It's className, not class
Note that JavaScript uses className to access the class attribute, because class is a reserved keyword, because in the future JavaScript may start to support classes like Java.
We encountered thorny details and browser differences when discussing the style attribute, which was like going through a stormy sea. The changes of class and id are like a calm oasis in the desert, where browsers live in harmony. Consider this example:
p {
color: #000000; /* black */
}
p.emphasis {
color: #cc0000; /* red */
}
Test
Initially, the paragraph has no class defined, so its font color It's black. However, a single line of JavaScript is enough to change its style:
document.getElementById('test').className = 'emphasis';
In an instant the text turns red. If you want to change it back, you can do the following:
document.getElementById('test').className = '';
You have removed the style and the paragraph returns to the default p{} rules.
For a practical example, take a look at "Limited length text input area". The counter has this structure and rendering style (the structure is dynamically generated by JavaScript, but that doesn't affect this example):
div.counter {
font-size: 80%;
padding-left: 10px;
}
span. toomuch {
font-weight: 600;
color: #cc0000;
}
When the script finds that the text entered by the user must have reached the maximum length , it modifies the class of as a counter to toomuch:
[Length-limited text input area, lines 20-23]
if (currentLength > maxLength)
this.relatedElement.className = 'toomuch';
else
this.relatedElement.className = '';
Now, the font of as counter changes in bold and red.
Change of id works almost exactly the same way:
p {
color: #000000; /* black */
}
p#emphasis {
color: #cc0000; /* red */
}
Test
document.getElementsByTagName('p')[0].id = 'emphasis';
The text of this paragraph turns red again. However, I recommend you not to change the id too much. In addition to being used as CSS hooks, they are also often used as JavaScript hooks, and changing them may have unspecified side effects.
Add class
Normally, you don’t set a new value for an element’s class, you just add a class. Because you don't want to remove any styles the element already has. Because CSS allows composite styles, the styles contained in the new class are added to the element without removing any CSS directives from the existing class.
The writeError() and removeError() functions in "Form Validation" are a good example. Generally I apply several classes to form fields because graphic designers often use two or even three widths for input fields. I want to add a special warning style when a form field contains an error, but I don't want to mess with the styles that the element already has. So, I can't simply overwrite the old class value, then I'll lose the width I've specified.
Look at this situation:
input.smaller {
width: 75px;
}
input.errorMessage {
border-color: #cc0000;
}
Initially, the width of the input box is 75px. If the script sets the class to 'errorMessage' and deletes the old value, the form field will get a red border but also lose its width, which can be very confusing to the user.
Therefore, I added the errorMessage class:
[Form validation, lines 105~106]
function writeError(obj,message) {
obj.className = ' errorMessage';
This code gets the existing className and appends a new class after it, adding a space before it. This space separates the new class from any class values the object may already have. Now the input box has a red border as we expected, in addition to being 75px wide. The form field now has two classes applied, and the HTML looks like this:
Class name in Mozilla with a blank
You may notice removeError( ) There is no leading space when removing the value of class errorMessage. That's because of a browser bug. When you add errorMessage to a class that originally had no value, Mozilla removes the leading spaces. If we then execute replace(/ errorMessage/,''), Mozilla cannot remove the class because it cannot find the string errorMessage because the leading spaces are no longer there.
Remove class
Once the user fixes her error, the value of class errorMessage should be removed, but any original class, such as smaller, should not be affected. The removeError() function provides this function:
[Form validation, lines 119-120]
function removeError() {
this.className = this.className. replace(/errorMessage/,'');
It first gets the class of the element and then replaces the string 'errorMessage' with '' (a null character). errorMessage is taken from the value of class, but has no effect on other values. The form field loses its red border color, but still maintains a width of 75px.
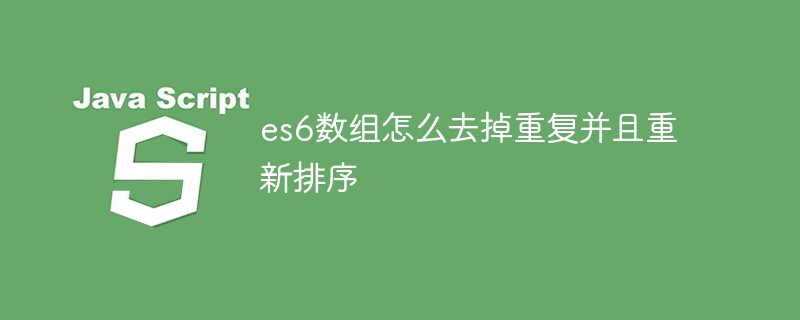
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
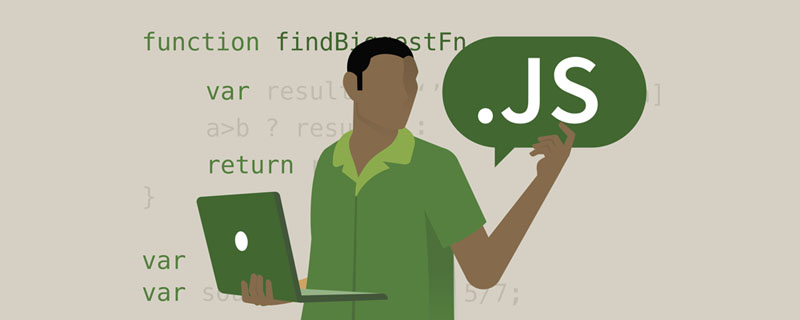
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
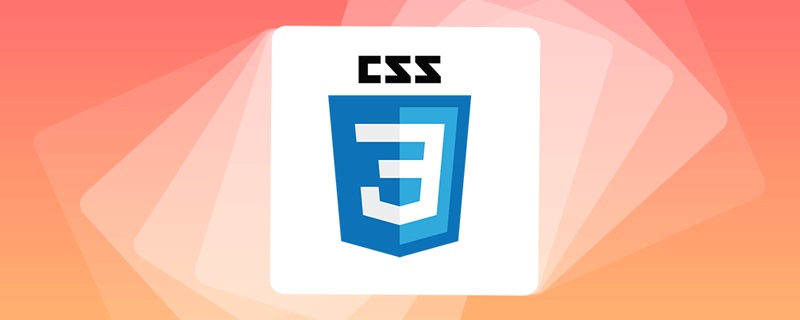
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
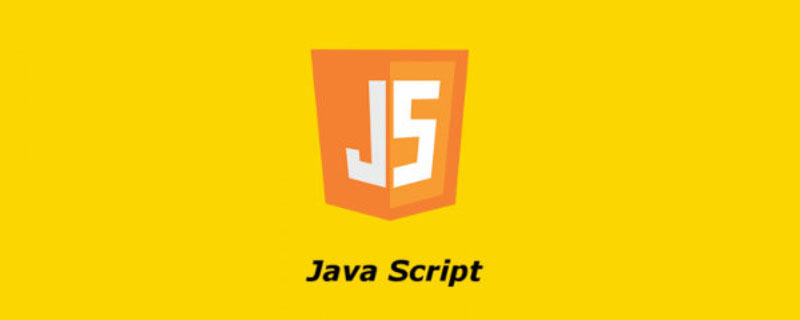
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
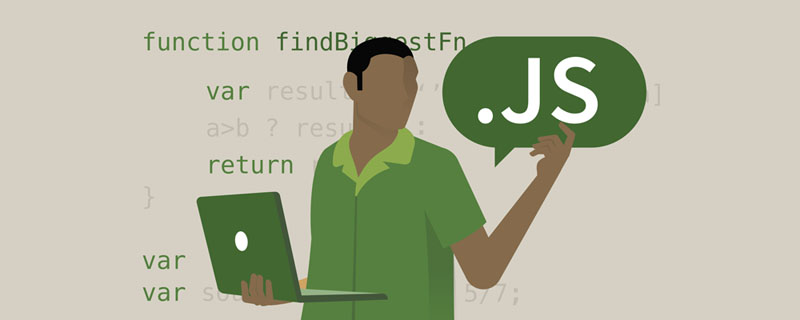
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
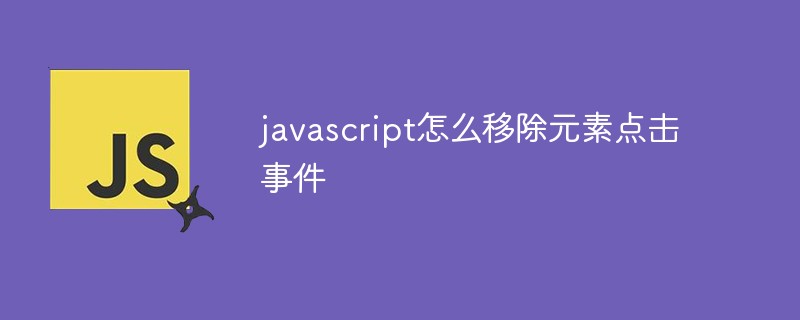
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
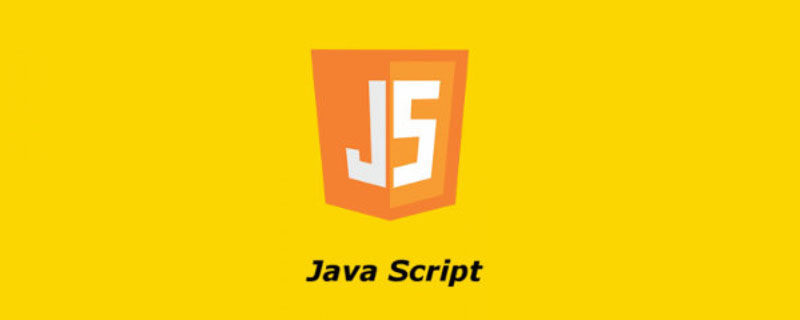
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
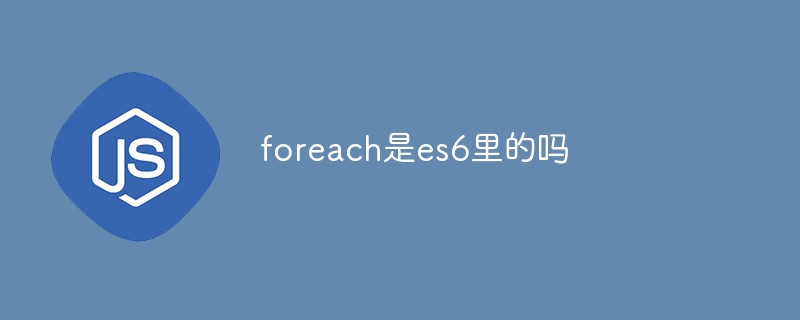
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
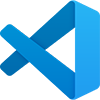
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
