


/**
* ecshop jpgraph chart library
* ================================================= =============
* Use the open source jpgraph library to display various types of data in the form of charts and trend charts.
* ================================================= =============
**/class cls_jpgraph
{
var $db = null;
var $ydata = array();
var $width = 350;
var $height = 250;var $graph = ''; //Graphic object
var $piegraph = ''; //Piegraph graphvar $lineplot = ''; //Linear object
var $barpot = ''; //Bar object
var $gbplat = ''; //Column group object
var $txt = ''; //Text object
var $p1 = ''; //Program objectvar $scale = ''; //Chart type? (textlin, textlog, intlin)
var $yscale = ''; // (log,)
var $xgrid = false; //x-axis grid display
var $ygrid = false; //Y-axis grid display
var $title = ''; //Title
var $subtitle = ''; //Subtitle (usually date)
var $xaxis = ''; //x-axis name
var $yaxis = ''; //y-axis name/*margin position*/
var $left = 0;
var $right = 0;
var $top = 0;
var $bottom = 0;/**
*Constructor
*/
function cls_jpgraph($width=350,$height=250)
{
$this->width = $width;
$this->height = $height;$this->graph = new graph($this->width,$this->height);
}
/**
*Constructor of chart class library
*
*/
/*
function __construct($parms,$width,$height)
{
cls_jpgraph($parms,$width,$height);
}
*//*Basic picture information settings*/
function set_jpgraph($scale='intlin',$title='',$subtitle='',$bgcolor='',$xaxis='',
$yaxis='',$xgrid=false,$ygrid=false,$margin='') {$this->scale = $scale;
$this->title = $title;
$this->subtitle = $subtitle;
$this->xaxis = $xaxis;
$this->yaxis = $yaxis;
$this->xgrid = $xgrid;
$this->ygrid = $ygrid;if(!empty($scale)) {
$this->graph->setscale($this->scale);
}
else {
$this->graph->setscale('intlin');
}
$this->graph->xgrid->show($this->xgrid,$this->xgrid);
$this->graph->ygrid->show($this->ygrid,$this->ygrid);if(!empty($bgcolor)) {
$this->graph->setmargincolor($bgcolor);
}/*If the image position is set manually*/
if(is_array($margin)) {
while(list($key,$val) = each($margin)) {
$this->$key = $val;
}
$this->graph->setmargin($this->left,$this->right,$this->top,$this->bottom);
}if(!empty($this->title)) {
$this->graph->title->set($this->title);
}
if(!empty($this->subtitle)) {
$this->graph->subtitle->set($this->subtitle);
}
else {
$this->graph->subtitle->set('('.date('y-m-d').')'); //The default subtitle is set to the current date
}
if(!empty($this->xaxis)) {
$this->graph->xaxis->title->set($this->xaxis);
}
if(!empty($this->yaxis)) {
$this->graph->yaxis->title->set($this->yaxis);
}}
/*创建线性关系图表(linear plot)*/
function create_lineplot($parms,$color='black',$weight=1)
{
$this->ydata = $parms;
$this->lineplot = new lineplot($this->ydata);
$this->lineplot->setcolor($color);
$this->lineplot->setweight($weight);return $this->lineplot;
}/*创建柱状图表(bar pot)*/
function create_barpot($parms,$color='black',$width='0')
{
$this->ydata = $parms;
$this->barpot = new barplot($this->ydata);
$this->barpot->setfillcolor($color);
if(!empty($width)) {
$this->barpot->setwidth($width);
}return $this->barpot;
}/*创建数据柱状图表组*/
function create_bargroup($plotarr,$width='0.8')
{
$this->gbplot = new groupbarplot($plotarr);
$this->gbplot->setwidth($width);return $this->gbplot;
}/*创建文本内容*/
function create_text($str,$postion='',$color='black')
{
$this->txt = new text($str);if(is_array($postion)) {
while(list($key,$val) = each($postion)) {
$this->$key = $val;
}
$this->txt->setpos($this->left,$this->top);
}
else {
$this->txt->setpos(10,20);
}
$this->txt->setcolor($color);$this->graph->add($this->txt);
}/*创建丙状图表*/
function create_pie($parms,$title,$type='3d',$size='0.5',$center='0.5',$width='350',$height='250')
{
$this->width = $width;
$this->height = $height;$this->piegraph = new piegraph(300,200);
$this->piegraph->setshadow();$this->piegraph->title->set($title);
if('3d' != $type) {
$this->p1 = new pieplot($parms);
$this->piegraph->add($this->p1);
}
else {
$this->p1 = new pieplot3d($parms);
$this->p1->setsize($size);
$this->p1->setcenter($center);
// $this->p1->setlegends($gdatelocale->getshortmonth());
$this->piegraph->add($this->p1);
}
$this->piegraph->stroke();
}function get_auth_code($length=4)
{
$spam = new antispam();
$chars = $spam->rand($length);if( $spam->stroke() === false ) {
return false;
}return $chars;
}/*完成图形创建并显示*/
function display($obj)
{
$this->graph->add($obj);
$this->graph->stroke();
}
}?>
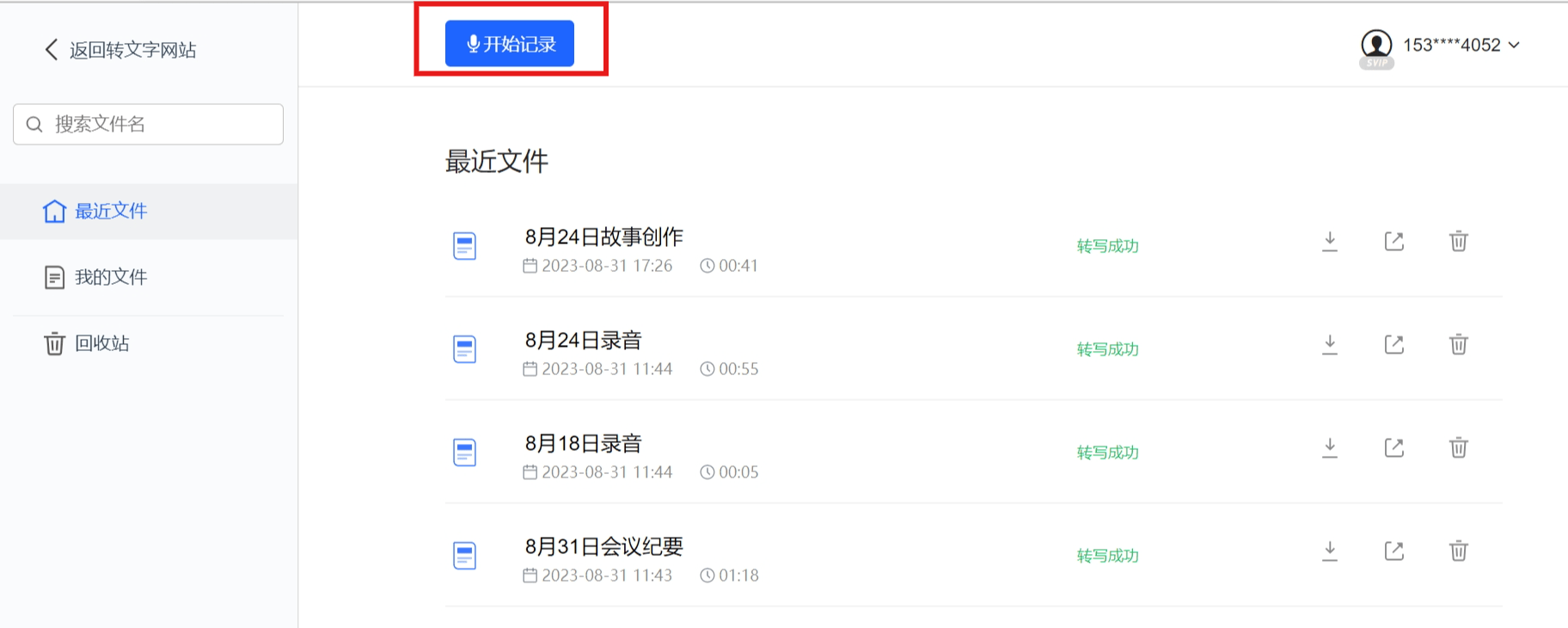
讯飞听见升级会议纪要功能,可以将口语表述直接转化为书面稿,AI能够根据录音总结会议纪要。AI能够帮助您完成会议纪要的撰写工作8月31日,讯飞听见网页端进行了版本升级,新增了PC端实时录音功能,能够利用人工智能智能生成会议纪要。这一功能的推出将大大提高用户在会议后整理内容、跟进重点工作事项的效率。对于经常参加会议的人来说,这个功能无疑是一个非常实用的工具,能够节省大量时间和精力该功能的应用场景主要是PC电脑端录音转文字自动生成会议纪要,旨在为用户提供最优质的服务和最先进的技术,快速提升办公效率的产
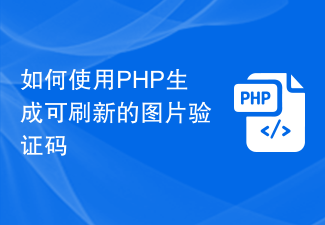
如何使用PHP生成可刷新的图片验证码随着互联网的发展,为了防止恶意攻击和机器自动操作现象,很多网站都使用了验证码来进行用户验证。其中一种常见的验证码类型就是图片验证码,通过生成一张包含随机字符的图片,要求用户输入正确的字符才能进行后续操作。本文将介绍如何使用PHP生成可刷新的图片验证码,并提供具体的代码示例。步骤一:创建验证码图片首先,我们需要创建一个用于生
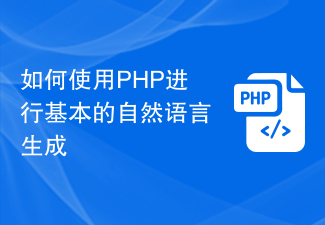
自然语言生成是一种人工智能技术,它能够将数据转换为自然语言文本。在当今的大数据时代,越来越多的业务需要将数据可视化或呈现给用户,而自然语言生成正是一种非常有效的方法。PHP是一种非常流行的服务器端脚本语言,它可以用于开发Web应用程序。本文将简要介绍如何使用PHP进行基本的自然语言生成。引入自然语言生成库PHP自带的函数库并不包括自然语言生成所需的功能,因此
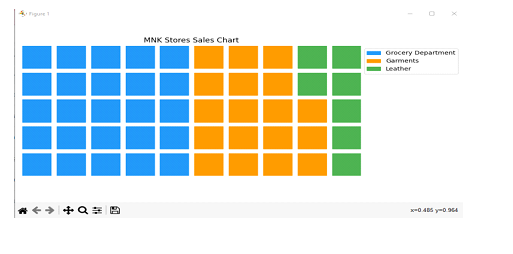
数据可视化对于高效的信息理解和展示至关重要。在众多可用的图表类型中,华夫饼图以方形瓦片在网格状结构中显示数据的新颖方式。强大的Python模块PyWaffle方便了华夫饼图的开发,类似于许多计算和数据分析方法。在本文中,我们将看看如何使用复杂的Python模块PyWaffle创建华夫饼图。让我们安装PyWafle并看看如何使用它来可视化分类数据。在您的cmd中运行以下命令来安装该库,然后将其导入到您的代码中pipinstallpywaffleExample1的中文翻译为:示例1在这个例子中,我们
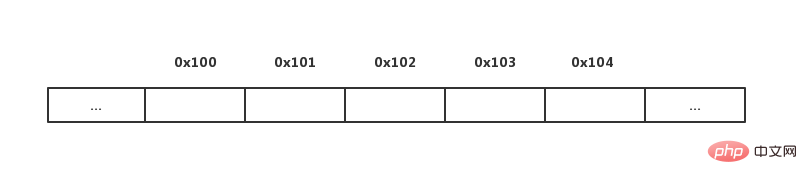
Part1聊聊Python序列类型的本质在本博客中,我们来聊聊探讨Python的各种“序列”类,内置的三大常用数据结构——列表类(list)、元组类(tuple)和字符串类(str)的本质。不知道你发现没有,这些类都有一个很明显的共性,都可以用来保存多个数据元素,最主要的功能是:每个类都支持下标(索引)访问该序列的元素,比如使用语法Seq[i]。其实上面每个类都是使用数组这种简单的数据结构表示。但是熟悉Python的读者可能知道这3种数据结构又有一些不同:比如元组和字符串是不能修改的,列表可以
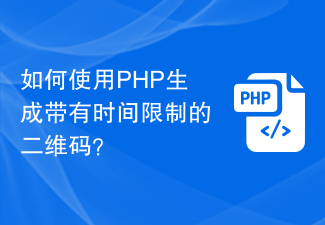
如何使用PHP生成带有时间限制的二维码?随着移动支付和电子门票的普及,二维码成为了一种常见的技术。在很多场景中,我们可能需要生成一种带有时间限制的二维码,即使在一定时间后,该二维码也将失效。本文将介绍如何使用PHP生成带有时间限制的二维码,并提供代码示例供参考。安装PHPQRCode库要使用PHP生成二维码,我们需要先安装PHPQRCode库。这个库
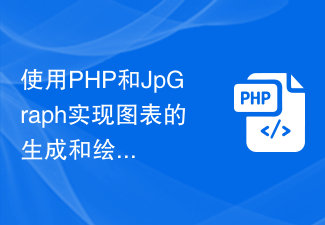
在开发Web应用程序中,图表的使用非常普遍。图表可以清晰地展示数据,让用户更容易地理解和分析信息。在PHP中,可以使用JpGraph库来生成和绘制图表,这是一个功能强大的图表生成工具,支持多种类型的图表,如柱状图、饼图、折线图等。在本文中,我们将介绍如何使用PHP和JpGraph来生成和绘制图表。安装JpGraph首先,需要下载JpGraph库,并解压到本地
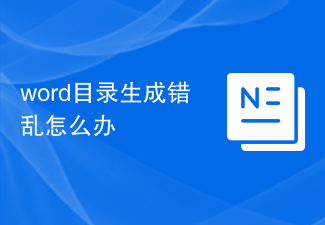
word目录生成错乱怎么办随着科技的发展,电子文档已经成为我们日常工作和学习中不可或缺的一部分。而在编辑电子文档时,尤其是长篇文章或论文中,目录的生成是一个非常重要的步骤。目录能够方便读者查找到文章的内容和结构,提高阅读效率。然而,有时候我们在生成目录的过程中会遇到一些问题,比如目录生成出错,顺序混乱等。那么,如果word目录生成错乱,我们应该如何解决呢?首


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
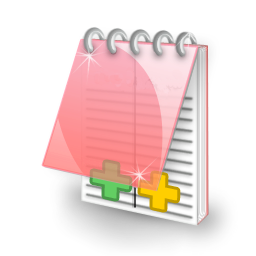
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
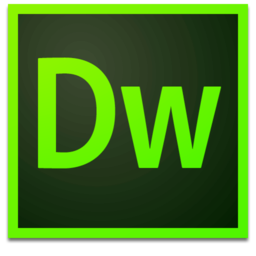
Dreamweaver Mac version
Visual web development tools
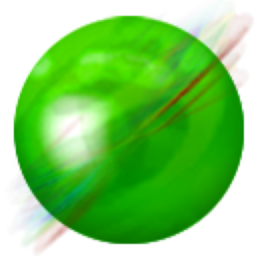
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
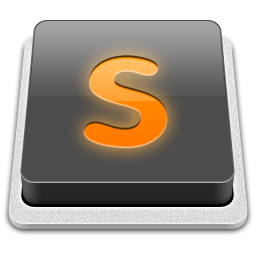
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
