Note: The article comes from Li Yanhui’s PHP video tutorial. This article is for communication only and may not be used for commercial purposes, otherwise you will be responsible for the consequences.
Learning points:
1. String formatting
2. Substring operations
3. String comparison
4. Find and replace strings
5. Processing Chinese characters
In daily programming work, processing, adjusting and finally controlling strings is an important part. It is generally believed that
this is the basis of all programming languages. Unlike other languages, PHP doesn't bother using data types to handle strings. This way, string manipulation in PHP couldn't be easier.
1. String formatting
The first step to clean up a string is to clean up the extra spaces in the string. Although this operation is not required, it is useful if is saving the string to a file or database, or comparing it to other strings.
The chop() function removes excess whitespace after the string, including new lines.
The ltrim() function removes excess white space at the beginning of the string.
The rtrim() function removes excess whitespace after the string, including new lines. This function is an alias of chop().
The trim() function removes excess whitespace on both sides of a string.
<?<span php </span><span echo</span> <span trim</span>(' PHP '<span ); </span>?>PHP has a series of functions available for reformatting strings, and these functions work in different ways
. The
nl2br() function takes a string as an input parameter and replaces the newline character in the string with the
tag in XHTML.
<?<span php </span><span echo</span> <span nl2br</span>("This is a Teacher!\nThis is a Student!"<span ); </span>?>To convert special characters to their HTML equivalent, you can use the htmlentities() and htmlspecialchars functions.
If you want to remove HTML from a string, you can use the strip_tags() function
<?<span php </span><span echo</span> <span htmlentities</span>('<strong>我是吴祁!</strong>'); <span //</span><span 转换所有字符</span> <span echo</span> <span htmlspecialchars</span>('<strong>我是吴祁!</strong>') <span //</span><span 转换特殊字符</span> <span echo</span> <span strip_tags</span>('<strong>我是吴祁!</strong>') <span //</span><span 去掉了<strong></span> ?>For strings, certain characters are definitely valid, but when inserting data into the database,
may cause some problems because the database will interpret these characters as control characters. These problematic characters are quotation marks (single quotation mark
and double quotation mark), backslash () and NULL characters.
PHP provides two functions specifically for escaping strings. Before writing any strings to the database, they should
be reformatted using addslashes().
After calling addslashes(), all quotes have slashes added and stripslashes() function removes them. these slashes.
<?<span php </span><span echo</span> <span addslashes</span>('This is \a" Teacher! '<span ); </span>?>You can reformat the case of letters in a string.
strtoupper() function converts the string to uppercase
strtolower() function converts the string to lowercase
ucfirst() function converts the first letter to uppercase
ucwords() function converts each letter to uppercase Convert the first letter of words to uppercase
<?<span php </span><span echo</span> <span strtoupper</span>('yc60.com@gmail.com'<span ); </span>?>Fill string function: str_pad() fills the string with the specified number of characters.
<?<span php </span><span echo</span> <span str_pad</span>('Salad',10).'is good.'<span ; </span>?>
2. Manipulate substrings
Often, we want to look at individual parts of a string. For example, look at words in a sentence, or split a domain name or email address into its component parts. PHP provides several string functions to achieve this functionality.Use the functions explode(), implode() and join()
To implement this functionality, the first function we will use is explode().
Use the implode() or join() function to achieve the opposite effect of the function explode(). The effects of these two functions are
consistent.
<?<span php </span><span $email</span> = 'yc60.com@gmail.com'<span ; </span><span $email_array</span> = <span explode</span>('@',<span $email</span><span ); </span>?>The strtok() function only takes out some fragments (called tokens) from the string at a time. For processing one
word from a string at a time, the strtok() function works better than the explode() function.
<?<span php </span><span $str</span> = "I,will.be#back"<span ; </span><span $tok</span> = <span strtok</span>(<span $str</span>,",.#"<span ); </span><span while</span>(<span $tok</span><span ) { </span><span echo</span> "<span $tok</span><br \>"<span ; </span><span $tok</span> = <span strtok</span>(",.#"<span ); } </span>?>The function substr() allows us to access a substring of a given start and end point of a string. This function is not suitable for our example, but it can be very useful when you need to get a part of a fixed format string.
Decompose the string: str_split() returns an array, where each array element is a character
<?<span php </span><span echo</span> <span substr</span>("abcdef", 1, 3<span ); </span>?>
Reverse a string: strrev() can reverse a string.
<?<span php </span><span print_r</span>(<span str_split</span>('This is a Teacher!'<span )); </span>?>3. String comparison
<?<span php </span><span echo</span> <span strrev</span>('This is a Teacher!'<span ); </span>?>
So far, we have used the "==" sign to compare two strings for equality. Some more complex comparisons can be performed using PHP. These comparisons fall into two categories: partial matches and other cases. String sorting: strcmp(), strcasecmp() and strnatcmp()
This function requires two parameter strings for comparison. This function returns 0 if the two strings are equal, a positive number if is lexicographically behind str1 and str2 (greater than str2), and a negative
number if str1 is less than str2. This function is case-sensitive.
The function strcasecmp() is the same as strcmp() except that it is not case sensitive.
The function strnatcmp() and the corresponding case-insensitive strnatcasecmp() function are new in PHP4.
These two functions compare strings according to "natural sorting". The so-called natural sorting is to sort in the order that people are accustomed to.
<?<span php </span><span echo</span> <span strcmp</span>('a','b'<span ); </span>?>
使用strspn()函数返回一个字符串中包含有另一个字符串中字符的第一部分的长度。也
就是求两个字符串之间相同的部分。
<?<span php </span><span echo</span> <span strspn</span>('gmail','yc60.com@gmail.com'<span ); </span>?>
使用strlen()函数测试字符串的长度
可以使用函数strlen()来检查字符串的长度。如果传给它一个字符串,这个函数将返回
字符串的长度。例如, strlen("hello") 将返回5.
<?<span php </span><span echo</span> <span strlen</span>('This is a Teacher!'<span ); </span>?>
确定字符串出现的频率:substr_count()返回一个字符串在另一个字符串中出现的次数。
<?<span php </span><span echo</span> <span substr_count</span>('yc60.com@gmail.com','c'<span ); </span>?>
四.查找替换字符串
通常,我们需要检查一个更长的字符串中是否含有一个特定的子字符串。这种部分匹配
通常比测试字符串的完全等价更有用处。
在字符串中查找字符串:strstr()、strchr()、strrchr()和stristr()
函数strstr()是最常见的,它可以用于在一个较长的字符串专供查找匹配的字符串或字
符。请注意,函数strchr()和strstr()完全一样。
<?<span php </span><span echo</span> <span strstr</span>('yc60.com@gmail.com','@'<span ); </span>?>
函数strstr()有两个变体。第一个变体是stristr(),它几乎和strstr()一样,其区别在于不区
分字符大小。对于我们的只能表单应用程序来说,这个函数非常有用,因为用户可以输入
"delivery"、"Delivery"和"DELIVERY"。
第二个变体是strrchr(),它也几乎和strstr()一样,只不过是strstr()的别名。
查找字符串的位置:strpos()、strrpos()。
函数strpos()和strrpos()的操作和strstr()类似,但它不是返回一个子字符串,而返回子字
符串needle 在字符串haystack 中的位置。更有趣的是,现在的PHP 手册建议使用strpos()
函数代替strstr()函数来查看一个子字符串在一个字符串中出现的位置,因为前者的运行速度
更快。
<?<span php </span><span echo</span> <span strrpos</span>('yc60.com@gmail.com','c'<span ); </span>?>
替换字符串:str_replace()、str_ireplace()、substr_replace()
<?<span php </span><span echo</span> <span str_replace</span>('@','#','yc60.com@gmail.com'<span ); </span><span echo</span> <span substr_replace</span>('yc60.com@gmail.com','###',0,5<span ); </span>?>
五.处理中文字符
对于以上的字符串函数,有些可以用于中文,但有些却不适用中文。所以,PHP 提供
了专门的函数来解决这样的问题。
中文字符可以是gbk,utf8,gb2312
mb_strlen() 对应的函数为strlen() 求字符串的长度
mb_strstr() 对应的函数为strstr() 求某字符串到结尾的字符
mb_strpos() 对应的函数为strpos() 求出字符最先出现处
mb_substr() 对应的函数为substr() 取出指定的字符串
mb_substr_count() 对应函数为substr_str() 返回字符串出现的次数
最后扫一遍帮助手册
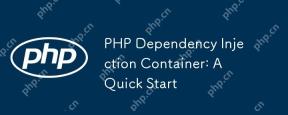
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
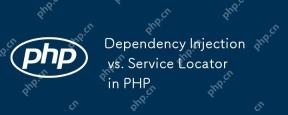
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
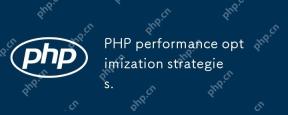
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
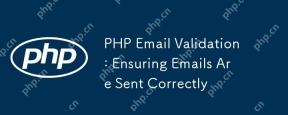
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl
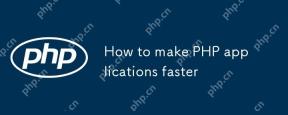
TomakePHPapplicationsfaster,followthesesteps:1)UseOpcodeCachinglikeOPcachetostoreprecompiledscriptbytecode.2)MinimizeDatabaseQueriesbyusingquerycachingandefficientindexing.3)LeveragePHP7 Featuresforbettercodeefficiency.4)ImplementCachingStrategiessuc
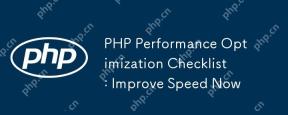
ToimprovePHPapplicationspeed,followthesesteps:1)EnableopcodecachingwithAPCutoreducescriptexecutiontime.2)ImplementdatabasequerycachingusingPDOtominimizedatabasehits.3)UseHTTP/2tomultiplexrequestsandreduceconnectionoverhead.4)Limitsessionusagebyclosin
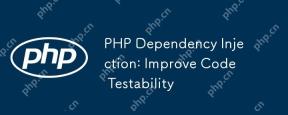
Dependency injection (DI) significantly improves the testability of PHP code by explicitly transitive dependencies. 1) DI decoupling classes and specific implementations make testing and maintenance more flexible. 2) Among the three types, the constructor injects explicit expression dependencies to keep the state consistent. 3) Use DI containers to manage complex dependencies to improve code quality and development efficiency.
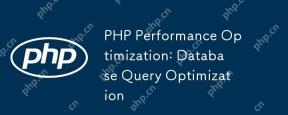
DatabasequeryoptimizationinPHPinvolvesseveralstrategiestoenhanceperformance.1)Selectonlynecessarycolumnstoreducedatatransfer.2)Useindexingtospeedupdataretrieval.3)Implementquerycachingtostoreresultsoffrequentqueries.4)Utilizepreparedstatementsforeffi


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
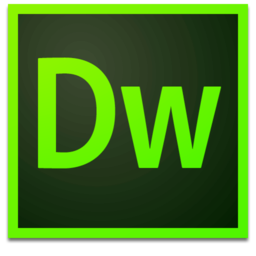
Dreamweaver Mac version
Visual web development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
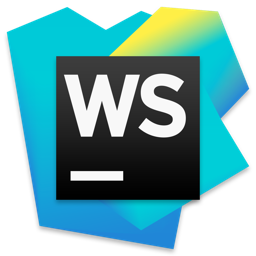
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
