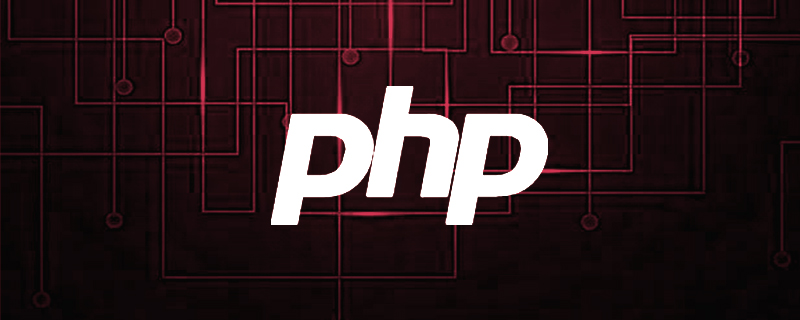
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
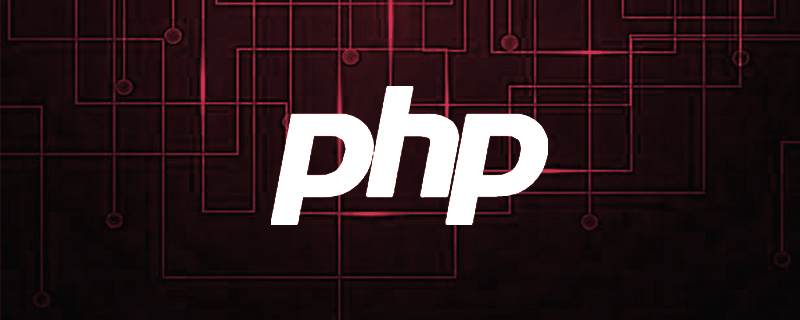
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。
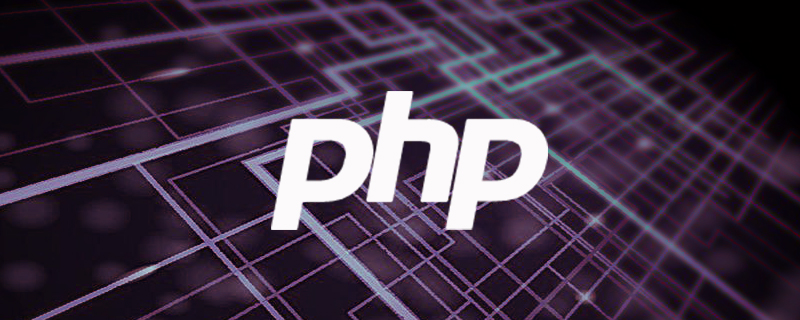
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
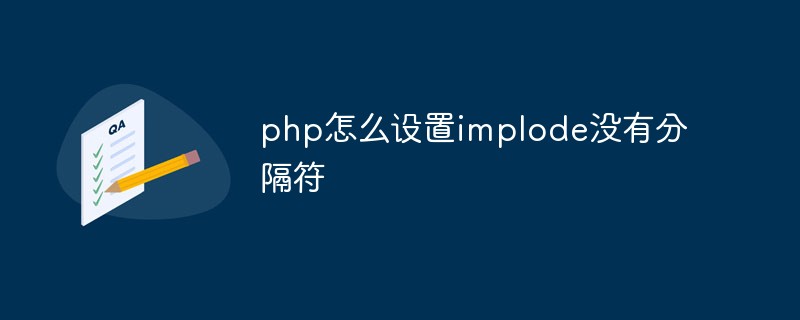
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
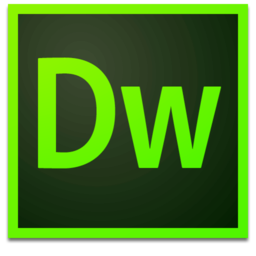
Dreamweaver Mac version
Visual web development tools
