


一个简洁实用的PHP缓存类完整实例,简洁实用php缓存
本文完整描述了一个简洁实用的PHP缓存类,可用来检查缓存文件是否在设置更新时间之内、清除缓存文件、根据当前动态文件生成缓存文件名、连续创建目录、缓存文件输出静态等功能。对于采用PHP开发CMS系统来说,离不开对缓存的处理,合理利用好缓存可有效的提高程序执行效率。
php缓存类文件完整代码如下:
<?php /* * 缓存类 cache */ class cache { //缓存目录 var $cacheRoot = "./cache/"; //缓存更新时间秒数,0为不缓存 var $cacheLimitTime = 0; //缓存文件名 var $cacheFileName = ""; //缓存扩展名 var $cacheFileExt = "php"; /* * 构造函数 * int $cacheLimitTime 缓存更新时间 */ function cache( $cacheLimitTime ) { if( intval( $cacheLimitTime ) ) $this->cacheLimitTime = $cacheLimitTime; $this->cacheFileName = $this->getCacheFileName(); ob_start(); } /* * 检查缓存文件是否在设置更新时间之内 * 返回:如果在更新时间之内则返回文件内容,反之则返回失败 */ function cacheCheck(){ if( file_exists( $this->cacheFileName ) ) { $cTime = $this->getFileCreateTime( $this->cacheFileName ); if( $cTime + $this->cacheLimitTime > time() ) { echo file_get_contents( $this->cacheFileName ); ob_end_flush(); exit; } } return false; } /* * 缓存文件或者输出静态 * string $staticFileName 静态文件名(含相对路径) */ function caching( $staticFileName = "" ){ if( $this->cacheFileName ) { $cacheContent = ob_get_contents(); ob_end_flush(); if( $staticFileName ) { $this->saveFile( $staticFileName, $cacheContent ); } if( $this->cacheLimitTime ) $this->saveFile( $this->cacheFileName, $cacheContent ); } } /* * 清除缓存文件 * string $fileName 指定文件名(含函数)或者all(全部) * 返回:清除成功返回true,反之返回false */ function clearCache( $fileName = "all" ) { if( $fileName != "all" ) { $fileName = $this->cacheRoot . strtoupper(md5($fileName)).".".$this->cacheFileExt; if( file_exists( $fileName ) ) { return @unlink( $fileName ); }else return false; } if ( is_dir( $this->cacheRoot ) ) { if ( $dir = @opendir( $this->cacheRoot ) ) { while ( $file = @readdir( $dir ) ) { $check = is_dir( $file ); if ( !$check ) @unlink( $this->cacheRoot . $file ); } @closedir( $dir ); return true; }else{ return false; } }else{ return false; } } /*根据当前动态文件生成缓存文件名*/ function getCacheFileName() { return $this->cacheRoot . strtoupper(md5($_SERVER["REQUEST_URI"])).".".$this->cacheFileExt; } /* * 缓存文件建立时间 * string $fileName 缓存文件名(含相对路径) * 返回:文件生成时间秒数,文件不存在返回0 */ function getFileCreateTime( $fileName ) { if( ! trim($fileName) ) return 0; if( file_exists( $fileName ) ) { return intval(filemtime( $fileName )); }else return 0; } /* * 保存文件 * string $fileName 文件名(含相对路径) * string $text 文件内容 * 返回:成功返回ture,失败返回false */ function saveFile($fileName, $text) { if( ! $fileName || ! $text ) return false; if( $this->makeDir( dirname( $fileName ) ) ) { if( $fp = fopen( $fileName, "w" ) ) { if( @fwrite( $fp, $text ) ) { fclose($fp); return true; }else { fclose($fp); return false; } } } return false; } /* * 连续建目录 * string $dir 目录字符串 * int $mode 权限数字 * 返回:顺利创建或者全部已建返回true,其它方式返回false */ function makeDir( $dir, $mode = "0777" ) { if( ! $dir ) return 0; $dir = str_replace( "\\", "/", $dir ); $mdir = ""; foreach( explode( "/", $dir ) as $val ) { $mdir .= $val."/"; if( $val == ".." || $val == "." || trim( $val ) == "" ) continue; if( ! file_exists( $mdir ) ) { if(!@mkdir( $mdir, $mode )){ return false; } } } return true; } } ?>
使用该缓存类的时候可将以上代码保存为cache.php,具体用法如下所示:
include( "cache.php" ); $cache = new cache(30); $cache->cacheCheck(); echo date("Y-m-d H:i:s"); $cache->caching();
一种是对 页面结果的缓存 应用服务器级别的 软件如 squid
一种是 内存级别的 一般是对 php 频繁调用的并且如果每次查询会消耗大量资源的数据 软件有 memcached
一种是 对php 程序进行优化编码的缓存 如 apache 里面的 apc, eAccelerator, XCache 等
还有一种就是文件缓存 这种一般是 用php自己实现的 没什么可说的.
看你的具体需求是怎样的了..有疑问 请联系 1465663870
//以下是缓存类:
class cache {
//缓存目录
var $cacheRoot = "./cache/";
//缓存更新时间秒数,0为不缓存
var $cacheLimitTime = 0;
//缓存文件名
var $cacheFileName = "";
//缓存扩展名
var $cacheFileExt = "html";
/*
* 构造函数
* int $cacheLimitTime 缓存更新时间
*/
function cache( $cacheLimitTime ) {
if( intval( $cacheLimitTime ) )
$this->cacheLimitTime = $cacheLimitTime;
$this->cacheFileName = $this->getCacheFileName();
//echo $this->cacheFileName;
ob_start();
}
/*
* 检查缓存文件是否在设置更新时间之内
* 返回:如果在更新时间之内则返回文件内容,反之则返回失败
*/
function cacheCheck(){
if( file_exists( $this->cacheFileName ) ) {
$cTime = $this->getFileCreateTime( $this->cacheFileName );
if( $cTime + $this->cacheLimitTime > time() ) {
echo file_get_contents( $this->cacheFileName );
ob_end_flush();
exit;
}
}
return false;
}
/*
* 缓存文件或者输出静态
* string $staticFileName 静态文件名(含相对路径)
*/
function caching( $staticFileName = "" ){
if( $this->cacheFileName ) {
$cacheContent = ob_get_contents();
//echo $cacheContent;
ob_end_flush();
if( $staticFileName ) {
$this->saveFile( $staticFileName, $cacheContent );
}
if( $this->cacheLimitTime )
$this->saveFile( $this->cacheFileName, $cacheContent );
}
}
/*
......余下全文>>
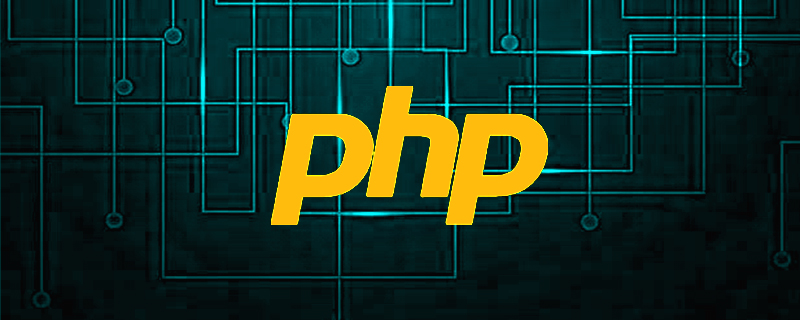
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
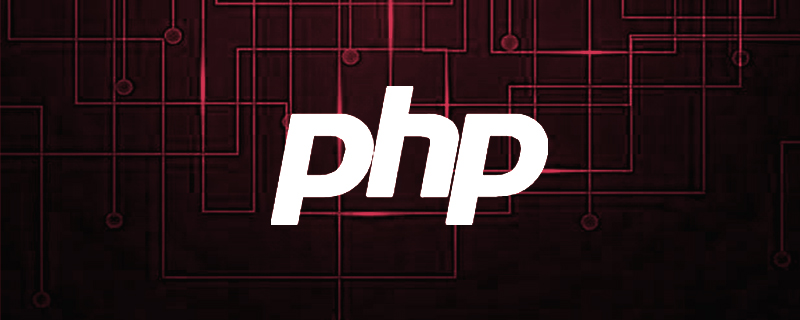
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
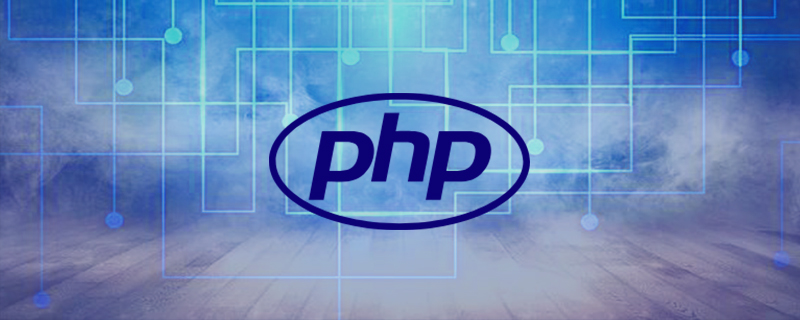
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
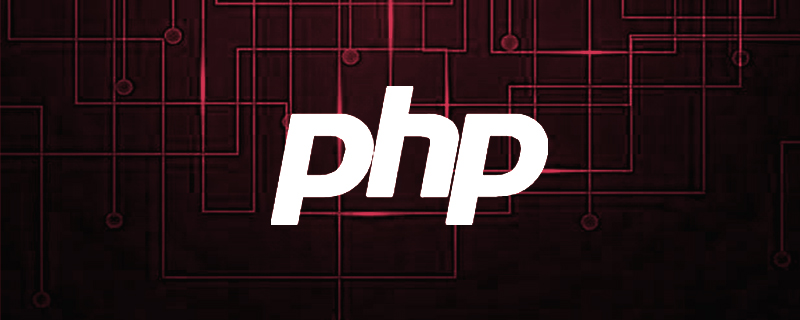
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
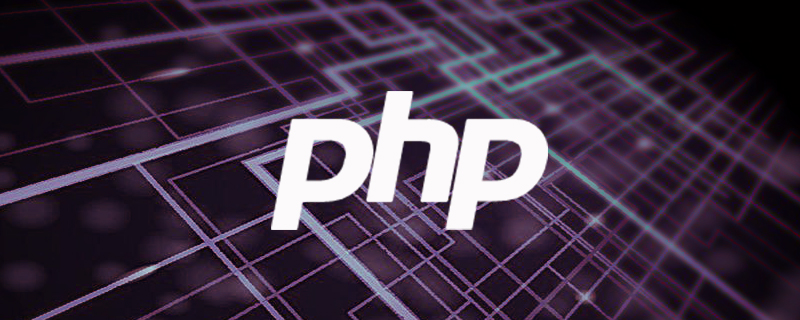
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
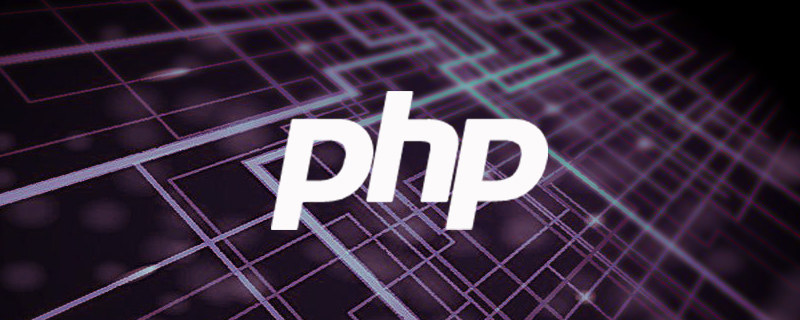
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
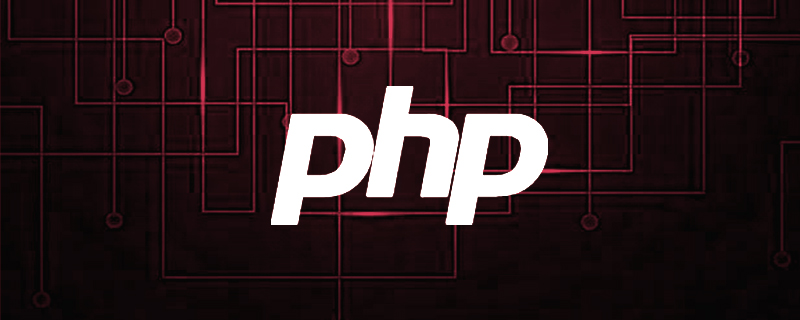
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
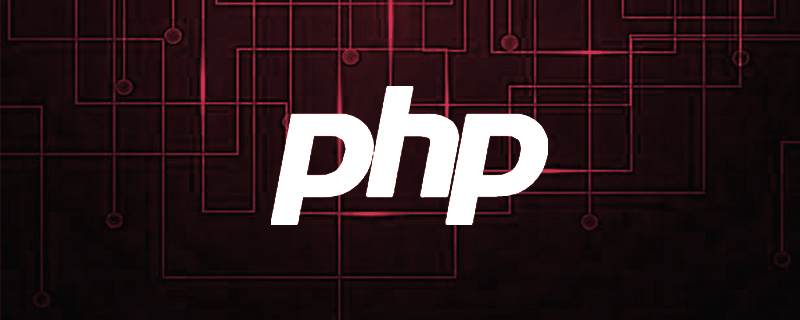
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
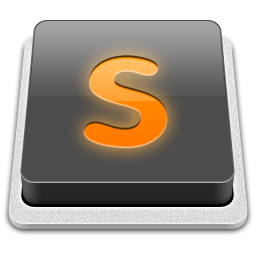
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Linux new version
SublimeText3 Linux latest version
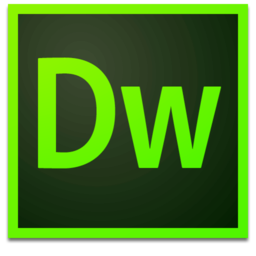
Dreamweaver Mac version
Visual web development tools
