It is necessary to sort out a lot of previously confusing knowledge: let’s start with the most basic variables.
1. Variable variable
a. Global Variable and Local Variable Private Variable
There is a difference between adding var and not adding var
--》If the keyword var is not used, declare a variable , then this is a global variable, which can be accessed by any sub-function, even where the curly braces are jumped out; , all functions can access this variable, such as:
function foo(){
i=8 🎜>function foo2(){
var i=88 //Variable i can be accessed under these curly braces
alert(i);
child()
function child(){alert(i )}
}
foo();foo2()
alert(i) //The i here is still 8
It is worth noting that function is a compile-time object and must be executed or instantiated to allocate this variable in memory.
Global variables are accustomed to start with _; p.s It is best to use all variables with caution, you know when this variable changes there!
-------" See js manual:
"Although not safe, it is legal JScript syntax to omit the var keyword in a declaration statement. At this time, the JScript interpreter gives the variable global scope visibility . When a variable is declared at the procedure level, it cannot be used in the global scope; in this case, the variable declaration must use the var keyword ”
b. The types of the variable. variable
Jscript has three main data types, two composite data types and two special data types.
The main (basic) data types are:
String
Numeric
Boolean
The composite (reference) data types are:
Object
Array
The special data types are:
Null
Undefined
The following is a brief description of the various object types in JavaScript:
Native Object: The JavaScript language does not rely on execution Host objects, some of which are built-in objects, such as Global and Math; some are created and used in the script running environment, such as: Array, Boolean, Date, Function, Number, Object, RegExp, Error.
Build-in Object: Built-in objects provided by the JavaScript language that do not depend on the execution host, such as Global and Math; built-in objects are all Native Objects.
Host Object: Any object provided by the JavaScript language that depends on the host environment. All non-Native Object objects are host objects, such as: window in IE, wscript instance in WScript, and any user-created class.
****How to check object type? ******
1.typeof()
The typeof operator returns type information as a string. There are six possible return values of typeof: "number," "string," "boolean," "object," "function," and "undefined."
2. val instanceof Array
returns a Boolean Value indicating whether the object is an instance of a specific class.
For example, when checking an array or date type (in fact, any type is allowed, see example), you must use instance of class name (without quotes), for example:
function foo(){ }
var f = new foo();
alert(f instanceof foo2) //false
3.constructor
The usage of constructor feels the same as the usage of instance, except that it does not return boolean value
x = new String("Hi");
if (x.constructor == String)
// Process (condition is true).
What type is *******var i={}? ****
Answer: object type is equivalent to var i = new Object
Object object is the carrier of all objects, a bit like the parent class
Object object is very simple,
It has only two properties and two methods
The two properties are:
prototype
constructor
The two functions are:
toString()
valueOf()
So how to text var obj = new MyObject()? In fact, it is very simple. The textual definition of obj is as follows:
var obj =
{
Properties1: 1, Properties2: '2', Properties3: [3] ,
Method1: function(){ return this.Properties1 this.Properties3[0];},
Method2: function(){ return this.Preperties2; }
};
The syntax for textual definition of class instances is to use a pair of "{}" to represent the class, which means that "{}" is completely equivalent to "new Object()". Then press "key:value" within "{}" to organize properties and methods. Key can be any combination of characters [A-Za-z0-9_], even numbers starting with @_@ are legal, and value is any legal Textual JavaScript data, and finally each key-value pair is separated by ",".
Usually used to exchange data with JSON.
Two meanings of *******undefined*****
1.undefined keyword 2.undefined attribute
Declared a variable but did not assign a value , it belongs to the first situation;
There is no declaration at all, and a variable is used to participate in the calculation, and its data type is the second situation;
Two same names but different meanings, it is recommended to change the name in the next version .
var declared;
if (declared == undefined) //Changing to uninitialized will be more accurate, js is loose language indeed!
document.write("declared has not been given a value.");
if (typeOf(notDeclared) == "undefined")
document.write("notDeclared has not been defined.");

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
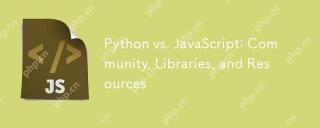
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
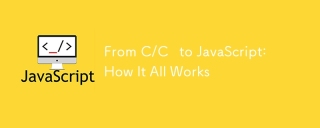
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
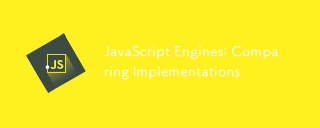
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
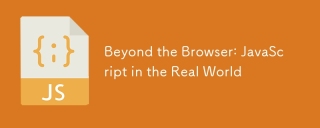
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
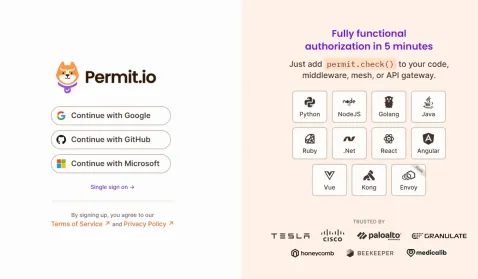
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
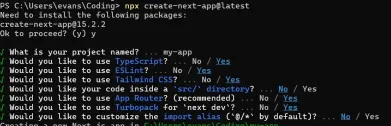
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
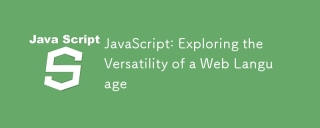
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
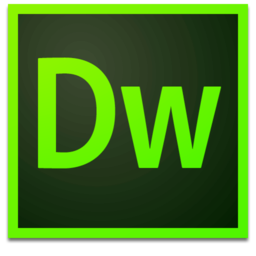
Dreamweaver Mac version
Visual web development tools
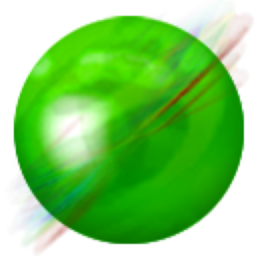
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.