


PHP implementation of memcache caching example explanation_PHP tutorial
Overview
Shared memory is an efficient way to exchange data between applications on the same machine. A process can create a memory segment that can be accessed by other processes, as long as it is assigned the correct permissions. Each memory segment has a unique ID (called a shmid) that points to an area of physical memory where other processes can operate on it. Once created and given the appropriate permissions, other processes on the same machine can operate on these memory segments: read, write, and delete.
This means that applications written in C can share information with applications written in other languages, such as Java™ or PHP. They can all share information as long as they have access to and understanding of that information. Shared memory is widely used in implementations for most languages, so access should not be an issue. To understand the information, we can use a standard format such as XML or JSON.
The use of shared memory is a fast way to exchange data between processes, mainly because the data is passed after the memory segment is created, without involving the kernel. This method is often called inter-process communication (IPC). Other IPC methods include pipes, message queues, RPC, and sockets. This ability to quickly and reliably exchange data between applications is useful when working with an ecosystem of applications that need to communicate with each other. Depending on the size of the ecosystem, the common method of using a database to exchange information between applications can often result in slow queries or even I/O blocking. With shared memory, there is no I/O to slow down developer progress.
The proposal of this article is very simple, learn how to use PHP to create and manipulate shared memory segments and use them to store data sets that can be used by other applications. Even if there are no plans to use shared memory to exchange data, it has many benefits in itself because it enables applications to stay away from I/O problems. Storing datasets directly in memory has many advantages, from web service data caching to session sharing. It is a very useful concept that every PHP developer should know.
Shared Memory and PHP
PHP has a rich set of extensions available, as does shared memory. Using some shared functions, developers can easily manipulate memory segments without installing any extensions.
Create memory segment
Shared memory functions are similar to file operation functions, but instead of handling a stream, you will handle a shared memory access ID. The first example is the shmop_open function, which allows you to open an existing memory segment or create a new memory segment. This function is very similar to the classic fopen function, which opens a stream for file operations and returns a resource for use by other functions wishing to read from or write to the opened stream. Let's look at shmop_open in Listing 1.
Listing 1. shmop_open function
$systemid = 864; // System ID for the shared memory segment
$mode = "c"; // Access mode
$permissions = 0755; // Permissions for the shared memory segment
$size = 1024; // Size, in bytes, of the segment
$shmid = shmop_open($systemid, $mode, $permissions, $size);
?>
The first thing that appears in this function is the system ID parameter. This is a number that identifies the shared memory segment in the system. The second parameter is the access mode, which is very similar to the access mode of the fopen function. You can access a memory segment in 4 different modes:
• Mode "a", which allows you to access read-only memory segments
• Mode "w", which allows you to access read-write memory segments
• Mode "c", which creates a new memory segment, or if the memory segment already exists, try to open it for reading and writing
• Mode "n", it creates a new memory segment and fails if the memory segment already exists
The third parameter is Permissions for the memory segment. You must provide an octal value here.
The fourth parameter provides the memory segment size in bytes. Before writing to a memory segment, you must allocate the appropriate number of bytes above it.
Note that this function returns an ID number that can be used by other functions to operate on this shared memory segment. This ID is the shared memory access ID, unlike the system ID, which is passed as a parameter. Be careful not to confuse the two. On failure, shmop_open will return FALSE.
Write data to memory segment
Use the shmop_write function to write data to the shared memory block. This function is simple to use and accepts only 3 parameters, as shown in Listing 2.
Listing 2. Using shmop_write to write data to a shared memory block
$shmid = shmop_open(864, 'c', 0755, 1024);
shmop_write($shmid, "Hello World!", 0);
?>
This function is similar to the fwrite function, which takes two parameters: the open stream resource (returned by fopen) and the data you wish to write. The shmop_write function also performs this task.
The first parameter is the ID returned by shmop_open, which identifies the shared memory block you are operating on. The second parameter is the data you wish to store, and the final third parameter is the position where you wish to start writing. By default, we always use 0 to indicate where to start writing. Note that this function returns FALSE on failure and the number of bytes written on success.
Reading data from a memory segment
Reading data from a shared memory segment is simple. All you need is an open memory segment and the shmop_read function. This function accepts some parameters and works like fread. See Listing 3 to read the contents of a PHP file.
Listing 3. Using shmop_read to read the contents of a file
$stream = fopen('file.txt', 'r+');
fwrite($stream, "Hello World!");
echo fread($stream, 11);
?>
The process of reading the contents of a shared memory segment is similar, as shown in Listing 4:
Listing 4. Reading the contents of a shared memory segment
$shmid = shmop_open(864, 'c', 0755, 1024);
shmop_write($shmid, "Hello World!", 0);
echo shmop_read($shmid, 0, 11);
?>
Please pay attention to the parameters here. The shmop_read function will accept the ID returned by shmop_open, which we already know, but it also accepts two other parameters. The second parameter is the location in the memory segment you wish to read from, and the third is the number of bytes you wish to read. The second parameter can always be 0, indicating the beginning of the data, but the third parameter can be problematic because we don't know how many bytes we want to read.
This is very similar to what we do in the fread function, which accepts two parameters: the open stream resource (returned by fopen) and the number of bytes you wish to read from the stream. Use the filesize function (which returns the number of bytes in a file) to read it completely.
Fortunately, when using shared memory segments, the shmop_size function returns the size of a memory segment in bytes, similar to the filesize function. See Listing 5.
Listing 5. The shmop_size function returns the memory segment size in bytes
$shmid = shmop_open(864, 'c', 0755, 1024);
shmop_write($shmid, "Hello World!", 0);
$size = shmop_size($shmid);
echo shmop_read($shmid, 0, $size);
?>
Back to top
Deleting Memory Segments
We learned how to open, write and read shared memory segments. To complete our CRUD class, we also need to learn how to delete a memory segment. This task can be easily accomplished using the shmop_delete function, which accepts only one parameter: the ID of the shared memory we wish to delete.
Listing 6. shmop_delete marks the memory segment to be deleted
$shmid = shmop_open(864, 'c', 0755, 1024);
shmop_write($shmid, "Hello World!", 0);
shmop_delete($shmid);
?>
This does not actually delete the memory segment. It marks the memory segment for deletion because a shared memory segment cannot be deleted while another process is using it. The shmop_delete function marks the memory segment for deletion, preventing any other process from opening it. To delete it, we need to close that memory segment.
Close memory segment
Opening a shared memory segment will "attach" it. After attaching this memory segment, we can read and write to it, but when we are done, we must detach from it. This is done using the shmop_close function in Listing 7.
This is very similar to the fclose function when working with files. After opening a stream containing a file and reading or writing data in it, we must close it otherwise locking will occur.
Listing 7. Detaching a memory segment using shmop_close
$shmid = shmop_open(864, 'c', 0755, 1024);
shmop_write($shmid, "Hello World!", 0);
shmop_delete($shmid);
shmop_close( $shmid);
?>
Using Shared Memory as a Storage Option
With a basic knowledge of shared memory and basic CRUD operations on shared memory segments, it is time to apply this knowledge. We can use shared memory as a unique storage option that provides benefits such as fast read/write operations and process interoperability. For web applications this means:
• Cache storage (database queries, web service data, external data)
• Session storage
• Data exchange between applications
Before continuing, I would like to introduce a small library called SimpleSHM . SimpleSHM is a smaller abstraction layer for manipulating shared memory using PHP, enabling easy manipulation of memory segments in an object-oriented manner. This library helps create very concise code when writing small applications that use shared memory for storage. To learn about SimpleSHM, visit the GitHub page.
You can use 3 methods to process: read, write and delete. Simply instantiating an object from this class can control the open shared memory segment. Listing 8 shows the basic usage.
List 8. Basic usage of SimpleSHM
$memory = new SimpleSHM;
$memory->write('Sample');
echo $memory->read();
?>
Note that an ID is not passed for this class. If no ID is passed, it will randomly choose a number and open a new memory segment with that number. We can pass a number as a parameter to the constructor to open an existing memory segment, or create a memory segment with a specific ID, as shown in Listing 9.
Listing 9. Opening a specific memory segment
$new = new SimpleSHM(897);
$new->write('Sample');
echo $new->read();
?>
The magic method __destructor is responsible for calling shmop_close on that memory segment to unset the object to detach from that memory segment. We call this “SimpleSHM 101”. Now let's put this method to a more advanced use: using shared memory as storage. Storing a dataset requires serialization because arrays or objects cannot be stored in memory. Although JSON is used here for serialization, any other method (such as XML or the built-in PHP serialization functionality) will also suffice. Listing 10 gives an example.
Listing 10. Using shared memory as storage
require('SimpleSHM.class.php');
$results = array(
'user' => 'John',
'password' => '123456',
'posts' => array('My name is John ', 'My name is not John')
);
$data = json_encode($results);
$memory = new SimpleSHM;
$memory->write($data);
$storedarray = json_decode($memory->read());
print_r($storedarray);
?>
We successfully serialized an array into a JSON string, stored it in a shared memory block, read data from it, deserialized the JSON string, and displayed the stored array. This seems simple, but imagine the possibilities with this code snippet. You can use it to store results from web service requests, database queries, or even template engine caches. Reading and writing in memory will result in higher performance than reading and writing in disk.
Using this storage technology is not only useful for caching, but also for exchanging data between applications, as long as the data is stored in a format readable by both ends. Don't underestimate the power of shared memory in web applications. There are many different ways to cleverly implement this storage, the only limit is the creativity and skill of the developer

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
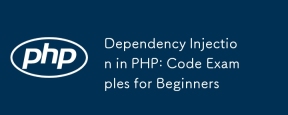
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
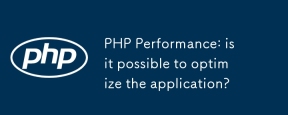
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
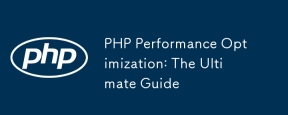
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
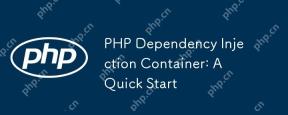
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
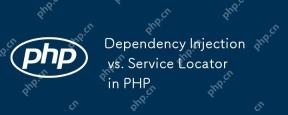
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
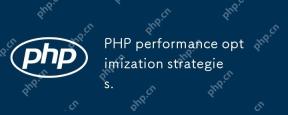
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
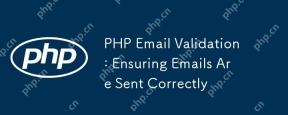
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
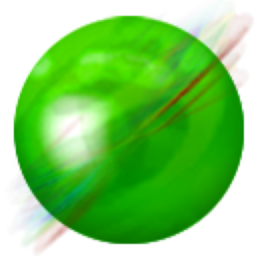
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
