RxJava Operators (7) Conditional and Boolean
In this article we will take a look at conditional and Boolean operators.1. All/Amb
The All operator judges all the data emitted by the source Observable according to a function, and the final result returned is the judgment result. This function uses the emitted data as a parameter and internally determines whether all the data meets the judgment conditions we defined. If all are met, it returns true, otherwise it returns false.

The Amb operator can combine up to 9 Observables and let them compete. Which Observable emits data first (including onError and onComplete) will continue to emit the data of this Observable, and the data emitted by other Observables will not be discarded.

These two operators are used below. We have made such restrictions for the all operator. When the tag is false when used for the first time, an Observable of 6 numbers will be created. In the future, 5 numbers will be created. Observable.
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>private Observable<Boolean> allObserver() {<br /></li><li>Observable<Integer> just;<br /></li><li>if (tag) {<br /></li><li>just = Observable.just(1, 2, 3, 4, 5);<br /></li><li>} else {<br /></li><li>just = Observable.just(1, 2, 3, 4, 5, 6);<br /></li><li>}<br /></li><li>tag = true;<br /></li><li>return just.all(integer -> integer < 6);<br /></li><li>}<br /></li><li><br /></li><li>private Observable<Integer> ambObserver() {<br /></li><li>Observable<Integer> delay3 = Observable.just(1, 2, 3).delay(3000, TimeUnit.MILLISECONDS);<br /></li><li>Observable<Integer> delay2 = Observable.just(4, 5, 6).delay(2000, TimeUnit.MILLISECONDS);<br /></li><li>Observable<Integer> delay1 = Observable.just(7, 8, 9).delay(1000, TimeUnit.MILLISECONDS);<br /></li><li>return Observable.amb(delay1, delay2, delay3);<br /></li><li>}</li></ol>Subscriptions
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>mLButton.setText("all");<br /></li><li>mLButton.setOnClickListener(e -> allObserver().subscribe(i -> log("all:" + i)));<br /></li><li>mRButton.setText("amb");<br /></li><li>mRButton.setOnClickListener(e -> ambObserver().subscribe(i -> log("amb:" + i)));</li></ol>are as follows. The Observable of the 6 data returned for the first time does not meet the condition that all of them are less than 6, so the result is false. The subsequent ones all meet the condition, so the result is true. Using the amb operator's Observable, the first emitted data is 7, so 7, 8, and 9 are output, and other data are discarded.

2. Contains, IsEmpty
The Contains operator is used to determine whether the data emitted by the source Observable contains a certain data. If it does, it will return true. If the source Observable has ended If the data has not been emitted, false will be returned.
The IsEmpty operator is used to determine whether the source Observable has emitted data. If it has emitted data, it will return false. If the source Observable has ended but the data has not been emitted, it will return true.


Use these two operators to determine whether two Observable objects contain certain data and whether they are empty
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>private Observable<Boolean> containsObserver() {<br /></li><li>if (tag) {<br /></li><li>return Observable.just(1, 2, 3).contains(3);<br /></li><li>}<br /></li><li>tag = true;<br /></li><li>return Observable.just(1, 2, 3).contains(4);<br /></li><li>}<br /></li><li><br /></li><li>private Observable<Boolean> defaultObserver() {<br /></li><li>return Observable.create(new Observable.OnSubscribe<Integer>() {<br /></li><li>@Override<br /></li><li>public void call(Subscriber<? super Integer> subscriber) {<br /></li><li>subscriber.onCompleted();<br /></li><li>}<br /></li><li>}).isEmpty();<br /></li><li>}</li></ol>respectively Subscribe
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>mLButton.setText("contains");<br /></li><li>mLButton.setOnClickListener(e -> containsObserver().subscribe(i -> log("contains:" + i)));<br /></li><li>mRButton.setText("isEmpty");<br /></li><li>mRButton.setOnClickListener(e -> defaultObserver().subscribe(i -> log("isEmpty:" + i)));</li></ol>The operation results are as follows

3. DefaultIfEmpty
DefaultIfEmpty operator will determine whether the source Observable emits data. If If the source Observable emits data, it will emit the data normally. If not, it will emit a default data

Next we use this operator to process an empty and a non-empty Observable, if it is empty Just return to the default value 10
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>private Observable<Integer> emptyObserver() {<br /></li><li>return Observable.create(new Observable.OnSubscribe<Integer>() {<br /></li><li>@Override<br /></li><li>public void call(Subscriber<? super Integer> subscriber) {<br /></li><li>subscriber.onCompleted();<br /></li><li>}<br /></li><li>}).defaultIfEmpty(10);<br /></li><li>}<br /></li><li><br /></li><li>private Observable<Integer> notEmptyObserver() {<br /></li><li>return Observable.create(new Observable.OnSubscribe<Integer>() {<br /></li><li>@Override<br /></li><li>public void call(Subscriber<? super Integer> subscriber) {<br /></li><li>subscriber.onNext(1);<br /></li><li>subscriber.onCompleted();<br /></li><li>}<br /></li><li>}).defaultIfEmpty(10);<br /></li><li>}</li></ol>Subscribe separately
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>mLButton.setText("empty");<br /></li><li>mLButton.setOnClickListener(e -> emptyObserver().subscribe(i -> log("empty:" + i)));<br /></li><li>mRButton.setText("notEmpty");<br /></li><li>mRButton.setOnClickListener(e -> notEmptyObserver().subscribe(i -> log("notEmpty:" + i)));</li></ol>The running results are as follows

4. SequenceEqual
The SequenceEqual operator is used to determine whether the data sequences emitted by two Observables are the same (the emitted data is the same, the data sequence is the same, and the ending status is the same). If they are the same, it returns true, otherwise it returns false

Next, use SequenceEqual to judge two identical and different Observables
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>private Observable<Boolean> equalObserver() {<br /></li><li>return Observable.sequenceEqual(Observable.just(1, 2, 3), Observable.just(1, 2, 3));<br /></li><li>}<br /></li><li><br /></li><li>private Observable<Boolean> notEqualObserver() {<br /></li><li>return Observable.sequenceEqual(Observable.just(1, 2, 3), Observable.just(1, 2));<br /></li><li>}</li></ol>and subscribe separately
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>mLButton.setText("equal");<br /></li><li>mLButton.setOnClickListener(e -> equalObserver().subscribe(i -> log("equal:" + i)));<br /></li><li>mRButton.setText("notequal");<br /></li><li>mRButton.setOnClickListener(e -> notEqualObserver().subscribe(i -> log("notequal:" + i)));</li></ol>The running results are as follows

5. SkipUntil and SkipWhile
Both operators skip some data based on conditions. The difference is that SkipUnitl is It is judged based on a flag Observable. When the flag Observable does not emit data, all data emitted by the source Observable will be skipped; when the flag Observable emits a data, it starts to emit data normally. SkipWhile determines whether to skip data based on a function. When the function returns true, the data emitted by the source Observable is skipped; when the function returns false, data is emitted normally.


These two operators are used below to skip some data items.
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>private Observable<Long> skipUntilObserver() {<br /></li><li>return Observable.interval(1, TimeUnit.SECONDS).skipUntil(Observable.timer(3, TimeUnit.SECONDS));<br /></li><li>}<br /></li><li><br /></li><li>private Observable<Long> skipWhileObserver() {<br /></li><li>return Observable.interval(1, TimeUnit.SECONDS).skipWhile(aLong -> aLong < 5);<br /></li><li>}</li></ol>Subscribe separately
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>mLButton.setText("skipUntil");<br /></li><li>mLButton.setOnClickListener(e -> skipUntilObserver().subscribe(i -> log("skipUntil:" + i)));<br /></li><li>mRButton.setText("skipWhile");<br /></li><li>mRButton.setOnClickListener(e -> skipWhileObserver().subscribe(i -> log("skipWhile:" + i)));</li></ol>运行结果如下


六、TakeUntil、TakeWhile
TakeUntil和TakeWhile操作符可以说和SkipUnitl和SkipWhile操作符是完全相反的功能。TakeUntil也是使用一个标志Observable是否发射数据来判断,当标志Observable没有发射数据时,正常发射数据,而一旦标志Observable发射过了数据则后面的数据都会被丢弃。TakeWhile则是根据一个函数来判断是否发射数据,当函数返回值为true的时候正常发射数据;当函数返回false的时候丢弃所有后面的数据。


下面使用这两个操作符来take两个Observable发射的数据
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>private Observable<Long> takeUntilObserver() {<br /></li><li>return Observable.interval(1, TimeUnit.SECONDS).takeUntil(Observable.timer(3, TimeUnit.SECONDS));<br /></li><li>}<br /></li><li><br /></li><li>private Observable<Long> takeWhileObserver() {<br /></li><li>return Observable.interval(1, TimeUnit.SECONDS).takeWhile(aLong -> aLong < 5);<br /></li><li>}</li></ol>分别进行订阅
<ol style="margin:0 1px 0 0px;padding-left:40px;" start="1" class="dp-css"><li>mLButton.setText("takeUntil");<br /></li><li>mLButton.setOnClickListener(e -> takeUntilObserver().subscribe(i -> log("takeUntil:" + i)));<br /></li><li>mRButton.setText("takeWhile");<br /></li><li>mRButton.setOnClickListener(e -> takeWhileObserver().subscribe(i -> log("takeWhile:" + i)));</li></ol>运行结果如下

关于条件和布尔操作符就到这了,本文中所有的demo程序见:https://github.com/Chaoba/RxJavaDemo
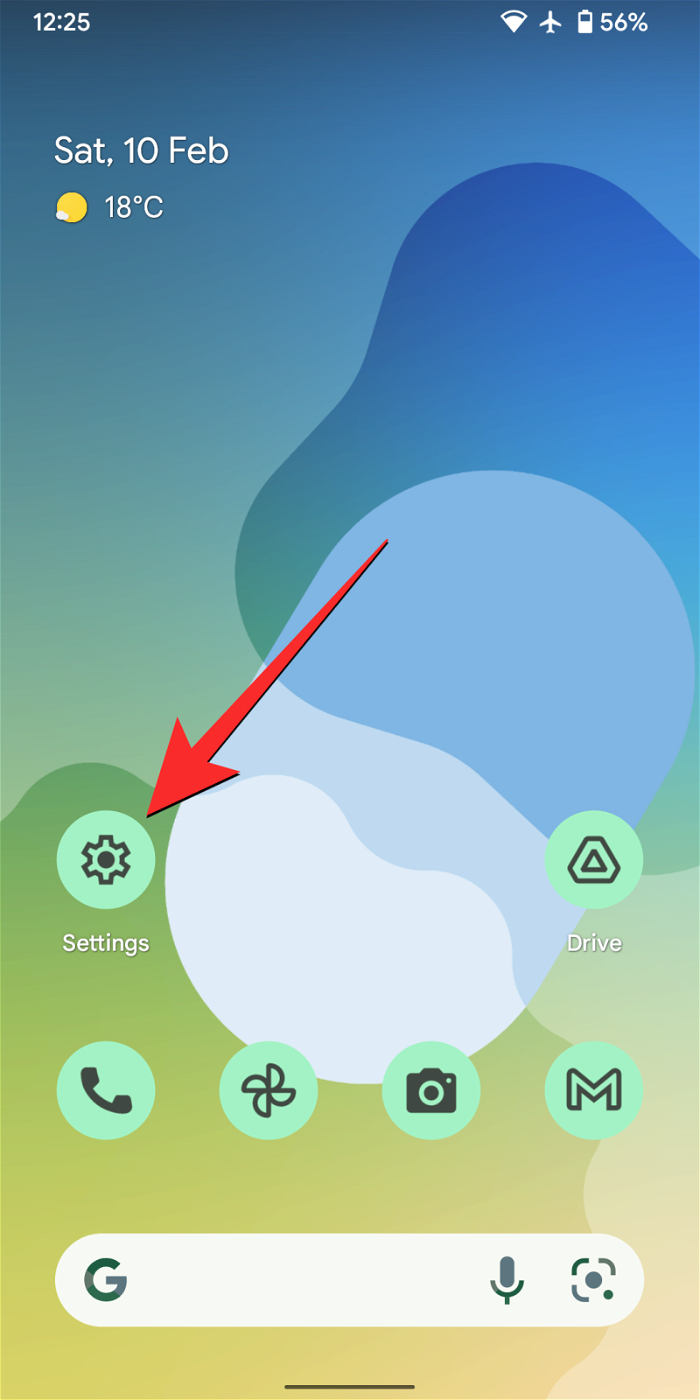
根据美国司法部的解释,蓝色警报旨在提供关于可能对执法人员构成直接和紧急威胁的个人的重要信息。这种警报的目的是及时通知公众,并让他们了解与这些罪犯相关的潜在危险。通过这种主动的方式,蓝色警报有助于增强社区的安全意识,促使人们采取必要的预防措施以保护自己和周围的人。这种警报系统的建立旨在提高对潜在威胁的警觉性,并加强执法机构与公众之间的沟通,以共尽管这些紧急通知对我们社会至关重要,但有时可能会对日常生活造成干扰,尤其是在午夜或重要活动时收到通知时。为了确保安全,我们建议您保持这些通知功能开启,但如果
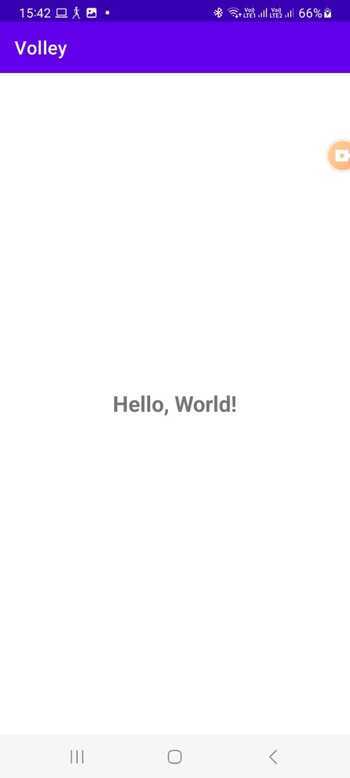
Android中的轮询是一项关键技术,它允许应用程序定期从服务器或数据源检索和更新信息。通过实施轮询,开发人员可以确保实时数据同步并向用户提供最新的内容。它涉及定期向服务器或数据源发送请求并获取最新信息。Android提供了定时器、线程、后台服务等多种机制来高效地完成轮询。这使开发人员能够设计与远程数据源保持同步的响应式动态应用程序。本文探讨了如何在Android中实现轮询。它涵盖了实现此功能所涉及的关键注意事项和步骤。轮询定期检查更新并从服务器或源检索数据的过程在Android中称为轮询。通过
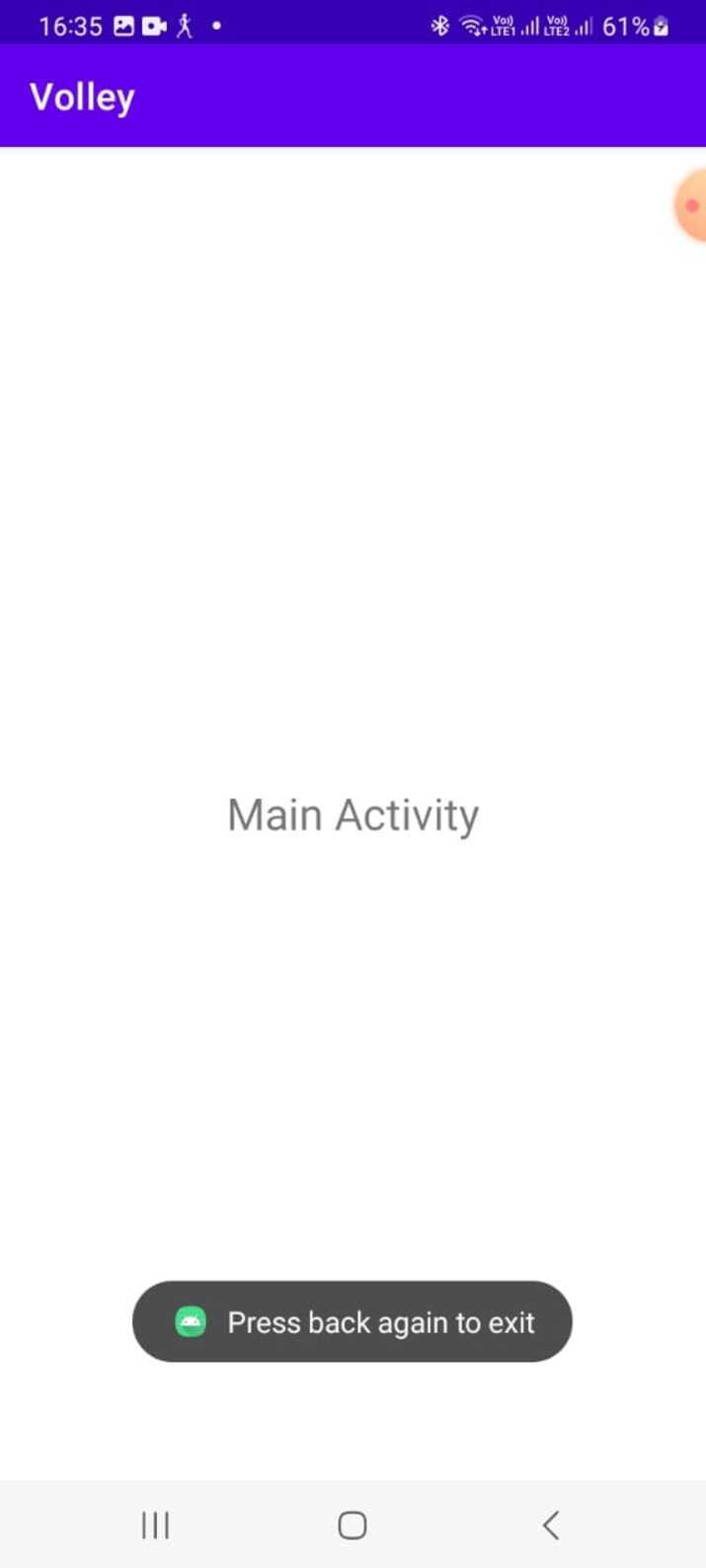
为了提升用户体验并防止数据或进度丢失,Android应用程序开发者必须避免意外退出。他们可以通过加入“再次按返回退出”功能来实现这一点,该功能要求用户在特定时间内连续按两次返回按钮才能退出应用程序。这种实现显著提升了用户参与度和满意度,确保他们不会意外丢失任何重要信息Thisguideexaminesthepracticalstepstoadd"PressBackAgaintoExit"capabilityinAndroid.Itpresentsasystematicguid
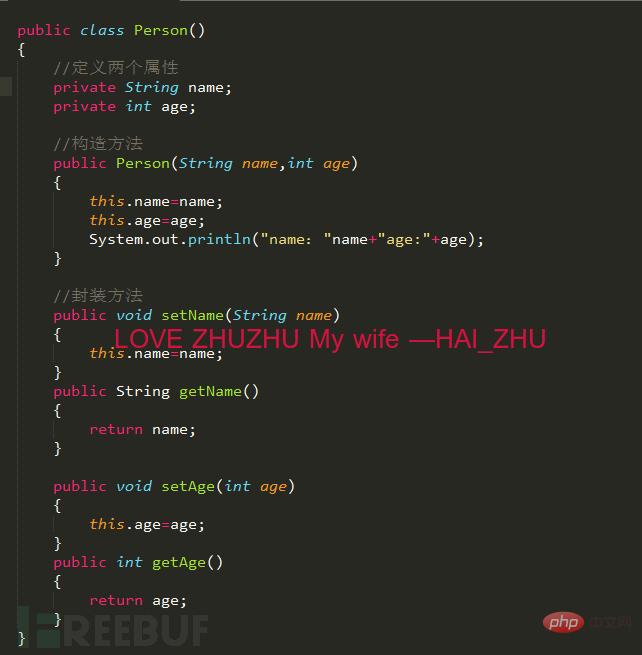
1.java复杂类如果有什么地方不懂,请看:JAVA总纲或者构造方法这里贴代码,很简单没有难度。2.smali代码我们要把java代码转为smali代码,可以参考java转smali我们还是分模块来看。2.1第一个模块——信息模块这个模块就是基本信息,说明了类名等,知道就好对分析帮助不大。2.2第二个模块——构造方法我们来一句一句解析,如果有之前解析重复的地方就不再重复了。但是会提供链接。.methodpublicconstructor(Ljava/lang/String;I)V这一句话分为.m
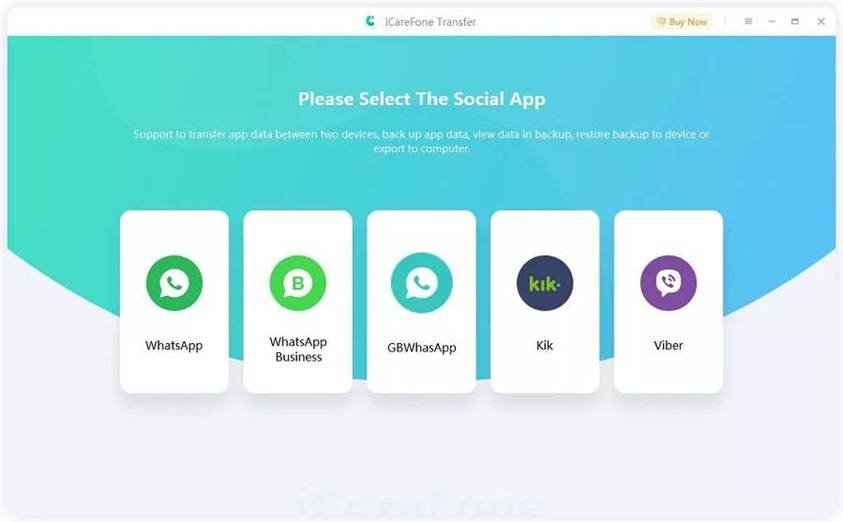
如何将WhatsApp聊天从Android转移到iPhone?你已经拿到了新的iPhone15,并且你正在从Android跳跃?如果是这种情况,您可能还对将WhatsApp从Android转移到iPhone感到好奇。但是,老实说,这有点棘手,因为Android和iPhone的操作系统不兼容。但不要失去希望。这不是什么不可能完成的任务。让我们在本文中讨论几种将WhatsApp从Android转移到iPhone15的方法。因此,坚持到最后以彻底学习解决方案。如何在不删除数据的情况下将WhatsApp
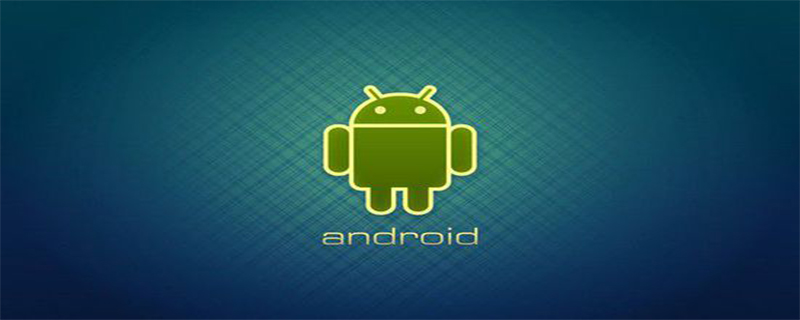
原因:1、安卓系统上设置了一个JAVA虚拟机来支持Java应用程序的运行,而这种虚拟机对硬件的消耗是非常大的;2、手机生产厂商对安卓系统的定制与开发,增加了安卓系统的负担,拖慢其运行速度影响其流畅性;3、应用软件太臃肿,同质化严重,在一定程度上拖慢安卓手机的运行速度。
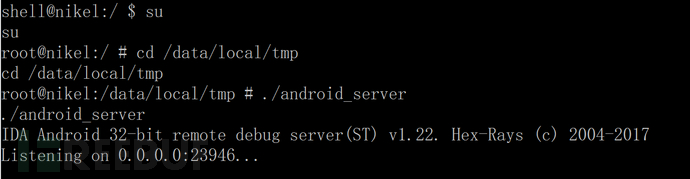
1.启动ida端口监听1.1启动Android_server服务1.2端口转发1.3软件进入调试模式2.ida下断2.1attach附加进程2.2断三项2.3选择进程2.4打开Modules搜索artPS:小知识Android4.4版本之前系统函数在libdvm.soAndroid5.0之后系统函数在libart.so2.5打开Openmemory()函数在libart.so中搜索Openmemory函数并且跟进去。PS:小知识一般来说,系统dex都会在这个函数中进行加载,但是会出现一个问题,后
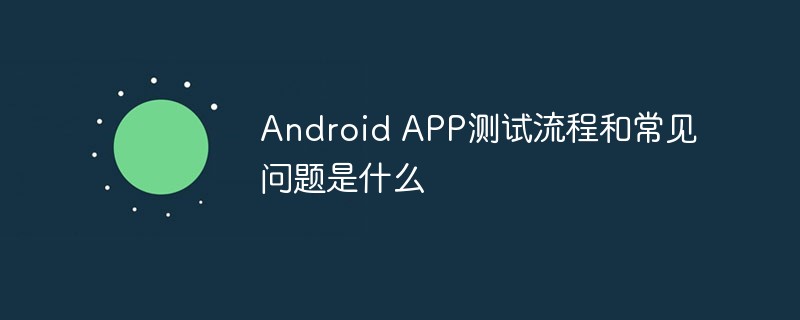
1.自动化测试自动化测试主要包括几个部分,UI功能的自动化测试、接口的自动化测试、其他专项的自动化测试。1.1UI功能自动化测试UI功能的自动化测试,也就是大家常说的自动化测试,主要是基于UI界面进行的自动化测试,通过脚本实现UI功能的点击,替代人工进行自动化测试。这个测试的优势在于对高度重复的界面特性功能测试的测试人力进行有效的释放,利用脚本的执行,实现功能的快速高效回归。但这种测试的不足之处也是显而易见的,主要包括维护成本高,易发生误判,兼容性不足等。因为是基于界面操作,界面的稳定程度便成了


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
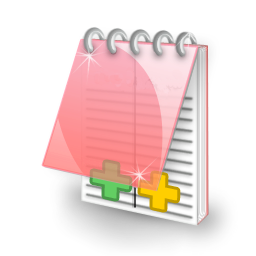
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Chinese version
Chinese version, very easy to use

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
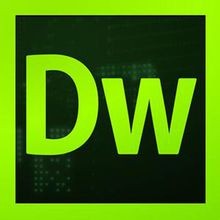
Dreamweaver CS6
Visual web development tools
