


php oauth v1.0 Detailed explanation of client and server processes and implementation_PHP tutorial
php oauth client and server process and implementation
Introduction:
1. Mainly used for third parties to obtain user resources. Commonly used for third-party login authorization to obtain user information
2. It is a protocol RFC-5849 (not a software or service)
3. Authentication + Authorization
Flow chart:
163 | 开心网 | 新浪微博 |
---|---|---|
![]() |
![]() |
![]() |
Client and server implementation:
1. From the flow chart, we can see that the following steps are required
0. Obtain the user Key and Secret (outside the flow chart) [server/create_consumer.php]
1. Get Request Token and Request Secret [client/get_request_token.php] 2. Return Request Token and Request Secret [server/request_token.php] 3. Redirect the authorization page——" 【server/authorize.php】 4. User authorization callback ——| 5. Obtain Access Token and Access Secret [client/get_access_token.php] 6. Return Access Token and Access Secret [server/access_token.php] 7. Call api (outside the flow chart) [client/get_api.php] 8. Return the data obtained by the api (outside the flow chart) [server/api.php]Code directory structure
2. Code implementation process
0: server/create_consumer.phpThe client generates consumer key and consumer secret
<?php echo 'Consumer key: ' . sha1(OAuthProvider::generateToken(40)); echo '<br/>'; echo 'Consumer secret: ' . sha1(OAuthProvider::generateToken(40));OAuthProvider: OAuth provider class
generateToken: Generate a random token
The generateToken function requires pay attention to performanceWe pay attention to the performance difference between the second parameter dev/random and dev/urandom. There is no detailed explanation here. Please tune it according to your own project
For specific performance information, please refer to: /dev/random One reason why Mcrypt responds slowly
sha1: Generate signature using HMAC-SHA1 algorithm
Baidu: OAuth requests can use HMAC-SHA1 or MD5 algorithms to generate signatures.
Sina Weibo: OAuth requests use the HMAC-SHA1 algorithm to generate signatures
Kaixin.com: Signature method, currently only supports HMAC-SHA1
Run results
1: client/get_request_token.php gets Request Token and Request Secret
<?php $consumer_key = '2b4e141bf09beecdeb3479cd106038100febf399'; $consumer_secret = 'fab40ca819c25d5fb4abf3e7cae8da5c25b67d05'; $request_url = 'http://localhost/test/server/request_token.php';//获取服务器request_token地址 $callback_url = 'http://localhost/test/client/get_access_token.php';//回调本地地址 $authorize_url = 'http://localhost/test/server/authorize.php';//服务端授权验证地址 $oauth = new OAuth($consumer_key, $consumer_secret); //获取到 //oauth_token指的是request_token //oauth_token_secret指的是request_secret //scope 申请权限所需参数 all或无此参数默认是所有权限 $token_info = $oauth->getRequestToken($request_url . '?callback_url=' . $callback_url . '&scope=all'); session_start(); $_SESSION['oauth_token_secret'] = $tokenInfo['oauth_token_secret']; //此时重定向到服务端授权并显示给用户 header('Location: '.$authorize_url.'?oauth_token=' . $token_info['oauth_token']); ?>
We will pass the above code
getRequestToken($request_url . '?callback_url=' . $callback_url . '&scope=all') runs the server code
2:server/request_token.php Return request_token
<?php $oauth_token = sha1(OAuthProvider::generateToken(40)); $oauth_token_secret = sha1(OAuthProvider::generateToken(40)); //oauth_callback_confirmed:对oauth_callback的确认信号 (true/false) echo "oauth_token=$oauth_token&oauth_token_secret=$oauth_token_secret&oauth_callback_confirmed=true";
Obtain $oauth_token, $oauth_token_secret and oauth_callback_confirmed
through code 1 in 2then redirect to 3
3:server/authorize.php
Authorization verification This should require the user to enter their account and password before calling back. For the most basic implementation of the code, I omitted the default authorization between users
<?php $callback_url = 'http://localhost/test/client/get_access_token.php';//回调本地地址 header('location: '.$callback_url.'?oauth_token=' . $_REQUEST['oauth_token']);
The verification here is simple. By default, the third-party callback address has been authorized and directly obtained (the normal situation is that after the user authorizes, the server obtains the third-party callback address through the database and authorizes the oauth_token. Before, the oauth_token has always been in an unauthorized state)
The above code passes the authorized request_token (oauth_token) to 5 through the callback address (if 4 users authorize themselves, you can add a form submission here as authorization verification)
5: client/get_access_token.php gets access token
<?php $consumer_key = '2b4e141bf09beecdeb3479cd106038100febf399'; $consumer_secret = 'fab40ca819c25d5fb4abf3e7cae8da5c25b67d05'; $access_url = 'http://localhost/test/server/access_token.php';//获取服务器access_token地址 $OAuth = new OAuth($consumer_key, $consumer_secret); $OAuth->setToken($_GET['oauth_token'], $_SESSION['oauth_token_secret']); $tokenInfo = $OAuth->getAccessToken($access_url); var_dump($tokenInfo);
$tokenInfo = $OAuth->getAccessToken($access_url); Method asked 6
6: server/access_token.php returns access token
<?php $access_token = sha1(OAuthProvider::generateToken(40)); $access_secret = sha1(OAuthProvider::generateToken(40)); echo "access_token=$access_token&access_secret=$access_secret";
2: get_request_token until 6: server/access_token.php process Get request_token——》Return request_token——》User authorization verification authorize——》Verification success callback——》Get access token——>Return access token
The running results are as follows
Redirected to get_access_token and obtained access_token and access_secret
Now our client (third-party platform) has obtained the following data $consumer_key: 2b4e141bf09beecdeb3479cd106038100febf399
$consumer_secret: fab40ca819c25d5fb4abf3e7cae8da5c25b67d05
$request_token:? Program intermediate data (this data is generally time-sensitive) $request_secret:? Program intermediate data (this data is generally time-sensitive)
$access_token: 12b6f8f6d6930e0e4d1d024c0f520527d0b84d19 (This data generally has unlimited validity) $access_secret: c77463aff2c1abbd670cfb03df4bb4247910cb78 (This data generally has unlimited validity)
Now we run 7:get_api.php to 8:api.php with these parameters7: client/get_api.php gets api user data
<?php $consumer_key = '2b4e141bf09beecdeb3479cd106038100febf399'; $consumer_secret = 'fab40ca819c25d5fb4abf3e7cae8da5c25b67d05'; $access_token = '12b6f8f6d6930e0e4d1d024c0f520527d0b84d19'; $access_secret = 'c77463aff2c1abbd670cfb03df4bb4247910cb78'; $api_url='http://localhost/test/server/api.php'; $OAuth = new OAuth($consumer_key, $consumer_secret); $OAuth->setToken($access_token, $access_secret); $result = $OAuth->fetch($api_url, array(), OAUTH_HTTP_METHOD_POST); echo $OAuth->getLastResponse();
8: server/api.php returns user data
<?php function consumerHandler($Provider) { $Provider->consumer_secret = 'fab40ca819c25d5fb4abf3e7cae8da5c25b67d05'; return OAUTH_OK; } function timestampNonceHandler($Provider) { return OAUTH_OK; } function tokenHandler($Provider) { $Provider->token = '12b6f8f6d6930e0e4d1d024c0f520527d0b84d19'; $Provider->token_secret = 'c77463aff2c1abbd670cfb03df4bb4247910cb78'; return OAUTH_OK; } $OAuthProvider = new OAuthProvider(); $OAuthProvider->consumerHandler('consumerHandler'); $OAuthProvider->timestampNonceHandler('timestampNonceHandler'); $OAuthProvider->tokenHandler('tokenHandler'); try { $OAuthProvider->checkOAuthRequest(); } catch (Exception $exc) { die(var_dump($exc)); } echo 'User Data..';
Running resultsDownload
Note: php oauth v1.0 must be configured and php_curl enabled to run this (above) code

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
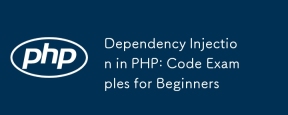
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
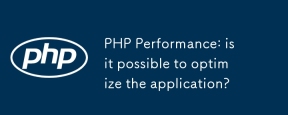
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
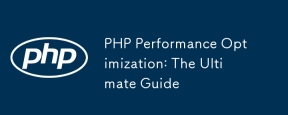
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
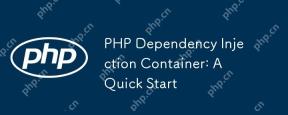
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
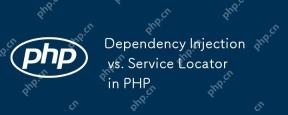
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
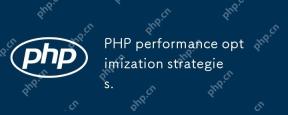
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
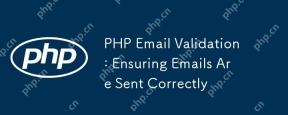
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use
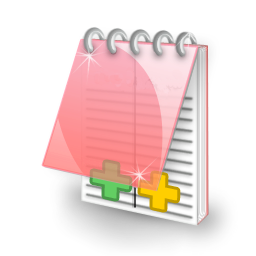
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Atom editor mac version download
The most popular open source editor
