Drag and drop effect, also called drag and drop. A simple drag and drop effect
Drag a div
[Program description]
First initialize the function, for drag and drop The object adds a mousedown listening event
initialize:function(drag){ this.Drag = $$(drag);//拖放对象 this._x = this._y = 0;//记录鼠标相对于拖放对象的位置 this._fM = BindAsEventListener(this, this.Move); //绑定拖动时执行的函数 this._fS = Bind(this, this.Stop); //绑定拖动结束执行的函数 this.Drag.style.position = "absolute"; EventUtil.addEventHandler(this.Drag, "mousedown", BindAsEventListener(this, this.Start)); //监听拖动对象mousedown事件 }
and then adds mousemove and mouseup events in the Start method to monitor the position of the drag and drop object and cancel the listening event respectively
Start:function(oEvent){ //记录mousedown触发时鼠标相对于拖放对象的位置 this._x = oEvent.clientX - this.Drag.offsetLeft; this._y = oEvent.clientY - this.Drag.offsetTop; //监听mousemove 和 mouseup事件 EventUtil.addEventHandler(document, "mousemove", this._fM); EventUtil.addEventHandler(document, "mouseup", this._fS); }
Full drag and drop program:
/** *拖放程序 */ var simpleDrag = Class.create(); simpleDrag.prototype = { //初始化对象 initialize:function(drag){ this.Drag = $$(drag);//拖放对象 this._x = this._y = 0;//记录鼠标相对于拖放对象的位置 this._fM = BindAsEventListener(this, this.Move); //绑定拖动时执行的函数 this._fS = Bind(this, this.Stop); //绑定拖动结束执行的函数 this.Drag.style.position = "absolute"; EventUtil.addEventHandler(this.Drag, "mousedown", BindAsEventListener(this, this.Start)); //监听拖动对象mousedown事件 }, //准备拖动 Start:function(oEvent){ //记录mousedown触发时鼠标相对于拖放对象的位置 this._x = oEvent.clientX - this.Drag.offsetLeft; this._y = oEvent.clientY - this.Drag.offsetTop; //监听mousemove 和 mouseup事件 EventUtil.addEventHandler(document, "mousemove", this._fM); EventUtil.addEventHandler(document, "mouseup", this._fS); }, //拖动 Move:function(oEvent){ this.Drag.style.left = oEvent.clientX - this._x + "px"; this.Drag.style.top = oEvent.clientY - this._y + "px"; }, //停止拖动 Stop:function(){ EventUtil.removeEventHandler(document, "mousemove", this._fM); EventUtil.removeEventHandler(document, "mouseup", this._fS); }, }; //初始化拖动 new simpleDrag('drag');
Full DEMO:
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <title>JavaScript拖放效果</title> <style type="text/css"> *{margin:0;padding:0;} .drag-wrap{height: 300px;margin:10px;border:5px solid #FF8000;background:#8080C0;position: relative;} .draggable{width: 100px;height: 100px;position: absolute;top: 100px;left:100px;background:#eee;border:1px solid #aceaac;cursor: move;} </style></head><body> <div class="drag-wrap"> <div class="draggable" id="drag">拖动div</div> </div> <div class="drag-status" id="drag-status" style="height:30px;padding:0 10px;"></div> <script type="text/javascript"> /** *工具函数 */ var isIE = (document.all) ? true : false ; var $$ = function(id){ return "string" == typeof id ? document.getElementById(id) : id; }; var Class = { create: function() { return function() { this.initialize.apply(this, arguments); } } }; var Extend = function(destination,source){ for(var property in source){ destination[property] = source[property]; } }; var BindAsEventListener = function(object,func){ return function(event){ return func.call(object,event || window.event); } }; var Bind = function(object,func){ return function(){ return func.apply(object,arguments); } }; /** *跨浏览器事件对象 */ var EventUtil = { //事件注册处理程序 addEventHandler:function(oTarget,sEventType,fnHandler){ if(oTarget.addEventListener){ oTarget.addEventListener(sEventType,fnHandler,false); }else if(oTarget.attachEvent){ oTarget.attachEvent("on"+sEventType,fnHandler); }else{ oTarget["on"+sEventType] = fnHandler; } }, //事件移除处理程序 removeEventHandler:function(oTarget,sEventType,fnHandler){ if(oTarget.removeEventListener){ oTarget.removeEventListener(sEventType,fnHandler,false); }else if(oTarget.detachEvent){ oTarget.detachEvent("on"+sEventType,fnHandler); }else{ oTarget["on"+sEventType] = null; } }, getEvent:function(event){ return event ? event : window.event ; }, getTarget:function(event){ return event.target || event.srcElement; } }; /** *拖放程序 */ var simpleDrag = Class.create(); simpleDrag.prototype = { //初始化对象 initialize:function(drag){ this.Drag = $$(drag);//拖放对象 this._x = this._y = 0;//记录鼠标相对于拖放对象的位置 this._fM = BindAsEventListener(this, this.Move); //绑定拖动时执行的函数 this._fS = Bind(this, this.Stop); //绑定拖动结束执行的函数 this.Drag.style.position = "absolute"; EventUtil.addEventHandler(this.Drag, "mousedown", BindAsEventListener(this, this.Start)); //监听拖动对象mousedown事件 }, //准备拖动 Start:function(oEvent){ //记录mousedown触发时鼠标相对于拖放对象的位置 this._x = oEvent.clientX - this.Drag.offsetLeft; this._y = oEvent.clientY - this.Drag.offsetTop; //监听mousemove 和 mouseup事件 EventUtil.addEventHandler(document, "mousemove", this._fM); EventUtil.addEventHandler(document, "mouseup", this._fS); }, //拖动 Move:function(oEvent){ this.Drag.style.left = oEvent.clientX - this._x + "px"; this.Drag.style.top = oEvent.clientY - this._y + "px"; }, //停止拖动 Stop:function(){ EventUtil.removeEventHandler(document, "mousemove", this._fM); EventUtil.removeEventHandler(document, "mouseup", this._fS); }, }; //初始化拖动 new simpleDrag('drag'); </script></body></html>
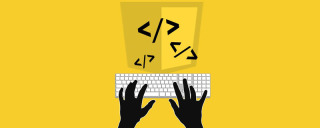
The official account web page update cache, this thing is simple and simple, and it is complicated enough to drink a pot of it. You worked hard to update the official account article, but the user still opened the old version. Who can bear the taste? In this article, let’s take a look at the twists and turns behind this and how to solve this problem gracefully. After reading it, you can easily deal with various caching problems, allowing your users to always experience the freshest content. Let’s talk about the basics first. To put it bluntly, in order to improve access speed, the browser or server stores some static resources (such as pictures, CSS, JS) or page content. Next time you access it, you can directly retrieve it from the cache without having to download it again, and it is naturally fast. But this thing is also a double-edged sword. The new version is online,
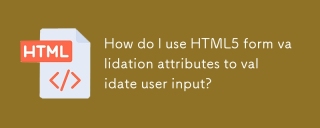
The article discusses using HTML5 form validation attributes like required, pattern, min, max, and length limits to validate user input directly in the browser.
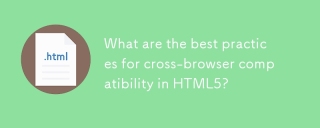
Article discusses best practices for ensuring HTML5 cross-browser compatibility, focusing on feature detection, progressive enhancement, and testing methods.
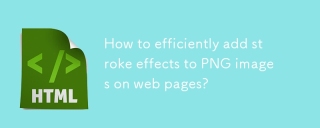
This article demonstrates efficient PNG border addition to webpages using CSS. It argues that CSS offers superior performance compared to JavaScript or libraries, detailing how to adjust border width, style, and color for subtle or prominent effect
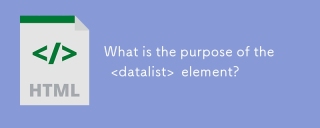
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
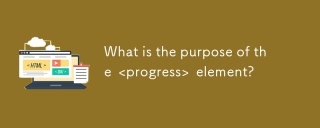
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
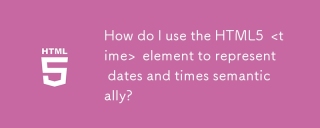
This article explains the HTML5 <time> element for semantic date/time representation. It emphasizes the importance of the datetime attribute for machine readability (ISO 8601 format) alongside human-readable text, boosting accessibilit
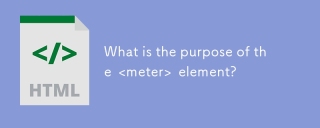
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
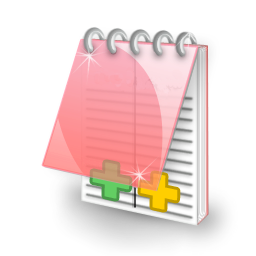
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
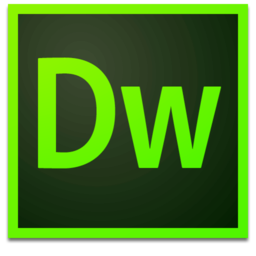
Dreamweaver Mac version
Visual web development tools
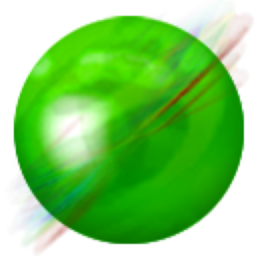
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
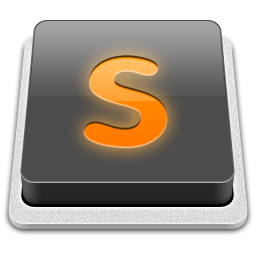
SublimeText3 Mac version
God-level code editing software (SublimeText3)

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
