


1. How to define variables? How to check if a variable is defined? How to delete a variable? How to check if a variable is set?
$Definition isset()// Check whether the variable is set
Defined()// Check whether the constant is set
unset()//Destroy the specified variable
empty()// Check Is the variable empty?
2. What is a variable variable?
The variable name of a variable can be set and used dynamically.
$a = 'hello', $$a = 'world', ${$a}=hello world
3. What are the methods of variable assignment?
1) Direct assignment 2) Assignment between variables 3) Reference assignment
4. What is the difference between reference and copy?
Copying is to copy the original variable content. The copied variable and the original variable use their own memory and do not interfere with each other.
Reference is equivalent to an alias of a variable. In fact, it means using different names to access the same variable content. When you change the value of one variable, the other one also changes.
5. What are the basic data types of variables in php?
PHP supports 8 primitive data types including:
Four scalar types (boolean, integer, float/double, string)
Two composite types ( Array, object)
Two special types (resource, NULL)
6. When other types are converted to boolean types, which ones are considered false?
Boolean value false, integer value 0, floating point value 0.0, blank string, string '0', empty array, special data type NULL, no variable set.
Under what circumstances does the empty() function return true?
Boolean value false, integer value 0, floating point value 0.0, blank string, string '0', array() empty array, special data type NULL, object without any attributes, variable with no assigned value.
7. If a variable $a is defined but no initial value is assigned
then $a==0? $a==false? $a==''?
Is $a==NULL? Is $a===NULL? Answer: echo=>Nothing, var_dump=>NULL
Is empty($b)==true? ———————————— echo=>1 , var_dump=>bool(true)
What is the output $a++ at this time? ———————— echo=>Nothing, var_dump=>NULL
What is the output of ++$a? —————————— echo=>1 , var_dump=>int(1)
8. How to convert a string into an integer? How many methods are there? How to achieve it?
Forced type conversion: (integer) string variable name;
Direct conversion: settype(string variable, integer);
intval(string variable);
9. What is the biggest difference between scalar data and arrays?
A scalar can only store one data, while an array can store multiple data.
10. How are constants defined? How to check whether a constant is defined? What data types can the value of a constant be?
define()//Define constant, defined()//Check whether the constant is defined
The value of a constant can only be scalar type data.
11. Constants are divided into system built-in constants and custom constants. Please name the most common system built-in constants?
__FILE__ , __LINE__ , PHP_OS , PHP_VERSION
12. If two identical constants are defined, which of the former and the latter will work?
The former works because once a constant is defined it cannot be redefined or undefined.
13. What are the differences between constants and variables?
1) There is no $ sign before constants;
2) Constants can only be defined through define(), not assignment statements;
3) Constants can be defined and accessed anywhere, and Variables are divided into global and local;
4) Once a constant is defined, it cannot be redefined or undefined, while a variable is redefined through assignment;
5) The value of a constant can only be scalar data, while a variable The database type has 8 primitive data types.
14. What are the several predefined global array variables commonly used in PHP?
There are 9 predefined built-in array variables:
$_POST, $_GET, $_REQUEST, $_SESSION, $_COOKIE, $_FILES, $_SERVER, $_ENV, $GLOBALS
15. In actual development, where are constants most commonly used?
1) Define the information to connect to the database as constants, such as the user name, password, database name, host name of the database server;
2) Define part of the path of the site as constants, such as the web absolute path, The installation path of smarty, the folder path of model, view or controller;
3) Public information of the website, such as website name, website keywords and other information.
16. What are the advantages of functions?
Improve the maintainability of the program Improve the reliability of the software Improve the reusability of the program Improve the development efficiency of the program
17. How to define a function? Are function names case sensitive?
1) Use the function keyword;
2) Function naming rules are the same as variables, starting with a letter or underscore, not a number;
3) Function names are not case-sensitive;
4) The function name cannot use the function name that has been declared or built by the system.
18. What is the visibility of variables or variable scope?
It is the scope of the variable in the program. According to the visibility of variables, variables are divided into local variables and global variables.
19. What are local variables and global variables? Can global variables be called directly within a function?
Local variables are variables defined inside a function, and their scope is the function in which they are located. If there is a variable with the same name as a local variable outside the function, the program will think that they are two completely different variables. When exiting the function, the local variables are cleared at the same time. Global variables are variables defined outside all functions. Their scope is the entire php file, but they cannot be used inside user-defined functions. If you must use global variables inside a user-defined function, you need to use the global keyword declaration. That is to say, if you add golbal in front of the variable in the function, then the global variable can be accessed inside the function. Not only can you use this global variable to perform operations, but you can also reassign the global variable. Global variables can also be called using $GLOBALS['var'].
21. What are static variables?
If a variable defined in a function is declared with the keyword static before it, then the variable is a static variable.
Generally, after the function call ends, the data stored in the variables within the function will be cleared and the memory space occupied will be released. When using a static variable, the variable will be initialized when the function is called for the first time, and the variable will not be cleared after initialization. When the function is called again, the static variable will no longer be initialized, but can save the last The value after the function is executed. We can say that static variables are shared between all calls to the function.
22. What are the ways to pass parameters to functions in php? What's the difference between the two?
Pass by value and pass by address (or pass by reference)
(1) Pass by value: The variable to be passed is stored in a different space from the variable passed to the function. Therefore, modifications to the variable value
in the function body will not affect the original variable value.
(2) Pass by address: Use the & symbol to indicate that the parameter is passed by address. It does not pass the specified value or target variable in the main program to the function, but imports the memory storage block address of the value or variable into the function, so the variable in the function body and the variable in the main program are in the memory. are the same.Modifications made to the function body directly affect the value of the variable outside the function body.
23. What is a recursive function? How to make a recursive call?
A recursive function is actually a function that calls itself, but it must meet the following two conditions:
1) Each time it calls itself, it must be closer to the final result;
2) There must be A certain recursion termination condition will not cause an infinite loop.
For example:
In actual work, it is often used when traversing folders.
If there is an example where you want to get all the files under the directory windows, then traverse the windows directory first. If you find that there are folders in it, then you will call yourself and continue to search, and so on,
until Traverse until there are no more folders, which means that all files have been traversed.
24. Determine whether a function exists?
function_exists( string $function_name ) Returns true if it exists, false if it does not exist.
25. What is the difference between func() and @func()?
The second function call will not report an error if it fails, but the first one will report an error
26. What is the usage and difference between include() and require() functions? What about include_once() and require_once()?
The error levels of include and require are different
Include_once() and require_once() need to determine whether they have been imported before loading
27. Say the prefix What is the difference between ++ and post++?
Prefix ++ first increments the variable by 1, and then assigns the value to the original variable;
Postfix ++ first returns the current value of the variable, and then increments the current value of the variable. 1.
28. What is the difference between the string operator "." and the arithmetic operator "+"?
When "." is used between "a" and "b", it is considered a hyphen. If there is a + between the two, PHP will consider it to be an operation.
1) If the string on both sides of the + sign is composed of numbers, the string will be automatically converted into an integer;
2) If the string on both sides of the + sign is pure letters, then 0 will be output;
3) If the string on both sides of the + sign starts with a number, then the number at the beginning of the string will be intercepted and then operated.
29. What is the ternary (or ternary) operator?
Choose one of the two expressions based on the result of one expression.
For example: ($a==true) ? 'good':'bad';
30. What are the control flow statements?
1: Three program structures sequential structure, branch structure, loop structure
2: Branch: if/esle/esleif/ switch/case/default
3: switch Need to pay attention to:
The constants in the case clause can be integers, string constants, or constant expressions, and are not allowed to be variables.
In the same switch clause, the case values cannot be the same, otherwise only the value in the first occurrence of the case can be obtained.
4: Loop for while do...while
do...while must be followed by a semicolon.
The difference between while and do...while
5: The difference between break and continue.
break can terminate the loop.
Continue is not as powerful as break. It can only terminate this cycle and enter the next cycle.
31. What is the concept of array? What are the two types of arrays based on indexes, and how to distinguish them? What are the two ways of assigning values to an array?
An array is a variable (composite variable) that can store a set or series of values
Index array (index value is a number, starting with 0) and associative array (with string as index value)
What are the two ways of assigning values to arrays?
There are two main ways to declare arrays.
1. Declare the array through the array() function;
You can define the index and value respectively through key=>value, or you can not define the index subscript of the array, and only give the element value of the array.
2. Directly assign values to array elements without calling the array() function. For example:
$arr[0] = 1; $arr[1] = 2;
Special note:
If the subscript of the array is a string value equivalent to an integer (but it cannot start with 0 ), will be treated as an integer.
For example: $array[3] and $array['3'] refer to the same element, while $array['03'] refers to another element.
32. How to traverse an array?
①for loop
②foreach loop, this is the most commonly used traversal method. The usage is as follows: foreach($arr as $key=>$value){}
③list each and while are combined to loop
33. How does the pointer point when foeach array?
How does the pointer point when list()/each()/while() loops through the array?
When foreach starts executing, the pointer inside the array will automatically point to the first unit. Because foreach operates on a copy of the specified array, not the array itself. After each() an array, the array pointer will stay at the next unit in the array or at the last unit when it reaches the end of the array. If you want to use each() to traverse the array again, you must use reset().
reset() to rewind the internal pointer of the array to the first unit and return the value of the first array unit.
34. How to calculate the length of an array (or count the number of all elements in the array)? How to get the length of a string?
count() -- Count the number of elements in the array.
You can use count (array name) or count (array name, 1). If there is a second parameter and it is the number 1, it means recursively counting the number of array elements. If the second parameter is the number 0, it is equivalent to the count() function with only one parameter.
sizeof() -- Alias of count() (count - Count the number of cells in an array or the number of attributes in an object)
String: strlen()—Get the length of a string
mb_strlen() — Get string length
35. What are the common functions related to arrays?
1) count -- (sizeof alias) - Count the number of cells in the array or the number of attributes in the object
For example: int count (mixed $var [, int $mode ] ) $var usually All are array types, any other type has only one unit. The default value of $mode is 0. 1 turns on recursively counting the array
2) in_array (mixed $needle, array $haystack [, bool $strict]) — Check whether a certain value exists in the array. If needle is a string, the comparison is case-sensitive. If the value of the third parameter strict is TRUE, the in_array() function will also check whether the type of needle is the same as that in haystack.
3) array_merge(array $array1 [, array $array2 [, array $... ]] ) Merges the cells of one or more arrays, and the values in one array are appended to the previous array. Returns the resulting array.
Special note: If the input array has the same string key name, the value after the key name will overwrite the previous value. However, if the array contains numeric keys, the subsequent values will not overwrite the original values but will be appended to them.
If only one array is given and the array is numerically indexed, the key names will be re-indexed in a continuous manner
4) Conversion between arrays and strings
(1)explode ( string $separator , string $string [, int $limit ] ) Use a separator character to separate a string.
(2)implode ( string $glue , array $arr ) Use a connector to connect each unit in the array into a string. join is an alias of implode
5) sort(array &$array [, int $sort_flags]) — Sort the array by value. When this function ends, the array cells will be rearranged from lowest to highest.
36. What is the difference between the array merging function array_merge() and the array addition operation $arr + $arr2?
array_merge()-> Use array_merge(), if it is an associative array merge, if the key names of the arrays are the same, then the latter value will overwrite the former; if it is a numeric index array merge, it will not be overwritten, and It is the latter that is appended to the former. "+"->Use array addition operation. Different from array_merge(), the addition operation, whether it is an associative array or a numerical index array, discards the values with the same key name, that is, only retains the element where the key name appears for the first time. , subsequent elements with the same key name will not be added.
37. What is the difference between single quotes and double quotes when defining strings?
Single quotes load faster than double quotes
38. What are the differences between echo(), print(), and print_r()?
(1)echo is a syntax, Output one or more strings, no return value;
(2)print is a function, cannot output arrays and objects, Output a string, print has a return value;
(3)print_r is a function that can output an array. print_r is an interesting function. It can output string, int, float, array, object, etc. When outputting array, it will be represented by a structure. print_r returns true when the output is successful; and print_r can be passed print_r($str,true), so that print_r Returns the value processed by print_r without outputting it. In addition, for echo and print, echo is basically used because its efficiency is higher than print.
39. What are the string processing functions according to functional classification? What do these functions do?
A. String output function
(1)echo $a,$b,$c...; is a language structure, not a real function.
(2)print($a) This function outputs a string. If successful, it will return 1, if it fails, it will return 0
(3)print_r($a)
(4)var_dump($a); Can output type, length, value
B. Remove spaces from the beginning and end of the string Function: trim ltrim rtrim (alias: chop) Using the second parameter, you can also remove specified characters.
C. Escape string function: addslashes()
D. Get string length function: strlen()
E. Intercept string length function: substr()
F. Retrieve string function: strstr(), strpos()
G. Replace string function: str_replace()
40. Please give the correct answer to the following questions?
1).$arr = array('james', 'tom', 'symfony'); Please split the value of the $arr array with ',' and merge it into a string for output? echo implode(',',$arr);
2).$str = 'jack,james,tom,symfony'; Please split $str with ',' and put the split value into $arr in an array? $arr = explode(',',$str);
3).$arr = array(3,7,2,1,'d','abc'); Please sort $arr from large to small sort order and keep their key values unchanged? arsort($arr); print_r($arr);
4).$mail = “gaofei@163.com”; Please take out the domain of this mailbox (163.com) and print it to see the maximum number you can write How many methods?
echo strstr($mail,'163');
echo substr($mail,7);
$arr = explode("@",$mail); echo $arr[1];
5). If there is a string, the string is "123, 234, 345,".How can I cut off the last comma in this string?
6). What are the functions for obtaining random numbers? Which execution speed is faster, mt_rand() or rand()?
41. How to solve the problem of garbled characters on the page?
1. First consider whether the current file has a character set set. Check whether charset is written in the meta tag. If it is a php page, you can also check whether
specifies charset in the header() function;
For example:
header("content-type:text/html;charset=utf-8");
2. If characters are set set (that is, charset), then determine whether the encoding format saved in the current file is consistent with the character set set on the page,
. Whether the character set when querying is consistent with the character set set on the current page, the two must be unified,
For example: mysql_query("set names utf8").
42. What is a regular expression? What are the commonly used functions related to regular expressions in PHP? Please write a regular expression for email, Chinese mobile phone number and landline number?
Regular expression is a grammatical rule used to describe character arrangement patterns. Regular expressions are also called pattern expressions. Regular expressions in website development are most commonly used for client-side verification before form submission information.
For example, verify whether the user name is input correctly, whether the password input meets the requirements, whether the input of Email, mobile phone number and other information is legal. .
preg series functions can handle it. The specific ones are as follows:
string preg_quote ( string str [, string delimiter] )
Escape regular expression characters. Special characters of regular expressions include: . \ + * ? [ ^ ] $ ( ) { } = ! | :.
preg_replace -- Perform regular expression search and replacement
mixed preg_replace ( mixed pattern, mixed replacement, mixed subject [, int limit]
preg_replace_callback -- Use callback function to perform regular expression search and replace
mixed preg_replace_callback (mixed pattern, callback callback, mixed subject [, int limit] )
preg_split -- Split a string with a regular expression
array preg_split ( string pattern, string subject [, int limit [, int flags]] )
Commonly used regular expression writing:
Chinese: /^[u4E00-u9FA5]+$/
Mobile phone number: /^(86)?0?1d{10 }$/
EMAIL:
/^[w-]+[w-.]?@[w-]+.{1}[A-Za-z]{2,5}$/
Password (medium security level):
/^(d+[A-Za-z]w*|[A-Za-z]+dw*)$/
Password (high security level):
/^(d+[a-zA-Z~!@#$%^&(){}][w~!@#$%^&(){}]*|[a-zA-Z~!@ #$%^&(){}]+d[w~!@#$%^&(){}]*)$/
44. The two functions preg_replace() and str_ireplace() are in What are the differences in usage?
preg_replace — perform regular expression search and replacement
str_ireplace — case-ignoring version of str_replace() str_replace — substring replacement
. Use PHP to print out today's time in the format of 2010-12-10 22:21:21?
Use PHP to print out the time of the previous day in a format of 2010-12-10 22:21:21? How to turn 2010-12-25 10:30:25 into a unix timestamp?
echo date ("Y-m-d H:i:s" ,strtotime('-1,days')); date('Y-m-d H:i:s',time()); $unix_time = Strtotime ("2009-9-2 10:30:25"); // turn into unix timestamp
Echo date ("y-m-d h: s", $ unix_time); Format
46. When using get to pass values in the URL, if the Chinese characters appear garbled, which function should be used to encode the Chinese characters?
When users submit data in website forms, in order to prevent script attacks (such as the user inputting <script>alert (111); </script>), how should the PHP side handle the data when it receives it?
Use urlencode() to encode Chinese and urldecode() to decode. Use htmlspecialchars($_POST[‘title’]) to filter form parameters to avoid script attacks.
48. What are the differences between mysql_fetch_row() and mysql_fetch_assoc() and mysql_fetch_array?
The first one returns a row in the result set as an index array, the second one returns an associative array, and the third one can return either an index array or an associative array, depending on its second parameter MYSQL_BOTH MYSQL_NUM MYSQL_ASSOC Default is MYSQL_BOTH
$sql =”select * from table1”;
$result = mysql_query($sql);
mysql_fetch_array($result, MYSQL_NUM);
49. Please tell me the functions you have learned so far that return resources?
Answer: fopen (open file)
imagecreatefromjpeg(png gif) — Create a new image from a JPEG file
imagecreatetruecolor — Create a new true color image
Imagecopymerge — Copy and merge part of an image
using 🎜>
50. What are the functions for opening and closing files? What are the functions of file reading and writing? Which function is used to delete files?
Which function is used to determine whether a file exists? Which function is used to create a new directory?
51. What details should be paid attention to when uploading files? How to save files to a specified directory? How to avoid the problem of uploading files with duplicate names?
1. First, you need to enable file upload in php.ini; 2. There is a maximum allowed upload value in php.ini, the default is 2MB. It can be changed when necessary;
3. When uploading the form, be sure to write enctype="multipart/form-data" in the form tag; 4. The submission method must be post;
5. Set the form control with type="file";
6. Pay attention to the size of the uploaded file MAX_FILE_SIZE, whether the file type meets the requirements, and whether the path to be stored after uploading exists.
You can get the file suffix from the uploaded file name, and then rename the file using the timestamp + file suffix, thus avoiding duplicate names. You can set the saving directory of the uploaded file yourself, and combine it with the file name to form a file path. Use move_uploaded_file() to save the file to the specified directory.
52. How many dimensional array is $_FILES? What are the index subscripts for the first and second dimensions? What should I pay attention to when uploading files in batches?
Two-dimensional array.The first dimension is the name of the upload control, and the two-dimensional subscripts are name/type/tmp_name/size/error.
53. What are the main functions of the header() function? What should you pay attention to during use?
Answer:
header() sends http header information
-header("content-type:text/html; charset=utf-8");--------- ----------//The output content of the current page is html, encoded in utf-8 format
-
-header("content-type:image/png gif jpeg");------------------------ ----------//The format of the output content of the current page is picture
-header("refresh:5;url=http://www.1004javag.com/five/string.php") ;--//The page will jump to the new URL after 5 seconds
-header("location:http://1004javag.com/five/string.php");---------- -//Page redirection
54. How to use the header() function when downloading files?
Answer: header("content-type: application/octet-stream;charset=UTF- 8"); //What is the difference between adding utf-8 here and defining it above? ,? ?
header("accept-ranges: bytes");
header("accept-length: ".filesize($filedir.$filename));
header("content-disposition: attachment; filename= ".$filedir.$filename);
55. What is ajax? What is the principle of ajax? What is the core technology of ajax? What are the advantages and disadvantages of ajax?
Ajax is the abbreviation of asynchronous javascript and xml. It is a combination of multiple technologies such as javascript, xml, css, and DOM. '$' is an alias for jQuery.
The user's request in the page communicates with the server asynchronously through the ajax engine. The server returns the result of the request to the ajax engine.
The ajax engine finally decides Display the returned data to the specified location on the page. Ajax finally enables loading all the output content of another page at a specified location on one page.
This way, a static page can also obtain the returned data information from the database. Therefore, ajax technology enables a static web page to communicate with the server without refreshing the entire page.
It reduces user waiting time, reduces network traffic, and enhances the friendliness of the customer experience.
The advantages of Ajax are:
1. It reduces the burden on the server, transfers some of the work previously burdened by the server to the client for execution, and utilizes the idle resources of the client for processing;
2. Updating the page with only partial refresh increases the page response speed and makes the user experience more friendly.
The disadvantage of Ajax is that it is not conducive to SEO promotion and optimization, because search engines cannot directly access the content requested by Ajax.
The core technology of ajax is XMLHttpRequest, which is an object in javascript.
56. What is jquery? What are the ways to simplify ajax in jquery?
jQuery is a framework for Javascript.
$.get(),$.post(),$.ajax(). $ is an alias for jQuery object.
The code is as follows:
$.post(url address for asynchronous access, {'parameter name': parameter value}, function(msg){
$("#result").html( msg);
});
$.get(url address for asynchronous access, {'parameter name': parameter value}, function(msg){
$("#result") .html(msg);
data ;
57. What is session control?
Simply put, session control is a mechanism for tracking and identifying user information. The idea of session control is to be able to track a variable in the website. Through this variable, the system can identify the corresponding user information. Based on this user information, the user permissions can be known, so as to display the page content suitable for the user's corresponding permissions. Currently, the most important session tracking methods include cookies and sessions.
58. Basic steps of session tracking
1). Access the session object related to the current request 2). Find information related to the conversation
3). Store session information 4). Discard session data
59. What are the precautions for using cookies?
1) There cannot be any page output before setcookie(), even spaces or blank lines;
2) After setcookie(), calling $_COOKIE['cookiename'] on the current page does not work There will be output, and you must refresh or go to the next page to see the cookie value; 3) Different browsers handle cookies differently. The client can disable cookies, and the browser can also idle the number of cookies. A browser The maximum number of cookies that can be created is 300, and each cookie cannot exceed 4kb. The total number of cookies that can be set by each web site cannot exceed 20.
4) Cookies are stored on the client side. If the user disables cookies, setcookie will not work. So don't rely too much on cookies.
60. When using session, what is used to represent the current user to distinguish it from other users?
sessionid, the current session_id can be obtained through the session_id() function.
61. What are the steps for using session and cookie? What is the life cycle of session and cookie? What is the difference between session and cookie?
Cookies are stored on the client machine. For cookies with no expiration time set, the cookie value will be stored in the machine's memory. As long as the browser is closed, the cookie will disappear automatically. If the cookie expiration time is set, the browser will save the cookie to the hard disk in the form of a text file, and the cookie value will still be valid when the browser is opened again.
Session is to save the information that the user needs to store on the server side. Each user's session information is stored on the server side like a key-value pair, where the key is the sessionid and the value is the information the user needs to store. The server uses sessionid to distinguish which user the stored session information belongs to. The biggest difference between the two is that the session is stored on the server side, while the cookie is on the client side. Session security is higher, while cookie security is weak.
Session plays a very important role in web development. It can record the user's correct login information into the server's memory. When the user accesses the management backend of the website with this identity, the user can get identity confirmation without logging in again. Users who have not logged in correctly will not be allocated session space, and will not be able to see the page content even if they enter the access address of the management background. The user's operation permissions on the page are determined through the session.
Steps to use session:
1. Start session: Use the session_start() function to start.
2. Register session: Just add elements to the $_SESSION array directly.
3. Use session: Determine whether the session is empty or registered. If it already exists, use it like an ordinary array.
4. Delete session:
1. You can use unset to delete a single session;
2. Use $_SESSION=array() to log out all session variables at once;
3. Use session_d estro( ) function to completely destroy the session.
How to use cookies?
1. Record part of the information visited by the user
2. Pass variables between pages
3. Store the viewed internet page in the cookies temporary folder, which can improve future browsing speed.
Create cookie: setcookie(string cookiename, string value, int expire);
Read cookie: Read the cookie value on the browser side through the super global array $_COOKIE.
Deleting cookies: There are two methods
1. Manual deletion method:
Right-click the browser properties, you can see delete cookies, perform the operation to delete all cookie files.
2.setcookie() method:
Same as setting cookie, but this time the cookie value is set to empty, and the valid time is 0 or less than the current timestamp.
62. How to set the name of a cookie to username, the value to jack, and make the cookie expire after one week?
How many cookies can a browser generate at most, and what is the maximum size of each cookie file?
setcookie('username','jack',time()+7*24*3600);
Up to 20 cookies can be generated, each of which cannot exceed 4K
63 . What needs to be done before setting or reading the session?
You can open session.auto_start = 1 directly in php.ini or use session_start() at the head of the page;
You can open the session. There should be no output before session_start(), including blank lines.
64. In actual development, in what situations is session used?
Session is used to store user login information and transfer values across pages.
1) Commonly used to assign user login information to the session after the user successfully logs in;
2) Used in verification code image generation, when the random code is generated and assigned to the session.
65. How many ways are there to log out of a session?
unset() $_SESSION=array(); session_destroy();
66. What is OOP? What are classes and objects? What are class attributes?
OOP (object oriented programming), which is object-oriented programming, the two most important concepts are classes and objects.
Everything in the world has its own attributes and methods, and different substances can be distinguished through these attributes and methods.
The collection of attributes and methods forms a class. Classes are the core and foundation of object-oriented programming.
Through classes, scattered codes used to implement a certain function can be effectively managed.
Class only has an abstract model of certain functions and attributes, and in practical applications requires one entity, that is, the classes need to be instantiated. The class is an object after instantiation. ★A class is an abstract concept of an object, and an object is an instantiation of a class.
The object is an advanced array, and the array is the most primitive object. The same object can also be traversed
OOP has three major characteristics:
1. Encapsulation: also known as information hiding, which is to hide an The use and implementation of the class are separated, and only some interfaces and methods are retained for external contact, or only some methods are exposed for developers to use. Therefore, developers only need to pay attention to how this class is used, without caring about its specific implementation process. This can achieve MVC division of labor and cooperation, and can also effectively avoid interdependence between programs and achieve loose coupling between code modules.
2. Inheritance: Subclasses automatically inherit the attributes and methods of their parent class, and can add new attributes and methods or rewrite some attributes and methods. Inheritance increases code reusability. PHP only supports single inheritance, which means that a subclass can only have one parent class.
3. Polymorphism: The subclass inherits the attributes and methods from the parent class and overrides some of the methods. Therefore, although multiple subclasses have the same method, objects instantiated by these subclasses can obtain completely different results after calling these same methods. This technology is polymorphism. Polymorphism increases software flexibility.
Advantages of OOP: 1. High code reusability (saving code) 2. High program maintainability (scalability) 3. Flexibility
67. Commonly used What are the access modifiers for properties? What do they mean?
private, protected, public.
Out of class: public, var
In subclass: public, protected, var
In this class: private, protected, public, var
If you do not use these three keywords, you can also use var keyword. But var cannot be used with permission modifiers. Variables defined by var can be accessed in subclasses and outside the class, equivalent to public
Before the class: only final, abstract
can be added Before the attribute: there must be access modifiers (private, protected, public, var)
In front of the method: static, final, private, protected, public, abstract
68. What do the three keywords $this and self and parent represent respectively? In what situations is it used?
$this current object self current class parent parent class of the current class
$this is used in the current class, use -> to call properties and methods.
Self is also used in the current class, but it needs to be called using ::. Parent is used in classes.
69. How to define constants in a class, how to call constants in a class, and how to call constants outside a class.
The constants in the class are also member constants. A constant is a quantity that does not change and is a constant value.
Use the keyword const.
to define constants. For example: const PI = 3.1415326;
Whether it is within a class or outside a class, the access of constants is different from that of variables. Constants do not need to instantiate objects,
The format for accessing constants is the class name plus the scope operator symbol (double colon) to call.
That is: class name :: class constant name;
70. How to use scope operator::? In what situations is it used?
Calling class constants Calling static methods
71. What is a magic method? What are some commonly used magic methods?
System-customized methods starting with __.
__construct() __destruct() __autoload() __call() __tostring()
72. What are construction methods and destructor methods?
The constructor method is a member method that is automatically executed when instantiating an object. Its function is to initialize the object.
Before php5, a method with exactly the same name as the class is a constructor method. After php5, the magic method __construct() is a constructor method. If there is no constructor defined in the class, PHP will automatically generate one. This automatically generated constructor has no parameters and no operations.
The format of the constructor is as follows:
function __construct(){}
Or: function class name(){}
The constructor can have no parameters or multiple parameters.
The role of the destructor method is exactly the opposite of the construction method. It is automatically called when the object is destroyed, and its function is to release memory.
The destructor method is defined as: __destruct();
Because PHP has a garbage collection mechanism that can automatically clear objects that are no longer used and release memory. Generally, you do not need to manually create a destructor method.
73. How does the __autoload() method work?
The basic condition for using this magic function is that the file name of the class file must be consistent with the name of the class.
When the program is executed to instantiate a certain class, if the class file is not introduced before instantiation, the __autoload() function will be automatically executed. This function will search for the path of this class file based on the name of the instantiated class. When it is determined that this class file does exist in the path of this class file, it will execute include or require to load the class, and then the program will continue to execute. If this path When the file does not exist,
will prompt an error. Using the autoloading magic function eliminates the need to write many include or require functions.
74. What are abstract classes and interfaces? What are the differences and similarities between abstract classes and interfaces?
An abstract class is a class that cannot be instantiated and can only be used as a parent class of other classes.
Abstract classes are declared through the keyword abstract.
“ rewritten.
The format of the abstract method is: abstract function abstractMethod();
Because PHP only supports single inheritance, if you want to implement multiple inheritance, you must use an interface. In other words, subclasses can implement multiple interfaces. Interface classes are declared through the interface keyword. Member variables and methods in interface classes are public. Methods do not need to write the keyword public, and methods in interfaces do not have method bodies. The methods in the interface are also inherently intended to be implemented by subclasses. The functions implemented by abstract classes and interfaces are very similar. The biggest difference is that interfaces can implement multiple inheritance. The choice between abstract class or interface in an application depends on the specific implementation. Subclasses inherit abstract classes using extends, and subclasses implement interfaces using implements.
Does an abstract class have at least one abstract method? ? ? ? ? ?
A: If a class is declared as an abstract class, it does not need an abstract method
If there is an abstract method in a class, the class must be an abstract class
75. The parameters of __call are: How many, what are the types and what is the significance? The function of the magic method __call() is that when the program calls a non-existent or invisible member method, PHP will first call the __call() method and change the method name and parameters of the non-existent method. Store it.
__call() contains two parameters. The first parameter is the method name of the non-existent method, which is a string type;
.
I think the __call() method is more about debugging and can locate errors. At the same time, exceptions can be caught, and if a method does not exist, other alternative methods will be executed.
76. What is the use of smarty template technology? In order to separate php and html, artists and programmers can perform their respective duties without interfering with each other.
77. What are the main configurations of smarty? 1. Introduce smarty.class.php;
2. Instantiate the smarty object;
3. Re-modify the default template path;
4. Re-modify the default compiled file Path;
5. Re-modify the default configuration file path;
6. Re-modify the default cache path.
7. You can set whether to enable cache.
8. You can set left and right delimiters.
78. What details do you need to pay attention to when using smarty? Smarty is a template engine based on the MVC concept. It divides a page program into two parts for implementation: the view layer and the control layer. In other words, smarty technology separates the user UI from the PHP code. In this way, programmers and artists perform their respective duties without interfering with each other.
You should pay attention to the following issues when using smarty:
1. Configure smarty correctly.It mainly needs to instantiate the smarty object and configure the path of the smarty template file;
2. Use assign assignment and display to display the page in the php page;
3. PHP code snippets and all comments are not allowed in the smarty template file. Variables and functions must be included in delimiters.
A.{}
🎜>
79. What is the concept of MVC? What are the main tasks of each level?
MVC (Model-View-Controller) is a software design pattern or programming idea.
M refers to the Model layer, V is the View layer (display layer or user interface), and C is the Controller layer.
The purpose of using mvc is to separate M and V so that one program can easily use different user interfaces. In website development, the model layer is generally responsible for adding, deleting, modifying, and checking database table information. The view layer is responsible for displaying page content. The controller layer plays a regulating role between M and V. , the controller layer decides which method of which model class to call.
After execution, the controller layer decides which view layer to assign the result to.
81. What do method rewriting and overloading mean in Java language? To be precise, does PHP support method overloading? How to actually understand the PHP overloading mentioned in many reference books correctly?
Answer:
PHP does not support method overloading. The PHP 'overloading' mentioned in many books should be 'rewriting'
82. final keyword Can you define member attributes in a class?
Answer: No, the member attributes of a class can only be defined by public, private, protected, var
83. Can a class defined by the final keyword be inherited?
Answer: Classes defined by final cannot be inherited
84. Tell us about the use cases of the static keyword? Can static be used before class?
Can static be used together with public, protected, and private? Can a constructor be static?
Answer: Static can be used in front of properties and methods. When calling static attributes or methods, it can be used as long as the class is loaded without instantiation
Static cannot be used in front of class
Static can be used with public. Protected and private are used together, in front of the method; Can abstract classes be instantiated?
Answer: Neither interfaces nor abstract classes can be instantiated
86. Can access modifiers be added in front of class? If it can be added, which access modifiers can it be? Can it be the permission access modifier public, protected, private?
Answer: Final and static can be added in front of class;
★public, protected, private cannot be added in front of class
87. There is no need to add access modifiers before attributes in a class ? Can the modifiers before member variables only be public, protected, or private? Which other ones could it be?
Answer: The attributes in the class must add modifiers. In addition to those 3, you can also add var
88. If you echo an array, what will the page output? What about echoing an object? What about printing an array or object?
Answer: The page can only output "Array"; echoing an object will result in "Catchable fatal error: Object of class t2 could not be converted to string in G:php2t2.php on line 33"
print When printing an array, it only outputs "Array". When printing an object, "Catchable fatal error: Object of class t2 could not be converted to string in G:php2t2.php" ▲print and echo are the same.
89. When is the __tostring() magic method automatically executed?
Does the __tostring() magic method have to return a return value?
When echo or print an object, it is automatically triggered. And __tostring() must return a value
90. What is an abstract method?
Answer: There is abstract in front of the method, and the method has no method body, not even "{ }"
91. If a method in a class is an abstract method, If this class is not defined as an abstract class, will an error be reported?
Answer: Yes, "Fatal error: Class t2 contains 1 abstract method and must therefore be declared abstract or implement the remaining methods (t2::ee) in"
92. If a class is an abstract class and the methods in the class are non-abstract methods, will an error be reported?
Answer: No error will be reported. If a class is an abstract class, it does not need to have an abstract method, but a method in a class is an abstract method, then this class must be an abstract class
94. What issues should be paid attention to when applying the final keyword?
Classes defined using the final keyword are prohibited from inheritance.
Methods defined using the final keyword are prohibited from overriding.
95. If a class wants to inherit a parent class and implement multiple interfaces, how should it be written?
Writing format such as: class MaleHuman extends Human implements Animal,Life { ... }
96. What is a single point of entry?
The so-called single point of entry means that there is only one entrance for the entire application, and all implementations are forwarded through this entrance.
For example, above we use index.php as the single point of entry for the program. Of course this It can be controlled at will by yourself.
There are several advantages to a single point of entry:
First, some variables, classes, and methods processed globally by the system can be processed here. For example, you need to perform preliminary filtering of data, you need to simulate session processing, you need to define some global variables, and you even need to register some objects or variables into the register
Second, the program structure is clearer.
97. PHP provides 2 sets of regular expression function libraries, which ones are they?
(1) PCRE Perl-compatible regular expressions preg_ is prefixed with
(2) POSIX Portable Operating System Interface ereg_ is prefixed with
98. What is the composition of regular expressions?
consists of atoms (ordinary characters, such as English characters),
metacharacters (characters with special functions)
pattern correction characters
A regular expression contains at least one atom
99. What is the triggering time for uncommon magic methods?
__isset() Trigger timing of __unset()
__sleep(), __wakeup() are called when serializing the object
If you serialize the object, do not write __sleep( ) method, all member attributes will be serialized, and if the __sleep() method is defined, only the variables in the specified array will be serialized. Therefore, this function is also useful if you have very large objects that do not need to be stored completely.
The purpose of using __sleep is to close any database connections the object may have, submit pending data, or perform similar cleanup tasks. Additionally, this function is useful if you have very large objects that do not need to be stored completely.
The purpose of using __wakeup is to re-establish any database connections that may have been lost during serialization and to handle other re-initialization tasks.
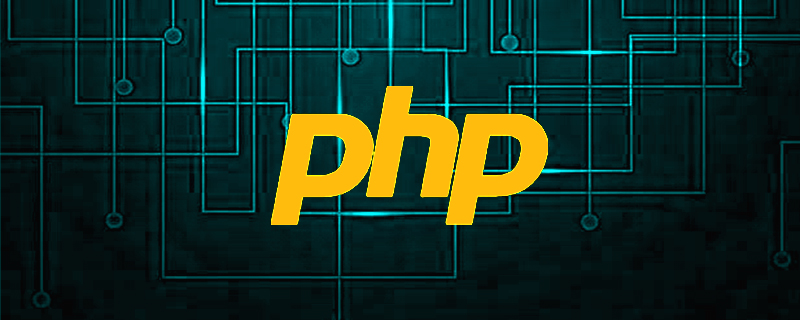
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
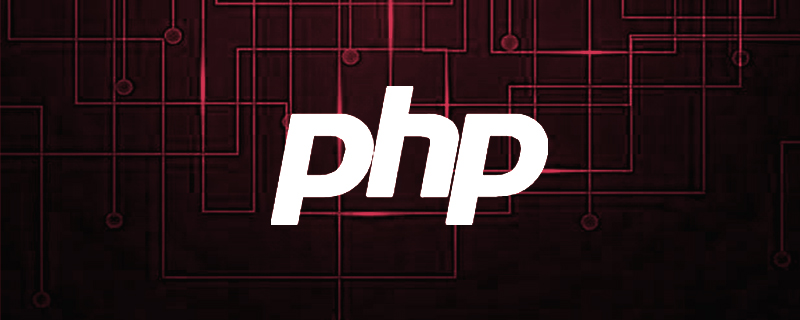
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
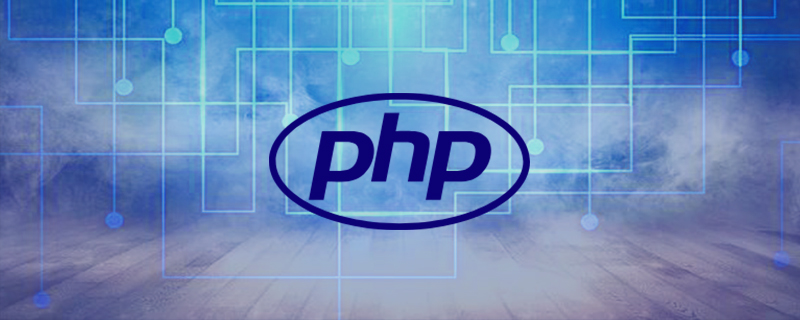
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
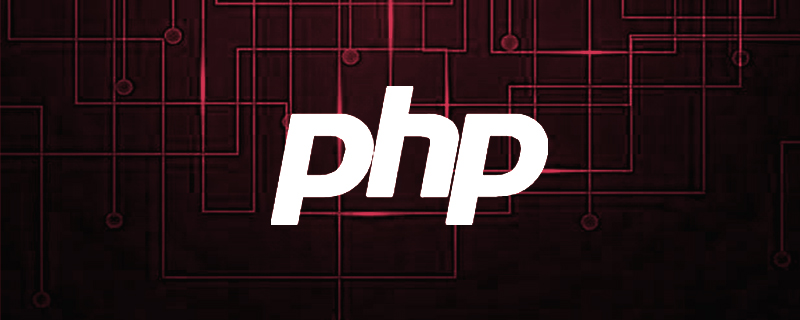
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
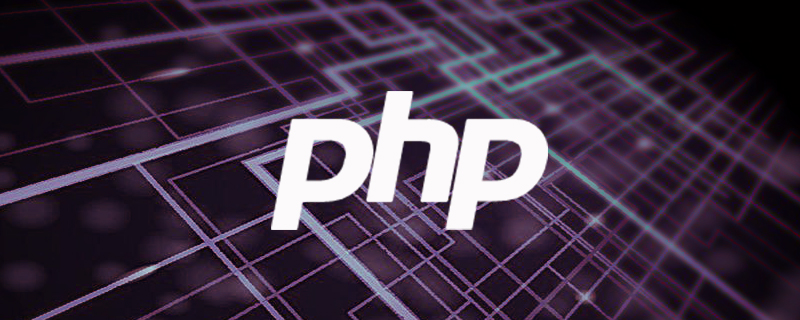
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
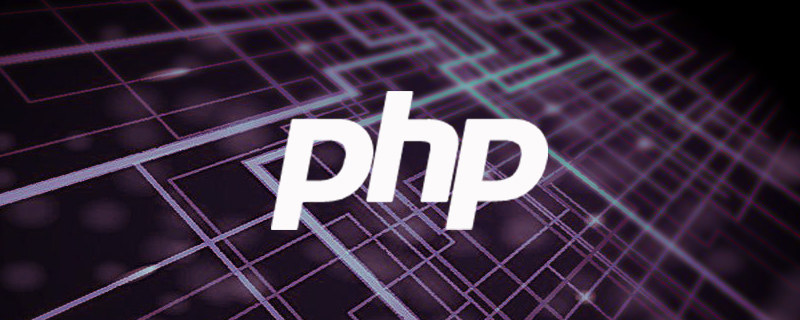
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
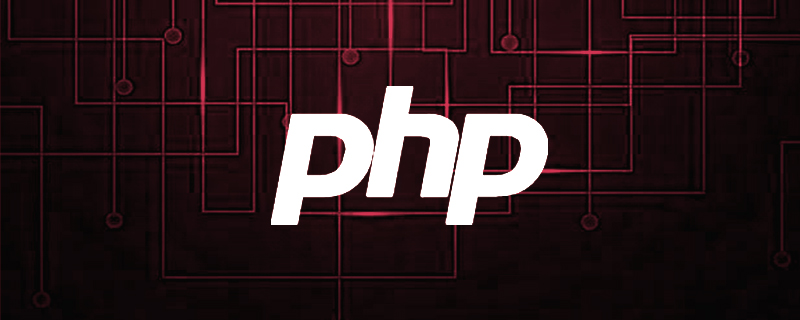
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
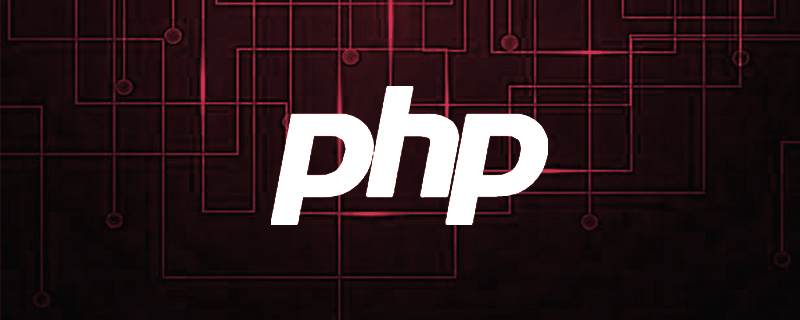
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
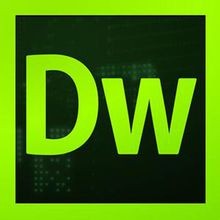
Dreamweaver CS6
Visual web development tools
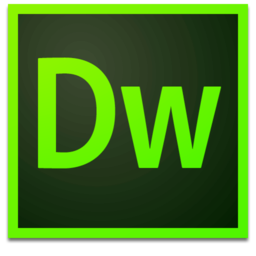
Dreamweaver Mac version
Visual web development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
