


PHP uses Pear to send emails (Windows environment), pear sends emails_PHP tutorial
PHP uses Pear to send emails (Windows environment), pear sends emails
This article introduces the example of PHP using Pear to send emails, and the steps on how to install PHP Pear under Windows , shared with everyone for your reference, the specific content is as follows
1. PEAR installation
1. Introduction to PEAR
PEAR is the abbreviation of the PHP Extension and Application Repository. It is a code repository for PHP extensions and applications. Simply put, PEAR is to PHP what CPAN (Comprehensive Perl Archive Network) is to Perl.
The basic goal of PEAR is to develop into a knowledge base for PHP extension and library code, and the most ambitious goal of the project is to try to define a standard that will help developers write portable, reusable code.
Install PEAR in WAMP integrated environment
1). First download a go-pear.phar file.
2) Enter the running directory of php to install
I use a wamp integrated development environment here. The version is wampserver2.2e-php5.4.3-httpd2.2.22-mysql5.5.24-32b. My local installation path is C:wampbinphpphp5.4.3>
Execute the following command:
php.exe -d phar.require_hash=0 go-pear.phar
At this step, you can modify the $prefix path of PEAR installation. I chose the default and pressed Enter all the way to install.
3). Modify the include_path option
Finally, after the PEAR default extension package is installed, you will be asked whether you need to configure the include_path option in the php.ini file to point to the pear installation directory
Of course it needs to be configured, otherwise it cannot be used, press Y and press Enter, the installation package will automatically modify the include_path option. It reminds us that the environment variables are not set, so we need to set the system environment variables next.
Create a new environment variable name, as shown below
Then add %php_home% to the PATH variable and you’re done~.
2. Common PEAR commands
In command line mode, you can use PEAR related commands to install the PEAR installation package you need.
- pear help Lists all commands of pear, similar to pear's command help command.
- pear help
List the specific information of a pear command - pear help shortcuts List the abbreviations of all pear commands
2. Install Mail, Mail_Mine, Net_SMTP
- Step one: Enter the PHP running directory in cmd, which is php.exe.
- Step 2: Use pear install Mail, pear install Mail_Mine, pear install Net_SMTP to install the class libraries needed to send emails
- Step 3: Use pear list to check whether the above three class libraries are installed.
3. Examples
<?php error_reporting(0); //PHP会出现不规范提示,故此设置 require_once "Mail.php"; //记得将Pear目录加入环境,才能这样引用,否则要引用绝对路径 $from = "sender@outlook.com"; //发送方 $to = "receiver@qq.com"; //接收方 $subject = "Hi!"; //主题 $body = "Hi,\n\nHow are you?"; //内容 $host = "smtp.live.com"; //SMTP服务器 $port = "587"; //端口 // $port = "25"; //两个端口都行 $username = "username@outlook.com";//用户名 $password = "password";//密码 $headers = array ('From' => $from, 'To' => $to, 'Subject' => $subject); //邮件头 $smtp = Mail::factory('smtp', array ('host' => $host, 'port' => $port, 'auth' => true, 'username' => $username, 'password' => $password)); //服务设置 //发送邮件 $mail = $smtp->send($to, $headers, $body); //错误处理 if (PEAR::isError($mail)) { echo("<p>". $mail->getMessage() ."</p>"); } else { echo("<p>Message successfully sent!</p>"); } ?>
4. How to send emails using pear:Net_SMTP class in php
Before using the following source code, please configure the pear path and download the net_smtp package.
Choose different setting methods according to your operating system in the php.ini file:
; UNIX: "/path1:/path2"
include_path = ".:./php/pear"
;
; Windows: "path1;path2"
;include_path = ".;c:phppear"
Code:
<?php require 'Net/SMTP.php'; $host = '126.com';//smtp服务器的ip或域名 $username= 'arcow';//登陆smtp服务器的用户名 $password= 'secret';//登陆smtp服务器的密码 $from = 'arcow@126.com'; //谁发的邮件 $rcpt = array('test@test.com', 'arcow@126.com');//可设多个接收者 $subj = "Subject: 你是谁\n";//主题 $body = "test it";//邮件内容 /* 建立一个类 */ if (! ($smtp = new Net_SMTP($host))) { die("无法初始化类Net_SMTP!\n"); } /* 开始连接SMTP服务器*/ if (PEAR::isError($e = $smtp->connect())) { die($e->getMessage() . "\n"); } /* smtp需要身份验证 */ $smtp->auth($username,$password,"PLAIN"); /*设置发送者邮箱 */ if (PEAR::isError($smtp->mailFrom($from))) { die("无法设置发送者邮箱为 <$from>\n"); } /* 设置接收邮件者 */ foreach ($rcpt as $to) { if (PEAR::isError($res = $smtp->rcptTo($to))) { die("邮件无法投递到 <$to>: " . $res->getMessage() . "\n"); } } /* 开始发送邮件内容 */ if (PEAR::isError($smtp->data($subj . "\r\n" . $body))) { die("Unable to send data\n"); } /* 断开连接 */ $smtp->disconnect(); echo "发送成功!"; ?>
The above is how PHP uses Pear to send emails. I hope this article will be helpful to everyone learning PHP programming.
Articles you may be interested in:
- PHP 5.0 Pear installation method
- php What is PEAR?
- PHP Pear installation and use
- How to install PEAR under windows and solve the error under php5.3.1
- How to install phpunit with pear package
- Install under windows pear and phpunit (pay attention to configuring the php command line environment)
- The difference between PHP extension modules Pecl, Pear and Perl
- How PHP uses pear’s own mail class library to send emails
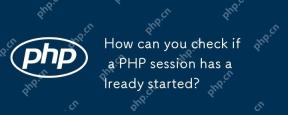
In PHP, you can use session_status() or session_id() to check whether the session has started. 1) Use the session_status() function. If PHP_SESSION_ACTIVE is returned, the session has been started. 2) Use the session_id() function, if a non-empty string is returned, the session has been started. Both methods can effectively check the session state, and choosing which method to use depends on the PHP version and personal preferences.
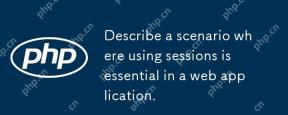
Sessionsarevitalinwebapplications,especiallyfore-commerceplatforms.Theymaintainuserdataacrossrequests,crucialforshoppingcarts,authentication,andpersonalization.InFlask,sessionscanbeimplementedusingsimplecodetomanageuserloginsanddatapersistence.
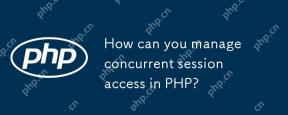
Managing concurrent session access in PHP can be done by the following methods: 1. Use the database to store session data, 2. Use Redis or Memcached, 3. Implement a session locking strategy. These methods help ensure data consistency and improve concurrency performance.
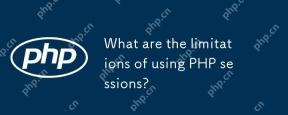
PHPsessionshaveseverallimitations:1)Storageconstraintscanleadtoperformanceissues;2)Securityvulnerabilitieslikesessionfixationattacksexist;3)Scalabilityischallengingduetoserver-specificstorage;4)Sessionexpirationmanagementcanbeproblematic;5)Datapersis
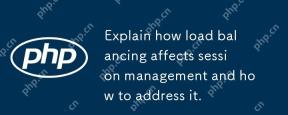
Load balancing affects session management, but can be resolved with session replication, session stickiness, and centralized session storage. 1. Session Replication Copy session data between servers. 2. Session stickiness directs user requests to the same server. 3. Centralized session storage uses independent servers such as Redis to store session data to ensure data sharing.
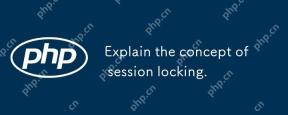
Sessionlockingisatechniqueusedtoensureauser'ssessionremainsexclusivetooneuseratatime.Itiscrucialforpreventingdatacorruptionandsecuritybreachesinmulti-userapplications.Sessionlockingisimplementedusingserver-sidelockingmechanisms,suchasReentrantLockinJ
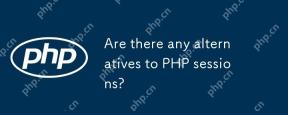
Alternatives to PHP sessions include Cookies, Token-based Authentication, Database-based Sessions, and Redis/Memcached. 1.Cookies manage sessions by storing data on the client, which is simple but low in security. 2.Token-based Authentication uses tokens to verify users, which is highly secure but requires additional logic. 3.Database-basedSessions stores data in the database, which has good scalability but may affect performance. 4. Redis/Memcached uses distributed cache to improve performance and scalability, but requires additional matching
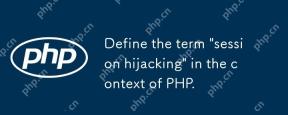
Sessionhijacking refers to an attacker impersonating a user by obtaining the user's sessionID. Prevention methods include: 1) encrypting communication using HTTPS; 2) verifying the source of the sessionID; 3) using a secure sessionID generation algorithm; 4) regularly updating the sessionID.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
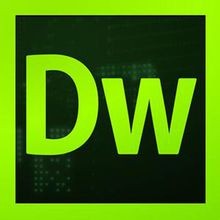
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
