


Detailed explanation of PHP5 PDO connection to database_PHP tutorial
This article introduces PHP5 PDO to connect to the database
1. Introduction to PDO
PDO (PHP Data Object) is something added in PHP 5. It is a major new feature added in PHP 5, because in PHP 5 In the past, php4/php3 had a bunch of database extensions to connect and process each database, such as php_mysql.dll, php_pgsql.dll, php_mssql.dll, php_sqlite.dll, etc.
PHP6 will also use PDO to connect by default, and the mysql extension will be used as an auxiliary
2. PDO configuration
In PHP.ini, remove "extension=php_pdo. dll". If you want to connect to the database, you also need to remove the ";" sign in front of the database extension related to PDO, and then restart the Apache server.
extension=php_pdo.dll
extension=php_pdo_mysql.dll
extension=php_pdo_pgsql.dll
extension=php_pdo_sqlite.dll
extension=php_pdo_mssql.dll
extension=php_pdo_odbc.dll
extension=php_pdo_firebird.dll
......
3. PDO connects to mysql database
new PDO("mysql:host=localhost;dbname=db_demo" ,"root","");
The default is not a long connection. If you want to use a long connection to the database, you need to add the following parameters at the end:
new PDO("mysql:host=localhost;dbname=db_demo"," root","","array(PDO::ATTR_PERSISTENT => true) ");
4. Common PDO methods and their applications
PDO::query() Mainly used for operations that return recorded results, especially SELECT operations
PDO::exec() Mainly used for operations that do not return a result set, such as INSERT, UPDATE and other operations
PDO::lastInsertId() returns In the last insertion operation, the primary key column type is the last auto-incremented ID
PDOStatement::fetch() is used to get a record
PDOStatement::fetchAll() is used to get all the record sets into one
5. PDO operates MYSQL database instance
$pdo = new PDO("mysql:host=localhost;dbname=db_demo","root", "");
if($pdo -> exec("insert into db_demo(name,content) values(title,content)")){
echo "Insertion successful!";
echo $ pdo -> lastinsertid();
}
?>
$pdo = new PDO("mysql:host=localhost;dbname=db_demo","root", "");
$rs = $pdo -> query("select * from test");
while($row = $rs -> fetch()){
print_r($row );
}
?>
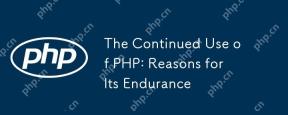
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
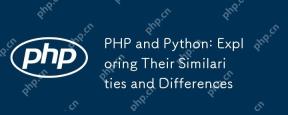
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
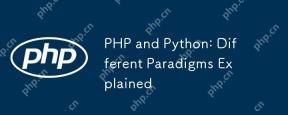
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
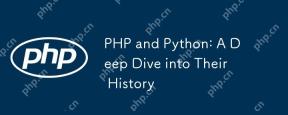
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
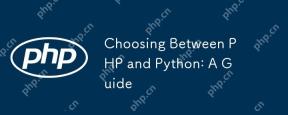
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
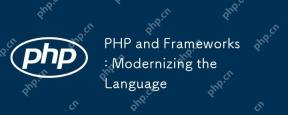
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
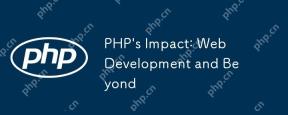
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
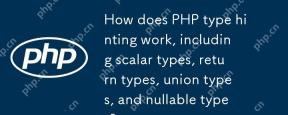
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
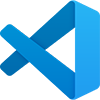
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
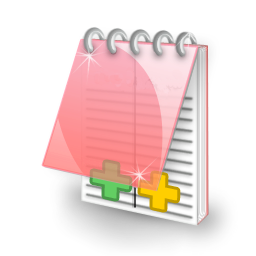
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use