5 major ways to determine whether an array is empty in PHP
1. isset function: determine whether the variable has been initialized
Note: It does not determine whether the variable is empty, and can be used to determine whether the elements in the array have been defined
Note: When using isset to determine whether an array element has been initialized, its efficiency is about 4 times higher than array_key_exists
<ol class="dp-c"><li class="alt"><span><span><?php </span></span></li><li><span><span class="vars">$a</span><span> = </span><span class="string">''</span><span>; </span></span></li><li class="alt"><span><span class="vars">$a</span><span>[</span><span class="string">'c'</span><span>] = </span><span class="string">''</span><span>; </span></span></li><li><span><span class="keyword">if</span><span> (!isset(</span><span class="vars">$a</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$a 未被初始化'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="keyword">if</span><span> (!isset(</span><span class="vars">$b</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$b 未被初始化'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li><span><span class="keyword">if</span><span> (isset(</span><span class="vars">$a</span><span>[</span><span class="string">'c'</span><span>])) </span><span class="func">echo</span><span> </span><span class="string">'$a 已经被初始化'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="comment">// 显示结果为</span><span> </span></span></li><li><span><span class="comment">// $b 未被初始化</span><span> </span></span></li><li class="alt"><span><span class="comment">// $a 已经被初始化</span><span> </span></span></li></ol>
2. empty function: detect whether the variable is "empty"
Note: Any uninitialized variable, a variable with a value of 0 or false or an empty string "" or null, an empty array, or an object without any attributes will be judged as empty==true
Note 1: Uninitialized variables can also be detected as "empty" by empty
Note 2: empty can only detect variables, not statements
<ol class="dp-c"><li class="alt"><span><span><?php </span></span></li><li><span><span class="vars">$a</span><span> = 0; </span></span></li><li class="alt"><span><span class="vars">$b</span><span> = </span><span class="string">''</span><span>; </span></span></li><li><span><span class="vars">$c</span><span> = </span><span class="keyword">array</span><span>(); </span></span></li><li class="alt"><span><span class="keyword">if</span><span> (</span><span class="func">empty</span><span class="keyword">empty</span><span>(</span><span class="vars">$a</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$a 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li><span><span class="keyword">if</span><span> (</span><span class="func">empty</span><span class="keyword">empty</span><span>(</span><span class="vars">$b</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$b 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="keyword">if</span><span> (</span><span class="func">empty</span><span class="keyword">empty</span><span>(</span><span class="vars">$c</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$c 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li><span><span class="keyword">if</span><span> (</span><span class="func">empty</span><span class="keyword">empty</span><span>(</span><span class="vars">$d</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$d 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li></ol>
3. var == null function: determine whether the variable is "empty"
Note: Variables and empty arrays whose value is 0 or false or empty string "" or null will be judged as null
Note: The significant difference from empty is that var == null will report an error when the variable is not initialized.
<ol class="dp-c"><li class="alt"><span><span><?php </span></span></li><li><span><span class="vars">$a</span><span> = 0; </span></span></li><li class="alt"><span><span class="vars">$b</span><span> = </span><span class="keyword">array</span><span>(); </span></span></li><li><span><span class="keyword">if</span><span> (</span><span class="vars">$a</span><span> == null) </span><span class="func">echo</span><span> </span><span class="string">'$a 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="keyword">if</span><span> (</span><span class="vars">$b</span><span> == null) </span><span class="func">echo</span><span> </span><span class="string">'$b 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li><span><span class="keyword">if</span><span> (</span><span class="vars">$c</span><span> == null) </span><span class="func">echo</span><span> </span><span class="string">'$b 为空'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="comment">// 显示结果为</span><span> </span></span></li><li><span><span class="comment">// $a 为空</span><span> </span></span></li><li class="alt"><span><span class="comment">// $b 为空</span><span> </span></span></li><li><span><span class="comment">// Undefined variable: c</span><span> </span></span></li></ol>
4. is_null function: detect whether the variable is "null"
Note: When the variable is assigned a value of "null", the detection result is true
Note 1: null is not case sensitive: $a = null; $a = NULL makes no difference
Note 2: The detection result is true only when the value of the variable is "null". 0, empty string, false, and empty array are all detected as false
Note 3: When the variable is not initialized, the program will report an error
<ol class="dp-c"><li class="alt"><span><span><?php </span></span></li><li><span><span class="vars">$a</span><span> = null; </span></span></li><li class="alt"><span><span class="vars">$b</span><span> = false; </span></span></li><li><span><span class="keyword">if</span><span> (</span><span class="func">is_null</span><span>(</span><span class="vars">$a</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$a 为NULL'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="keyword">if</span><span> (</span><span class="func">is_null</span><span>(</span><span class="vars">$b</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$b 为NULL'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li><span><span class="keyword">if</span><span> (</span><span class="func">is_null</span><span>(</span><span class="vars">$c</span><span>)) </span><span class="func">echo</span><span> </span><span class="string">'$c 为NULL'</span><span> . </span><span class="string">""</span><span>; </span></span></li><li class="alt"><span><span class="comment">// 显示结果为</span><span> </span></span></li><li><span><span class="comment">// $a 为NULL</span><span> </span></span></li><li class="alt"><span><span class="comment">// Undefined variable: c</span><span> </span></span></li></ol>
5. var === null function: detect whether the variable is "null", and the type of the variable must also be "null"
Note: When a variable is assigned a value of "null" and the type of the variable is also "null", the detection result is true
Note 1: When judging "null", equal to is_null has the same effect
Note 2: When the variable is not initialized, the program will report an error
Summary:
In PHP, "NULL" and "empty" are two concepts.
isset is mainly used to determine whether a variable has been initialized
empty can judge variables with values of "false", "empty", "0", "NULL", and "uninitialized" as TRUE
is_null only evaluates variables with a value of "NULL" as TRUE
var == null Judges variables with values of "false", "empty", "0", and "NULL" as TRUE
var === null Only variables with a value of "NULL" are judged as TRUE
Note: When judging whether a variable is really "NULL", is_null is mostly used to avoid interference from "false", "0" and other values.

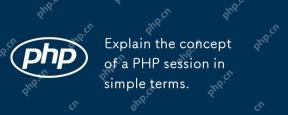
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
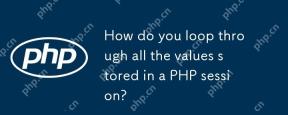
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
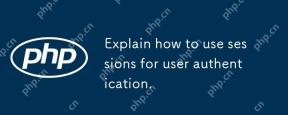
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.
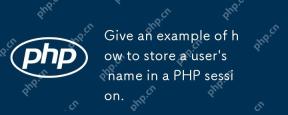
Tostoreauser'snameinaPHPsession,startthesessionwithsession_start(),thenassignthenameto$_SESSION['username'].1)Usesession_start()toinitializethesession.2)Assigntheuser'snameto$_SESSION['username'].Thisallowsyoutoaccessthenameacrossmultiplepages,enhanc
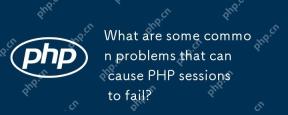
Reasons for PHPSession failure include configuration errors, cookie issues, and session expiration. 1. Configuration error: Check and set the correct session.save_path. 2.Cookie problem: Make sure the cookie is set correctly. 3.Session expires: Adjust session.gc_maxlifetime value to extend session time.
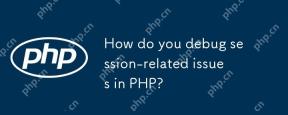
Methods to debug session problems in PHP include: 1. Check whether the session is started correctly; 2. Verify the delivery of the session ID; 3. Check the storage and reading of session data; 4. Check the server configuration. By outputting session ID and data, viewing session file content, etc., you can effectively diagnose and solve session-related problems.
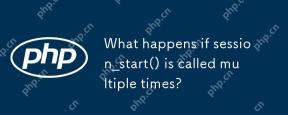
Multiple calls to session_start() will result in warning messages and possible data overwrites. 1) PHP will issue a warning, prompting that the session has been started. 2) It may cause unexpected overwriting of session data. 3) Use session_status() to check the session status to avoid repeated calls.
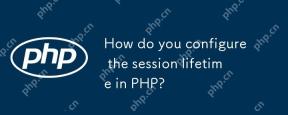
Configuring the session lifecycle in PHP can be achieved by setting session.gc_maxlifetime and session.cookie_lifetime. 1) session.gc_maxlifetime controls the survival time of server-side session data, 2) session.cookie_lifetime controls the life cycle of client cookies. When set to 0, the cookie expires when the browser is closed.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
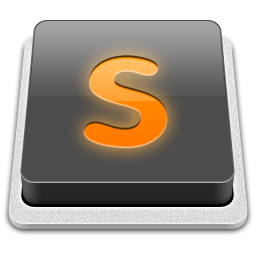
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor
