Javascript is of course no exception, but have you ever considered the issue of object references? The usual approach is for a series of objects to share the methods of a class, rather than duplicating a function for each object. Let's look at how to copy a function for each object.
var myobject=function(param1,param2)
{
this.name=param1;
this.age=param2;
this.showmsg=function()
{
alert("name:" this.name "n" "age :" this.age);
}
}
var objectone=new myobject('liu',20);
alert(objectone.name); //liu
var objecttwo= new myobject('lin',20);
alert(objecttwo.name); //lin
alert(objecttwo.showmsg());
var objectthree=new myobject('lhking',22) ;
alert(objectthree.showmsg());
It looks good and works well. There is no interference between the objects and the work can be completed normally. Everything seems natural. But every time you generate a new object, the script engine will copy the properties and methods to the object. Do you think this is a waste of memory? This method of copying objects on the client side is extremely easy to cause memory leaks, because every time a new instance is generated, all properties and methods will be copied, occupying a large amount of memory.
The first consideration in large JavaScript applications is memory issues.
The correct way to use it is to use the prototype keyword to define a method or attribute of a class
var myobject=function(param1,param2)
{
this.name=param1;
this.age=param2;
myobject.prototype.showmsg=function( )
{
alert("name:" this.name "n" "age:" this.age);
}
}
var objectone=new myobject('liu', 20);
alert(objectone.name);
var objecttwo=new myobject('lin',20);
alert(objecttwo.name);
alert(objecttwo.showmsg()) ;
var objectthree=new myobject('lhking',22);
alert(objectthree.showmsg);
In this case, the objects you create can share methods, and That is, the showmsg() function is only defined once, and other objects share this method instead of copying their own methods.
The prototype in Javascript has been finished.
Look at object expansion in JavaScript
function rand(x)
{
return Math.ceil(Math.random()*x);
}
An extension method to generate a random array
Array.prototype.random= function()
{
for(var i=(this.length-1);i>1;i--)
{
var j=rand(this.length-1);
var cache=this[i];
this[i]=this[j];
this[j]=cache;
}
}
var array=new Array ("1","2","3","4","5");
for(var a in array)
{
alert(array[a]);
}
array.random();
for(var b in array)
{
alert(array[b]);
}
See again Look at object reflection
Reflection is an object mechanism that allows you to understand its properties and methods without knowing anything about the object. Normally, programmers are very ignorant of how the objects they manipulate are composed. Understood, but in some special cases when using a complex object written by someone else, we need to quickly understand the properties and methods of this object, and we need to use the reflection mechanism. Of course, the application of reflection is not limited to Therefore, here is just an introduction to the use of reflection in Javascript.
First of all, we may want to know whether there is a specific property or method in an object. At this time we can test it:
if (typeof(myobject.someproperty)!="undefined")
{
}
If an object or variable is not defined in Javascript, it always returns the undefined type.
You can also use other Built-in classes to narrow the scope of testing:
if(myobject instanceof Object)
{
}
instanceof is an operator used to test built-in or custom classes. The built-in class refers to Array , String, Date, Number, Math, RegExp, Boolean, Function and other built-in classes. For example: Function and Array both inherit from the Object class, so if you test an Array object in your code, if you First test whether it is an Object and it will return true. If you test whether it is an Array, it will also return true.
A simpler and more useful method is to traverse all the properties and methods of an object to quickly understand the internal state of an object:
function myobject(){
this.name="name";
this.age="age";
this.sex="sex";
this.func=function(){
}
}
var myobj=new myobject();
for(var i in myobj){
alert(myobj[i]);
}

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
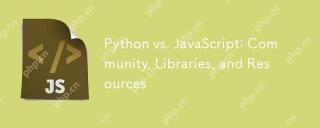
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
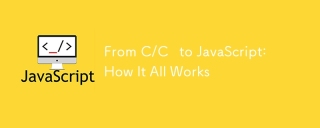
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
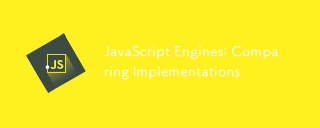
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
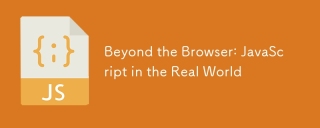
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
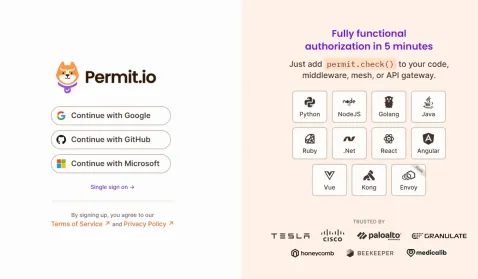
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
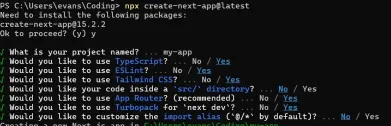
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
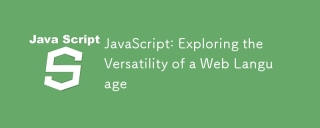
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
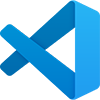
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.