Share the method of backing up the database with thinkphp
This article mainly introduces the method of backing up the database with thinkphp. It is very simple and practical. It is recommended to friends in need
It seems that THINKPHP does not have a way to back up the database, so I wrote one myself. The database connection and transaction processing are using pdo. If you need it, you can contact me and write a mysql or mysqli
The code is as follows:
class SqlAction extends Action{
function outsql(){
header(“Content-Type:text/html;charset=utf-8″);
/*Read database configuration using C method*/
$host=C(‘DB_HOST’);
$db_name=C(‘DB_NAME’);
$user=C(‘DB_USER’);
$password=C(‘DB_PWD’);
/*Call the private method of exporting the database*/
$outstream=$this->outputSql($host, $dbname, $user, $password);
/*Download export database*/
header(“Content-Disposition: attachment; filename=$dbname.sql”);
echo $outstream;
}
/*
* Database export function outputSql
* Export database data using PDO method
* $host host name such as localhost
* $dbname database name
* $user username
* $password password
* $flag flag bit 0 or 1. 0 means exporting only the database structure. 1 means exporting the database structure and data. The default is 1
*/
private function outputSql($host, $dbname, $user, $password, $flag=1) {
try {
$pdo = new PDO(“mysql:host=$host;dbname=$dbname”, $user, $password); //Connect to database
$pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); //Set tuning parameters and throw an exception when encountering an error
} catch (PDOException $e) {
echo $e->getMessage(); //If the connection is abnormal, an error message will be thrown
exit;
}
$mysql = "DROP DATABASE IF EXISTS `$dbname`;n"; //$mysql loads the sql statement. If a database exists here, drop the database
$creat_db=$pdo->query("show create database $dbname")->fetch();//Use show create database to view the sql statement
preg_match(‘/DEFAULT CHARACTER SET(.*)*/’, $creat_db[‘Create Database’],$matches);//Regularly remove the character set after DEFAULT CHARACTER SET
$mysql.=”CREATE DATABASE `$dbname` DEFAULT CHARACTER SET $matches[1]”;//This statement is such as CREATE DATABASE `test_db` DEFAULT CHARACTER SET utf8
/*Find the character sequence of the database such as COLLATE utf8_general_ci*/
$db_collate=$pdo->query(“SELECT DEFAULT_COLLATION_NAME FROM information_schema.SCHEMATA WHERE SCHEMA_NAME =’$dbname’ LIMIT 1″)->fetch();
$mysql.=”COLLATE “.$db_collate[‘DEFAULT_COLLATION_NAME’].”;nUSE `$dbname`;nn”;
$statments = $pdo->query(“show tables”); //Return the result set, show tables to view all table names
foreach ($statments as $value) {//Traverse this result set and export the information corresponding to each table name
$table_name = $value[0]; //Get the table name
$mysql.=”DROP TABLE IF EXISTS `$table_name`;n”; //Prepare Drop statements before each table
$table_query = $pdo->query(“show create table `$table_name`”); //Get the result set of the table creation information
$create_sql = $table_query->fetch(); //Use the fetch method to retrieve the array corresponding to the result set
$mysql.=$create_sql[‘Create Table’] . “;rnrn”; //Write table creation information
if ($flag != 0) {//If the flag is not 0, continue to retrieve the contents of the table and generate an insert statement
$iteams_query = $pdo->query(“select * from `$table_name`”); //Get the result set of all fields in the table
$values = ""; //Prepare empty string to load insert value
$items = ""; //Prepare empty string to load the table field name
while ($item_query = $iteams_query->fetch(PDO::FETCH_ASSOC)) { //Use associated query to return an array of field names and values in the table
$item_names = array_keys($item_query); //Get the array key value, that is, the field name
$item_names = array_map(“addslashes”, $item_names); //Translate special characters and add
$items = join(‘`,`’, $item_names); //Joint field names such as: items1`, `item2 `symbol is backticks. Next to keyboard 1, field names are enclosed in backticks
$item_values = array_values($item_query); //Get the array value, that is, the value corresponding to the field
$item_values = array_map(“addslashes”, $item_values); //Translate special characters and add
$value_string = join(“‘,’”, $item_values); //Joint values such as: value1′,'value2 values are enclosed in single quotes
$value_string = “(‘” . $value_string . “‘),”; //Add brackets around the value
$values.=”n” . $value_string; //Finally returned to $value
}
if ($values != “”) {//If $values is not empty, that is, the table has content
//Write insert statement
$insert_sql = “INSERT INTO `$table_name` (`$items`) VALUES” . rtrim($values, “,”) . “;nr”;
//Write this statement into $mysql
$mysql.=$insert_sql;
}
}
}
return $mysql;
}
}
?>
Isn’t it a very practical function? Friends can transplant it directly into their own projects.
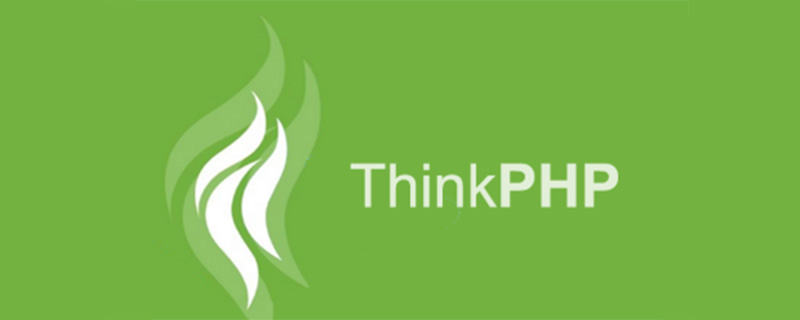
thinkphp是国产框架。ThinkPHP是一个快速、兼容而且简单的轻量级国产PHP开发框架,是为了简化企业级应用开发和敏捷WEB应用开发而诞生的。ThinkPHP从诞生以来一直秉承简洁实用的设计原则,在保持出色的性能和至简的代码的同时,也注重易用性。
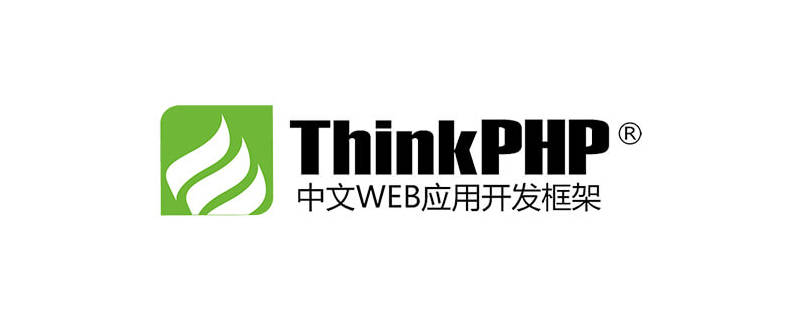
本篇文章给大家带来了关于thinkphp的相关知识,其中主要介绍了关于使用think-queue来实现普通队列和延迟队列的相关内容,think-queue是thinkphp官方提供的一个消息队列服务,下面一起来看一下,希望对大家有帮助。
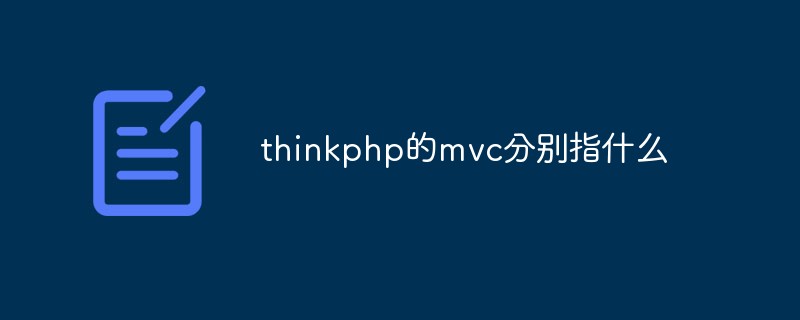
thinkphp基于的mvc分别是指:1、m是model的缩写,表示模型,用于数据处理;2、v是view的缩写,表示视图,由View类和模板文件组成;3、c是controller的缩写,表示控制器,用于逻辑处理。mvc设计模式是一种编程思想,是一种将应用程序的逻辑层和表现层进行分离的方法。
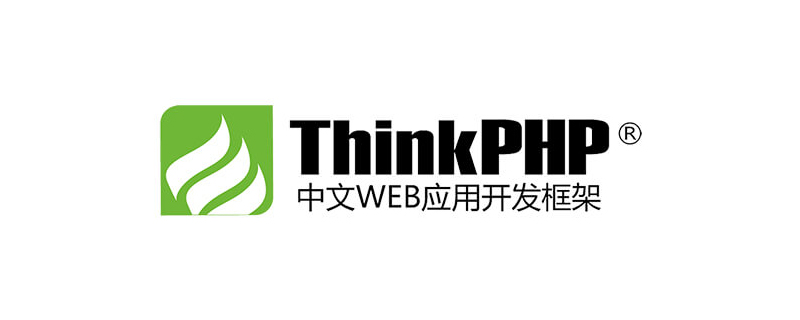
本篇文章给大家带来了关于thinkphp的相关知识,其中主要介绍了使用jwt认证的问题,下面一起来看一下,希望对大家有帮助。
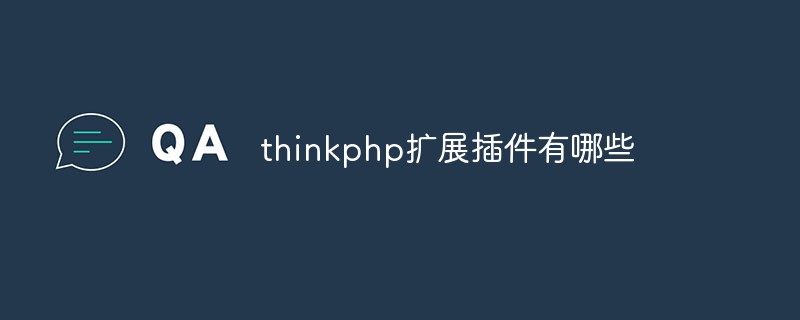
thinkphp扩展有:1、think-migration,是一种数据库迁移工具;2、think-orm,是一种ORM类库扩展;3、think-oracle,是一种Oracle驱动扩展;4、think-mongo,一种MongoDb扩展;5、think-soar,一种SQL语句优化扩展;6、porter,一种数据库管理工具;7、tp-jwt-auth,一个jwt身份验证扩展包。
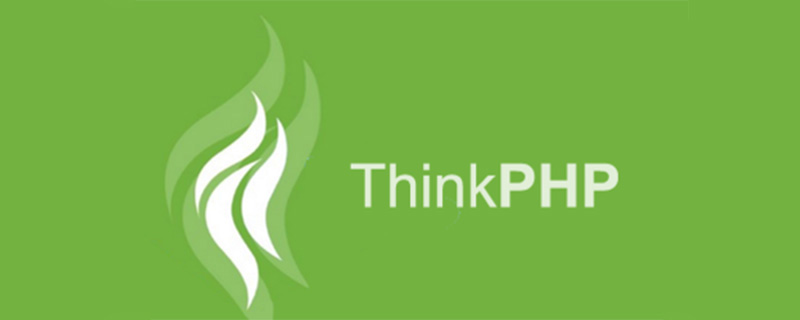
本篇文章给大家带来了关于ThinkPHP的相关知识,其中主要整理了使用think-queue实现redis消息队列的相关问题,下面一起来看一下,希望对大家有帮助。
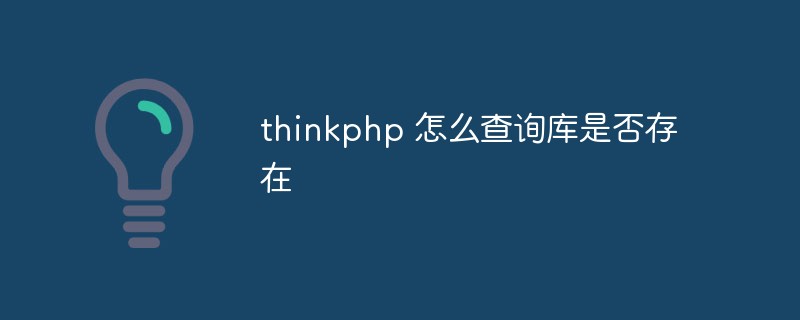
thinkphp查询库是否存在的方法:1、打开相应的tp文件;2、通过“ $isTable=db()->query('SHOW TABLES LIKE '."'".$data['table_name']."'");if($isTable){...}else{...}”方式验证表是否存在即可。
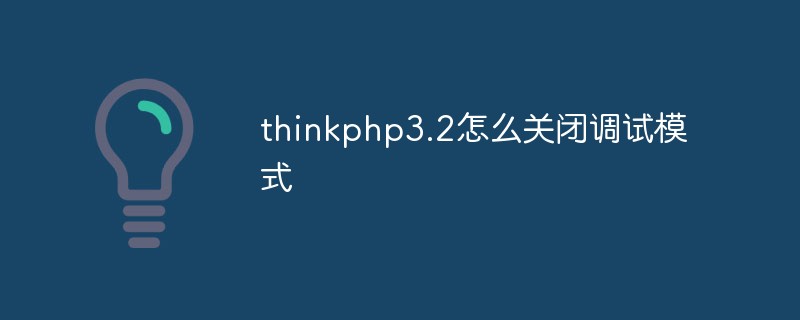
在thinkphp3.2中,可以利用define关闭调试模式,该标签用于变量和常量的定义,将入口文件中定义调试模式设为FALSE即可,语法为“define('APP_DEBUG', false);”;开启调试模式将参数值设置为true即可。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor
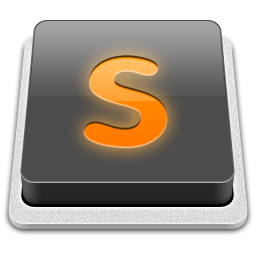
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.