Refactoring is the process of improving code after it is written by changing its internal structure without changing its external behavior. 1. When refactoring is needed 1. There are duplicate codes in the code; if there are duplicate code blocks in the class, it needs to be refined into a new independent method. If there is the same code in different classes, it should be refined into A new class. 2
Refactoring is the process of improving code after it is written by changing its internal structure without changing its external behavior.
1. When is reconstruction necessary
1. There are duplicate codes in the code;
If there is a duplicate code block in the class, it needs to be refined into a new independent method. If there is the same code in different classes, it should be refined into a new class.
2. Overly large classes and overly long methods;
Overly large classes are often the result of unreasonable class abstraction. Unreasonable class abstraction will reduce the code reuse rate. When you see a method that is too long, you need to find a way to divide it into multiple smaller methods.
The more lines of code in a method, the harder it is to understand. We recommend only 20-25 lines of code per method. But some people say rows 1-10 are more reasonable, this is just personal preference, there are no hard and fast rules. Extraction methods are one of the most common refactoring methods. If you find that a method is too long, or already needs a comment to describe its purpose, then you can apply the extraction method. People always ask how long a method should be, but length is not the root of the problem. When you're dealing with complex methods, keeping track of all the local variables is the most complex and time-consuming, and you can save some time by extracting a method. You can use Visual Studio to extract methods, which will help you track local variables and pass them to new methods or receive the return value of a method.
3. Modifications that affect the whole body in one move;
When you modify a small function or add a small function, it triggers a code earthquake. It may be caused by your design abstraction being not ideal enough and the functional code being too scattered.
4. Too much communication is required between classes;
Class A needs to call too many methods of class B to access the internal data of B. Shouldn't the two classes be separated at all?
5. Overcoupled information chain;
Middle layers are often used in code to achieve the purpose of loose coupling. However, if there are too many middle layers and they are connected layer by layer, do you need to consider reducing the number of middle layers?
6. Classes or methods with similar functions;
7. Imperfect design;
8. Lack of necessary comments;
9. Always control the size of the class
Oversized classes are trying to do too many things, which violates the Single Responsibility Principle (SRP), the S in object-oriented design principle SOLID.
Why must we separate the two responsibilities into separate classes? Because every responsibility is the center of change. When requirements change, the change will appear in the class responsible for that responsibility. If a class takes on multiple responsibilities, there will be more than one reason for its change. If a class has multiple responsibilities, these responsibilities are coupled together. And changes to a certain responsibility may weaken or limit the ability of this class to meet other responsibilities. This coupling will lead to a very fragile design, which may be broken unexpectedly when responsibilities change.
The following two items can be classified as rewriting and code specification issues.
10. Avoid too many parameters
Replace multiple parameters by declaring a class. Create a class to contain all parameters. Generally speaking, this is a better design, and this abstraction is very valuable.
11. Avoid complex expressions
Complex expressions mean that there is some hidden meaning behind them. We can use attributes to encapsulate these expressions to make the code more readable.
<span style="color: #0000ff;">if</span>(PRoduct.Price><span style="color: #800080;">500</span> && !product.IsDeleted && !product.IsFeatured &&<span style="color: #000000;"> product.IsExported) { </span><span style="color: #008000;">//</span><span style="color: #008000;"> do something</span> }
2. Several reconstruction points in C# VS2010
1. Extraction method reconstruction
2. Rename and reconstruct
3. Encapsulation field reconstruction
4. Extraction interface reconstruction
5. Remove parameter reconstruction
6. Rearrange parameters and reconstruct

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
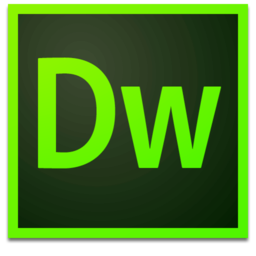
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.