


1: Why can’t I get the variable?
I POST data name from one web page to another web page, why can’t I get any value when I output $name?
In PHP4. In versions 2 and later, register_global defaults to off
If you want to get the variables submitted from another page:
Method one: Find register_global in PHP.ini and set it to on.
Method two: Put this extract($_POST);extract($_GET); at the front of the receiving web page (note that there must be Session_Start() before extract($_SESSION)).
Method 3:Read variables $a=$_GET["a"];$b=$_POST["b"], etc. one by one. Although this method is troublesome, it is safer.
2: Debugging your program
You must know the value of a certain variable at runtime. This is what I did, create a file debug.php with the following content:
PHP code:-------------------------- ---------
Ob_Start();
Session_Start( );
Echo "
"; <br>Echo "The _GET variables obtained on this page are:"; <br>Print_R($_GET); <br>Echo "The _POST variables obtained on this page There are: "; <br>Print_R($_POST); <br>Echo "The _COOKIE variables obtained on this page are:"; <br>Print_R($_COOKIE); <br>Echo "The _SESSION variables obtained on this page There are: "; <br>Print_R($_SESSION); <br>Echo "";
---------------- ----------------------------------
Then set in php.ini: include_path = "c:/php", And put debug.php in this folder,
You can include this file in every web page in the future and view the obtained variable names and values.
3: How to use session
Everything related to session must call the function session_start() before;
Paying value for session is very simple, such as:
Session_start();
$Name = "This is a Session example";
Session_Register("Name ");//Note, do not write: Session_Register("$Name");
Echo $_SESSION["Name"];
//After that $_SESSION["Name"] is "This is a Session example "
After php4.2, you can pay directly for the session:
Session_Start();
$_SESSION["name"]="value";
Cancel the session like this:
session_start();
session_unset();
session_destroy();
Cancel a certain The session variable still has bugs in php4.2 and above.
Note:
1: There cannot be any output before calling Session_Start(). For example, the following is wrong.
========== =================================
1 line
2 lines [php]
3 Line Session_Start();//There has been output in the first line before
4 lines....
5 lines[/php]
============== =============================
Tip 1:
Whenever "... .....headers already sent.....", which is the information output to the browser before Session_Start().
Remove the output and it will be normal. (This error will also occur in COOKIE. The reason for the error Same)
Tip 2:
If your Session_Start() is placed in a loop statement and it is difficult to determine where the information was output to the browser before, you can use the following method:
1 Line [php] Ob_Start(); [/php]
.....Here is your program...
2: What is the error?
Warning: session_start( ): open(/tmpsess_7d190aa36b4c5ec13a5c1649cc2da23f, O_RDWR) failed:....
Because you did not specify the storage path of the session file.
Solution:
(1) Create the folder tmp in the c drive
(2) Open php.ini, find session.save_path, and change it to session.save_path= "c: /tmp"
4: Why when I send variables to another web page, I only get the first half, and all the ones starting with spaces are lost
$Var="hello php";//Change to $Var=" hello php"; Try to get the result
$ post= "receive.php?Name=".$Var;
header("location:$post");
Content of receive.php:
Echo "
"; <br>Echo $_GET["Name"]; <br>Echo "< ;/pre>"; <br>
The correct method is:
$Var="hello php";
$post= "receive.php?Name=".urlencode($Var);
header("location:$post");
You don’t need to use Urldecode() on the receiving page, the variables will be automatically encoded.
5: How to intercept Chinese characters of a specified length without ending with "[/php]", and the excess part is replaced with "..."
Generally speaking, the variables to be intercepted come from Mysql, first ensure that the field length is long enough, usually char(200), which can hold 100 Chinese characters, including punctuation.
$str="This character is so long, ^_^";
$Short_Str=showShort($str,4);//Intercept the first 4 Chinese characters, the result is: This character...
Echo "$Short_Str";
Function csubstr($str,$start,$len)
{
$strlen=strlen($str);
$clen =0;
for($i=0;$i{
if ($clen>=$start+$len)
break;
if(ord(substr($str,$i,1))>0xa0)
{
if ($clen>=$start)
$tmpstr.=substr($str,$i ,2);
$i++;
}
else
{
if ($clen>=$start)
$tmpstr.=substr($str,$i,1 );
}
}
return $tmpstr;
}
Function showShort($str,$len)
{
$tempstr = csubstr($str,0, $len);
if ($str$tempstr)
$tempstr .= "..."; //What you want to end with, just modify it here.
return $tempstr;
}
6: Standardize your SQL statements
Add "`" in front of tables and fields so that they will not appear due to misuse of keywords Wrong,
Of course I don’t recommend you to use keywords.
For example
$Sql="INSERT INTO `xltxlm` (`author`, `title`, `id`, `content`, `date`) VALUES ('xltxlm', ' use`', 1, 'criterion your sql string ', '2003-07-11 00:00:00')"
How to enter "`"? On the TAB key.
7: How to make the Html/PHP format string not to be interpreted, but displayed as it is
$str="
PHP
";Echo "Interpreted: ".$str."
Processed: ";
Echo htmlentities(nl2br($str));
8: How to get the variable value outside the function in the function
$a="PHP";
foo();
Function foo()
{
global $a;//Delete here to see what the result is
Echo "$a";
}
9: How do I know what functions are supported by the system by default?
$arr = get_defined_functions();
Function php() {
}
echo "
"; <br>Echo "This displays all functions supported by the system, and the custom function phpn"; <br>print_r($arr); <br>echo "";
10: How to compare the number of days between two dates
$Date_1="2003-7-15";//It can also be:$Date_1="2003-6-25 23:29:14";
$Date_2="1982-10-1";
$Date_List_1=explode("-",$Date_1);
$Date_List_2=explode("-",$Date_2);
$d1= mktime(0,0,0,$Date_List_1[1],$Date_List_1[2],$Date_List_1[0]);
$d2=mktime(0,0,0,$Date_List_2[1],$Date_List_2[ 2],$Date_List_2[0]);
$Days=round(($d1-$d2)/3600/24);
Echo "I have struggled for $Days days^_^";
11: Why after I upgraded PHP, the original program showed a full screen Notice: Undefined variable:
This is a warning, caused by the variable being undefined.
Open php .ini, find the error_reporting at the bottom, change it to error_reporting = E_ALL & ~E_NOTICE
For Parse error error
error_reporting(0) cannot be closed.
If you want to close any error prompts, open php.ini, Find display_errors and set it to display_errors = Off. Any errors in the future will not be prompted.
Then what is error_reporting?
12: I want to add a file at the beginning and end of each file. But adding them one by one is troublesome
1: Open the php.ini file
Set include_path= "c:"
2: Write two files
auto_prepend_file.php and auto_append_file.php are saved in the c drive, they will be automatically attached to the head and tail of each php file.
3: Found in php.ini:
Automatically add files before or after any PHP document.
auto_prepend_file = auto_prepend_file.php; attached to the head
auto_append_file = auto_append_file .php; attached to the tail
In the future, each of your php files will be equivalent to
Include "auto_prepend_file.php" ;
.....//Here is your program
Include "auto_append_file.php";
13: How to upload files using PHP
14: How to configure the GD library
The following is my configuration process
1: Use the dos command (you can also do it manually, copy all the dll files in the dlls folder to the system32 directory ) copy c:phpdlls*.dll c:windowssystem32
2: Open php.ini
Set extension_dir = "c:/php/extensions/";
3:
extension=php_gd2.dll; Remove the comma in front of the extension. If there is no php_gd2.dll, the same goes for php_gd.dll. Make sure this file does exist c:/php/extensions/php_gd2.dll
4: Run the following program to test
Ob_end_flush();
/ /Note, no information can be output to the browser before this, please pay attention to whether auto_prepend_file is set.
header ("Content-type: image/png");
$im = @imagecreate (200, 100)
or die ("Unable to create image");
$background_color = imagecolorallocate ($im, 0,0, 0);
$text_color = imagecolorallocate ($im, 230, 140, 150);
imagestring ($im, 3, 30, 50, "A Simple Text String", $text_color);
imagepng ($im);
15: What is UBB Code
UBB code is a variant of HTML and a special TAG used by Ultimate Bulletin Board (a foreign BBS program, which is also used in many places in China).
Even if it is prohibited Using HTML, you can also use UBBCode? Maybe you prefer to use UBBCode? instead of HTML, even if the forum allows the use of HTML, because it uses less code and is safer.
There are examples in Q3boy's UBB. You can run tests directly
16: I want to modify the MySQL user and password
First of all, I must declare that in most cases, modifying MySQL requires root permissions in mysql.
So ordinary users Password cannot be changed without requesting an administrator.
Method 1
Use phpmyadmin, this is the simplest, modify the user table of the mysql library,
But don’t forget to use the PASSWORD function.
Method 2
Using mysqladmin, this is a special case stated earlier.
Mysqladmin -u root -p password mypasswd
After entering this command, you need to enter the original password of root, and then the root password will be changed to mypasswd.
Change root in the command to your username, and you can change your own password.
Of course, if your mysqladmin cannot connect to the mysql server, or you cannot execute mysqladmin,
then this method is invalid.
And mysqladmin cannot clear the password.
The following methods are used at the mysql prompt and must have root permissions for mysql:
Method 3
mysql> INSERT INTO mysql.user ( Host,User,Password)
VALUES('%','jeffrey',PASSWORD('biscuit'));
mysql> FLUSH PRIVILEGES
To be precise, this is adding a user with the user name jeffrey, the password is biscuit.
There is this example in the "mysql Chinese Reference Manual", so I wrote it out.
Be careful to use the PASSWORD function, and then use FLUSH PRIVILEGES.
Method 4
Same as method 3, except that the REPLACE statement is used
mysql> REPLACE INTO mysql.user (Host,User,Password)
VALUES('%' ,'jeffrey',PASSWORD('biscuit'));
mysql> FLUSH PRIVILEGES
Method 5
Use the SET PASSWORD statement,
mysql> SET PASSWORD FOR jeffrey@"%" = PASSWORD('biscuit');
You must also use PASSWORD() Function,
But there is no need to use FLUSH PRIVILEGES.
Method 6
Use GRANT... IDENTIFIED BY statement
mysql> GRANT USAGE ON *.* TO jeffrey@"%" IDENTIFIED BY 'biscuit';
Here PASSWORD () function is unnecessary, and there is no need to use FLUSH PRIVILEGES.
Note: PASSWORD() [does not] perform password encryption in the same way as Unix password encryption.
17: I want to know which website he connected to this page through
//You must enter through a super connection to have output
Echo $_SERVER['HTTP_REFERER'];
18: What should you pay attention to when putting data into the database and taking it out to display on the page
When entering the database
$str=addslashes($str);
$sql="insert into `tab` (`content`) values('$str')";
When leaving the library
$str=stripslashes($str);
When displaying
$str=htmlspecialchars(nl2br ($str)) ;
19: How to read the current address bar information
$s="http://{$_SERVER['HTTP_HOST']}:{$_SERVER["SERVER_PORT"]}{$_SERVER['SCRIPT_NAME']}";
$se='';
foreach ($_GET as $key => $value) {
$se.=$key."=".$value."&";
}
$se=Preg_Replace("/( .*)&$/","$1",$se);
$se?$se="?".$se:"";
echo $s."?$se";
20: When I clicked the back button, why did the previously filled out stuff disappear?
This is because you used session.
Solution:
session_cache_limiter('private, must-revalidate');
session_start();
.... .......
..........
21: How to display the IP address in the picture
Header("Content-type: image/png");
$img = ImageCreate(180,50 );
$ip = $_SERVER['REMOTE_ADDR'];
ImageColorTransparent($img,$bgcolor);
$bgColor = ImageColorAllocate($img, 0x2c,0x6D,0xAF); // Background color
$shadow = ImageColorAllocate($img, 250,0,0); // Shadow color
$textColor = ImageColorAllocate($img, oxff,oxff,oxff); // Font color
ImageTTFText($ img,10,0,78,30,$shadow,"d:/windows/fonts/Tahoma.ttf",$ip); //Display background
ImageTTFText($img,10,0,25,28, $textColor,"d:/windows/fonts/Tahoma.ttf","your ip is".$ip); // Display IP
ImagePng($img);
imagecreatefrompng($img);
ImageDestroy($img);
22: How to get the user’s real IP
function iptype1 () {
if (getenv("HTTP_CLIENT_IP")) {
return getenv("HTTP_CLIENT_IP");
}
else {
return "none";
}
}
function iptype2 () {
if (getenv("HTTP_X_FORWARDED_FOR")) {
return getenv("HTTP_X_FORWARDED_FOR");
}
else {
return "none";
}
}
function iptype3 () {
if (getenv("REMOTE_ADDR")) {
return getenv( "REMOTE_ADDR");
}
else {
return "none";
}
}
function ip() {
$ip1 = iptype1();
$ip2 = iptype2();
$ip3 = iptype3();
if (isset($ip1) && $ip1 != "none" && $ip1 != "unknown") {
return $ip1;
}
elseif (isset($ip2) && $ip2 != "none" && $ip2 != "unknown") {
return $ip2;
}
elseif (isset($ip3) && $ip3 != "none" && $ip3 != "unknown") {
return $ip3;
}
else {
return "none";
}
}
Echo ip();
23: How to read all records within three days from the database
First there must be a The DATETIME field records the time,
The format is '2003-7-15 16:50:00'
SELECT * FROM `xltxlm` WHERE TO_DAYS(NOW()) - TO_DAYS(`date`)
24: How to remotely connect to Mysql database
There is a host field in the mysql table of adding users, change it to "%", or specify the IP address that allows the connection, so that you can Called remotely.
$link=mysql_connect("192.168.1.80:3306","root","");
25: How to use regular expressions
Special characters in regular expressions
26: After using Apache, garbled characters appear on the homepage
Method 1:
AddDefaultCharset ISO-8859-1 is changed to AddDefaultCharset off
method Two:
AddDefaultCharset GB2312
====================================== ===================
tip:
When you post the code, GB2312 will be interpreted as??????
Changed to This way it won’t
GB2312
10: How to compare the number of days between two dates, (simpler algorithm)
$Date_1="2003-7-15";//It can also be: $Date_1="2003-7-15 23:29:14";
$Date_2="1982-10- 1";
$d1=strtotime($Date_1);
$d2=strtotime($Date_2);
$Days=round(($d1-$d2)/3600/24);
Echo "I have struggled for $Days days^_^";
27: Why do single and double quotes become ('") on the acceptance page?
Solution:
Method 1: Set: magic_quotes_gpc = Off in php.ini
Method 2: $str=stripcslashes($str )
28: How to keep the program running instead of stopping after more than 30 seconds
set_time_limit(60)//The maximum running time is one minute
set_time_limit(0)//Run until the program ends by itself, or Manual stop
29: Calculate the number of people currently online
Example 1: Use text to implement
//First of all, you must have permission to read and write files
//This program can be run directly. If an error is reported for the first time, it can be run later
$online_log = "count.dat"; // Save the file of the number of people,
$timeout = 30; //If there is no action within 30 seconds, it is considered offline
$entries = file($online_log);
$temp = array();
for ($i=0;$i
if (($entry[0] != getenv('REMOTE_ADDR')) && ($entry[1] > time())) {
array_push($temp,$entry[0].",".$ entry[1]."n"); //Get the information of other viewers, remove the timeout ones, and save it into $temp
}
}
array_push($temp,getenv('REMOTE_ADDR') .",".(time() + ($timeout))."n"); //Update the viewer's time
$users_online = count($temp); //Calculate the number of people online
$entries = implode("",$temp);
//Write file
$fp = fopen($online_log,"w");
flock($fp,LOCK_EX); //flock() Cannot work properly in NFS and some other network file systems
fputs($fp,$entries);
flock($fp,LOCK_UN);
fclose($fp);
echo " Currently there is ".$users_online."People are online";
Example 2:
Use database to implement online users
30: What is a template and how to use it
I use phplib template
Here are the uses of several functions
$T->Set_File("Define whatever you want ","Template file.tpl");
$T->Set_Block("defined in set_file","","define as you like");
$T->Parse("Defined in Set_Block","",true);
$T->Parse("Output the result as you like"," ");
defined in Set_File sets the loop format to:
How to generate the template Static web page
//The phplib template is used here
…. .....
............
$tpl->parse("output","html");
$output = $tpl-> get("output");// $output is the entire web page content
function wfile($file,$content,$mode='w') {
$oldmask = umask(0);
$ fp = fopen($file, $mode);
if (!$fp) return false;
fwrite($fp,$content);
fclose($fp);
umask($ oldmask);
return true;
}
// Write to file
Wfile($FILE,$output);
header("location:$FILE");//Repeat Directed to the generated web page
}
31: How to use php to interpret the characters
For example: input 2+2*(1+2), automatically output 8
You can use the eval function
[php]
$str=$ _POST['str'];
eval("$o=$str;");
Echo "$o";
-------------------------------------------------- -----------------------
In addition, you must be particularly careful when using this function!!
What will be the result if someone enters format: d:?
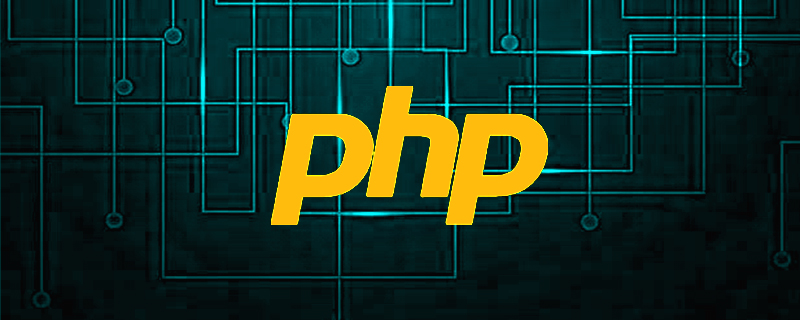
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
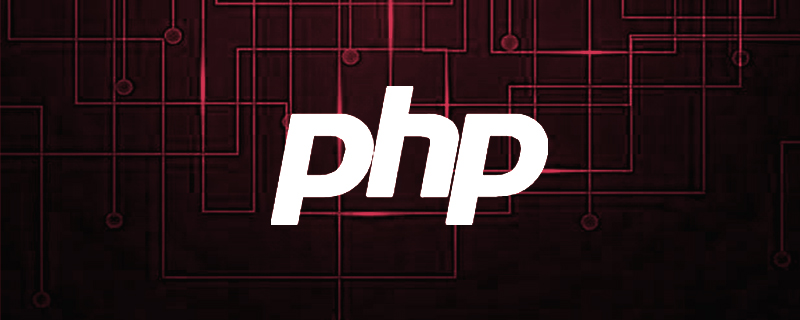
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
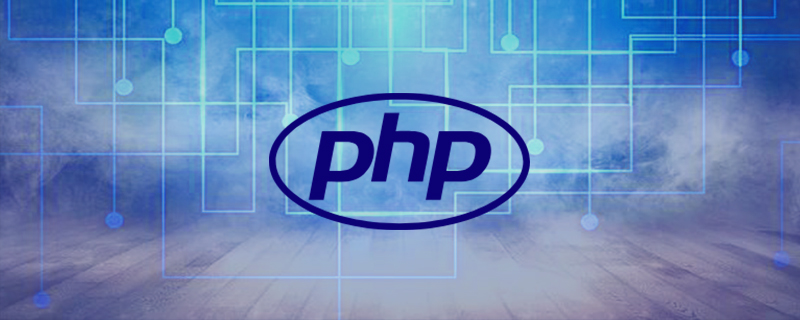
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
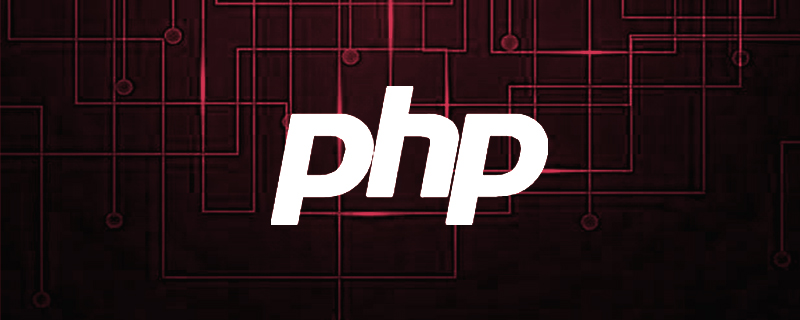
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
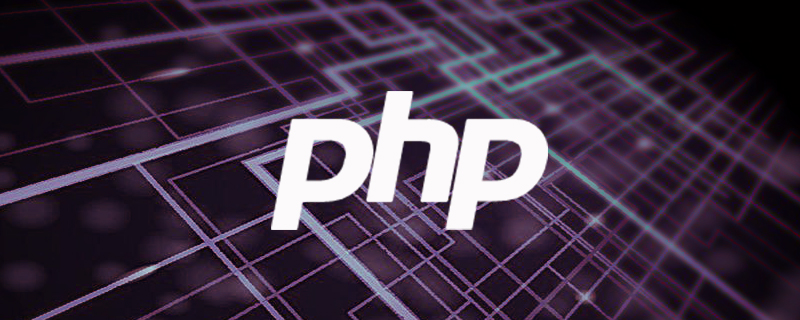
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
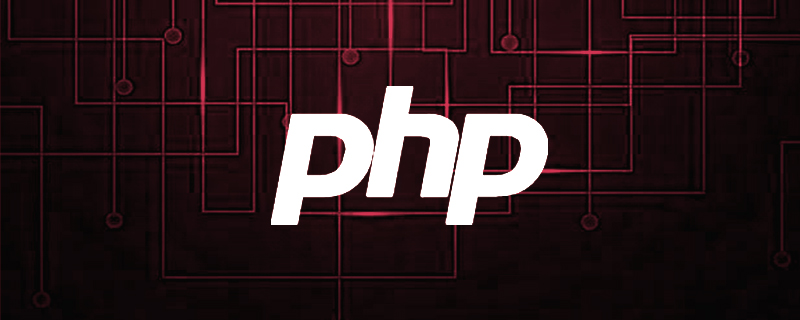
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
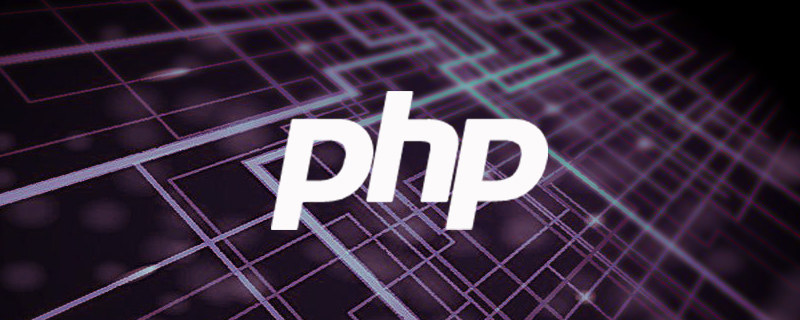
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
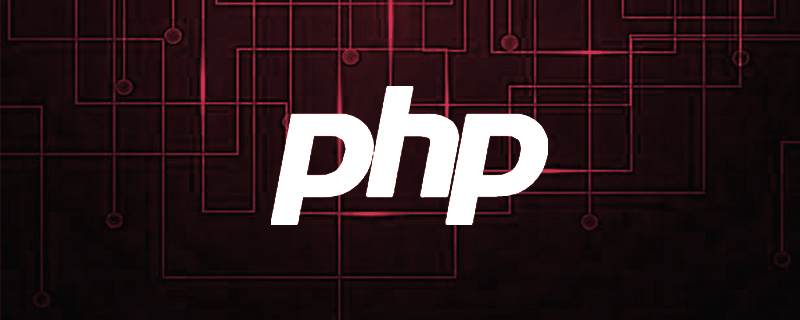
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
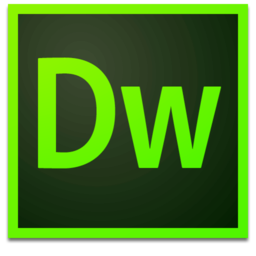
Dreamweaver Mac version
Visual web development tools
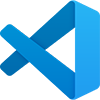
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
