Author: excelence Repost Date of compilation: June 15, 2004
This article is not just about JavaScript, it is about JavaScript in Notes/Domino!
That being said, it’s still worth a look!
Hope you gain something!
Getting up close and personal with radio buttons, checkboxes, and some fun stuff with JavaScript
I’ll start with the object model and some common objects, because everything in JavaScript starts with objects . Remember, this article isn't just about JavaScript, it's about JavaScript in Notes/Domino.
Window
The window is the top object of the object model. Typically, the window is your browser. If you have frame structures in your window, then each frame structure is in turn a small window contained in the top-level window - the browser. I'll talk about frame structure in another article, but for now, let's look at the situation where there is only one Web page in the browser.
The window has its properties, such as its address (that is, its URL), the text on the status bar at the bottom of the browser, etc.; it also has methods, such as opening and closing. Generally speaking, because the window is at the top level of the object hierarchy, JavaScript assumes that the Window already exists, and you don't have to write it deliberately. In other words, "window.location" and "location" have the same effect.
The window is a Web page, and its object hierarchy starts from the document. You can reference it as Window.document, or simply document. There is only one document per window. Documents have several varying options depending on your browser. In MSIE, the document.all array contains all objects in the document. In some versions of Netscape Navigator, you can access the document.layers array. Each browser interprets objects differently, but forms arrays are accessible in all browsers.
Theoretically, each document contains at least one form, but can contain multiple. However, in Notes, unless you explicitly write the HTML code to accomplish a specific function (which I never do), there is usually only one form. However, because there can be multiple forms, when you reference the form, you still have to reference the form through the array element, and the subscript starts from 0. Unlike LotusScript, which uses parentheses to enclose subscript numbers, such as doc.CompanyName(0), in JavaScript you will usually see numbers that refer to array subscripts enclosed in square brackets. Therefore, you should reference forms in the following way:
window.document.forms[0];
or abbreviated as:
document.forms[0];
Strictly speaking, the above method is not the only way to reference the form. The following are legal expressions to reference the form:
document.forms[0];
document.forms(0);
document.forms.0;
However, what you often see is still the bracketed approach. Note that I added a semicolon at the end of each line, which is one of the similarities between JavaScript and formula languages. You should add a semicolon at the end of each statement. Unlike formula languages, semicolons are not always necessary in JavaScript. Some browsers are more capable than others of running JavaScript statements without semicolons, so while you can sometimes skip semicolons, it's a good idea to get in the habit of adding them to every statement.
When you get to the form, you're ready to access the elements you care about most. A form is a container for fields, buttons, text, images, and other elements that you will use JavaScript to process in the form.
A few simple elements
For LotusScript, one thing is worth mentioning: except for elements outside the Rich-Text domain, such as text, radio buttons, list boxes, check boxes, etc., you can use Almost the same code to get their values. For example: If there is a "Location" field, no matter what type it is, you can get its value using the following LotusScript code:
fieldVals = doc.Location
Or like this:
fieldVals = doc.GetItemValue("Location")
In LotusScript, the field type is what you want The code that gets the values (array of values) doesn't matter. Unfortunately, this doesn't work with JavaScript. In JavaScript, in addition to displaying options (such as radio buttons, check boxes, or text), different types of fields are not like in Notes. They are different types of objects, each of which needs to be processed in a different way. Quote. In fact, that is not absolute. Some objects are similar, but the reference process is not as smooth as in LotusScript. You will find that you will spend a lot of time manually looking for domain (name) errors in your code to make them run correctly, and that will look bad.
The first thing you need to know is: in JavaScript, there is no so-called Rich-Text domain, and there is no such thing in HTML.Notes provides a rich text Java (not JavaScript) applet that can be placed in the browser, so that you can get some functions of rich text, but you cannot use JavaScript to program it, and it is not a real HTML. Object type.
What surprises Notes developers even more is that there are no numeric or time fields on the Web. HTML fields are all text type. Although you can use them to collect numerical information, such as quantities or unit prices, the data saved is still text. In order to use it like a number, you have to convert it to a numeric type. I'll explain it in detail later. Now, realize that everything is text, just like the information you enter in Notes' @Prompt dialog box.
Figure 1
Creating a form
In order to understand more deeply, you will do a small experiment with my help. Open Domino Designer, create a new form, create a button named "Get editable field value" and a text field named "CreatedBy" on the form, and set the default value of this field to: @V3UserName (as shown in the figure) 1).
If you use R5’s Designer, please change the language of the trigger button to JavaScript. If you use R6's Designer like me, it's a little more troublesome. In Notes/Domino 6 (ND6), you can use JavaScript in the Notes client just like you use JavaScript in the browser. More importantly, the same button can run different codes in different clients. In Figure 2, you'll see some options that let you decide whether the code you write will run in Notes ("client") or in a browser ("web").
Picture 2
Picture 3
This function is very useful when you run different codes in different environments, but when you want to What should I do if I run the same code in two environments? Of course, there is a better way than copying the button code. You can select Common JavaScript at the bottom in the drop-down list on the right to write code for both environments at the same time (Figure 3).
In this example, select “Common JavaScript”.
In the button script, enter the code that prompts the user for the CreatedBy field value. If you read the previous article, you know that you can use dots to separate hierarchical elements in JavaScript. Your button script should look like this:
alert(document.forms[0].CreatedBy.value);
It is important to note that JavaScript case sensitive. This rule is not only valid for JavaScript elements (documents, forms, etc.) (the first letter is lowercase), but also for field names. Whatever casing effects you use in your fields must be reflected faithfully in your code. Experiment with the button's functionality in the browser [Design>Preview in Web Browser>Internet Explorer (or your own browser)]. If you use the Domino R6 Designer, you can experiment in the Notes client (Design>Preview in Notes).
Figure 4
If you are testing with a local database (corresponding to the server), the user name you see in the browser will be "Anonymous", Instead of your name, it is your name in the Notes client. The reason is that when you access the local database using a Web page, you are not logged in to the server, and the browser does not know who you are. But in Notes, whether it is local or server, you must log in to use the system. This is the subtle difference between the two clients.
Another difference is that there are no calculated fields on the web, but that doesn’t mean you can’t add calculated fields to your forms. You can add calculated fields and the calculated values will be displayed in the web page unless the fields are hidden. The point is that even though the fields are displayed there, HTML normally handles them without defining fields. If you change the CreatedBy field to a calculated field instead of editable, you will know what I mean when you retest, and you will get a message similar to Figure 4.
But when you run it in Notes client (R6), there are no errors even for the calculated domain. Frankly, I haven't concluded yet whether this feature is a good or bad feature, but that's what we get.
The errors generated here are very important. You need to start understanding the errors generated in JavaScript, because it is very useful for debugging your code. Then when it prompts you that "document.forms.0.CreatedBy.value" is null or not an object, it means that you did not get the data you want.
When you return to the browser, right-click on the background and select "View Source", you will see the HTML code hidden under the web page. When you briefly browse the code, you'll see references to buttons and calculated fields like this:
Created By:Anonymous
When you have formatted the page, your code may have fonts, paragraphs or other markup mixed in with the code for buttons and calculated fields. Those are used for formatting documents and can be ignored in the discussion here. Notice that "Anonymous" is another word on the form, it doesn't have any markings on either side to remind you that it was generated from the domain. In the source code, there is no difference between Anonymous and "Created By": two static texts preceded by the domain (if you are logged in, you will see your name instead of Anonymous).
For comparison, change the CreatedBy field back to editable text, save the form, return to the browser and refresh the page, then look at the source file of the page, it will look like this:
Created by:
Instead of the word Anonymous (or your name) is the HTML code for the domain (or Strictly speaking, it is an HTML text input box). Its name is "CreatedBy" and its value is "Anonymous".These are properties that can be obtained through JavaScript, but ordinary text does not have these properties. So you can't reference computed fields using JavaScript, at least in the browser. Another point of confusion is that when the document is in a non-editable state, even editable fields cannot be referenced using JavaScript. In other words, when you save the document and open it again without setting it to edit mode, the HTML code of the page will be the same as if the CreatedBy field was a computed field. Another interesting thing about JavaScript is that outside of Domino, we don't have many opportunities to deal with the editing and non-editing states of forms, and for us Domino developers, this is a big problem.
Did you notice that buttons and fields are converted to input objects? That's how HTML works. What is confusing is that both objects have the value attribute. For the button, the value is "Get editable field value", which I guess is the label of the button, but the value of the field is its actual value. Some other types of objects have both value and text properties. If you're like me, you sometimes have trouble figuring out what's what! From my experience, the easiest way is to read the HTML code of the Web page.
Multi-valued
HTML fields do not have multi-valued attributes like Notes fields. Try this: add a second button and a second field to the form. Name the field "Letters", type Editable Text, and select the "Allow multiple values" checkbox. Write the default value as a list of letters like this:
"A":"B":"C":"D":"E":"F":"G"
Name the button "Get multiple values" and type the following JavaScript code:
alert(document.forms[0].Letters.value);
You can change the value of this field with different delimiters, but when you click the button, you will notice that no matter what delimiter you use, you will always be prompted for all of the fields value. This is in contrast to @Formulas and LotusScript running in the Notes client. With @Prompt, the prompt you get is just the first value of the field: "A". You can also only display one value using LotusScript, but you have to specify the array subscript, otherwise you will get an error message. Neither formulas nor LotusScript can get all the values of a multi-valued field in a prompt statement.
The reason is that in the Notes language, there are indeed multiple values in the field. For HTML and JavaScript, there is only one value. Look at the source code of the Web page again and you will find code similar to the following:
Note that its value is "a pair" Delimited value enclosed in double quotes. How to separate individual values will be discussed in detail later, but for now, you should realize that multivalue is not exactly multivalue on the web (at least for text input fields). Other types of fields will be handled differently than text fields.
Radio Buttons
Another interesting thing is radio buttons. As far as Notes and Web pages are concerned, a radio button in Notes is a single field with multiple values. On the Web it is An array of input text boxes with the same name. To understand the above, take a look at the following example:
On the form, add another new field named "RadioButtn". As the name suggests, change it to a radio button type field. In the second tab of the Domain Properties window, enter the following options and aliases (Figure 5):
Figure 5
One | A
Two | B
Three | C
Four | D
Set the default value of this field to the alias of the first option, also It's the letter "A" in quotes.Now preview the form in the browser and look at the source file. You will see that the code for the radio buttons is very different from the code for the normal fields. The HTML code will look similar to the following:
One
Two
Three
Four
Here you should pay attention to some points. First, all four radio button objects have the same name: RadioButtn, so that HTML and JavaScript know that they are objects in the same array. Secondly, the value of each option is the alias of the option, not the text you see (such as "One", "Two", etc.). This is the same as saving an alias in Notes, not the text you see (of course, if there is no alias, the saved value and text are the same). Finally, the way you check the first option is by using the word "checked" in the HTML statement, which is added to the first radio button statement.
If you add another button and get the value of RadioButtn in the same way as the other two field values, you will get a strange error, that is, use the following code:
alert(document.forms[0].RadioButtn.value);
You will see an error dialog box prompting "undefined" (as shown in the picture 6).
Figure 6
The problem here is not that its value is not defined. After all, as shown above, there are a total of 4 values in the radio button's code. That said, the problem lies with the RadioButtn itself, at least the one used here. A radio button is an array of input options. If you want to know the value of one of the elements, you must specify which one it is. Try the following code:
alert(document.forms [0].RadioButtn[0].value);
Okay, what is returned is the value "A" of the current field, but when you select other options and then click the button, you get It's still "A" instead of the value you selected, which still isn't great.
In order to get the value of the selected option, you must first know which option is selected, and then use the subscript value of that selected option to correctly reference the value of the current option in the alert statement. That is, if the first option is selected, you should take RadioButtn[0].value, if the second option is selected, you should take RadioButtn[1].value, and so on.
In JavaScript, certain types of fields have a selectedIndex attribute, which represents the array subscript value of the currently selected option. However, radio buttons were not so lucky, and neither were checkboxes. To get the value of the currently selected radio button, you must look for the "checked" attribute in the RadioButtn array element. Compared with LotusScript, LotusScript can obtain the currently selected value of the radio button just like operating other types of fields. It's a very cumbersome thing to do, but, that's what it is.
The following is the code for the button:
var doc = document.forms[0];
for(i = 0; i
doc.RadioButtn.length; i ){
if(doc.RadioButtn.checked){
alert(doc.RadioButtn.value);
break ;
}
}
A few new concepts are coming up here right away, let me take a moment to explain them. First of all, if you read the previous article, you will know that "var" is used to declare variables or assign values to variables in JavaScript, just like "Dim" and "Set" in LotusScript.In this case, in order to avoid entering document.forms[0] over and over again, I assign document.forms[0] to the variable doc for later use:
var doc = document .forms[0];
The following is a loop. When there is no code in the loop body, it looks as follows:
for(i = 0; i
}
Are you a little dazzled? I used to get dizzy every time I saw code like this, but it's not as bad as it looks. First of all, the curly braces are only used to contain the body of the loop. JavaScript's for loop has three options:
1. Counting variable and its initial value (i=0);
2. How to know to continue looping (i
3. How to accumulate counting variables (i).
The first is i=0, it’s very simple, I used the variable i and its initial value is 0.
Part 2: i
The third parameter in the loop body: i is a low-level error for LotusScript developers. This is a shorthand way in JavaScript that the value of i is the same as the value of i=i 1. In fact, you can use either of the two forms in the loop, so the following is equivalent to the above:
for(i = 0; i
}
i=i 1 can also run normally, but the problem is that no one does that, because you are used to i Finally, it is shorter and simpler. The value is that you can also write i--, which is the same as i=i-1, but of course it won't work in this loop. There are a lot of interesting-looking things you can do with i, but that's for another day.
Look at the loop again: the three parameters are separated by semicolons and surrounded by parentheses, the loop body code is surrounded by curly braces, and the loop body is an if statement:
if(doc.RadioButtn.checked){
}
The true and false statements here are also surrounded by parentheses. The doc.RadioButtn.checked in the experiment doesn't seem to provide enough information, but together with the loop it forms a JavaScript shorthand. What if we use LotusScript? I will first check whether the current RadioButton element is equal to checked, like the following:
doc.RadioButton[0] = "checked"
Since checked is a property and not a value, the above code will not run correctly. You'll also notice that in the HTML it's not enclosed in quotes. In fact, checked is a "yes" or "not" thing. To be more precise, it is either true or false, so the meaning of the if statement is: if this element of RadioButtn is checked (selected), that is: true, then do the following things... The test here looks like It's a little strange because there's never any mention of authenticity. If that makes you uncomfortable, you can easily write an if statement like this:
if(doc.RadioButtn.checked == true){
}
Note that true here is all lowercase and has two equal signs. Unlike LotusScript, the equal sign (=) in JavaScript is only used to assign a value to something else:
var doc = document.forms[0];
To compare two items for equality, you must use two equal signs. In my head, I think it means: doc.RadioButtn.checked equals true, which helps me remember to use two equal signs. The double sign mark also applies to the ampersand (one is used to append or connect characters; two is used to represent "and", such as when using two conditions in an if statement).
Finally, inside the if statement is an alert statement, followed by a break. break is Exit For in JavaScript. It terminates the loop because a radio button can only have one option selected.
If you put all the code into the button, you will see that no matter which option of the radio button you select, the value of the option will be displayed correctly after clicking the button. Great, now you are getting started with JavaScript.
Checkbox
Checkbox is similar to a radio button.On the form, copy the radio button field and rename it "CheckBx", change the field's type to "CheckBox", save the form, refresh the web page and view the source file. You will see that the HTML code for the checkbox is almost identical to that of RadioButtn:
One
Two
Three
Four
The real difference is not the domain name, but the type is checkbox, not radio button. Another difference is that you can select multiple values in a checkbox. Although you have to iterate through the entire checkbox to determine which option is selected like a radio button, you cannot stop traversing before checking whether they are all selected. action. The code in the button to check the checkbox selection will look like this:
var doc = document.forms[0];
for(i = 0 ; i
if(doc.CheckBx.checked){
alert(doc.CheckBx.value);
}
}
I’m sure by now you should be more or less familiar with this code, because it’s basically the same as a radio button, except the domain name has been changed and the “bread” has been removed. statement. In a practical program, this code does a lot more work than I did, but first I want you to feel comfortable learning the basics.
Waiting for more objects
You have a few more types of fields to learn about, each with their own characteristics, but that’s fodder for another article. Now, you may want to familiarize yourself with the examples here by experimenting on your own. You may also want to add more fields to the form that I haven't covered yet and look at their HTML source files, but that's where we'll start next time. See you later.
Reference resources
This article is excerpted from the 2003.6 "Lotus Magazine Simplified Chinese Edition": "Lotus Magazine Simplified Chinese Edition" is the first domestic publication focusing on IBM Lotus technology, and its content is mainly derived from The top technology publication related to Lotus in the United States is aimed at Lotus managers and developers and shows you the latest and most cutting-edge Lotus related technologies. Welcome to apply for free subscription.
Lotus related topics: If you are a developer interested in Lotus, want to know more about the Lotus product family, be familiar with how Lotus products work together with other IBM products, how Lotus supports things like XML, Web services, etc. Waiting for the industry's open standards, how to use Lotus for J2EE development, immediately visit the Lotus-related topics on the IBM developerWorks China website. Here, we have carefully collected relevant articles, tutorials, and red books for you. We believe they will be helpful to your development.
About the issue of active drop-down list box and fuzzy query
I want to enter some text in the drop-down list box, and then display the results of the fuzzy query from the data table class in the drop-down item , I wrote the following code, but I am not allowed to enter content in
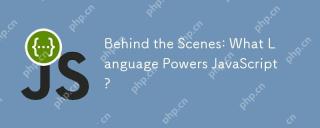
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
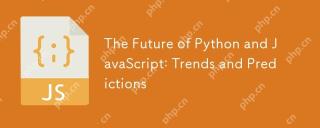
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
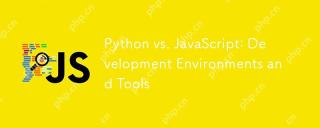
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
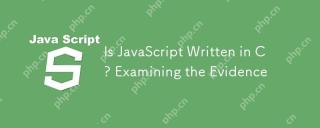
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
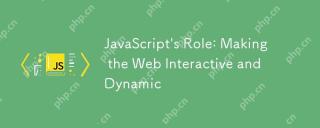
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
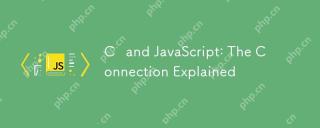
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
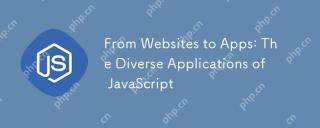
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
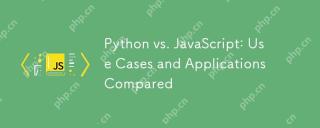
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
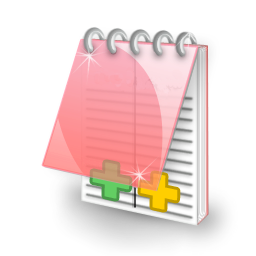
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
