


PHP Design Pattern Singleton Pattern Example Analysis_PHP Tutorial
Analysis of Singleton Pattern Examples of PHP Design Pattern
This article mainly introduces the Singleton Pattern of PHP Design Pattern, and analyzes the principles and related usage techniques of Singleton Pattern with examples. , has certain reference value, friends in need can refer to it
The example in this article describes the singleton mode of PHP design pattern. Share it with everyone for your reference. The specific analysis is as follows:
Singleton mode (responsibility mode):
To put it simply, an object (you need to understand object-oriented thinking before learning design patterns) is only responsible for a specific task;
Single instance class:
1. The constructor needs to be marked private (access control: prevent external code from using the new operator to create objects). Singleton classes cannot be instantiated in other classes and can only be instantiated by themselves;
2. Have a static member variable that holds an instance of the class
3. Have a public static method to access this instance (the getInstance() method is commonly used to instantiate a singleton class, and the instanceof operator can be used to detect whether the class has been instantiated)
In addition, you need to create a __clone() method to prevent the object from being copied (cloned)
Why use PHP singleton pattern?
1. PHP is mainly used in database applications, so there will be a large number of database operations in an application. Using the singleton mode can avoid a large number of resources consumed by new operations.
2. If a class is needed in the system to globally control certain configuration information, it can be easily implemented using the singleton mode. This can be found in the FrontController section of ZF.
3. In a page request, it is easy to debug because all the code (such as database operation class db) is concentrated in one class. We can set hooks in the class and output logs to avoid var_dump and echo everywhere.
Code implementation:
?
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 |
/1** * Design Pattern Singleton Pattern * $_instance must be declared as a static private variable * The constructor and destructor must be declared private to prevent external programs from new * class thus loses the meaning of singleton pattern * The getInstance() method must be set to public and this method must be called * to return a reference to the instance *::Operator can only access static variables and static functions * new objects will consume memory * Usage scenarios: The most commonly used place is database connection. * After using singleton mode to generate an object, * This object can be used by many other objects. */ class Danli { //Save static member variables of class instances private static $_instance; //Construction method of private tag private function __construct(){ echo 'This is a Constructed method;'; } //Create the __clone method to prevent the object from being copied and cloned public function __clone(){ trigger_error('Clone is not allow!',E_USER_ERROR); } //Singleton method, a public static method used to access the instance public static function getInstance(){ if(!(self::$_instance instanceof self)){ self::$_instance = new self; } return self::$_instance; } public function test(){ echo 'Calling method successfully'; } } //Using new to instantiate a class with a private mark constructor will report an error //$danli = new Danli(); //The correct method is to use the double colon::operator to access the static method to obtain the instance $danli = Danli::getInstance(); $danli->test(); //Copying (cloning) the object will result in an E_USER_ERROR $danli_clone = clone $danli; |
I hope this article will be helpful to everyone’s PHP programming design.
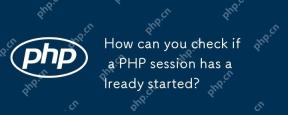
In PHP, you can use session_status() or session_id() to check whether the session has started. 1) Use the session_status() function. If PHP_SESSION_ACTIVE is returned, the session has been started. 2) Use the session_id() function, if a non-empty string is returned, the session has been started. Both methods can effectively check the session state, and choosing which method to use depends on the PHP version and personal preferences.
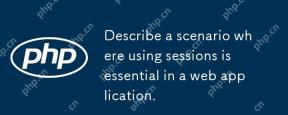
Sessionsarevitalinwebapplications,especiallyfore-commerceplatforms.Theymaintainuserdataacrossrequests,crucialforshoppingcarts,authentication,andpersonalization.InFlask,sessionscanbeimplementedusingsimplecodetomanageuserloginsanddatapersistence.
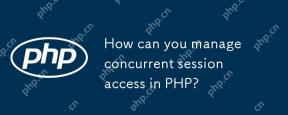
Managing concurrent session access in PHP can be done by the following methods: 1. Use the database to store session data, 2. Use Redis or Memcached, 3. Implement a session locking strategy. These methods help ensure data consistency and improve concurrency performance.
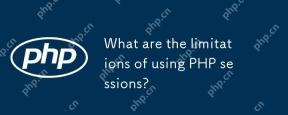
PHPsessionshaveseverallimitations:1)Storageconstraintscanleadtoperformanceissues;2)Securityvulnerabilitieslikesessionfixationattacksexist;3)Scalabilityischallengingduetoserver-specificstorage;4)Sessionexpirationmanagementcanbeproblematic;5)Datapersis
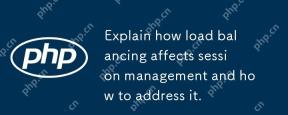
Load balancing affects session management, but can be resolved with session replication, session stickiness, and centralized session storage. 1. Session Replication Copy session data between servers. 2. Session stickiness directs user requests to the same server. 3. Centralized session storage uses independent servers such as Redis to store session data to ensure data sharing.
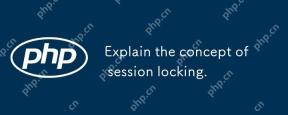
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
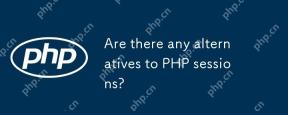
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
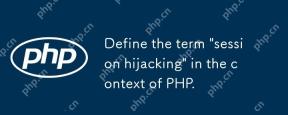
Sessionhijacking refers to an attacker impersonating a user by obtaining the user's sessionID. Prevention methods include: 1) encrypting communication using HTTPS; 2) verifying the source of the sessionID; 3) using a secure sessionID generation algorithm; 4) regularly updating the sessionID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
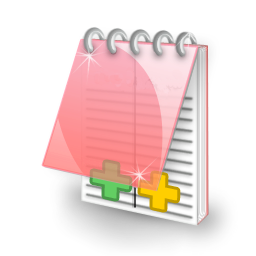
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
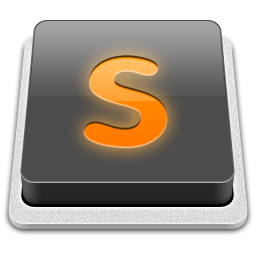
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
