Javascript implements form verification_javascript skills
The example in this article explains the detailed code of javascript to implement form verification, and shares it with everyone for your reference. The specific content is as follows
Rendering:
Specific code:
<html> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>无标题文档</title> </head> <script type="text/javascript"> function check() { //真实姓名(不能为空,其它没有要求) var name = document.getElementById("name").value; if(name==""||name==null) { alert("不能为空!"); return false; } //登录名(登录名不能为空,长度在5-8之间,可以包含中文字符())一个汉字算一个字符 var loginName = document.getElementById("loginName").value; if(loginName==""||loginName==null) { alert("登录名不能为空"); return false; } //\u4e00-\u9fa5 验证中文字符 var reg=/^[A-Za-z0-8\u4e00-\u9fa5]{5,8}$/; var result = reg.test(loginName); if(!result) { alert("登录名长度在5-8之间!"); return false; } //密码(不能为空,长度6-12字符或数字,不能包含中文字符) var pwd = document.getElementById("pwd").value; if(pwd==""||pwd==null) { alert("密码不能为空!"); return false; } var regpwd = /^[A-Za-z0-9]{6,12}$/; if(!regpwd.test(pwd)) { alert("密码长度在6-12之间"); return false; } //确认密码(不能为空,长度6-12字符或数字,不能包含中文字符,与密码一致) var repwd = document.getElementById("repwd").value; if(repwd==""||repwd==null) { alert("确认密码不能为空!"); return false; } if(repwd!=pwd) { alert("确认密码与密码不一致"); return false; } //身份证(15或18位) var idcard = document.getElementById("idcard").value; if(idcard==""||idcard==null) { alert("身份证不能空!"); return false; } if((idcard.length!=15)&&(idcard.length!=18)) { alert("身份证必选为15或18位"); return false; } if(idcard.length==15) { var regIDCard=/^\d{15}$/; if(!regIDCard.test(idcard)) { alert("身份证输入错误"); return false; } } if(idcard.length==18) { var regIDCard =/^\d{18}|\d{17}[x|X]{1}$/; if(!regIDCard.test(idcard)) { alert("身份证输入错误"); return false; } } } </script> <body> <h3 id="javascript验证">javascript验证</h3> <table width="854" border="1"> <tr> <td width="633">真实姓名(不能为空,其它没有要求)</td> <td width="205"><input id="name" name="name" type="text"/></td> </tr> <tr> <td>登录名(登录名不能为空,长度在5-8之间,可以包含中文字符())一个汉字算一个字符</td> <td><input id="loginName" name="loginName" type="text"/></td> </tr> <tr> <td>密码(不能为空,长度6-12字符或数字,不能包含中文字符)</td> <td><input id="pwd" name="pwd" type="password"/></td> </tr> <tr> <td>确认密码(不能为空,长度6-12字符或数字,不能包含中文字符,与密码一致)</td> <td><input id="repwd" name="repwd" type="password"/></td> </tr> <tr> <td>性别(必选其一)</td> <td><input id="sex" name="sex" type="radio" value="男" checked="checked"/>男 <input id="sex" name="sex" type="radio" value="女" />女 </td> </tr> <tr> <td>身份证(15或18位)</td> <td><input type="text" id="idcard" name="idcard"/></td> </tr> <tr> <td colspan="2" align="center"><input type="button" id="check" value="提交" onclick="check()"/></td> </tr> </table> </body> </html>
I hope this article will be helpful to everyone in learning javascript programming.
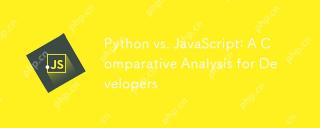
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
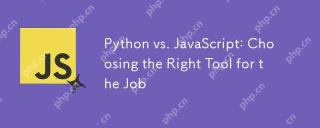
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
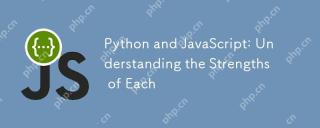
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
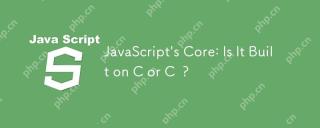
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
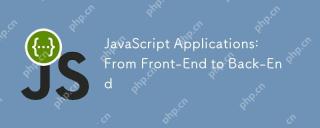
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
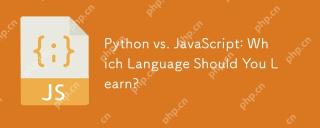
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
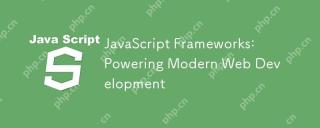
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
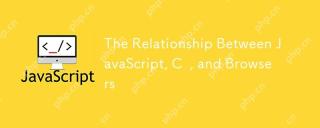
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Notepad++7.3.1
Easy-to-use and free code editor

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
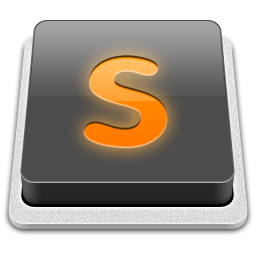
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
