


Each product has an id, title, and price. Every time I click to buy, I add it to the array. However, I want to judge based on the Id. When adding a product to the shopping cart repeatedly, add the same product to an array. How to write a dimensional array?
<code>[ [ {鸡腿},{鸡腿},{鸡腿},{鸡腿} ], [ {狗腿},{狗腿},{狗腿} ], [ {猫咪},{猫咪},{猫咪},{猫咪},{猫咪},{猫咪} ] ] </code>
Thank you everyone, it’s true that my idea is really stupid, thank you for your suggestions!
Reply content:
Each product has an id, title, and price. Every time I click to buy, I add it to the array. However, I want to judge based on the Id. When adding a product to the shopping cart repeatedly, add the same product to an array. How to write a dimensional array?
<code>[ [ {鸡腿},{鸡腿},{鸡腿},{鸡腿} ], [ {狗腿},{狗腿},{狗腿} ], [ {猫咪},{猫咪},{猫咪},{猫咪},{猫咪},{猫咪} ] ] </code>
Thank you everyone, it’s true that my idea is really stupid, thank you for your suggestions!
The friend above is right, your idea is not advisable. Two-dimensional arrays are all the same thing. . A bit of a trap.
This format is better, please refer to it
<code>var cart = { 'id01':{n:'鸡腿', count: 4}, 'id02':{n:'鸭腿', count: 3}, 'id03':{n:'猪腿', count: 2}, 'id04':{n:'狗腿子', count: 1} } </code>
However, if you insist on doing it this way
<code>var list = [ [{n:'鸡腿'},{n:'鸡腿'},{n:'鸡腿'},{n:'鸡腿'},{n:'鸡腿'}], [{n:'鸭腿'},{n:'鸭腿'},{n:'鸭腿'},{n:'鸭腿'}], [{n:'猪腿'},{n:'猪腿'},{n:'猪腿'},{n:'猪腿'}] ]; function fn(o){ var inArray = false; list.map(function(item){ if( item.indexOf(o) > -1){ inArray = true; item.push(o); } }); inArray || list.push([o]); } fn(list[1][1]); fn({n:'狗腿子'}); </code>
This is just a reference and is not recommended
It is not recommended to write like this. The data structure of the shopping cart should store the product ID and quantity (assuming that the product ID here is the name)
<code>{ "鸡腿": 4, "狗腿": 3, "猫咪": 6 } </code>
In actual implementation, when adding or subtracting items in the shopping cart, you only need to add or subtract the following number
Your thinking is wrong. What you said above is correct. The main body of the shopping cart should be $a = ['id'=>number]
, then the price and name should be another array $b = ['id'=>['name'=>name,'price'=>price]]
, and the total price is $totalPrice = $a['id']*$b['id']['price']
I agree with the point above that the items in the shopping cart should be as a whole, but I personally feel that the price should not be stored in the shopping cart array, because the price when you add it and the price when you pay are not necessarily the same. What should be stored is the unique identifier id and quantity number. If you store title, price, if the merchant changes the name or price, how should your design be handled?
<code>$shoppingCart = [ '101' => 4,//鸡腿 '102' => 5,//狗腿 '103' => 6//鸭腿 ];</code>
First of all, thank you for the invitation.
Actually, I saw this question yesterday, and I think the answers above were pretty good. But seeing that the author of the question invited me again, I can only express my opinion as a way to attract others.
Actually, I think the number of dimensions of the array is not important. It is not important how to write it. What is important is the idea. I mainly want to make an introductory statement on this aspect.
In fact, in this day and age, although object-oriented is a commonplace thing, many people still don’t know how to use it, so the complexity of the problem has skyrocketed. Let’s try to use object-oriented to solve this problem:
//全局对象 var item_arr = {}; //操作函数 function add_(id, name, price, count) { var item = { id: id, name: name, price: price, count: count } var obj = item_arr[id] if (obj) { item.count = obj.count + count; } item_arr[item.id] = item; } //code by rozbo ,强力免山寨 //模拟添加购物操作 add_(19, "狗腿子", 16, 20); add_(1, "鸡腿子", 12, 2); add_(126, "羊腿子", 6, 6); add_(126, "羊腿子", 6, 6); //输出信息,计算价格 var price_totle = 0; for (var id in item_arr) { var item = item_arr[id]; var price_curr = item.count * item.price; price_totle += price_curr; console.info("当前有%s%d个,总价%d元", item.name, item.count, price_curr); } console.info("共计%d元,祝您购物愉快!", price_totle);
Output results
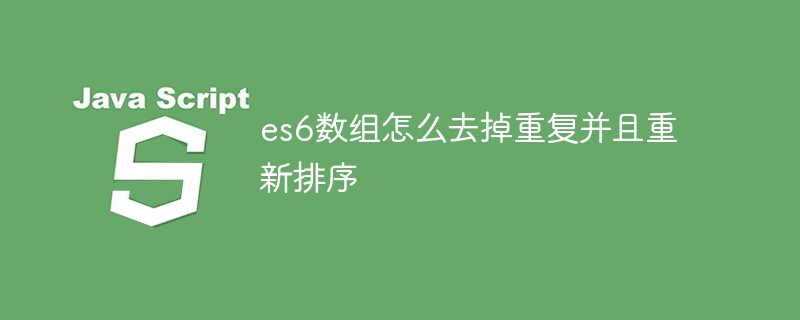
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
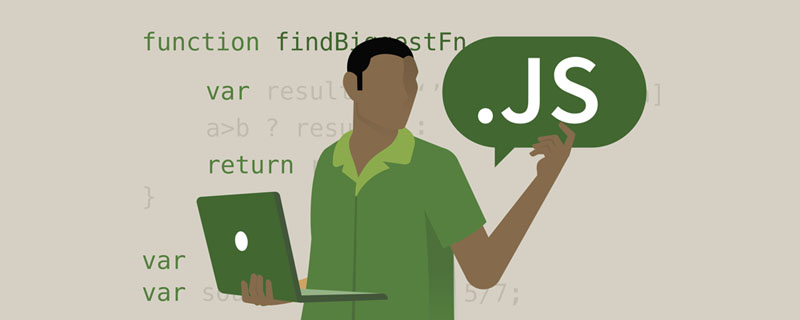
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
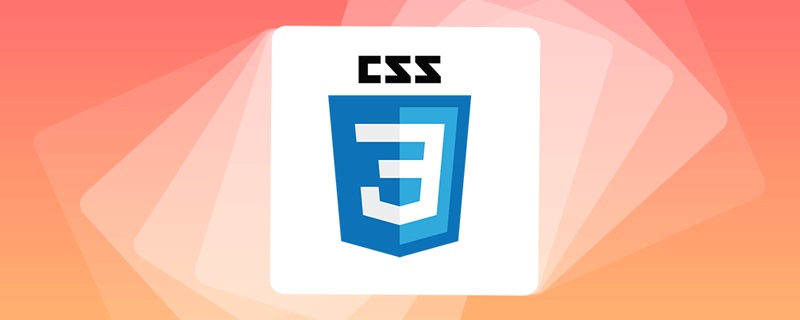
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
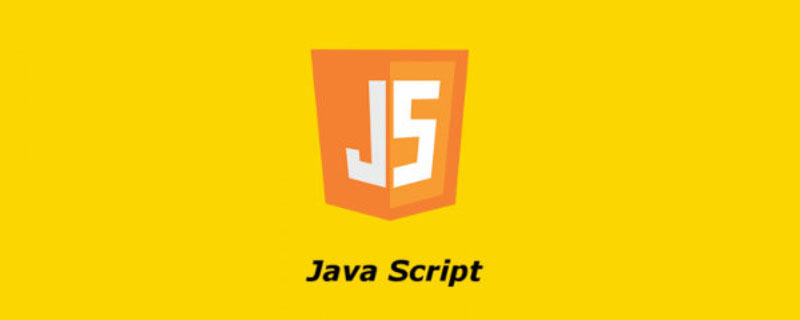
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
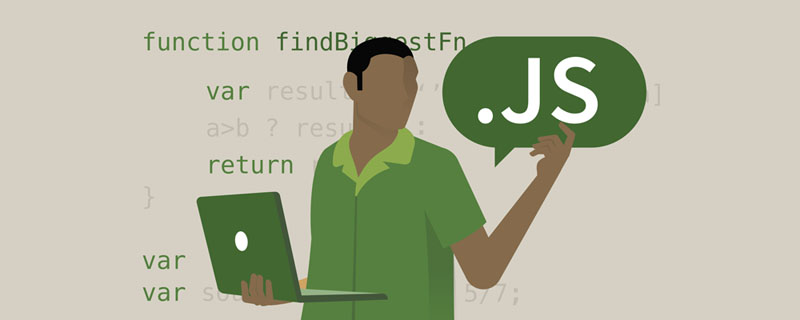
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
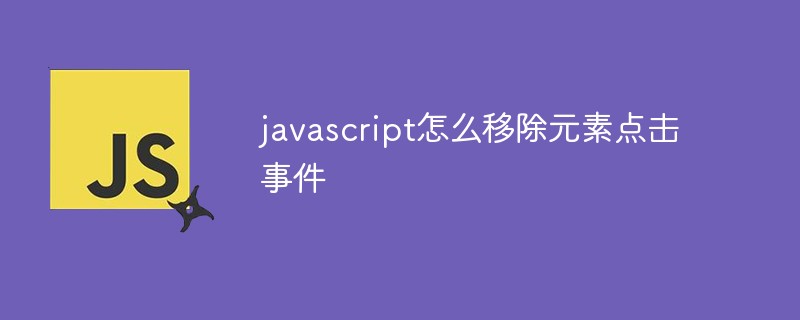
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
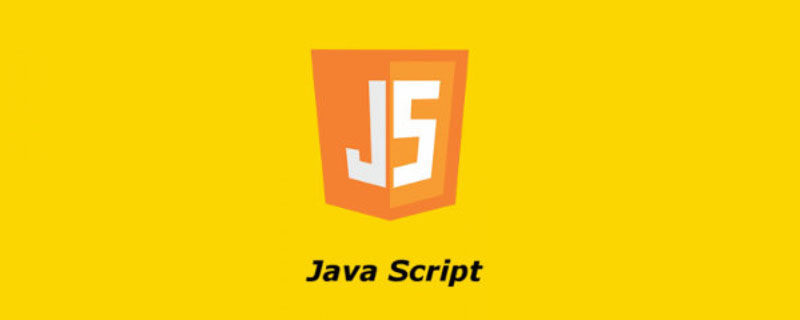
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
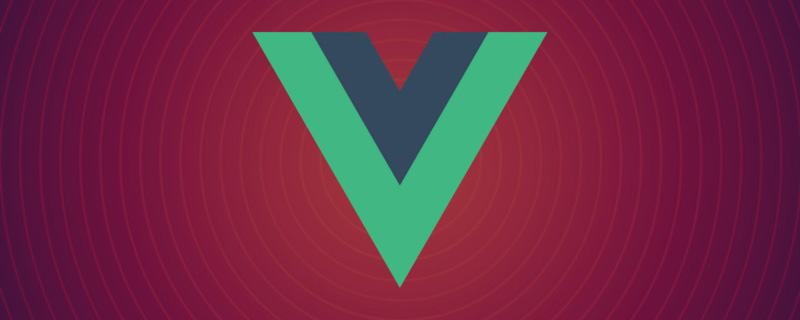
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
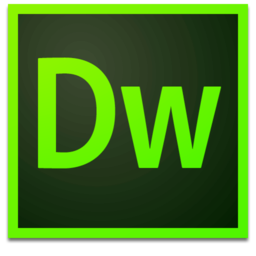
Dreamweaver Mac version
Visual web development tools
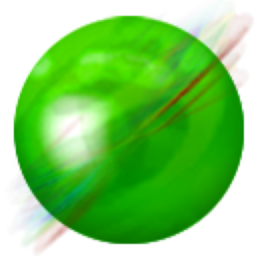
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
