The existing array is as follows:
[site003] => Array
<code> ( [0] => Array ( [key] => site003 [riqi] => 2016-06-14 [shijian] => 00 [num] => 1 ) [1] => Array ( [key] => site003 [riqi] => 2016-06-14 [shijian] => 04 [num] => 2 ) [2] => Array ( [key] => site003 [riqi] => 2016-06-14 [shijian] => 07 [num] => 6 ) )</code>
Since there are no 01, 02, and 03 time points in the time shijian field from 00 to -04, I want to supplement the num field of the assembled data at 01, 02, and 03 time points with 0,
The latter is between 04-07 At time points 05 and 06, the time point of num is also added to the array as 0
Please give some guidance from experts! Thank you, brother!
Reply content:
The existing array is as follows:
[site003] => Array
<code> ( [0] => Array ( [key] => site003 [riqi] => 2016-06-14 [shijian] => 00 [num] => 1 ) [1] => Array ( [key] => site003 [riqi] => 2016-06-14 [shijian] => 04 [num] => 2 ) [2] => Array ( [key] => site003 [riqi] => 2016-06-14 [shijian] => 07 [num] => 6 ) )</code>
Since there are no 01, 02, and 03 time points in the time shijian field from 00 to -04, I want to supplement the num field of the assembled data at 01, 02, and 03 time points with 0,
The latter is between 04-07 At time points 05 and 06, the time point of num is also added to the array as 0
Please give some guidance from experts! Thank you, brother!
First of all, you need to determine whether you need to insert relevant data and the plan of the data you insert. If you are not sure about the beginning and end of the missing shijian field, you need to traverse the array to determine it, and then add the missing fields, and then Use usort to sort.
You can also determine the position of your insertion when inserting, but since your insertion will affect the subscript of your array, the first method above is more convenient.
Reference Code
<?php $max_shijian = date("H"); // 填充主方法 $fill_date = function ($input,$key) use($max_shijian) { $hours = range(0,$max_shijian); $riqi = null; // 筛选出不存在的时间 // http://php.net/manual/zh/function.array-map.php array_map(function(&$item,$key) use(&$hours,&$riqi){ empty($riqi) and $riqi = $item['riqi']; unset($hours[intval($item['shijian'])]); },$input); // 填充不存在的时间 foreach ($hours as $hour) { $input[] = [ 'key' => $key, 'riqi' => $riqi, 'shijian' => getFullHour($hour), 'num' => 0, ]; } // 排序 // http://php.net/manual/zh/function.usort.php usort($input,function($a,$b){ return (intval($a['shijian'])<intval($b['shijian']))?-1:1; }); return $input; }; // 将小时补全为2位 function getFullHour($hour){ return (strlen($hour)==1)?("0".$hour):$hour; } $json = '{"site001":[{"key":"site001","riqi":"2016-06-14","shijian":"00","num":"10"},{"key":"site001","riqi":"2016-06-14","shijian":"01","num":"4"},{"key":"site001","riqi":"2016-06-14","shijian":"02","num":"1"},{"key":"site001","riqi":"2016-06-14","shijian":"03","num":"3"},{"key":"site001","riqi":"2016-06-14","shijian":"04","num":"2"},{"key":"site001","riqi":"2016-06-14","shijian":"05","num":"1"},{"key":"site001","riqi":"2016-06-14","shijian":"07","num":"9"},{"key":"site001","riqi":"2016-06-14","shijian":"08","num":"2"}],"site002":[{"key":"site002","riqi":"2016-06-14","shijian":"00","num":"3"},{"key":"site002","riqi":"2016-06-14","shijian":"01","num":"13"},{"key":"site002","riqi":"2016-06-14","shijian":"02","num":"8"},{"key":"site002","riqi":"2016-06-14","shijian":"03","num":"23"},{"key":"site002","riqi":"2016-06-14","shijian":"04","num":"14"},{"key":"site002","riqi":"2016-06-14","shijian":"05","num":"6"},{"key":"site002","riqi":"2016-06-14","shijian":"06","num":"4"},{"key":"site002","riqi":"2016-06-14","shijian":"07","num":"7"},{"key":"site002","riqi":"2016-06-14","shijian":"08","num":"18"},{"key":"site002","riqi":"2016-06-14","shijian":"09","num":"6"}],"site003":[{"key":"site003","riqi":"2016-06-14","shijian":"00","num":"1"},{"key":"site003","riqi":"2016-06-14","shijian":"04","num":"2"},{"key":"site003","riqi":"2016-06-14","shijian":"07","num":"6"}],"site004":[{"key":"site004","riqi":"2016-06-14","shijian":"00","num":"3"},{"key":"site004","riqi":"2016-06-14","shijian":"02","num":"1"},{"key":"site004","riqi":"2016-06-14","shijian":"03","num":"4"},{"key":"site004","riqi":"2016-06-14","shijian":"04","num":"7"},{"key":"site004","riqi":"2016-06-14","shijian":"05","num":"4"},{"key":"site004","riqi":"2016-06-14","shijian":"06","num":"2"}]}'; $data = json_decode($json,true); foreach ($data as $key => $value) { $data[$key] = call_user_func($fill_date,$value,$key); } print_r($data); ?>
array_map
usort
Let me talk about the idea I understand. First, you must first take the maximum value of the shijian
field in your array, and then fill it in a loop based on the maximum value.
You try the following method,
The main ideas are:
1. First filter out the largest shijian value from the given array, and store the existing time value at the same time,
2. Add data that is less than the maximum shijian value and does not exist in the array
<code> $srcArray = array (array( 'key' => 'site003', 'riqi'=> '2016-06-14', 'shijian' => 00, 'num' => 1, ) ,array ( 'key' => 'site003', 'riqi' => '2016-06-14', 'shijian' => 02, 'num'=> 2 ) ,array ( 'key' => 'site003', 'riqi' => '2016-06-14', 'shijian' => 04, 'num'=> 2 ) ); $data=array(-1); foreach($srcArray as $key=>$innerArray){ array_push($data,$innerArray['shijian']); if($innerArray['shijian'] > $data[0]){ $data[0] = intval($innerArray['shijian']); } } print_r($data); for($index = 0;$index 'site003', 'riqi'=> '2016-06-14', 'shijian' => $index 0, ); array_push($srcArray,$temp); } } print_r($srcArray);</code>
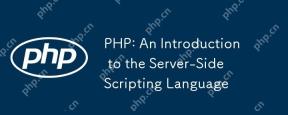
PHP is a server-side scripting language used for dynamic web development and server-side applications. 1.PHP is an interpreted language that does not require compilation and is suitable for rapid development. 2. PHP code is embedded in HTML, making it easy to develop web pages. 3. PHP processes server-side logic, generates HTML output, and supports user interaction and data processing. 4. PHP can interact with the database, process form submission, and execute server-side tasks.
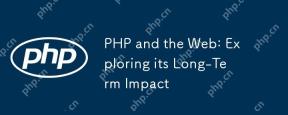
PHP has shaped the network over the past few decades and will continue to play an important role in web development. 1) PHP originated in 1994 and has become the first choice for developers due to its ease of use and seamless integration with MySQL. 2) Its core functions include generating dynamic content and integrating with the database, allowing the website to be updated in real time and displayed in personalized manner. 3) The wide application and ecosystem of PHP have driven its long-term impact, but it also faces version updates and security challenges. 4) Performance improvements in recent years, such as the release of PHP7, enable it to compete with modern languages. 5) In the future, PHP needs to deal with new challenges such as containerization and microservices, but its flexibility and active community make it adaptable.
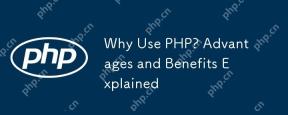
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
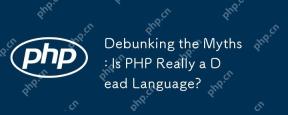
PHP is not dead. 1) The PHP community actively solves performance and security issues, and PHP7.x improves performance. 2) PHP is suitable for modern web development and is widely used in large websites. 3) PHP is easy to learn and the server performs well, but the type system is not as strict as static languages. 4) PHP is still important in the fields of content management and e-commerce, and the ecosystem continues to evolve. 5) Optimize performance through OPcache and APC, and use OOP and design patterns to improve code quality.
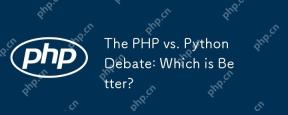
PHP and Python have their own advantages and disadvantages, and the choice depends on the project requirements. 1) PHP is suitable for web development, easy to learn, rich community resources, but the syntax is not modern enough, and performance and security need to be paid attention to. 2) Python is suitable for data science and machine learning, with concise syntax and easy to learn, but there are bottlenecks in execution speed and memory management.
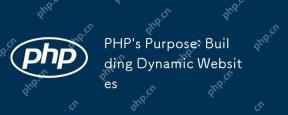
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.

PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
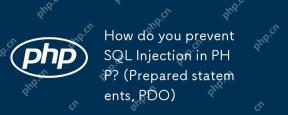
Using preprocessing statements and PDO in PHP can effectively prevent SQL injection attacks. 1) Use PDO to connect to the database and set the error mode. 2) Create preprocessing statements through the prepare method and pass data using placeholders and execute methods. 3) Process query results and ensure the security and performance of the code.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
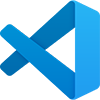
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

Atom editor mac version download
The most popular open source editor

SublimeText3 Chinese version
Chinese version, very easy to use