


1. AMQP_EX_TYPE_DIRECT: direct connection type
Direct connection types also include: 1 to 1 and 1 to N (N to 1, N to N)
The code of receive.php on the receiving end is as follows
<?php $connect = new AMQPConnection(); $connect->connect(); $channel = new AMQPChannel($connect); $exchange = new AMQPExchange($channel); $exchange->setName('exchange'); $exchange->setType(AMQP_EX_TYPE_DIRECT); $exchange->declare(); $queue = new AMQPQueue($channel); $queue->setName('logs'); $queue->declare(); $queue->bind('exchange', 'logs'); while (true) { $queue->consume('callback'); } $connection->close(); function callback($envelope, $queue) { var_dump($envelope->getBody()); $queue->nack($envelope->getDeliveryTag()); }
The send.php code on the sending end is as follows
<?php $connect = new AMQPConnection(); $connect->connect(); $channel = new AMQPChannel($connect); $exchange = new AMQPExchange($channel); $exchange->setName('exchange'); $exchange->setType(AMQP_EX_TYPE_DIRECT); $exchange->declare(); $exchange->publish('direct type test','logs'); var_dump("Send Message OK"); $connect->disconnect();
The running results are as shown in the figure


<?php $connect = new AMQPConnection(); $connect->connect(); $channel = new AMQPChannel($connect); $exchange = new AMQPExchange($channel); $exchange->setName('exchange'); $exchange->setType(AMQP_EX_TYPE_DIRECT); $exchange->declare(); $queue = new AMQPQueue($channel); $queue->setName('logs'); @$queue->declare(); $queue->bind('exchange', 'logs'); while (true) { $queue->consume('callback'); } $connection->close(); function callback($envelope, $queue) { var_dump($envelope->getBody()); $queue->nack($envelope->getDeliveryTag()); }
send.php
<?php $connect = new AMQPConnection(); $connect->connect(); $channel = new AMQPChannel($connect); $exchange = new AMQPExchange($channel); $exchange->setName('exchange'); $exchange->setType(AMQP_EX_TYPE_DIRECT); $exchange->declare(); for ($index = 1; $index < 5; $index++) { $exchange->publish($index,'logs'); var_dump("Send:$index"); } $exchange->delete(); $connect->disconnect();
The running results are as follows

The queue will distribute the message to each receiving end for distribution and processing. This seems perfect, but if you want to better handle different tasks, you need fair scheduling
For example, when 1 and 3 deal with simple people and 2 and 4 deal with complex tasks, if there are too many tasks, receive_one.php will be idle and receive_two.php will have heavy tasks. We conduct the following tests Change send.php to 5 to 50
for ($index = 1; $index < 50; $index++) { $exchange->publish($index,'logs'); var_dump("Send:$index"); }
receive_two.php plus sleep(3)
function callback($envelope, $queue) { var_dump($envelope->getBody()); sleep(3); $queue->nack($envelope->getDeliveryTag()); }
When we run the program, the results are as follows

After all receive_one runs, receive_two only runs one. After that, receive_one has been idle. We can set it on the receiving end by $channel->setPrefetchCount(1);
New messages will not be received until no one completes the task, and the message will be sent to other receivers.
As follows receive_one.php and receive_two.php
$channel = new AMQPChannel($connect);Change it to the following
$channel = new AMQPChannel($connect); $channel->setPrefetchCount(1);
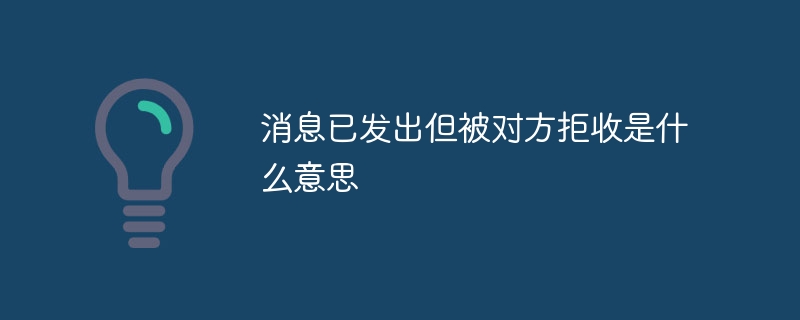
消息已发出但被对方拒收是所发送的信息已经成功地从设备发送出去,但由于某种原因,对方并没有接收到这条信息。更具体地说,这通常是因为对方已经设置了某些权限或采取了某些操作,导致你的信息无法被正常接收。
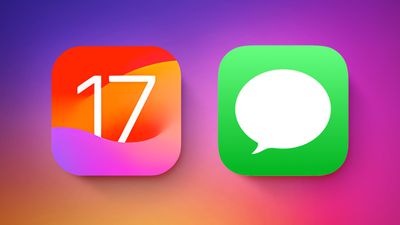
在iOS17中,Apple在其消息应用程序中添加了几项新功能,以使与其他Apple用户的交流更具创意和乐趣。其中一个功能是能够使用表情符号作为贴纸。贴纸已经在消息应用程序中存在多年了,但到目前为止,它们并没有太大变化。这是因为在iOS17中,Apple将所有标准表情符号视为贴纸,允许它们以与实际贴纸相同的方式使用。这本质上意味着您不再局限于在对话中插入它们。现在,您还可以将它们拖到消息气泡上的任何位置。您甚至可以将它们堆叠在一起,以创建小表情符号场景。以下步骤向您展示了它在iOS17中的工作方式
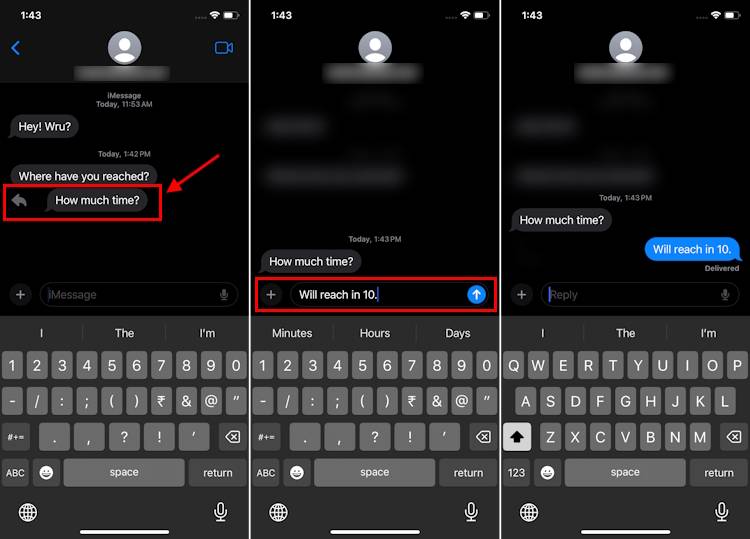
如何在iPhone上使用滑动在iMessages中回复注意:滑动回复功能仅适用于iOS17中的iMessage对话,不适用于“信息”应用程序中的常规SMS对话。在iPhone上打开“消息”应用。然后,前往iMessage对话,只需在您要回复的iMessage上向右滑动即可。完成此操作后,所选的iMessage将成为焦点,而所有其他消息将在背景中模糊不清。您将看到一个文本框,用于键入回复以及“+”图标,用于访问iMessage应用程序,如“签到”、“位置”、“贴纸”、“照片”等。只需输入您的消息,
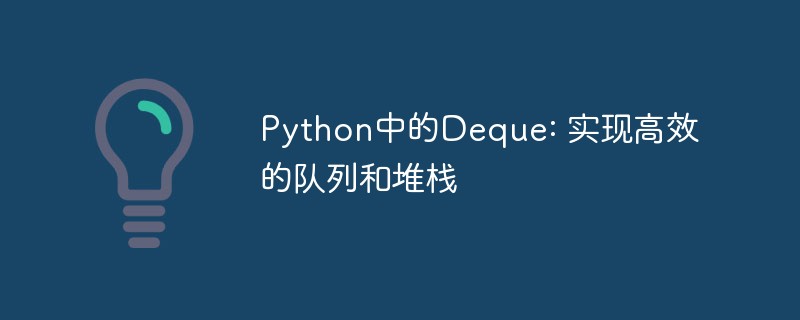
Python 中的 deque 是一个低级别的、高度优化的双端队列,对于实现优雅、高效的Pythonic 队列和堆栈很有用,它们是计算中最常见的列表式数据类型。本文中,云朵君将和大家一起学习如下:开始使用deque有效地弹出和追加元素访问deque中的任意元素用deque构建高效队列开始使用Deque向 Python 列表的右端追加元素和弹出元素的操作,一般非常高效。如果用大 O 表示时间复杂性,那么可以说它们是 O(1)。而当 Python 需要重新分配内存来增加底层列表以接受新的元素时,这些
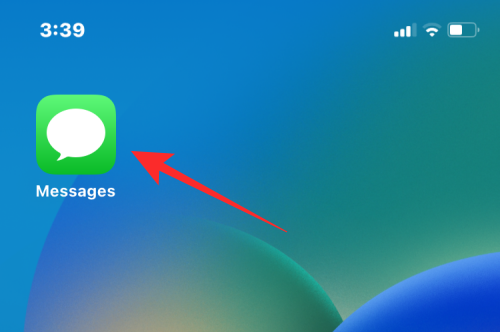
iPhone上的原生“信息”应用可让您轻松编辑已发送的文本。这样,您可以纠正您的错误、标点符号,甚至是自动更正可能已应用于您的文本的错误短语/单词。在这篇文章中,我们将了解如何在iPhone上编辑消息。如何在iPhone上编辑消息必需:运行iOS16或更高版本的iPhone。您只能在“消息”应用程序上编辑iMessage文本,并且只能在发送原始文本后的15分钟内编辑。不支持非iMessage信息文本,因此无法检索或编辑它们。在iPhone上启动消息应用程序。在“信息”中,选择要从中编辑消息的对话
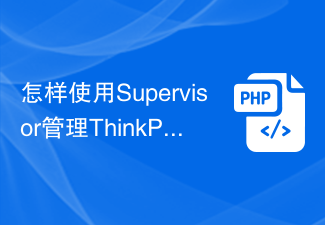
随着Web应用的不断发展,我们需要处理大量的任务来保持应用的稳定性和可用性。使用队列系统就是一种解决方案。ThinkPHP6提供了内置的队列系统来管理任务。然而,处理大量的任务需要更好的队列管理,这时候可以使用Supervisor来实现。本文将介绍如何使用Supervisor管理ThinkPHP6队列。在此之前,我们需要了解一些基础的概念:队列系统队列系统是
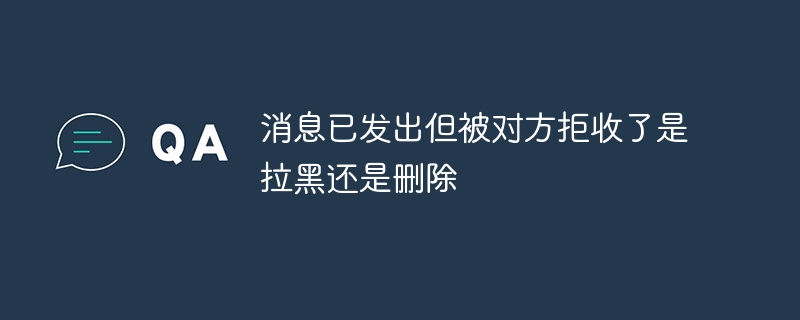
1、被加入黑名单:消息已发出但被对方拒收了一般是被拉黑了,这时你将无法向对方发送消息,对方也无法收到你的消息。2、网络问题:如果接收方的网络状况不佳,或者存在网络故障,就可能导致消息无法成功接收。此时,可以尝试等待网络恢复正常后再次发送消息。3、对方设置了免打扰:如果接收方在微信中设置了免打扰功能,那么在一定时间内,发送方的消息将不会被提醒或显示。
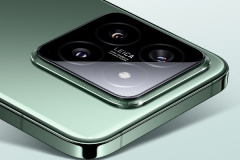
小米14Pro是一款性能配置非常出色的旗舰机型,自从正式发布以来就拥有很高的销量,小米14Pro的很多小功能是会被大家忽视的,比如说是设置来消息亮屏,功能虽小,但是是十分实用的,在使用手机的过程中大家会遇到各种问题,那么小米14Pro怎么设置来消息亮屏呢?小米14Pro怎么设置来消息亮屏?步骤一:打开手机的“设置”应用。步骤二:向下滑动直到找到“锁定屏幕和密码”选项,并点击进入。步骤三:在“锁定屏幕和密码”菜单中,找到并点击“接收通知时亮屏”选项。步骤四:在“接收通知时亮屏”页面中,打开开关以启


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor
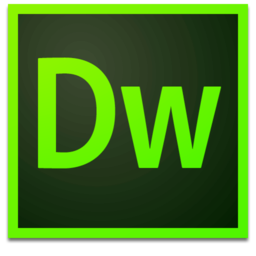
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
