AngularJS is a very powerful front-end MVC framework. The controller in AngularJS is a function that is used to add additional functions to the scope of the view ($scope). We use it to set the initial state of the scope object and Add custom behavior.
When we create a new controller, angularJS will help us generate and pass a new $scope object to this controller. In any part of the angularJS application, there is a parent scope, top-level This is the level where ng-app is located, and its parent scope is $rootScope.
$root of each $scope points to $rootScope, and $cope.$parent points to the parent scope.
The communication between cotrollers is essentially how the $scope where the current controller is located communicates with the $scopes on other controllers.
There are usually 3 solutions:
Using the principle of scope inheritance, the child controller accesses the content in the parent controller. Use events in angularJS, that is, use $on, $emit, $broadcast for message delivery. Use services in angularJS
The first way
That is, scopes are nested within scopes. There are certain usage restrictions and scopes need to be nested. In actual development, this scenario is relatively rare, but it is not impossible. This method is simpler and more direct.
By default in angularJS, when an attribute cannot be found in the current scope, it will be searched in the parent scope. If it cannot be found, it will be searched until $rootScope is found. If it cannot be found in $rootScope, the program will still run, but the view will not be updated.
Example
javascript
//Javascript app.controller('ParentController', function($scope) { $scope.person = {greeted: false}; }); app.controller('ChildController', function($scope) { $scope.sayHello = function() { $scope.person.name = 'Ari Lerner'; }; }); //HTML <div ng-controller="ParentController"> <div ng-controller="ChildController"> <a ng-click="sayHello()">Say hello</a> </div> {{ person }} </div> //result {"greeted":true, "name": "Ari Lerner"}
The second way
Because scopes are hierarchical, scope chains can be used to pass events.
There are two ways to deliver events: * $broadcast: The triggered event must be propagated downward to notify the entire event system (allowing any scope to handle this event). * $emit: If you want to remind a global module, you need to pass the event upward when you need to notify a higher-level scope (such as $rootscope).
Use $on on the scope for event monitoring.
Example
JavaScript
app.controller('ParentController', function($scope) { $scope.$on('$fromSubControllerClick', function(e,data){ console.log(data); // hello }); }); app.controller('ChildController', function($scope) { $scope.sayHello = function() { $scope.$emit('$fromSubControllerClick','hello'); }; }); //HTML <div ng-controller="ParentController"> <div ng-controller="ChildController"> <a ng-click="sayHello()">Say hello</a> </div> </div>
Another issue I want to talk about here is the performance issue of event propagation. If the $broadcast+$on method notifies all sub-scopes, there will be performance issues here, so it is recommended to use the $emit+$on method. In order to further improve performance, the defined event handling function must be released together when the scope is destroyed.
Using $emit+$on requires us to bind the event listener to $rootScope, for example:
JavaScript
angular .module('MyApp') .controller('MyController', ['$scope', '$rootScope', function MyController($scope, $rootScope) { var unbind = $rootScope.$on('someComponent.someCrazyEvent', function(){ console.log('foo'); }); $scope.$on('$destroy', unbind); } ]);
But this method is a bit cumbersome, and it makes me uncomfortable when defining multiple event handling functions, so let’s improve it
Use a decorator to define a new event binding function:
JavaScript
angular .module('MyApp') .config(['$provide', function($provide){ $provide.decorator('$rootScope', ['$delegate', function($delegate){ Object.defineProperty($delegate.constructor.prototype, '$onRootScope', { value: function(name, listener){ var unsubscribe = $delegate.$on(name, listener); this.$on('$destroy', unsubscribe); return unsubscribe; }, enumerable: false }); return $delegate; }]); }]);
Then when we define the event handler function in the controller:
JavaScript
angular .module('MyApp') .controller('MyController', ['$scope', function MyController($scope) { $scope.$onRootScope('someComponent.someCrazyEvent', function(){ console.log('foo'); }); } ]);
I highly recommend this approach
The third way
Using the characteristics of the service singleton mode in angularJS, service (service) provides a way to maintain data throughout the entire life cycle of the application, communicate between controllers, and ensure data consistency .
Generally, we encapsulate the server to provide an interface for applications to access data, or to interact with data remotely.
Example
JavaScript
var myApp = angular.module("myApp", []); myApp.factory('Data', function() { return { name: "Ting" } }); myApp.controller('FirstCtrl', function($scope, Data) { $scope.data = Data; $scope.setName = function() { Data.name = "Jack"; } }); myApp.controller('SecondCtrl', function($scope, Data) { $scope.data = Data; $scope.setName = function() { Data.name = "Moby"; } });
The above is the correct communication method for the AngularJS controller. I hope it can help everyone.
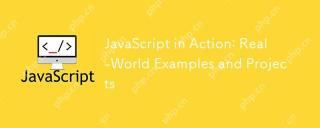
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
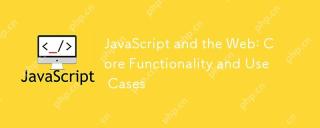
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
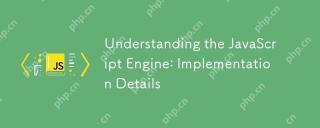
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
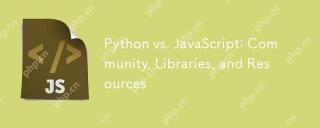
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
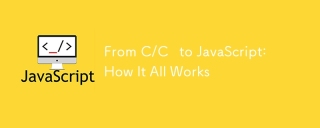
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
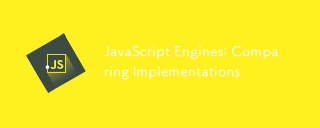
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
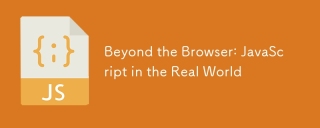
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor
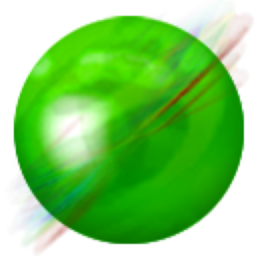
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment