


Using gzip to compress and output the JSON format data returned in the PHP program, jsongzip
1. Comparison of HTTP output using compression and without compression
2. Enable gzip
Use apache mod_deflate module to enable gzip
How to activate:
sudo a2enmod deflate sudo /etc/init.d/apache2 restart
Close method:
sudo a2dismod deflate sudo /etc/init.d/apache2 restart
3. Set the type of gzip compressed output
The output type of json is application/json, so it can be set like this
Add
<IfModule mod_deflate.c> AddOutputFilterByType DEFLATE application/json </IfModule>
<?php $data = array( array('name'=>'one','value'=>1), array('name'=>'two','value'=>2), array('name'=>'three','value'=>3), array('name'=>'four','value'=>4), array('name'=>'five','value'=>5), array('name'=>'six','value'=>6), array('name'=>'seven','value'=>7), array('name'=>'eight','value'=>8), array('name'=>'nine','value'=>9), array('name'=>'ten','value'=>10), ); header('content-type:application/json'); echo json_encode($data); ?>
Output before setting gzip:
Output after setting gzip:
4. A single json is output using gzip compression
After setting AddOutputFilterByType DEFLATE application/json, all data output in json format will be output using gzip compression.
If you only want to use gzip compression for a certain json, but not others, you can use the ob_start(); method.
First there is no need to set AddOutputFilterByType, then add ob_start('ob_gzhandler');
at the beginning of the code<?php ob_start('ob_gzhandler'); $data = array( array('name'=>'one','value'=>1), array('name'=>'two','value'=>2), array('name'=>'three','value'=>3), array('name'=>'four','value'=>4), array('name'=>'five','value'=>5), array('name'=>'six','value'=>6), array('name'=>'seven','value'=>7), array('name'=>'eight','value'=>8), array('name'=>'nine','value'=>9), array('name'=>'ten','value'=>10), ); header('content-type:application/json'); echo json_encode($data); ?>
Articles you may be interested in:
- jQuery sends a request to the PHP server through Ajax and returns JSON data
- php simply implements querying the database and returning json data
- PHP implements class sharing for returning JSON and XML
- ThinkPHP has two implementation methods for returning JSON through AJAX
- php json_encode() function returns json data instance code
- php Return json data function instance
- Solution for PHP to process Json string decoding and return NULL
- Ajax processing PHP to return json data instance code
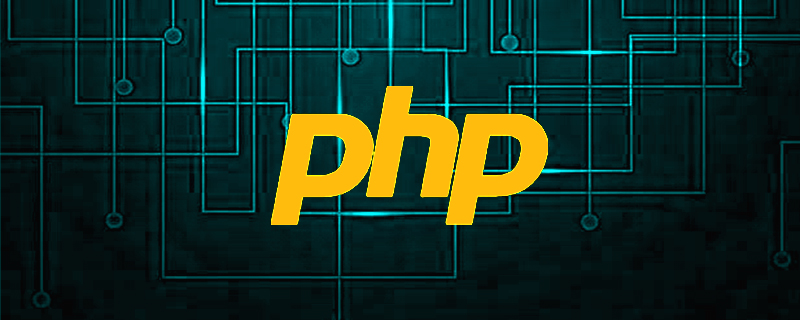
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
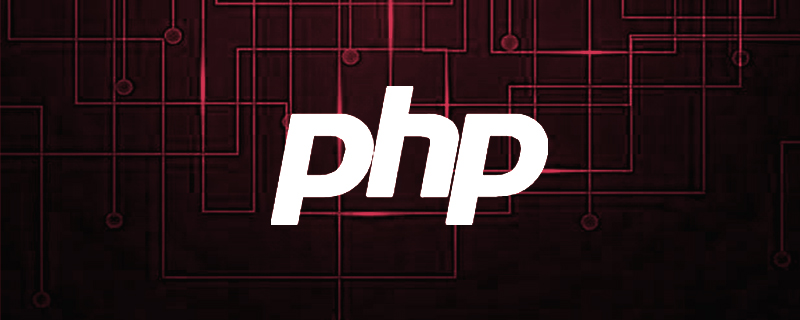
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
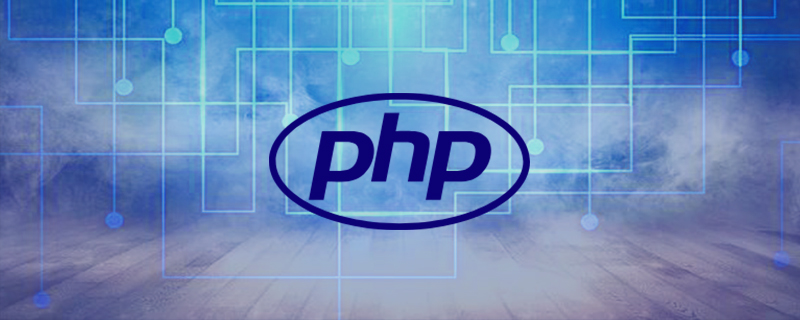
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
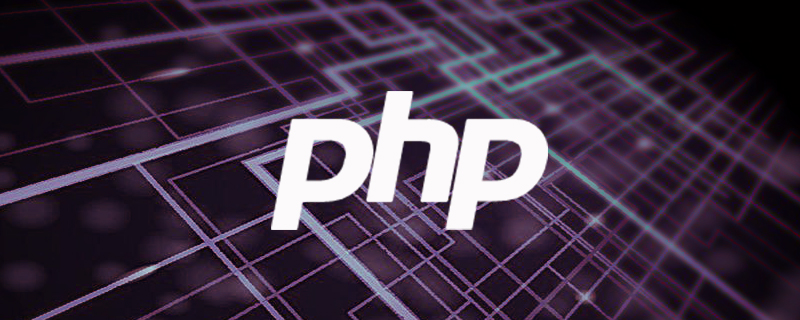
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
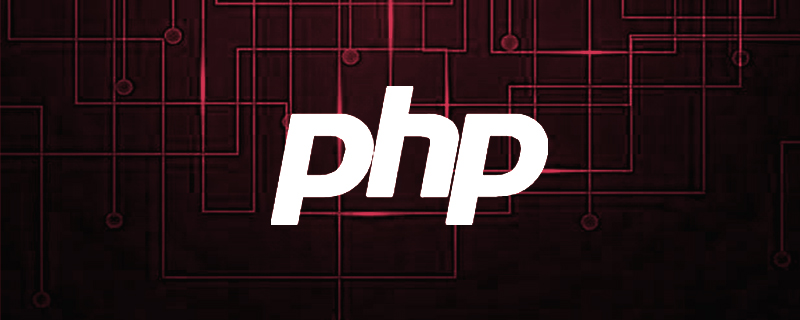
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
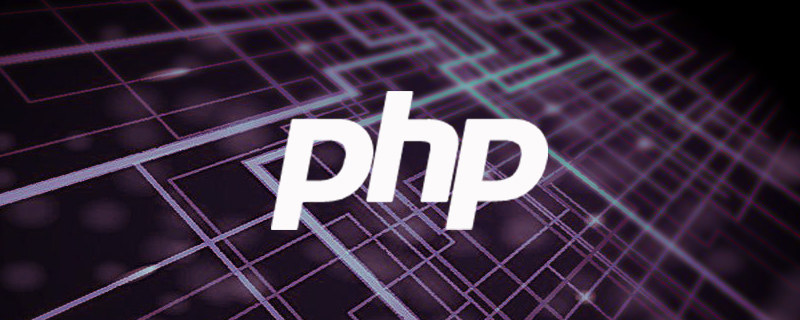
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
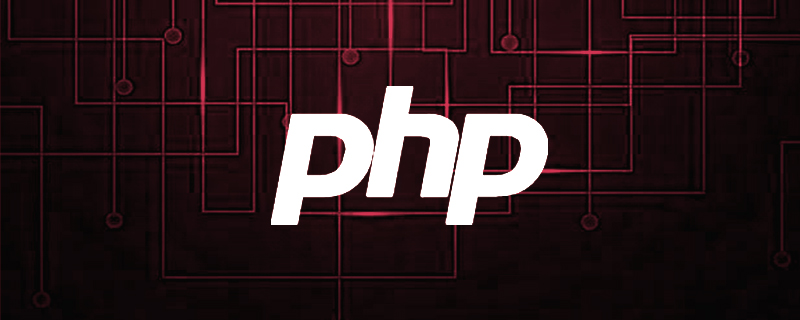
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
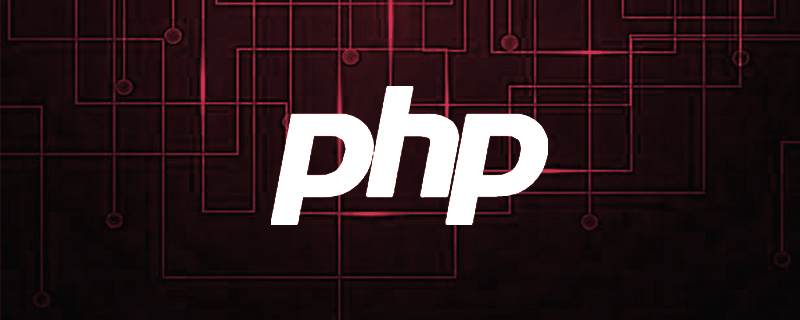
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
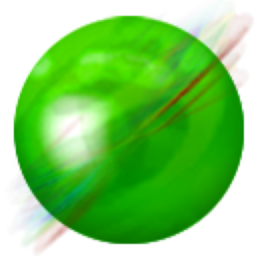
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
