


Detailed explanation of the evolution process of PHP's mvc framework_PHP tutorial
1) /********** Mixed php and html to complete the add, delete, modify and check functions*******************************/
1. Design idea:
Based on the usual practice of adding, deleting, modifying and checking functions, that is, completing the connection operation to the database in a php file
And display html code in php file. The html is submitted to the php part of the current page for processing and storage.
$_SERVER['SCRIPT_FILENAME'] contains the path of the current script.
This is useful when the page needs to point to itself.
Different from __FILE__ constant, it contains the full path and file name of the current script (such as an include file).
First class: Add product file: addProduct.php
if(isset($_POST['submit'])){
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
//Connect to database operation
mysql_connect('localhost','root','master');
mysql_select_db('test');
mysql_query('set names gbk');
$query= "insert into product values(null,'$name','$price','$description')";
mysql_query($query);
mysql_close();
}
?>
Product name
Product price
Product description
Idea: Both operate the database and display in the same file (in the current file):
Mysql_fetch_assoc returns an array. Generally, it needs to be placed in an empty array to form a two-dimensional array and returned to the page. mysql_num_rows returns the number of rows
Generally used to query data:
Php
$result = Mysql_query(“select * fromproduct order by id desc”);
$data = array();
While ($row = Mysql_fecth_assoc($result)){
$data[]= $row;
}
Html:
…
Query product file: listProduct.php
//Connect database operation
mysql_connect('localhost','root','master');
mysql_select_db('test');
mysql_query('set names gbk');
$query = "select * from product order by id desc";
$result = mysql_query($query);
$data = array();
while($row = mysql_fetch_assoc($result)){
$data[]= $row;
}
?>
foreach($dataas$row):
?>
Idea: Next, delete and update operations are performed, and the same request is made to a new php file to handle the deletion and update operations. Create a new php file: delProduct.php
delProduct.php:
//Connect database operation
mysql_connect('localhost','root','master');
mysql_select_db('test');
mysql_query('set names gbk');
$id = $_GET['id'];
$query = "delete from product where id = '$id'";
mysql_query($query);
mysql_close();
//At the same time jump to listProduct to display the results
header('location:listProduct.php');
Update operation:
updateProduct.php (The following class is only for display. Submitting modifications also requires a php file. In order not to add another file, modifications are mentioned on this page and a parameter is added to the action to distinguish the display and submission of modifications)
//Connect database operation
mysql_connect('localhost','root','master');
mysql_select_db('test');
mysql_query('set names gbk');
$id = $_GET['id'];
$query = "select * from product where id = '$id'";
$result = mysql_query($query);
$row = mysql_fetch_row($result);
?>
Product name
Product price
Product description
The revised content is as follows:
//Connect database operation
mysql_connect('localhost','root','master');
mysql_select_db('test');
mysql_query('set names gbk');
if(isset($_REQUEST['flag'])){
$id= $_POST['id'];
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
$query= "update product set name = '$name', price = '$price' , description = '$description' where id='$id'";
mysql_query($query);
mysql_close();
header('location:listProduct.php');
}else{
$id= $_GET['id'];
$query= "select * from product where id = '$id'";
$result= mysql_query($query);
$row= mysql_fetch_row($result);
}
?>
Product name
Product price
Product Description
/**************Add the DB class to encapsulate the operation of the database*****************/
At this point, the basic functions of adding, deleting, modifying and checking products have been completed. However, there are a lot of redundant codes, such as database operations. At this time, you can consider extracting the data operations:
DB.class.php:
/**
* Database operation class
* @author heyongjia
*
*/
class DB{
private$host ='localhost';
private$username ='root';
private$password ='master';
private$dbname ='test';
private$setCoding ='set names gbk';//Pay attention to the encoding settings, sometimes the insertion is unsuccessful
private$conn;//Connect resources
private$result;//result set
public functionconnect(){
$this->conn =mysql_connect($this->host,$this->username,$this->password);
mysql_select_db($this->dbname);
mysql_query($this->setCoding);
}
public functionquery($query){
$this->result = mysql_query($query);
}
public functionclose(){
mysql_close();
}
}
Then modify the database operation parts of addProduct.php, delProduct.php, listProduct.php and updateProduct.php respectively, and extract the connection data and query parts in each file into the database.class.php. After modification, it is as follows:
addProduct.php
if(isset($_POST['submit'])){
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
$query= "insert into product values(null,'$name','$price','$description')";
$db =newDB();
$db->connect();
$db->query($query);
$db->close();
}
delProduct.php:
include 'class/DB.class.php';
$id = $_GET['id'];
$query = "delete from product where id = '$id'";
$db = newDB();
$db->connect();
$db->query($query);
$db->close();
//At the same time jump to listProduct to display the results
header('location:listProduct.php');
listProduct.php:
//Connect database operation
include 'class/DB.class.php';
$query = "select * from product order by id desc";
$db = newDB();
$db->connect();
$db->query($query);
$data = array();
while($row = $db->fetch_assoc()){
$data[]= $row;
}
$db->close();
?>
/***********Refer to the product object for data transfer, that is, the data model*************************/
Idea: The operations of adding, deleting, modifying and checking belong to business logic and should be encapsulated.
Encapsulated into class Product.class.php
class Product {
private$id;
private$name;
private$price;
private$description;
public function__set($name,$value){
$this->$name= $value;
}
public function__get($name){
return$this->$name;
}
public functionadd(){
$query= "insert into product values(null,'$this->name','$this->price','$this->description')";
$db= newDB();
$db->connect();
$db->query($query);
$db->close();
}
}
Then introduce this class in addProduct.php and modify the operation database action:
$product= newProduct();
$product->name = $name;
$product->price = $price;
$product->description = $description;
$product->add();
Other methods of operating the database are similar.
Idea: By observing that addProduct.php, delProduct.php, listProduct.php, and updateProduct.php all have similar codes, can they be integrated together to complete the related requests of all modules?
Create a new product.php file, copy the relevant operation database codes in addProduct.php and delProduct.php to the product.php file, and use action parameters in product.php to distinguish different requests.
//Request for completing all related product operations
include 'class/DB.class.php';
include 'class/Product.class.php';
$action = $_GET['action'];
if($action =='add'){
//Add
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
$product= newProduct();
$product->name = $name;
$product->price = $price;
$product->description = $description;
$product->add();
}
if($action =='del'){
//Delete
$id= $_GET['id'];
$product= newProduct();
$product->id = $id;
$product->del();
// Jump to listProduct to display the results at the same time
header('location:listProduct.php');
}
At this time, you can delete the php code part in addProduct.php and separate the html part into an addProduct.html file,
At the same time, you can also delete the delProduct.php file, because the php code of these two php files has been moved to product.php.
/********************Join Smarty simulation class library****************************** ***/
Complete the above two functions first,
1. Access the addProduct.html file, complete the addition operation, and jump back to the addProduct.html page. Visit the listProduct.php file to view the added results.
2. Access the listProduct.php file and modify the request point for deleting the link. And complete the deletion function at the same time.
The code of product.php at this time is as follows:
//Request for completing all related product operations
include 'class/DB.class.php';
include 'class/Product.class.php';
$action = $_GET['action'];
if($action =='add'){
//Add
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
$product= newProduct();
$product->name = $name;
$product->price = $price;
$product->description = $description;
$product->add();
header('location:addProduct.html');
}
if($action =='del'){
//Delete
$id= $_GET['id'];
$product= newProduct();
$product->id = $id;
$product->del();
// Jump to listProduct to display the results at the same time
header('location:listProduct.php');
}
/**************Add smarty class library*************************/
Idea: At this time, the add and delete functions have been integrated into product.php, as well as query and update operations. Since listProduct.php contains the function of displaying a data list, it is not easy to display (you can use include one page to complete.). At this point, it can be done with smarty, which is reasonable.
At this time, you can consider putting addProduct.html into product.php for display, and modify it to:
include 'class/Template.class.php';//Pay attention to importing this file, which is a real class file that simulates smarty. (Considering that the query function is also used and multiple data needs to be displayed, the class file that simulates Smarty is not suitable, so smarty is directly introduced as a display)
/***********************Join smarty class library*************************/
At this time, you can inherit the Templates class you write from the Smarty class, so that you can use the functions in Smarty and extend its functions.
Template.class.php content is as follows:
/**
* Template class that inherits Smarty class
* @author heyongjia
*
*/
class TemplateextendsSmarty{
/**
* Call the constructor method of the parent class to ensure that the constructor method in the parent class has been executed
* Because if the subclass has a constructor, the constructor in the parent class will not be executed
*/
function__construct(){
parent::__construct();
//Initialize template directory
$this->setTemplateDir('tpl');
}
}
At this point, the CRUD function has been integrated into a product.php file, which means that all requests pass through it, such as:
Localhost/test/mvc/product.php?action=add/list/update and other requests. At this time product.php is equivalent to the role of a controller.
/**************** Add entry file *************************** ****、
In our program, there will be many controllers. In order to facilitate management, we define an entry file. That is to say, all requests go through this entry file and are then distributed to each controller through this entry.
Add an index.php file:
For distribution to controller:
Requested url:
Localhost/test/mvc/index.php?module=product&action=add/list/update
So the index.php file is as follows:
$module = $_GET['module'];
$action = $_GET['action'];
$file = $module.'Control.php?action='.$action;
header('location:'.$file);
At this point, the above entry file can be used to distribute to different operations (functions) on different controllers (modules). However, since header jump is used, the address bar on the browser becomes The address after the jump is gone, that is, it is out of the entry file.
So, how to achieve it?
Change product.php into a class to implement. Transform product.php to ProductControl.class.php and move it to the control folder. The content is as follows:
class ProductControl{
public functionaddok(){
//Add
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
$product= newProduct();
$product->name = $name;
$product->price = $price;
$product->description = $description;
$product->add();
header('location:product.php?action=list');
}
public functionadd(){
$smarty= newTemplate();
//$tpl = new Template();
//$tpl->display('addProduct.html');
$smarty->display('addProduct.html');
}
public functiondel(){
//Delete
$id= $_GET['id'];
$product= newProduct();
$product->id = $id;
$product->del();
//同时跳到listProduct展示结果
header('location:product.php?action=list');
}
public functionupdate(){
$smarty= newTemplate();
$id= $_GET['id'];
$product= newProduct();
$product->id = $id;
$row= $product->getRow();
$smarty->assign('id',$row['id']);
$smarty->assign('name',$row['name']);
$smarty->assign('price',$row['price']);
$smarty->assign('description',$row['description']);
$smarty->display('updateProduct.html');
}
public functionupdateok(){
$id= $_POST['id'];
$name= $_POST['name'];
$price= $_POST['price'];
$description= $_POST['description'];
$product= newProduct();
$product->id = $id;
$product->name = $name;
$product->price = $price;
$product->description = $description;
$product->update();
header('location:product.php?action=list');
}
}
此时,如何调用这个类的方法呢?在index.php中实例化该对象调用。
//用于完成所有相关product操作的请求
include 'class/DB.class.php';
include 'class/Product.class.php';
include 'smarty/Smarty.class.php';
include 'class/Template.class.php';
$module = $_GET['module'];
$module = ucfirst($module);
$action = $_GET['action'];
$className = $module.'Control';
include 'control/'.$className.'.class.php';
$class = new$className; //根据模块名引用类文件并实例化该对象
$class->$action(); //调用对应的action方法。
修改调用的所有路径为:index.php?module=product&action=xxx
addProduct.html updateProduct.htmllistProduct.html
优化:一般地不会在index.php文件中写太多的代码,可以将代码移动配置文件中,在index.php中引入即可。
一般地,整个工程会有一个主控制器类,用于处理分发控制器,Application.class.php:
class Application{
static functionrun(){
global$module; //函数内要使用外部的变量
global$action;
$className= $module.'Control';
include'control/'.$className.'.class.php';
$class= new$className;
$class->$action();
}
}
init.php:
//用于完成所有相关product操作的请求
include 'class/DB.class.php';
include 'class/Product.class.php';
include 'smarty/Smarty.class.php';
include 'class/Template.class.php';
include 'control/Application.class.php';
$module = $_GET['module'];
$module = ucfirst($module);
$action = $_GET['action'];
Index.php:
include 'config/init.php';
Application::run();
At this time, the visited url is: index.php?module=product&action=xxx
But if you access index.php directly, an error will be reported:
Notice: Undefined index: module in F:ampapachestudydocstestphp_jsmvcconfiginit.phpon line10
Notice: Undefined index: action in F:ampapachestudydocstestphp_jsmvcconfiginit.phpon line12
This is because the module and action are not initialized in init.php.
And configure the value into the conig.php file under the config folder.
Since there are many similar codes for operating the database in the product.php file (actually the model class), this part of the code can be encapsulated into the parent class implementation, and the model class only needs to be inherited.
/**************** encapsulates the core file core********************** **
Create a new folder core, and move DB.class.php and Application.php to core because they are public files and are not functional files.
Create a new base class file (model base class) to encapsulate database instantiation, while subclass models only need to inherit to obtain instances of database objects.
Model.class.php:
class Medel{
protected$db;
public function__construct(){
$this->db =newDB();
}
}
Create a new folder model, then move product.php to the model folder and rename it ProductModel.class.php:
class ProductModelextendsModel {
private$id;
private$name;
private$price;
private$description;
public function__set($name,$value){
$this->$name= $value;
}
public function__get($name){
return$this->$name;
}
public functionadd(){
$query= "insert into product values(null,'$this->name','$this->price','$this->description')";
$this->db->connect();
$this->db->query($query);
$this->db->close();
}
public functiondel(){
$query= "delete from product where id = '$this->id'";
$this->db->connect();
$this->db->query($query);
$this->db->close();
}
public functionselect(){
$query= "select * from product order by iddesc";
$this->db->connect();
$this->db->query($query);
$data= array();
while($row = $this->db->fetch_assoc()){
$data[]= $row;
}
$this->db->close();
return$data;
}
public functionupdate(){
$query= "update product set name = '$this->name', price = '$this->price' , description = '$this->description' where id='$this-> ;id'";
$this->db->connect();
$this->db->query($query);
$this->db->close();
}
public functiongetRow(){
$query= "select * from product where id = '$this->id'";
$this->db->connect();
$this->db->query($query);
$row= $this->db->fetch_assoc();
$this->db->close();
return$row;
}
}
/***********************View Encapsulation******************************
The Template class must be instantiated every time in the controller and the display method must be called.
Move Template.class.php to the core folder and rename it View.class.php:
The advantage of doing this is that each control inherits from this class (construct a controller base class Control.class.php, and construct a view instance in the class), as long as each controller inherits this class (Control. class.php), that is, you have a smarty instance.
/**
* 1. The Template class inherits the Smarty class
* 2. There is no need to inherit Smarty, it is an instance of smarty
* @author heyongjia
*
*/
class Viewextends Smarty{
}
The advantage of doing this is that each control inherits from this class (construct a controller base class Control.class.php, and construct a view instance in the class), as long as each controller inherits this class (Control. class.php), that is, you have a smarty instance.
/**
* Controller base class
* @author heyongjia
*
*/
class Controlextends View{
protected$view;
public function__construct(){
$this->view = new View();
$this->view->setTemplateDir('tpl');
}
}
Optimization, it is wrong to include models directly in init.php, because many models can appear. At this time, you can consider automatic loading onboard to achieve this. Note: The automatic loading function is also used in smarty3.0, so registration must be used to complete it.
function autoload1($classname){
$filename= 'model/'.$classname.'.class.php';
if(is_file($filename)){
include"$filename";
}
}
spl_autoload_register('autoload1');
function addslashes_func(&$str){
$str= addslashes($str);
}
if(!get_magic_quotes_gpc()){
array_walk_recursive($_POST,'addslashes_func');
array_walk_recursive($_GET,'addslashes_func');
}
At this point, the simple MVC framework is almost here.
Let’s summarize it with a picture, so it’s more intuitive:
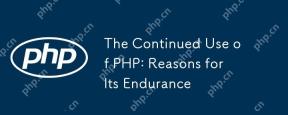
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
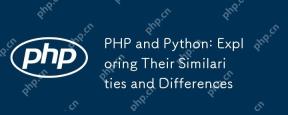
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
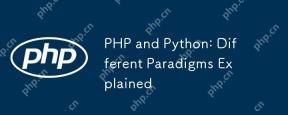
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
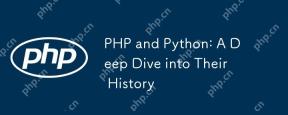
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
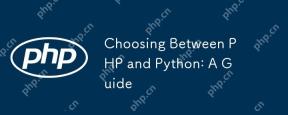
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
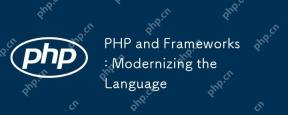
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
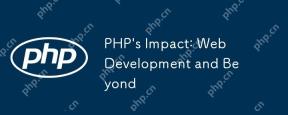
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
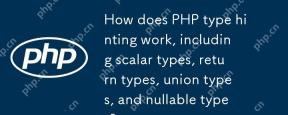
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
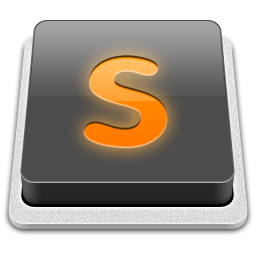
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.