AngularJS is a very powerful front-end MVC framework. Use $http in AngualrJS to send a request to the remote API each time and wait for the response. There is a slight waiting process in between. How to handle this waiting process gracefully?
It would be a more elegant approach if we pop up a mask layer while waiting.
This involves intercepting $http request responses. When requesting, a mask layer pops up, and when the response is received, the mask layer is hidden.
In fact, $httpProvider has already provided us with a $httpProvider.interceptors property, and we only need to put the custom interceptors into this collection.
How to behave? It’s roughly like this:
<div data-my-overlay> <br/><img src="/static/imghwm/default1.png" data-src="spinner.gif" class="lazy" / alt="A mask layer Directive_AngularJS for each $http request in AngularJS" > Loading </div>
Show that the loaded image is included in the Directive, and transclusion will definitely be used.
It also involves the issue of the mask layer pop-up delay time. We hope to set this through the API in the config, so it is necessary for us to create a provider and set the delay time through this.
$http request response mask layer Directive:
(function(){ var myOverlayDirective =function($q, $timeout, $window, httpInterceptor, myOverlayConfig){ return { restrict: 'EA', transclude: true, scope: { myOverlayDelay: "@" }, template: '<div id="overlay-container" class="onverlayContainer">' + '<div id="overlay-background" class="onverlayBackground"></div>' + '<div id="onverlay-content" class="onverlayContent" data-ng-transclude>' + '</div>' + '</div>', link: function(scope, element, attrs){ var overlayContainer = null, timePromise = null, timerPromiseHide = null, inSession = false, queue = [], overlayConfig = myOverlayConfig.getConfig(); init(); //初始化 function init(){ wireUpHttpInterceptor(); if(window.jQuery) wirejQueryInterceptor(); overlayContainer = document.getElementById('overlay-container'); } //自定义Angular的http拦截器 function wireUpHttpInterceptor(){ //请求拦截 httpInterceptor.request = function(config){ //判断是否满足显示遮罩的条件 if(shouldShowOverlay(config.method, config.url)){ processRequest(); } return config || $q.when(config); }; //响应拦截 httpInterceptor.response = function(response){ processResponse(); return response || $q.when(response); } //异常拦截 httpInterceptor.responseError = function(rejection){ processResponse(); return $q.reject(rejection); } } //自定义jQuery的http拦截器 function wirejQueryInterceptor(){ $(document).ajaxStart(function(){ processRequest(); }); $(document).ajaxComplete(function(){ processResponse(); }); $(document).ajaxError(function(){ processResponse(); }); } //处理请求 function processRequest(){ queue.push({}); if(queue.length == 1){ timePromise = $timeout(function(){ if(queue.length) showOverlay(); }, scope.myOverlayDelay ? scope.myOverlayDelay : overlayConfig.delay); } } //处理响应 function processResponse(){ queue.pop(); if(queue.length == 0){ timerPromiseHide = $timeout(function(){ hideOverlay(); if(timerPromiseHide) $timeout.cancel(timerPromiseHide); },scope.myOverlayDelay ? scope.myOverlayDelay : overlayConfig.delay); } } //显示遮罩层 function showOverlay(){ var w = 0; var h = 0; if(!$window.innerWidth){ if(!(document.documentElement.clientWidth == 0)){ w = document.documentElement.clientWidth; h = document.documentElement.clientHeight; } else { w = document.body.clientWidth; h = document.body. clientHeight; } }else{ w = $window.innerWidth; h = $window.innerHeight; } var content = docuemnt.getElementById('overlay-content'); var contetWidth = parseInt(getComputedStyle(content, 'width').replace('px','')); var contentHeight = parseInt(getComputedStyle(content, 'height').replace('px','')); content.style.top = h / 2 - contentHeight / 2 + 'px'; content.style.left = w / 2 - contentWidth / 2 + 'px'; overlayContainer.style.display = 'block'; } function hideOverlay(){ if(timePromise) $timeout.cancel(timerPromise); overlayContainer.style.display = 'none'; } //得到一个函数的执行结果 var getComputedStyle = function(){ var func = null; if(document.defaultView && document.defaultView.getComputedStyle){ func = document.defaultView.getComputedStyle; } else if(typeof(document.body.currentStyle) !== "undefined"){ func = function(element, anything){ return element["currentStyle"]; } } return function(element, style){ reutrn func(element, null)[style]; } }(); //决定是否显示遮罩层 function shouldShowOverlay(method, url){ var searchCriteria = { method: method, url: url }; return angular.isUndefined(findUrl(overlayConfig.exceptUrls, searchCriteria)); } function findUrl(urlList, searchCriteria){ var retVal = undefined; angular.forEach(urlList, function(url){ if(angular.equals(url, searchCriteria)){ retVal = true; return false;//推出循环 } }) return retVal; } } } }; //配置$httpProvider var httpProvider = function($httpProvider){ $httpProvider.interceptors.push('httpInterceptor'); }; //自定义interceptor var httpInterceptor = function(){ return {}; }; //提供配置 var myOverlayConfig = function(){ //默认配置 var config = { delay: 500, exceptUrl: [] }; //设置延迟 this.setDelay = function(delayTime){ config.delay = delayTime; } //设置异常处理url this.setExceptionUrl = function(urlList){ config.exceptUrl = urlList; }; //获取配置 this.$get = function(){ return { getDelayTime: getDelayTime, getExceptUrls: getExceptUrls, getConfig: getConfig } function getDelayTime(){ return config.delay; } function getExeptUrls(){ return config.exceptUrls; } function getConfig(){ return config; } }; }; var myDirectiveApp = angular.module('my.Directive',[]); myDirectiveApp.provider('myOverlayConfig', myOverlayConfig); myDirectiveApp.factory('httpInterceptor', httpInterceptor); myDirectiveApp.config('$httpProvider', httpProvider); myDirectiveApp.directive('myOverlay', ['$q', '$timeout', '$window', 'httpInceptor', 'myOverlayConfig', myOverlayDirective]); }());
In global configuration:
(functioin(){ angular.module('customersApp',['ngRoute', 'my.Directive']) .config(['$routeProvider, 'myOverlayConfigProvider', funciton($routeProvider, myOverlayConfigProvider){ ... myOverlayConfigProvider.setDealy(100); myOverlayConfigProvider.setExceptionUrl({ method: 'GET', url: '' }); }]); }());
The above is a mask layer Directive for each $http request in AngualrJS that the editor shared with you. I hope it will be helpful to everyone.
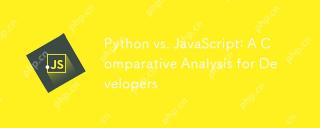
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
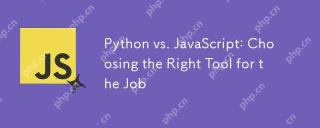
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
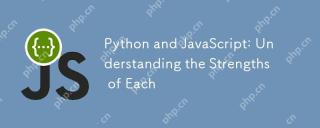
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
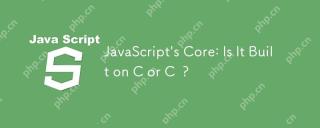
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
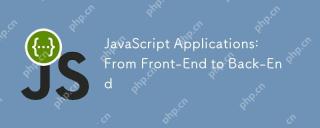
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
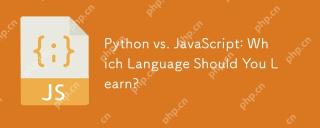
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
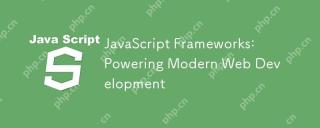
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
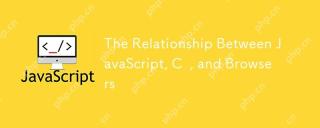
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
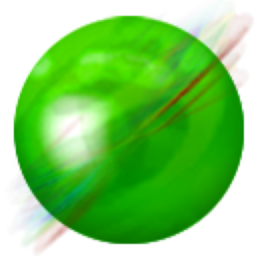
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
