PHP reads large file program code_PHP tutorial
There are some differences between reading large files in PHP and reading ordinary files. If your file reaches hundreds of MB or GB, ordinary PHP may be slow to read the file or get stuck. Let me introduce it below. PHP reading large file tips.
Generally, we use fopen or file_get_contents to read files. The former can be read in a loop, and the latter can be read in one go, but they both load the file contents at once. If the loaded file is particularly large, such as several hundred M, and the performance drops when it reaches G size, is there any function or class for processing large files in PHP? The answer is: yes.
PHP is really becoming more and more "object-oriented", and some of the original basic SPL methods have begun to implement classes one after another.
Starting from PHP 5.1.0, the SPL library has added two standard file operation classes: SplFileObject and SplFileInfo. SplFileInfo is implemented starting with PHP 5.1.2.
From the literal meaning, it can be seen that SplFileObject is more powerful than SplFileInfo.
Yes, SplFileInfo is only used to obtain some attribute information of the file, such as file size, file access time, file modification time, suffix name and other values, and SplFileObject inherits these functions of SplFileInfo.
The code is as follows | Copy code | ||||
* @param string $filename file name * @param int $startLine The starting line number * @param int $endLine Ending line number * @return string */ function getFileLines($filename, $startLine = 1, $endLine=50, $method='rb') { $content = array(); $count = $endLine - $startLine; // Determine the php version (because SplFileObject is used, PHP>=5.1.0) If(version_compare(PHP_VERSION, '5.1.0', '>=')){ $fp = new SplFileObject($filename, $method); $fp->seek($startLine-1);//Go to line N, the seek method parameters start counting from 0 for($i = 0; $i $content[]=$fp->current();// current() gets the current row content $fp->next();//Next line } }else{//PHP $fp = fopen($filename, $method); If(!$fp) return 'error:can not read file'; for ($i=1;$i fgets($fp); } for($i;$i $content[]=fgets($fp);//Read file line content } fclose($fp); } Return array_filter($content); //array_filter filter: false,null,'' } |
Ps: There is no "read to the end judgment" added above: !$fp->eof() or !feof($fp). In addition, this judgment affects efficiency. I have to add many, many, many lines of tests myself. The running time is already known, and it is completely unnecessary to add it here.
It can be seen from the above function that using SplFileObject is much faster than fgets below, especially when the number of file lines is very large and the following content needs to be fetched. fgets requires two loops, and it needs to loop $endLine times.
This method took a lot of effort and tested many Chinese writing methods, just to find the most efficient method. If anyone thinks there is anything worth improving, please let me know.
Used, return the content of lines 35270-35280:
The code is as follows
|
Copy code
|
||||
';<br> var_dump(getFileLines('test.php',35270,35280));<br> echo '';
|
The code is as follows
|
Copy code |
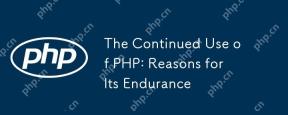
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
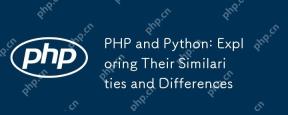
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
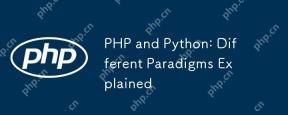
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
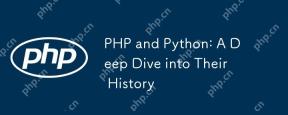
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
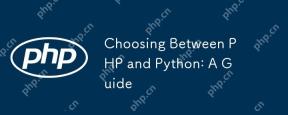
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
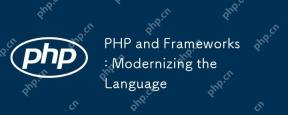
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
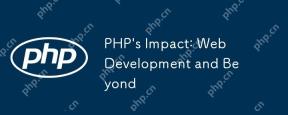
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
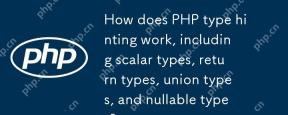
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
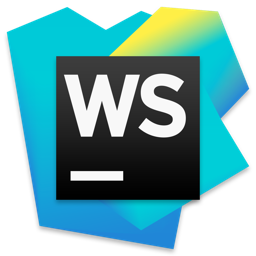
WebStorm Mac version
Useful JavaScript development tools
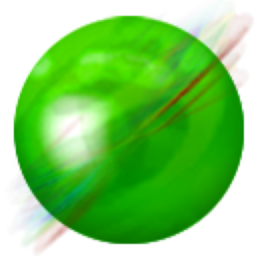
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.