


Summary of some commonly used add, delete, and insert operation functions for arrays in PHP_php skills
Sometimes we need to expand an array or delete a part of the array. PHP provides some functions for expanding and shrinking arrays. These functions provide convenience for programmers who wish to emulate various queue implementations (FIFO, LIFO). As the name suggests, the function names of these functions (push, pop, shift, and unshift) clearly reflect their functions.
PS: The traditional queue is a data structure. The order of deleting elements and adding elements is the same, which is called first-in-first-out, or FIFO. In contrast, a stack is another data structure in which elements are removed in the reverse order in which they were added. This becomes last-in-first-out, or LIFO.
Add elements to the head of the array
The array_unshift() function adds elements to the head of the array. All existing numeric keys are modified to reflect their new positions in the array, but associated keys are not affected. Its form is as follows:
int array_unshift(array array,mixed variable[,mixed variable])
The following example adds two fruits in front of the $fruits array:
$fruits = array("apple","banana"); array_unshift($fruits,"orange","pear") // $fruits = array("orange","pear","apple","banana");
Add elements to the end of the array
The return value of the array_push() function is of type int, which is the number of elements in the array after pushing the data. You can pass multiple variables as parameters to this function and push multiple variables into the array at the same time. Its form is:
(array array,mixed variable [,mixed variable...])
The following example adds two more fruits to the $fruits array:
$fruits = array("apple","banana"); array_push($fruits,"orange","pear") //$fruits = array("apple","banana","orange","pear")
Delete value from array head
The array_shift() function removes and returns the element found in the array. The result is that if numeric keys are used, all corresponding values are shifted down, while arrays using associative keys are not affected. Its form is:
mixed array_shift(array array)
The following example deletes the first element apple in the $fruits array:
$fruits = array("apple","banana","orange","pear"); $fruit = array_shift($fruits); // $fruits = array("banana","orange","pear") // $fruit = "apple";
Delete elements from the end of the array
The array_pop() function removes and returns the last element of the array. Its form is:
mixed array_pop(aray target_array);
The following example removes the last state from the $states array:
$fruits = array("apple","banana","orange","pear"); $fruit = array_pop($fruits); //$fruits = array("apple","banana","orange"); //$fruit = "pear";
Finding, filtering and searching array elements are some common functions of array operations. Here are some related functions.
in_array() function
The in_array() function searches for a specific value in an array and returns true if the value is found, otherwise it returns false. Its form is as follows:
boolean in_array(mixed needle,array haystack[,boolean strict]);
Let’s look at the following example to find whether the variable apple is already in the array. If it is, output a piece of information:
$fruit = "apple"; $fruits = array("apple","banana","orange","pear"); if( in_array($fruit,$fruits) )
echo "$fruit is already in the array";
The third argument is optional and forces in_array() to consider types when searching.
array_key_exists() function
If a specified key is found in an array, the function array_key_exists() returns true, otherwise it returns false. Its form is as follows:
boolean array_key_exists(mixed key,array array);
The following example will search for apple in the array key, and if found, will output the color of the fruit:
$fruit["apple"] = "red"; $fruit["banana"] = "yellow"; $fruit["pear"] = "green"; if(array_key_exists("apple", $fruit)){ printf("apple's color is %s",$fruit["apple"]); }
The result of executing this code:
apple's color is red
array_search() function
The array_search() function searches for a specified value in an array and returns the corresponding key if found, otherwise it returns false. Its form is as follows:
mixed array_search(mixed needle,array haystack[,boolean strict])
The following example searches $fruits for a specific date (December 7), and if found, returns information about the corresponding state:
$fruits["apple"] = "red"; $fruits["banana"] = "yellow"; $fruits["watermelon"]="green"; $founded = array_search("green", $fruits); if($founded) printf("%s was founded on %s.",$founded, $fruits[$founded])
The results of running the program are as follows:
watermelon was founded on green.
array_keys() function
The array_keys() function returns an array containing all keys found in the searched array. Its form is as follows:
array array_keys(array array[,mixed search_value])
If the optional parameter search_value is included, only keys matching that value will be returned. The following example will output all arrays found in the $fruit array:
$fruits["apple"] = "red"; $fruits["banana"] = "yellow"; $fruits["watermelon"]="green"; $keys = array_keys($fruits); print_r($keys);
The results of running the program are as follows:
Array ( [0] => apple [1] => banana [2] => watermelon )
array_values() function
The array_values() function returns all the values in an array and automatically provides a numerical index for the returned array. Its form is as follows:
array array_values(array array)
The following example will get the value of each element found in $fruits:
$fruits["apple"] = "red"; $fruits["banana"] = "yellow"; $fruits["watermelon"]="green"; $values = array_values($fruits); print_r($values);
The results of running the program are as follows:
Array ( [0] => red [1] => yellow [2] => green )
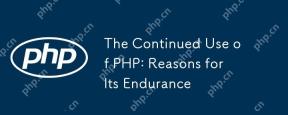
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
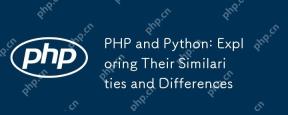
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
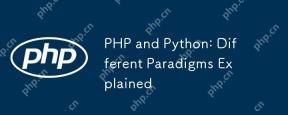
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
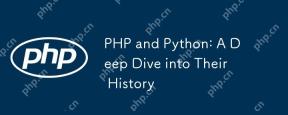
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
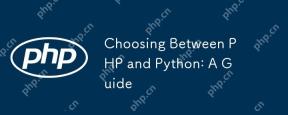
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
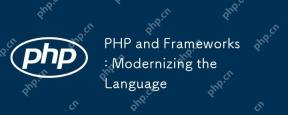
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
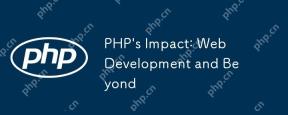
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
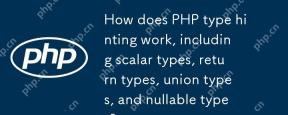
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
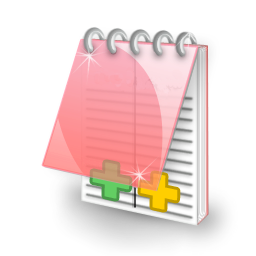
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool