


PHP practical news management system using bootstrap framework_PHP tutorial
PHP practical news management system uses the bootstrap framework
I just came into contact with PHP and I wrote a news management system imitating the video, which also used the bootstrap framework
Write it down to organize your thoughts.
This is a very simple system. The first step is to create a database table.
mysql>create database newsdb
mysql> create table news(
-> id int unsigned not null auto_increment primary key,//This is the id of the news
-> title varchar(64) not null,//This is the title of the news
-> keywords varchar(64) not null,//This is the keyword of the news
-> author varchar(16) not null,//This is the author of the news
-> addtime int unsigned not null,//This is the adding time of the news
-> content text not null);//This is the content of the news
In this way, the database table is completed, and now we can start writing the page.
First, I wrote a database configuration file dbconfig.php:
define(HOST,"localhost");//Host name
define(USER,"root");//Username
define(PASS,"");//Password
define(DBNAME,"newsdb");//Database name
?>
Then there is a menu.php file
body{background:orange;}
@media(max-width:997px){body{background:#0FC;}}
After completing the above two simple steps, it is time to write the homepage http://blog.csdn.net/q114942784/article/details/index.php:
First, import the navigation bar menu.php
Then add a title and table
Browse news
新闻id | 标题 | 关键字 | 作者 | 时间 | 内容 | 操作 |
---|---|---|---|---|---|---|
{$row['id']} | ";{$row['tilte']} | ";{$row['keywords']} | ";{$row['author']} | ";{$row['addtime']} | ";{$row['content']} | ";
删除;//此处的“#”只是一个代号,后面会把它替换掉,由于增删操作比较复杂,所以单独写一个action.php文件 修改; | ";
News id | Title | Keywords | Author | Time | Content | Operation |
---|---|---|---|---|---|---|
{$row['id']} | "; echo"{$row['tilte']} | "; echo"{$row['keywords']} | "; echo"{$row['author']} | "; echo"{$row['addtime']} | "; echo"{$row['content']} | "; echo"Delete;//The "#" here is just a code name, which will be replaced later. Since the addition and deletion operations are complicated, write a separate action.php file Modify; | "; echo"
action.php:
//This is a page for adding, deleting, modifying and checking data
//1. Import configuration file
require("dbconfig.php");
//2. Link to mysql and select the database
$link=@mysql_connect(HOST,USER,PASS) or die("Database link failed");
mysql_select_db(DBNAME,$link);
//3. Based on the value of action, determine the operation and execute the corresponding code
switch($_GET["action"]){
case "add":
//1. Obtain the information to be added and supplement other information
$tilte=$_POST["title"];
$keywords=$_POST["keywords"];
$author=$_POST["author"];
$content=$_POST["content"];
$addtime=time();
//2. Information filtering
//3. Splice sql statements and perform corresponding operations
$sql=insert into news value(null,'($title)','($keywords)','($author)',$addtime,'($content)');
mysql_query($sql,$link);
//4. Determine whether it is successful
$id=mysql_insert_id($link);
if($id>0){
echo "
News information added successfully
";}
else{
echo "
Failed to add news information
";}
echo("return");
echo("Browse news");
break;
case "del":
///1. Get the news id to be deleted:
$id=$_GET['id'];
//2. Assemble the delete sql statement and perform the corresponding deletion operation
$sql="delete from news where id=($id)";
mysql_query($sql,$link);
//3. Automatically jump to the news browsing interface after deletion
header("location:http://blog.csdn.net/q114942784/article/details/index.php");
break;
case "update":
//1. Get the information to be modified
$title = $_POST['title'];
$keywords = $_POST['keywords'];
$author = $_POST['author'];
$content = $_POST['content'];
$id = $_POST['id'];
//2. Filter the information to be modified (omitted here)
//3. Assemble and modify the sql statement and perform the modification operation
$sql="update news set title="($title)",keywords='($keywords)',author='($author)',content='($content)' where id=($id)" ;
//echo $sql;
mysql_query($sql,$link);
//4. Jump to the browsing interface
header("location:http://blog.csdn.net/q114942784/article/details/index.php");
break;
}
//4. Close the database link
mysql_close("$link");
?>
Write the page to add news below http://blog.csdn.net/q114942784/article/details/add.php file:
Publish news
Then the edited page edit.php page:
//1.Import configuration file
require("dbconfig.php");
//2. Connect to mysql and select the database
$link=@mysql_connect(HOST,USER,PASS)or die("Database link failed");
mysql_select_db(DBNAME,$link);
//3. Get the ID of the information to be modified, assemble and view the sql statement, execute the query, and obtain the information to be modified
$sql="select * from news where id={$_GET['id']}";
$result=mysql_query($sql,$link);
//4. Determine whether the information to be modified has been obtained
if($result && mysql_num_rows($result)>0){
$news=mysql_fetch_assoc($result);
}else{
die("No information found to be modified");
}
?>
Editor News
Finally, let me mention what should be used to replace the deleted and modified "#"
In order to be more humane, a tip is given using js code
For the first "#", use javascript: dodel({$row["id"]}) to replace
For the second "#", use edit.php? id={$row["id"]}replacement
At this point, a complete php news management system is basically completed. It will be improved tomorrow.

ThesecrettokeepingaPHP-poweredwebsiterunningsmoothlyunderheavyloadinvolvesseveralkeystrategies:1)ImplementopcodecachingwithOPcachetoreducescriptexecutiontime,2)UsedatabasequerycachingwithRedistolessendatabaseload,3)LeverageCDNslikeCloudflareforservin
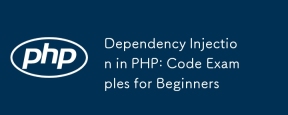
You should care about DependencyInjection(DI) because it makes your code clearer and easier to maintain. 1) DI makes it more modular by decoupling classes, 2) improves the convenience of testing and code flexibility, 3) Use DI containers to manage complex dependencies, but pay attention to performance impact and circular dependencies, 4) The best practice is to rely on abstract interfaces to achieve loose coupling.
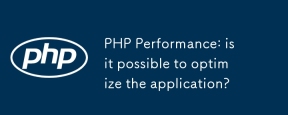
Yes,optimizingaPHPapplicationispossibleandessential.1)ImplementcachingusingAPCutoreducedatabaseload.2)Optimizedatabaseswithindexing,efficientqueries,andconnectionpooling.3)Enhancecodewithbuilt-infunctions,avoidingglobalvariables,andusingopcodecaching
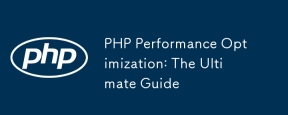
ThekeystrategiestosignificantlyboostPHPapplicationperformanceare:1)UseopcodecachinglikeOPcachetoreduceexecutiontime,2)Optimizedatabaseinteractionswithpreparedstatementsandproperindexing,3)ConfigurewebserverslikeNginxwithPHP-FPMforbetterperformance,4)
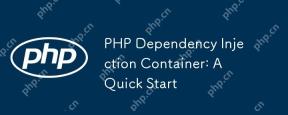
APHPDependencyInjectionContainerisatoolthatmanagesclassdependencies,enhancingcodemodularity,testability,andmaintainability.Itactsasacentralhubforcreatingandinjectingdependencies,thusreducingtightcouplingandeasingunittesting.
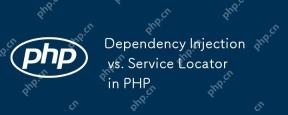
Select DependencyInjection (DI) for large applications, ServiceLocator is suitable for small projects or prototypes. 1) DI improves the testability and modularity of the code through constructor injection. 2) ServiceLocator obtains services through center registration, which is convenient but may lead to an increase in code coupling.
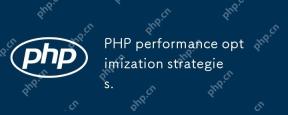
PHPapplicationscanbeoptimizedforspeedandefficiencyby:1)enablingopcacheinphp.ini,2)usingpreparedstatementswithPDOfordatabasequeries,3)replacingloopswitharray_filterandarray_mapfordataprocessing,4)configuringNginxasareverseproxy,5)implementingcachingwi
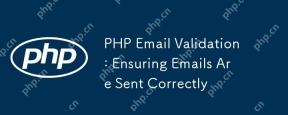
PHPemailvalidationinvolvesthreesteps:1)Formatvalidationusingregularexpressionstochecktheemailformat;2)DNSvalidationtoensurethedomainhasavalidMXrecord;3)SMTPvalidation,themostthoroughmethod,whichchecksifthemailboxexistsbyconnectingtotheSMTPserver.Impl


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
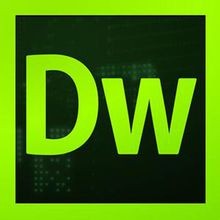
Dreamweaver CS6
Visual web development tools
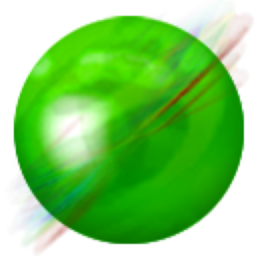
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
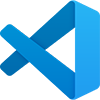
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
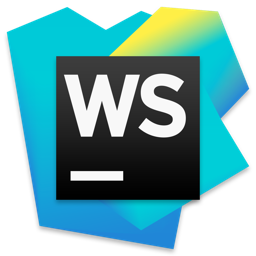
WebStorm Mac version
Useful JavaScript development tools
