PHP batch generate static html_PHP tutorial
As we all know, most websites’ news information or product information are static pages. The main benefits of doing this are: 1. Speeding up access and avoiding excessive database operations; 2. SEO optimization to facilitate search engine inclusion.
This example is based on the static page solution of the CMS system and demonstrates the function of batch generating static html.
Note: The program in this article can only be run by executing PHP commands under Windows, DOS or Linux.
This example mainly has 4 files: config.inc.php (configuration file), Db.class.php (database PDO class), Model.class.php (PDO database operation class), index.php (execution file )
config.inc.php
view plaincopy to clipboardprint?
header('Content-Type:text/html;Charset=utf-8');
date_default_timezone_set('PRC');
define('ROOT_PATH', dirname(__FILE__)); // Root directory
define('DB_DSN', 'mysql:host=localhost;dbname=article'); // MySQL's PDO dsn
define('DB_USER', 'root'); // Database user name
define('DB_PWD', '1715544'); // Database password (please set it according to the actual situation)
function __autoload($className) {
require_once ROOT_PATH . '/includes/'. ucfirst($className) .'.class.php';
}
?>
header('Content-Type:text/html;Charset=utf-8');
date_default_timezone_set('PRC');
define('ROOT_PATH', dirname(__FILE__)); // Root directory
define('DB_DSN', 'mysql:host=localhost;dbname=article'); // MySQL's PDO dsn
define('DB_USER', 'root'); // Database user name
define('DB_PWD', '1715544'); // Database password (please set it according to the actual situation)
function __autoload($className) {
require_once ROOT_PATH . '/includes/'. ucfirst($className) .'.class.php';
}
?>
Db.class.php
view plaincopy to clipboardprint?
// Connect to database
class Db {
static public function getDB() {
try {
$pdo = new PDO(DB_DSN, DB_USER, DB_PWD);
$pdo->setAttribute(PDO::ATTR_PERSISTENT, true); // Set the database connection to a persistent connection
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Setup throws an error
$pdo->setAttribute(PDO::ATTR_ORACLE_NULLS, true); // Set when the string is empty, it is converted to NULL in SQL
$pdo->query('SET NAMES utf8'); // Set database encoding
} catch (PDOException $e) {
exit('Database connection error, error message:'. $e->getMessage());
}
return $pdo;
}
}
?>
//Connect to database
class Db {
static public function getDB() {
try {
$pdo = new PDO(DB_DSN, DB_USER, DB_PWD);
$pdo->setAttribute(PDO::ATTR_PERSISTENT, true); //Set the database connection as a persistent connection
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Set throw error
$pdo->setAttribute(PDO::ATTR_ORACLE_NULLS, true); //Set when the string is empty, it is converted to SQL NULL
$pdo->query('SET NAMES utf8'); // Set database encoding
} catch (PDOException $e) {
exit('Database connection error, error message:'. $e->getMessage());
}
return $pdo;
}
}
?>
Model.class.php
view plaincopy to clipboardprint?
// 操作 SQL
class Model {
/**
* SQL addition, deletion and modification operations, return the number of affected rows
* @param string $sql
* @return int
*/
public function aud($sql) {
try {
$pdo = Db::getDB();
$row = $pdo->exec($sql);
} catch (PDOException $e) {
exit($e->getMessage());
}
return $row;
}
/**
* Return all data, return PDOStatement object
* @param string $sql
* @return PDOStatement
*/
public function getAll($sql) {
try {
$pdo = Db::getDB();
$result = $pdo->query($sql);
return $result;
} catch (PDOException $e) {
exit($e->getMessage());
}
}
}
?>
// 操作 SQL
class Model {
/**
* SQL addition, deletion and modification operations, return the number of affected rows
* @param string $sql
* @return int
*/
public function aud($sql) {
try {
$pdo = Db::getDB();
$row = $pdo->exec($sql);
} catch (PDOException $e) {
exit($e->getMessage());
}
return $row;
}
/**
* Return all data and return PDOStatement object
* @param string $sql
* @return PDOStatement
*/
public function getAll($sql) {
try {
$pdo = Db::getDB();
$result = $pdo->query($sql);
return $result;
} catch (PDOException $e) {
exit($e->getMessage());
}
}
}
?>
index.php
view plaincopy to clipboardprint?
require_once './config.inc.php';
$m = new Model();
$ids = $m->getAll("SELECT id FROM article ORDER BY id ASC");
foreach ($ids as $rowIdArr) {
$idStr .= $rowIdArr['id'].',';
}
$idStr = rtrim($idStr, ','); // Collection of ID numbers of all articles
$idArr = explode(',', $idStr); // Split into arrays
// The following program loops to generate static pages
foreach ($idArr as $articleId) {
$re = $m->getAll("SELECT id,title,date,author,source,content FROM article WHERE id =". $articleId); // $re is the content of each article. Note: its type is :PDOStatement
$article = array(); // $article is an array, saving the title, date, author, content, source of each article
foreach ($re as $r) {
$article = array(
'title'=>$r['title'],
‘date’=>$r['date'],
‘author’=>$r['author'],
'source'=>$r['source'],
'content'=>$r['content']
);
}
$articlePath = ROOT_PATH. '/article'; // $articlePath is the directory where static pages are placed
If (!is_dir($articlePath)) mkdir($articlePath, 0777); // Check whether the directory exists, create it if it does not exist
$fileName = ROOT_PATH . '/article/' . $articleId . '.html'; // Static file name generated by $fileName, format: article ID.html (primary key ID cannot conflict)
$articleTemPath = ROOT_PATH . '/templates/article.html'; // $articleTemPath article template path
$articleContent = file_get_contents($articleTemPath); // Get the content in the template
//Replace the variables set in the template. That is, for example: replace in the template with the title read from the database, and then assign the value to the variable $articleContent
$articleContent = getArticle(array_keys($article), $articleContent, $article);
$resource = fopen($fileName, 'w');
File_put_contents($fileName, $articleContent); // Write HTML file
}
/**
* getArticle($arr, $content, $article) replaces the template
* @param array $arr replacement variable array
* @param string $content template content
* @param array $article Array of content of each article, format: array('title'=>xx, 'date'=>xx, 'author'=>xx, 'source'=>xx, 'content '=>xx);
*/
function getArticle($arr, $content, $article) {
// Loop replacement
foreach ($arr as $item) {
$content = str_replace('', $article[$item], $content);
}
Return $content;
}
?>
require_once './config.inc.php';
$m = new Model();
$ids = $m->getAll("SELECT id FROM article ORDER BY id ASC");
foreach ($ids as $rowIdArr) {
$idStr .= $rowIdArr['id'].',';
}
$idStr = rtrim($idStr, ','); // Collection of ID numbers of all articles
$idArr = explode(',', $idStr); // Split into arrays
//The following program loops to generate static pages
foreach ($idArr as $articleId) {
$re = $m->getAll("SELECT id,title,date,author,source,content FROM article WHERE id =". $articleId); // $re is the content of each article. Note: its type is :PDOStatement
$article = array(); // $article is an array, saving the title, date, author, content, source of each article
foreach ($re as $r) {
$article = array(
'title'=>$r['title'],
'date'=>$r['date'],
'author'=>$r['author'],
'source'=>$r['source'],
'content'=>$r['content']
);
}
$articlePath = ROOT_PATH. '/article'; // $articlePath is the directory where static pages are placed
if (!is_dir($articlePath)) mkdir($articlePath, 0777); // Check whether the directory exists, create it if it does not exist
$fileName = ROOT_PATH . '/article/' . $articleId . '.html'; // Static file name generated by $fileName, format: article ID.html (primary key ID cannot conflict)
$articleTemPath = ROOT_PATH . '/templates/article.html'; // $articleTemPath article template path
$articleContent = file_get_contents($articleTemPath); // Get the content in the template
//Replace the variables set in the template. That is, for example: replace in the template with the title read from the database, and assign the value to the variable $articleContent
after the replacement.
$articleContent = getArticle(array_keys($article), $articleContent, $article);
$resource = fopen($fileName, 'w');
file_put_contents($fileName, $articleContent); //Write HTML file
}
/**
* getArticle($arr, $content, $article) replaces the template
* @param array $arr replacement variable array
* @param string $content template content
* @param array $article Array of content of each article, format: array('title'=>xx, 'date'=>xx, 'author'=>xx, 'source'=>xx, 'content '=>xx);
*/
function getArticle($arr, $content, $article) {
// Loop replacement
foreach ($arr as $item) {
$content = str_replace('', $article[$item], $content);
}
return $content;
}
?>
Run the screenshot (Windows DOS as an example)
Screenshot after running:
It takes about 2 minutes to generate more than 9,000 html.
Excerpted from Lee.’s column
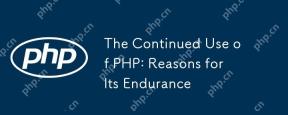
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
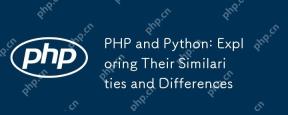
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
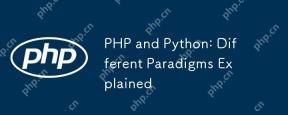
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
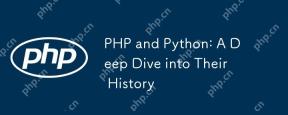
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
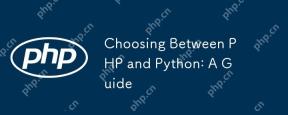
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
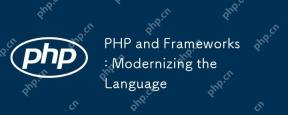
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
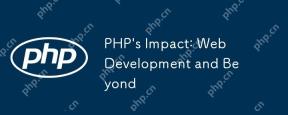
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
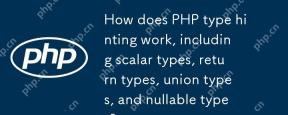
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
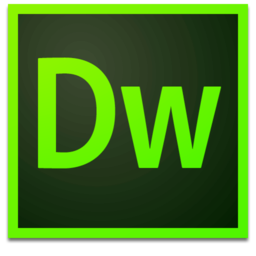
Dreamweaver Mac version
Visual web development tools
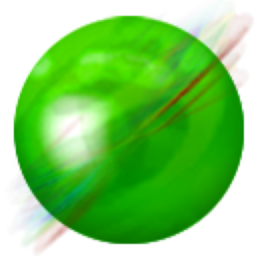
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
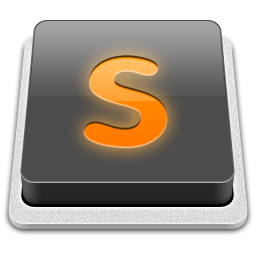
SublimeText3 Mac version
God-level code editing software (SublimeText3)