


PHP extension and embedding--Arrays and hash tables in PHP extension 2_PHP tutorial
Following the above section, we continue to talk about the array and hash table API in the PHP extension. This section mainly talks about
Callback traversal function, normal traversal function, destructor, sorting, comparison, extreme value function
Iteration by hash Apply:
To traverse the array, the simplest way is to use a function similar to the foreach statement in the PHP language, zend_hash_apply, which receives a callback function and passes each element of the hashtable to it.
typedef int (*apply_func_t)(void *pDest TSRMLS_DC); void zend_hash_apply(HashTable *ht, apply_func_t apply_func TSRMLS_DC);
typedef int (*apply_func_arg_t)(void *pDest, void *argument TSRMLS_DC); void <strong>zend_hash_apply_with_argument</strong>(HashTable *ht, apply_func_arg_t apply_func, void *data TSRMLS_DC);One can pass parameters, and the other does not pass parameters, only the value in the hash table. For functions that can pass arguments, the possibility of applying them in extensions is greater. Callback functions may have different return values:
Table return value of callback function
Constant Meaning
ZEND_HASH_APPLY_KEEP ends the current request and enters the next cycle. It has the same effect as when a loop in the forech statement of the PHP language is executed or the continue keyword is encountered.
ZEND_HASH_APPLY_STOP jumps out, which has the same effect as the break keyword in the forech statement of the PHP language.
ZEND_HASH_APPLY_REMOVE removes the current element and continues processing the next one. Equivalent to in PHP language: unset($foo[$key]);continue;
For a simple php traversal code
<?php foreach($arr as $val) { echo "The value is: $val\n"; } ?>The expanded form is as follows: First define the callback function
int php_sample_print_zval(zval **val TSRMLS_DC) { //重新copy一个zval,防止破坏原数据 zval tmpcopy = **val; zval_copy_ctor(&tmpcopy); //转换为字符串 INIT_PZVAL(&tmpcopy); convert_to_string(&tmpcopy); //开始输出 php_printf("The value is: "); PHPWRITE(Z_STRVAL(tmpcopy), Z_STRLEN(tmpcopy)); php_printf("\n"); //毁尸灭迹 zval_dtor(&tmpcopy); //返回,继续遍历下一个~ return ZEND_HASH_APPLY_KEEP; }Then define the loop function: zend_hash_apply(arrht, php_sample_print_zval TSRMLS_CC);
A hash table named arrht whose element type is zval* is traversed.
Note that what is stored in the hash table is not an element, but a pointer, which is a zval**. When copying, the pointer is also copied, and the content of the hash table itself will not be changed.
In order to receive both the value and the key during the loop, the third form of zend_hash_apply(): zend_hash_apply_with_arguments()
<?php foreach($arr as $key => $val) { echo "The value of $key is: $val\n"; } ?>C code for this php code:
int php_sample_print_zval_and_key(zval **val, int num_args, va_list args, zend_hash_key *hash_key) { /* 复制zval从而使得原来的内容被保存下来 */ zval tmpcopy = **val; /* tsrm_ls is needed by output functions */ TSRMLS_FETCH(); zval_copy_ctor(&tmpcopy); /* Reset refcount & Convert */ INIT_PZVAL(&tmpcopy); convert_to_string(&tmpcopy); /* 输出 */ php_printf("The value of "); if (hash_key->nKeyLength) { /* 如果是字符串类型的key */ PHPWRITE(hash_key->arKey, hash_key->nKeyLength); } else { /* 如果是数字类型的key */ php_printf("%ld", hash_key->h); } php_printf(" is: "); PHPWRITE(Z_STRVAL(tmpcopy), Z_STRLEN(tmpcopy)); php_printf("\n"); /* Toss out old copy */ zval_dtor(&tmpcopy); /* continue; */ return ZEND_HASH_APPLY_KEEP; }
Execute the traversal: zend_hash_apply_with_arguments(arrht, php_sample_print_zval_and_key, 0); When we check whether the hash_key is a string type or a numeric type, it is detected through the nKeyLength attribute, not the arKey attribute. This is because the kernel sometimes leaves dirty data in the arKey attribute, but the nKeyLength attribute is safe and can be used safely. It can handle even empty string indexes. For example: $foo[''] = "Bar"; the index value is NULL character, but its length includes the last NULL character, so it is 1.
Iteration by move forward Hash table traversal can be implemented without callback. At this time, the internal pointer of the hash table is used.
There are many functions available in user space:
<?php $arr = array('a'=>1, 'b'=>2, 'c'=>3); reset($arr); while (list($key, $val) = each($arr)) { /* Do something with $key and $val */ } reset($arr); $firstkey = key($arr); $firstval = current($arr); $bval = next($arr); $cval = next($arr); ?>Each corresponding function will have a zend version:
* /* reset() */ void zend_hash_internal_pointer_reset(HashTable *ht); /* key() */ int zend_hash_get_current_key(HashTable *ht, char **strIdx, unit *strIdxLen, ulong *numIdx, zend_bool duplicate); * /* current() */ int zend_hash_get_current_data(HashTable *ht, void **pData); * /* next()/each() */ int zend_hash_move_forward(HashTable *ht); * /* prev() */ int zend_hash_move_backwards(HashTable *ht); * /* end() */ void zend_hash_internal_pointer_end(HashTable *ht); * /* Other... */ int zend_hash_get_current_key_type(HashTable *ht); int zend_hash_has_more_elements(HashTable *ht);next() prev() end() actually finds the corresponding index value, and then uses zend_hash_get_current_data() to return the element value
each() is the same as the next step, but it calls and returns zend_hash_get_current_key()
So here is a hash table traversal method without a callback function:
void php_sample_print_var_hash(HashTable *arrht) { for(zend_hash_internal_pointer_reset(arrht); zend_hash_has_more_elements(arrht) == SUCCESS; zend_hash_move_forward(arrht)) { char *key; uint keylen; ulong idx; int type; zval **ppzval, tmpcopy; type = zend_hash_get_current_key_ex(arrht, &key, &keylen, &idx, 0, NULL);//获得返回的key的类型。这个类型可能有三种 if (zend_hash_get_current_data(arrht, (void**)&ppzval) == FAILURE) {//获得当前索引所指的数据值 /* Should never actually fail * since the key is known to exist. */ continue; } /* 复制zval的值,从而原来的值不会被破坏掉 */ tmpcopy = **ppzval; zval_copy_ctor(&tmpcopy); /* 重新设定refcount 并且转换 */ INIT_PZVAL(&tmpcopy); convert_to_string(&tmpcopy); /* 输出 */ php_printf("The value of "); if (type == HASH_KEY_IS_STRING) { /* String Key / Associative */ PHPWRITE(key, keylen); } else { /* Numeric Key */ php_printf("%ld", idx); } php_printf(" is: "); PHPWRITE(Z_STRVAL(tmpcopy), Z_STRLEN(tmpcopy)); php_printf("\n"); /* 销毁原来的副本 */ zval_dtor(&tmpcopy); } }Let’s take a look at the possibility of zend_hash_get_current_key_ex return value: Constant Meaning
HASH_KEY_IS_STRING The index of the current element is of type string. therefore, a pointer to the element's key name will be populated into strIdx, and its length will be populated into stdIdxLen. If the duplicate flag is set to a nonzero value, the key will be estrndup()'d before being populated into strIdx. The calling application is expected to free this duplicated string.
HASH_KEY_IS_LONG The index of the current element is numeric.
HASH_KEY_NON_EXISTANT The internal pointer in the HashTable has been moved to the end and does not point to any element.
Destruction Note that there are only four destructors: The first two are used to delete individual elements from the hash table:
int zend_hash_del(HashTable *ht, char *arKey, uint nKeyLen); int zend_hash_index_del(HashTable *ht, ulong h);Return to SUCCESS OR FAILURE
Versions corresponding to string and numeric indexes respectively.
When an element is removed from the hash table, the hash table's destructor is called with a pointer to the element.
When completely deleting the hash table: void zend_hash_clean(HashTable *ht); which is equivalent to calling zend_hash_del in a loop. In addition to executing clean, calling the following function will also destroy all the space applied for by zend_hash_init: void zend_hash_destroy(HashTable *ht);
Let's look at the life cycle of a hash table to have a clearer understanding of the entire process:
int sample_strvec_handler(int argc, char **argv TSRMLS_DC) { HashTable *ht; /* 为哈希表分配空间 */ ALLOC_HASHTABLE(ht); /* 初始化哈希表的内部状态 */ if (zend_hash_init(ht, argc, NULL, ZVAL_PTR_DTOR, 0) == FAILURE) { FREE_HASHTABLE(ht); return FAILURE; } /* 把每个字符串变成zval* */ while (argc) { zval *value; MAKE_STD_ZVAL(value); ZVAL_STRING(value, argv[argc], 1); argv++; if (zend_hash_next_index_insert(ht, (void**)&value, sizeof(zval*)) == FAILURE) { /* 对于分配失败的情况应该跳掉 */ zval_ptr_dtor(&value); } } /* Do some work */ process_hashtable(ht); /* 毁坏哈希表 * 释放所有的分配的空旷 */ zend_hash_destroy(ht); /* Free the HashTable itself */ FREE_HASHTABLE(ht); return SUCCESS; }
Sorting, Comparing, and Going to the Extreme(s) For size comparison of two hash tables: typedef int (*compare_func_t)(void *a, void *b TSRMLS_DC); This function is just like qsort. Expect your own function to compare a and b and return -1 0 1
Here is an example using size comparison:
int zend_hash_minmax(HashTable *ht, compare_func_t compar, int flag, void **pData TSRMLS_DC);If flag is 0, the minimum value is returned, otherwise it is the maximum value.
下面则给出一个更为具体的例子,通过不同的flag就可以控制到底是返回最大值还是最小值:
int fname_compare(zend_function *a, zend_function *b TSRMLS_DC) { return strcasecmp(a->common.function_name, b->common.function_name); } void php_sample_funcname_sort(TSRMLS_D) { zend_function *fe; if (zend_hash_minmax(EG(function_table), fname_compare, 0, (void **)&fe) == SUCCESS) { php_printf("Min function: %s\n", fe->common.function_name); } if (zend_hash_minmax(EG(function_table), fname_compare, 1, (void **)&fe) == SUCCESS) { php_printf("Max function: %s\n", fe->common.function_name); } }
还有一个进行哈希比较的函数: int zend_hash_compare(HashTable *hta, HashTable *htb,
compare_func_t compar, zend_bool ordered TSRMLS_DC); 先比较哈希表的个数,哪个多哪个大。 如果一样多的,就每个元素去比较。
另外还有一个专门的排序函数:
typedef void (*sort_func_t)(void **Buckets, size_t numBuckets, size_t sizBucket, compare_func_t comp TSRMLS_DC); int zend_hash_sort(HashTable *ht, sort_func_t sort_func, compare_func_t compare_func, int renumber TSRMLS_DC);一般就用zend_qsort作为sort_func就够了。renumber这个参数如果设为1的话,那么就会抛弃原有的索引键值关系,赋予新的数字键值。 zend_hash_sort(target_hash, zend_qsort,array_data_compare, 1 TSRMLS_CC); array_data_compare是一个返回compare_func_t类型数据的函数,它将按照HashTable中zval*值的大小进行排序。
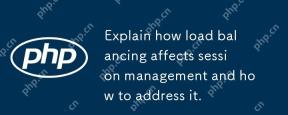
Load balancing affects session management, but can be resolved with session replication, session stickiness, and centralized session storage. 1. Session Replication Copy session data between servers. 2. Session stickiness directs user requests to the same server. 3. Centralized session storage uses independent servers such as Redis to store session data to ensure data sharing.
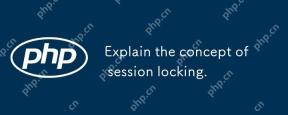
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
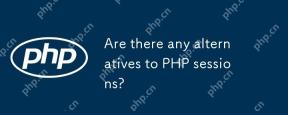
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
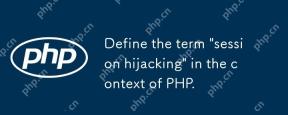
Sessionhijacking refers to an attacker impersonating a user by obtaining the user's sessionID. Prevention methods include: 1) encrypting communication using HTTPS; 2) verifying the source of the sessionID; 3) using a secure sessionID generation algorithm; 4) regularly updating the sessionID.
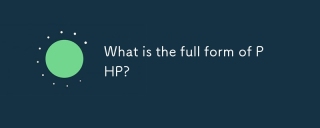
The article discusses PHP, detailing its full form, main uses in web development, comparison with Python and Java, and its ease of learning for beginners.
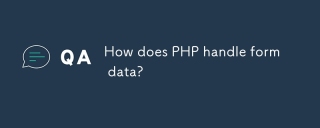
PHP handles form data using $\_POST and $\_GET superglobals, with security ensured through validation, sanitization, and secure database interactions.
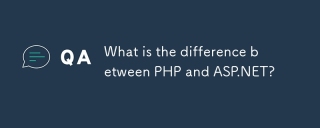
The article compares PHP and ASP.NET, focusing on their suitability for large-scale web applications, performance differences, and security features. Both are viable for large projects, but PHP is open-source and platform-independent, while ASP.NET,
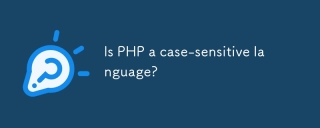
PHP's case sensitivity varies: functions are insensitive, while variables and classes are sensitive. Best practices include consistent naming and using case-insensitive functions for comparisons.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
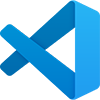
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
