Three ways to write loops:
<!doctype html> <title>js循环 by 脚本之家</title> <meta charset="utf-8"/> <meta name="keywords" content="js循环 by 脚本之家" /> <meta name="description" content="js循环 by 脚本之家" /> </head> <body> //while循环 <script type="text/javascript"> i = 1; while (i <= 6) { document.write("<h" + i+">脚本之家,这是标题"+i); document.write("</h"+i+">"); i++; } </script> //do_whilel循环 <script type="text/javascript"> i = 1; do { document.write("<h" + i+">jb51.net ,这是标题"+i); document.write("</h"+i+">"); i++; } while(i<=6); </script> //for循环 <script type="text/javascript"> for(i=1;i<=6;i++) { document.write("<h"+i+">脚本之家,这是标题"+i); document.write("</h"+i+">"); } </script> </body> </html>
Different types of loops
JavaScript supports different types of loops:
•for - loop through a block of code a certain number of times
•for/in - Loop through the properties of an object
•while - Loop through the specified block of code when the specified condition is true
•do/while - also loops through the specified code block when the specified condition is true
For loop
The for loop is a tool you will often use when you want to create a loop.
The following is the syntax of the for loop:
for (statement 1; statement 2; statement 3)
{
The executed code block
}
Statement 1 is executed before the loop (code block) starts
Statement 2 defines the condition for running the loop (block of code)
Statement 3 is executed after the loop (block of code) has been executed
Example
for (var i=0; i {
x=x + "The number is " + i + "
";
}
Try it for yourself
From the example above, you can see:
Statement 1 sets the variable (var i=0) before the loop starts.
Statement 2 defines the conditions for the loop to run (i must be less than 5).
Statement 3 increments a value (i++) each time the block of code has been executed.
Statement 1
Usually we use statement 1 to initialize the variables used in the loop (var i=0).
Statement 1 is optional, which means you can do it without using statement 1.
You can initialize any (or multiple) values in statement 1:
Example:
for (var i=0,len=cars.length; i
document.write(cars[i] + "
");
}
You can also omit statement 1 (for example, when the value has been set before the loop starts):
Example:
var i=2,len=cars.length;
for (; i
document.write(cars[i] + "
");
}
Statement 2
Usually statement 2 is used to evaluate the condition of the initial variable.
Statement 2 is also optional.
If statement 2 returns true, the loop starts again, if it returns false, the loop ends.
Tip: If you omit statement 2, you must provide a break inside the loop. Otherwise the cycle cannot be stopped. This may crash the browser. Please read about break later in this tutorial.
Statement 3
Normally statement 3 will increase the value of the initial variable.
Statement 3 is also optional.
Statement 3 can be used in several ways. The increment can be negative (i--), or larger (i=i+15).
Statement 3 can also be omitted (for example, when there is corresponding code inside the loop):
Example:
var i=0,len=cars.length;
for (; i
document.write(cars[i] + "
");
i++;
}
For/In loop
JavaScript for/in statement loops through the properties of an object:
Example
var person={fname:"John",lname:"Doe",age:25};
for (x in person)
{
txt=txt + person[x];
}
You'll learn more about for / in loops in the chapter on JavaScript objects.
For details, please refer to this article: http://www.jb51.net/w3school/js/js_loop_for.htm
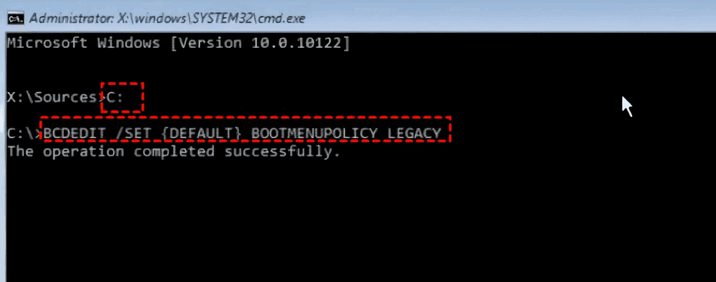
Kernelsecuritycheckfailure(内核检查失败)就是一个比较常见的停止代码类型,可蓝屏错误出现不管是什么原因都让很多的有用户们十分的苦恼,下面就让本站来为用户们来仔细的介绍一下17种解决方法吧。kernel_security_check_failure蓝屏的17种解决方法方法1:移除全部外部设备当您使用的任何外部设备与您的Windows版本不兼容时,则可能会发生Kernelsecuritycheckfailure蓝屏错误。为此,您需要在尝试重新启动计算机之前拔下全部外部设备。
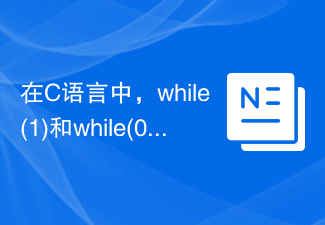
我们知道在C语言中,'while'关键字用于定义一个循环,该循环根据传递给循环的条件来工作。现在,由于条件可以有两个值,即真或假,所以如果条件为真,则while块内的代码将被重复执行,如果条件为假,则代码将不会被执行。现在,通过将参数传递给while循环,我们可以区分while(1)和while(0),因为while(1)是一个条件始终被视为真的循环,因此块内的代码将开始重复执行。此外,我们可以说明,传递给循环并使条件为真的不是1,而是如果任何非零整数传递给while循环,则它将被视为真条件,因
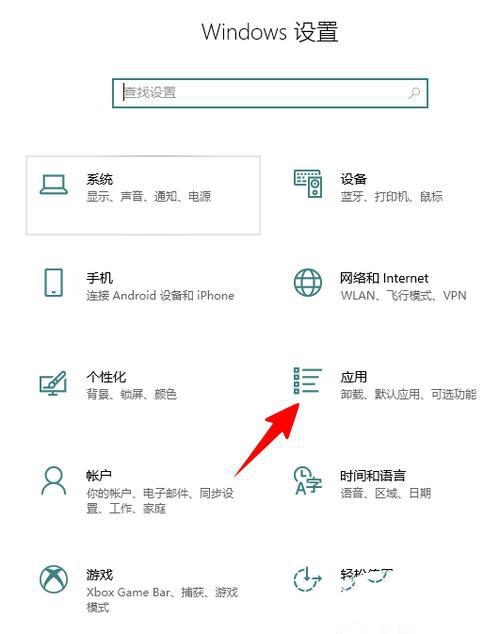
Win10skype可以卸载吗是很多用户们都想知道的一个问题,因为很多的用户们发现自己电脑上的默认程序上有这个应用,担心删除后会影响到系统的运行,下面就让本站来为用户们来仔细的介绍一下Win10如何卸载SkypeforBusiness吧。Win10如何卸载SkypeforBusiness1、在电脑桌面点击Windows图标,再点击设置图标进入。2、点击“应用”。3、在搜索框中输入“Skype”,点击选中找到的结果。4、点击“卸载”。5
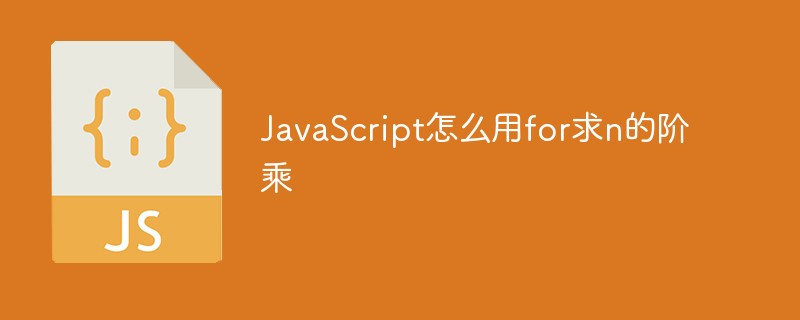
用for求n阶乘的方法:1、使用“for (var i=1;i<=n;i++){}”语句控制循环遍历范围为“1~n”;2、循环体中,使用“cj*=i”将1到n的数相乘,乘积赋值给变量cj;3、循环结束后,变量cj的值就n的阶乘,输出即可。
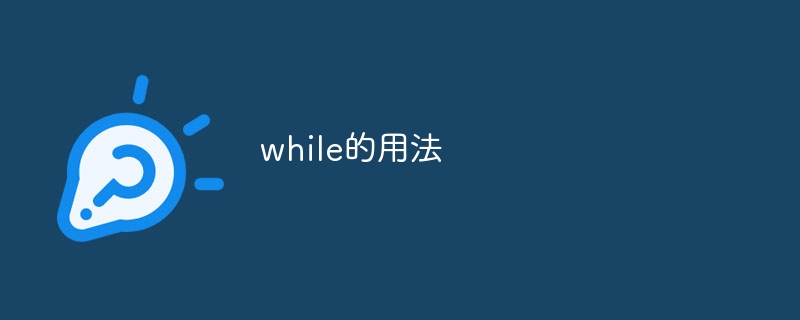
while的用法是“while 条件: 代码块”,条件是一个表达式,当条件为真时,执行代码块,然后再次判断条件是否为真,如果为真则继续执行代码块,直到条件为假为止。while是一个常用的循环控制语句,用于在满足一定条件的情况下重复执行一段代码块。
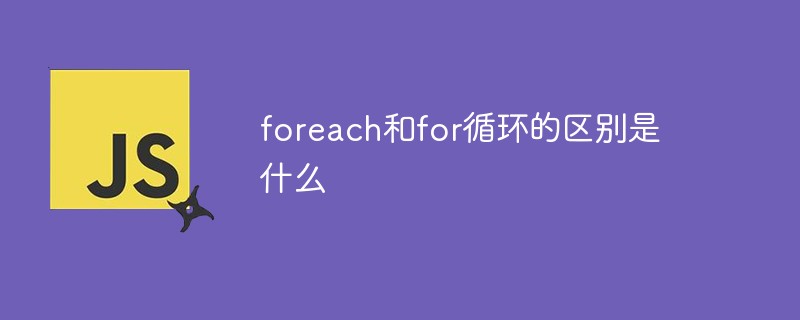
区别:1、for通过索引来循环遍历每一个数据元素,而forEach通过JS底层程序来循环遍历数组的数据元素;2、for可以通过break关键词来终止循环的执行,而forEach不可以;3、for可以通过控制循环变量的数值来控制循环的执行,而forEach不行;4、for在循环外可以调用循环变量,而forEach在循环外不能调用循环变量;5、for的执行效率要高于forEach。
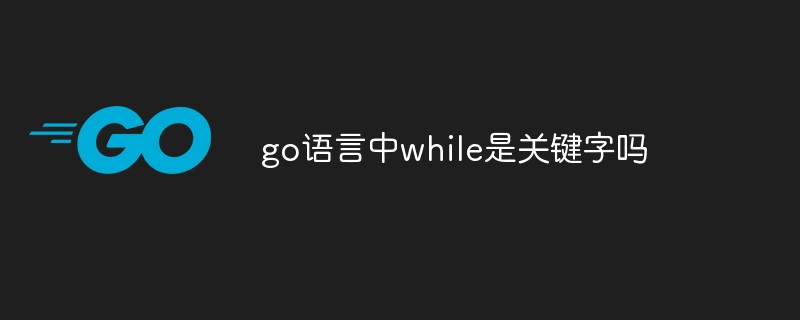
在go语言中,while不是关键字,可以用for语句加break来实现while循环的效果,例“for {sum++ if sum>10{break}else{...}}”。go语言有break、default 、func、select、case、defer、go、map、else、goto、for、if、var等25个关键字。
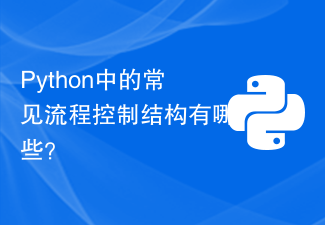
Python中常见的流程控制结构有哪几种?在Python中,流程控制结构是用来决定程序的执行顺序的重要工具。它们允许我们根据不同的条件执行不同的代码块,或者重复执行一段代码。下面将介绍Python中常见的流程控制结构,并提供相应的代码示例。条件语句(if-else):条件语句允许我们根据不同的条件执行不同的代码块。它的基本语法是:if条件1:#当条件


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
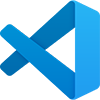
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
