


Detailed explanation of usage examples of php ZipArchive class_PHP tutorial
php ZipArchive can be said to be a function that comes with PHP. It can compress and decompress files. However, before using this class, we must change the semicolon in front of extension=php_zip.dll in php.ini. Have you removed it and then restarted Apache so that you can use this class library?
ziparchive optional parameter
1.ZipArchive::addEmptyDir
Add a new file directory
2.ZipArchive::addFile
Add files to the specified zip archive.
3.ZipArchive::addFromString
The content of the added files will be added at the same time
4.ZipArchive::close
Close ziparchive
5.ZipArchive::extractTo
Extract the compressed package
6.ZipArchive::open
Open a zip archive
7.ZipArchive::getStatusString
Returns the status content during compression, including error information, compression information, etc.
8.ZipArchive::deleteIndex
Delete a certain file in the compressed package, such as: deleteIndex(0) deletes the first file
9.ZipArchive::deleteName
Delete a certain file name in the compressed package and also delete the file.
......
*/
Example
1. Unzip the zip file
The code is as follows | Copy code | ||||||||||||
$zip = new ZipArchive;//Create a new one ZipArchive object
Processing zip files through ZipArchive objects The parameter of the $zip->open method indicates the name of the zip file to be processed.
*/ if ($zip->open('test.zip') === TRUE)
|
The code is as follows | Copy code |
$zip = new ZipArchive; /* The first parameter of the $zip->open method indicates the name of the zip file to be processed. The second parameter indicates the processing mode. ZipArchive::OVERWRITE indicates that if the zip file exists, the original zip file will be overwritten. If the parameter uses ZIPARCHIVE::CREATE, the system will add content to the original zip file. If you are not adding content to the zip file multiple times, it is recommended to use ZipArchive::OVERWRITE. Using these two parameters, if the zip file does not exist, the system will automatically create it. If the operation on the zip file object is successful, the $zip->open method will return TRUE */ if ($zip->open('test.zip', ZipArchive::OVERWRITE) === TRUE) { $zip->addFile('image.txt');//Assume that the added file name is image.txt, in the current path $zip->close(); } |
The code is as follows | Copy code |
$zip = new ZipArchive; $res = $zip->open('test.zip', ZipArchive::CREATE); if ($res === TRUE) { $zip->addFromString('test.txt', 'file content goes here'); $zip->close(); echo 'ok'; } else { echo 'failed'; } |
4. Pack the folder into a zip file
The code is as follows
|
Copy code
|
||||||||
function addFileToZip($path, $zip) {
/*
|
Be sure to use !==, because if a file name is called '0', or something is considered by the system to represent false, using != will stop the loop
if (is_dir($path . "/" . $filename)) {// If an object read is a folder, recurse
}The code is as follows | Copy code | ||||
$zip = new ZipArchive;
if ($zip->open('test.zip') === TRUE) {
$index=$zip->locateName('example.php', ZIPARCHIVE::FL_NOCASE|ZIPARCHIVE::FL_NODIR);
$contents = $zip->getFromIndex($index);
}
?>
The index obtained above relies on the locateName method. If there are files with the same name in multiple paths in the compressed package, it seems that only the index of the first one can be returned. If you want to get the index of all files with the same name, you can only use a stupid method and search in a loop.
|
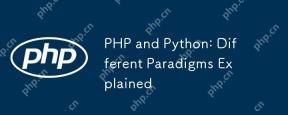
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
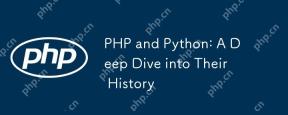
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
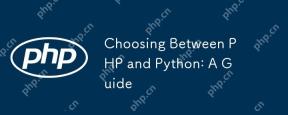
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
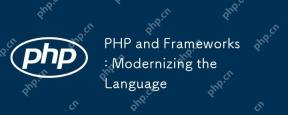
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
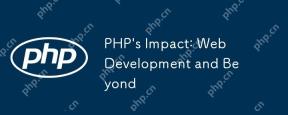
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
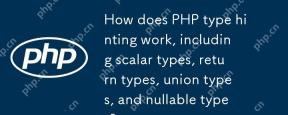
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
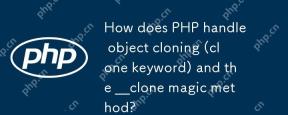
In PHP, use the clone keyword to create a copy of the object and customize the cloning behavior through the \_\_clone magic method. 1. Use the clone keyword to make a shallow copy, cloning the object's properties but not the object's properties. 2. The \_\_clone method can deeply copy nested objects to avoid shallow copying problems. 3. Pay attention to avoid circular references and performance problems in cloning, and optimize cloning operations to improve efficiency.
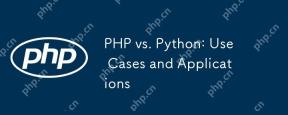
PHP is suitable for web development and content management systems, and Python is suitable for data science, machine learning and automation scripts. 1.PHP performs well in building fast and scalable websites and applications and is commonly used in CMS such as WordPress. 2. Python has performed outstandingly in the fields of data science and machine learning, with rich libraries such as NumPy and TensorFlow.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
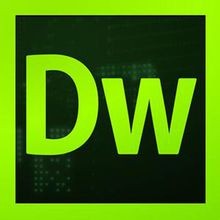
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor

Zend Studio 13.0.1
Powerful PHP integrated development environment
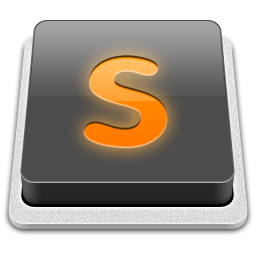
SublimeText3 Mac version
God-level code editing software (SublimeText3)

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software